Python创建JSON文件的方法有多种,常用的有使用内置的json
库、使用第三方库如ujson
等。 首先,了解如何使用内置的json
库是非常重要的,因为它是最常用且方便的方法。使用内置的json
库、使用json.dumps
或json.dump
方法、处理JSON序列化和反序列化。
详细描述:使用内置的json
库是创建JSON文件的最常见方法。你可以通过json.dumps
方法将Python对象转换为JSON字符串,然后将其写入文件;或者更直接地使用json.dump
方法将Python对象写入文件。以下是一个示例代码:
import json
创建一个Python字典
data = {
"name": "John Doe",
"age": 30,
"city": "New York",
"is_student": False,
"courses": ["Math", "Science", "English"]
}
将Python字典转换为JSON并写入文件
with open('data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
这个示例展示了如何使用json.dump
方法将字典对象直接写入JSON文件,并且使用indent
参数使JSON文件更具可读性。
一、内置json
库的使用
1、json.dumps与json.dump的区别
json.dumps
和json.dump
都是用于将Python对象转换为JSON格式的函数,但它们有一些重要的区别。json.dumps
用于将Python对象转换为JSON字符串,而json.dump
则直接将Python对象转换并写入文件。
以下是使用json.dumps
的示例:
import json
data = {
"name": "John Doe",
"age": 30,
"city": "New York",
"is_student": False,
"courses": ["Math", "Science", "English"]
}
将Python字典转换为JSON字符串
json_string = json.dumps(data, indent=4)
print(json_string)
在这个示例中,json.dumps
将Python字典转换为JSON字符串并打印出来。你可以将这个字符串写入文件。
而使用json.dump
直接将数据写入文件的示例如下:
import json
data = {
"name": "John Doe",
"age": 30,
"city": "New York",
"is_student": False,
"courses": ["Math", "Science", "English"]
}
将Python字典转换为JSON并写入文件
with open('data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
2、处理JSON序列化和反序列化
在处理复杂数据类型时,例如日期时间对象或自定义类对象,可能需要自定义序列化和反序列化方法。你可以通过定义自定义的编码器和解码器来实现这一点。
例如,自定义日期时间对象的序列化:
import json
from datetime import datetime
class DateTimeEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, datetime):
return obj.isoformat()
return super().default(obj)
data = {
"name": "John Doe",
"timestamp": datetime.now()
}
使用自定义的编码器进行序列化
json_string = json.dumps(data, cls=DateTimeEncoder, indent=4)
print(json_string)
在这个示例中,我们定义了一个DateTimeEncoder
类来处理日期时间对象的序列化。使用cls
参数,我们可以在调用json.dumps
时指定自定义的编码器。
3、读取和解析JSON文件
除了创建JSON文件,Python还可以轻松地读取和解析JSON文件。使用json.load
可以将JSON文件内容解析为Python对象。例如:
import json
读取JSON文件并解析
with open('data.json', 'r') as json_file:
data = json.load(json_file)
print(data)
这个示例展示了如何读取JSON文件并解析为Python字典对象。
二、使用第三方库如ujson
除了内置的json
库,Python还有一些第三方库可以用于处理JSON数据,其中一个流行的库是ujson
(UltraJSON)。它比内置的json
库更快,适用于处理大规模JSON数据。
1、安装ujson
你可以使用pip
安装ujson
:
pip install ujson
2、使用ujson
创建和读取JSON文件
ujson
的API与内置的json
库非常相似,因此很容易上手。以下是一个使用ujson
创建JSON文件的示例:
import ujson
data = {
"name": "John Doe",
"age": 30,
"city": "New York",
"is_student": False,
"courses": ["Math", "Science", "English"]
}
将Python字典转换为JSON并写入文件
with open('data.json', 'w') as json_file:
ujson.dump(data, json_file, indent=4)
同样,读取JSON文件的示例如下:
import ujson
读取JSON文件并解析
with open('data.json', 'r') as json_file:
data = ujson.load(json_file)
print(data)
三、JSON的基本概念和应用场景
1、JSON的基本概念
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,易于人阅读和编写,同时也易于机器解析和生成。JSON使用键值对(key-value pairs)的形式来表示数据,通常用于客户端和服务器之间的数据传输。
2、JSON的应用场景
JSON被广泛应用于以下几个场景:
-
API通信:在Web开发中,JSON是API通信的主要数据格式。客户端和服务器通常使用JSON来传递数据,因为它易于解析和生成。
-
配置文件:许多应用程序使用JSON文件作为配置文件,因为JSON格式简单、易读且易于解析。
-
数据存储:一些NoSQL数据库(如MongoDB)使用JSON格式来存储数据,允许开发人员以灵活的方式操作数据。
-
数据交换:JSON常用于不同系统之间的数据交换,因为它具有跨平台的兼容性。
四、处理大型JSON文件的技巧
在处理大型JSON文件时,可能需要一些技巧来提高效率和性能。以下是一些常见的方法:
1、逐行解析
对于非常大的JSON文件,逐行解析可以节省内存并提高效率。你可以使用json
库的JSONDecoder
类来逐行解析JSON文件。
import json
def parse_large_json(file_path):
with open(file_path, 'r') as json_file:
decoder = json.JSONDecoder()
buffer = ''
for line in json_file:
buffer += line.strip()
try:
while buffer:
obj, idx = decoder.raw_decode(buffer)
yield obj
buffer = buffer[idx:].lstrip()
except json.JSONDecodeError:
continue
