通过正则表达式进行模式匹配、使用字符串的内置方法、利用第三方库(如NLTK和spaCy)进行文本分析、结合机器学习模型进行自然语言处理,这些都是Python中常用的文本定位方法。接下来,我将详细展开其中一种方法——使用正则表达式进行模式匹配。
使用正则表达式进行模式匹配
正则表达式(Regular Expressions,简称regex)是一种强大的文本搜索与匹配工具。Python的re
模块提供了对正则表达式的支持,使得我们可以通过定义匹配模式来定位文本中的特定内容。
基本概念和语法
正则表达式由普通字符(如字符a到z)和特殊字符(如元字符)组成。下面是一些常用的正则表达式语法:
.
匹配除换行符外的任何字符^
匹配字符串的开头$
匹配字符串的结尾*
匹配前面的字符0次或多次+
匹配前面的字符1次或多次?
匹配前面的字符0次或1次{n}
匹配前面的字符恰好n次{n,}
匹配前面的字符至少n次{n,m}
匹配前面的字符至少n次,但不超过m次[]
匹配括号内的任意字符|
表示或关系
示例:在文本中查找电子邮件地址
假设我们需要在一段文本中查找所有的电子邮件地址,可以使用以下正则表达式:
import re
text = "Please contact us at info@example.com for further information. Alternatively, you can reach out to support@domain.net."
定义匹配电子邮件地址的正则表达式
email_pattern = r'[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+'
使用re.findall()查找所有匹配的电子邮件地址
emails = re.findall(email_pattern, text)
print("Found emails:", emails)
这段代码将输出:
Found emails: ['info@example.com', 'support@domain.net']
使用正则表达式进行复杂文本匹配
通过组合不同的正则表达式语法,可以实现更加复杂的文本匹配需求。以下是一些示例:
- 匹配电话号码:
phone_pattern = r'\(\d{3}\) \d{3}-\d{4}'
text = "Call us at (123) 456-7890 or (987) 654-3210."
phones = re.findall(phone_pattern, text)
print("Found phone numbers:", phones)
- 匹配日期格式:
date_pattern = r'\b\d{2}/\d{2}/\d{4}\b'
text = "The event is scheduled on 12/25/2023 and the registration deadline is 11/30/2023."
dates = re.findall(date_pattern, text)
print("Found dates:", dates)
- 匹配URL:
url_pattern = r'https?://(?:[-\w.]|(?:%[\da-fA-F]{2}))+'
text = "Visit our website at https://www.example.com or follow us at http://blog.example.com."
urls = re.findall(url_pattern, text)
print("Found URLs:", urls)
使用字符串的内置方法
Python字符串类提供了一些内置方法,可以帮助我们进行简单的文本定位和处理。例如:
- find()方法: 返回子字符串在字符串中的最低索引,如果未找到则返回-1。
text = "Hello, welcome to the world of Python."
index = text.find("Python")
print("Index of 'Python':", index)
- index()方法: 与find()类似,但如果子字符串未找到则会引发ValueError异常。
try:
index = text.index("Python")
print("Index of 'Python':", index)
except ValueError:
print("Substring not found.")
- split()方法: 将字符串按指定分隔符分割成列表。
text = "apple,banana,cherry"
fruits = text.split(",")
print("List of fruits:", fruits)
- replace()方法: 将字符串中的子字符串替换为另一个子字符串。
text = "I love Python."
new_text = text.replace("Python", "programming")
print("Updated text:", new_text)
利用第三方库进行文本分析
Python中有许多强大的第三方库,可以帮助我们进行更加复杂的文本定位和分析任务。其中,NLTK和spaCy是两个非常流行的自然语言处理库。
使用NLTK进行文本分析
NLTK(Natural Language Toolkit)是一个用于处理人类语言数据的库。它提供了多种工具和资源,可以用于文本标注、语法解析、情感分析等任务。
- 分词:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
text = "NLTK is a powerful library for natural language processing."
tokens = word_tokenize(text)
print("Tokens:", tokens)
- 词性标注:
from nltk import pos_tag
tokens = word_tokenize(text)
pos_tags = pos_tag(tokens)
print("Part-of-speech tags:", pos_tags)
- 命名实体识别:
nltk.download('maxent_ne_chunker')
nltk.download('words')
from nltk import ne_chunk
chunks = ne_chunk(pos_tags)
print("Named entities:", chunks)
使用spaCy进行文本分析
spaCy是另一个流行的自然语言处理库,提供了高性能的文本处理工具,并支持多种语言。
- 加载模型:
import spacy
nlp = spacy.load("en_core_web_sm")
- 分词和词性标注:
doc = nlp("spaCy is an advanced library for NLP.")
for token in doc:
print(token.text, token.pos_, token.dep_)
- 命名实体识别:
for ent in doc.ents:
print(ent.text, ent.label_)
结合机器学习模型进行自然语言处理
除了使用正则表达式和第三方库,结合机器学习模型进行文本处理也是一种常见的方法。通过训练模型,我们可以实现更加智能和复杂的文本定位任务。
使用scikit-learn进行文本分类
scikit-learn是一个流行的机器学习库,提供了多种算法和工具,可以用于文本分类、回归、聚类等任务。
- 文本预处理:
from sklearn.feature_extraction.text import TfidfVectorizer
corpus = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?"
]
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(corpus)
- 训练分类模型:
from sklearn.naive_bayes import MultinomialNB
from sklearn.model_selection import train_test_split
y = [0, 1, 2, 0] # 类别标签
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.25, random_state=42)
clf = MultinomialNB()
clf.fit(X_train, y_train)
- 进行预测:
predicted = clf.predict(X_test)
print("Predicted labels:", predicted)
使用深度学习进行文本处理
深度学习在自然语言处理领域取得了显著的进展,尤其是基于神经网络的方法,如LSTM和Transformer架构。TensorFlow和PyTorch是两个流行的深度学习框架,广泛用于文本处理任务。
- 使用TensorFlow进行文本分类:
import tensorflow as tf
from tensorflow.keras.layers import Embedding, LSTM, Dense
from tensorflow.keras.models import Sequential
model = Sequential([
Embedding(input_dim=5000, output_dim=64),
LSTM(128),
Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
- 训练模型:
# 假设X_train和y_train是预处理后的训练数据
model.fit(X_train, y_train, epochs=5, batch_size=32)
- 进行预测:
predictions = model.predict(X_test)
print("Predictions:", predictions)
通过上述不同的方法,可以在Python中实现文本定位和处理任务。选择哪种方法取决于具体的应用场景和需求。正则表达式适合简单的模式匹配任务,而第三方库和机器学习模型则适合更复杂和智能的文本处理任务。无论选择哪种方法,都需要结合实际需求和数据特点进行综合考虑。
相关问答FAQs:
如何在Python中使用文本定位查找特定信息?
在Python中,可以使用正则表达式(re模块)或字符串方法(如find、index等)来进行文本定位。通过这些工具,你可以精准地查找特定的单词或短语,并获取其在文本中的位置。
文本定位时,哪些库或工具可以帮助我提高效率?
除了内置的字符串处理功能,Python还提供了一些强大的库,如BeautifulSoup用于解析HTML和XML文档,以及pandas用于处理表格数据。这些工具可以帮助你在复杂的文本结构中快速定位所需的信息。
如何处理大型文本文件中的文本定位问题?
在处理大型文本文件时,可以使用逐行读取的方法来降低内存消耗。结合使用生成器和正则表达式,可以有效地定位和提取所需的信息,而不需要将整个文件加载到内存中。使用这种方法可以提高效率,并减少程序的运行时间。
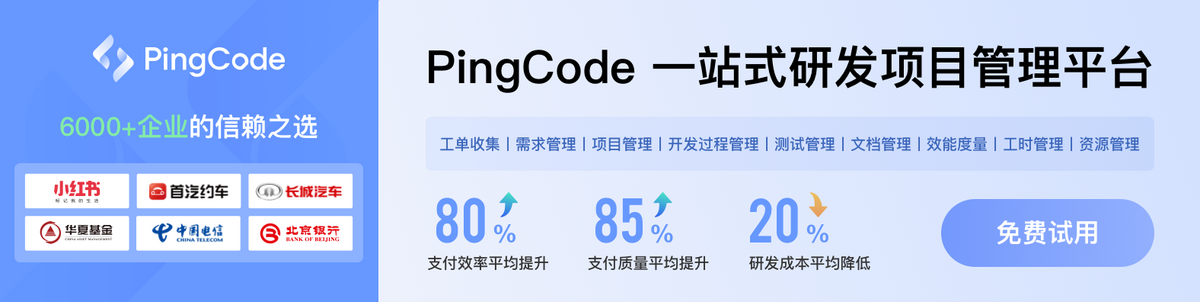