使用逐行解析器读取大型JSON文件
for item in parse_large_json('large_data.json'):
print(item)
这个示例展示了如何逐行解析大型JSON文件并生成Python对象。
2、使用内存映射
在处理非常大的JSON文件时,可以使用内存映射(memory mapping)来提高性能。内存映射允许你将文件的一部分映射到内存中,从而避免将整个文件加载到内存中。
import json
import mmap
def parse_large_json_with_mmap(file_path):
with open(file_path, 'r+') as json_file:
mmapped_file = mmap.mmap(json_file.fileno(), 0)
decoder = json.JSONDecoder()
buffer = ''
for line in mmapped_file:
buffer += line.strip().decode('utf-8')
try:
while buffer:
obj, idx = decoder.raw_decode(buffer)
yield obj
buffer = buffer[idx:].lstrip()
except json.JSONDecodeError:
continue
mmapped_file.close()
使用内存映射解析大型JSON文件
for item in parse_large_json_with_mmap('large_data.json'):
print(item)
这个示例展示了如何使用内存映射来解析大型JSON文件,从而提高性能。
五、处理嵌套和复杂JSON数据
在实际应用中,你可能会遇到嵌套和复杂的JSON数据结构。以下是一些处理嵌套和复杂JSON数据的技巧:
1、递归解析嵌套数据
对于嵌套的JSON数据,可以使用递归函数来解析。例如:
def parse_nested_json(data):
if isinstance(data, dict):
for key, value in data.items():
print(f"Key: {key}")
parse_nested_json(value)
elif isinstance(data, list):
for item in data:
parse_nested_json(item)
else:
print(f"Value: {data}")
nested_data = {
"name": "John Doe",
"details": {
"age": 30,
"address": {
"city": "New York",
"zip": "10001"
}
},
"courses": ["Math", "Science", "English"]
}
递归解析嵌套JSON数据
parse_nested_json(nested_data)
这个示例展示了如何使用递归函数解析嵌套的JSON数据结构。
2、使用pandas处理复杂JSON数据
pandas
是一个强大的数据分析库,能够处理复杂的JSON数据。以下是一个使用pandas
解析复杂JSON数据的示例:
import pandas as pd
complex_data = [
{
"name": "John Doe",
"age": 30,
"courses": [
{"name": "Math", "score": 90},
{"name": "Science", "score": 85}
]
},
{
"name": "Jane Smith",
"age": 25,
"courses": [
{"name": "Math", "score": 95},
{"name": "English", "score": 88}
]
}
]
将复杂JSON数据转换为DataFrame
df = pd.json_normalize(complex_data, 'courses', ['name', 'age'])
print(df)
这个示例展示了如何使用pandas.json_normalize
方法将复杂的嵌套JSON数据转换为DataFrame,以便进行数据分析和处理。
六、JSON文件的常见操作
1、更新JSON文件
在某些情况下,你可能需要更新现有的JSON文件。以下是一个示例,展示了如何读取、修改和保存JSON文件:
import json
读取JSON文件
with open('data.json', 'r') as json_file:
data = json.load(json_file)
修改数据
data['age'] = 31
data['courses'].append('History')
保存修改后的数据
with open('data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
这个示例展示了如何读取JSON文件、修改其中的数据并保存修改后的数据。
2、删除JSON文件中的数据
有时你可能需要删除JSON文件中的某些数据。以下是一个示例,展示了如何删除JSON文件中的某些键:
import json
读取JSON文件
with open('data.json', 'r') as json_file:
data = json.load(json_file)
删除某些键
del data['is_student']
保存修改后的数据
with open('data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
这个示例展示了如何读取JSON文件、删除某些键并保存修改后的数据。
七、JSON文件的最佳实践
在处理JSON文件时,遵循一些最佳实践可以帮助你编写更加高效和可维护的代码:
1、使用上下文管理器
使用上下文管理器(即with
语句)来处理文件操作,可以确保文件在使用后正确关闭,避免资源泄漏。
import json
data = {"name": "John Doe", "age": 30}
使用上下文管理器处理文件操作
with open('data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
2、使用indent
参数格式化输出
在将Python对象转换为JSON格式时,使用indent
参数可以使输出的JSON文件更具可读性。
import json
data = {"name": "John Doe", "age": 30}
使用indent参数格式化输出
json_string = json.dumps(data, indent=4)
print(json_string)
3、处理异常
在处理JSON文件时,可能会遇到各种异常情况,如文件不存在、JSON格式错误等。通过捕获和处理异常,可以提高代码的健壮性。
import json
try:
with open('data.json', 'r') as json_file:
data = json.load(json_file)
except FileNotFoundError:
print("文件未找到")
except json.JSONDecodeError:
print("JSON格式错误")
这个示例展示了如何捕获和处理文件操作和JSON解析中的异常情况。
八、总结
通过学习如何在Python中创建和处理JSON文件,你可以在实际应用中更高效地处理数据交换、配置管理和数据存储等任务。内置的json
库提供了简单而强大的工具来处理JSON数据,而第三方库如ujson
则可以在处理大规模数据时提供更高的性能。在处理复杂和嵌套JSON数据时,递归解析和使用pandas
等工具可以帮助你更轻松地处理数据。最后,遵循最佳实践可以帮助你编写更加高效和可维护的代码。希望本文能为你在实际项目中处理JSON数据提供有价值的参考。
相关问答FAQs:
如何在Python中将字典转换为JSON格式并保存到文件中?
在Python中,可以使用内置的json
模块将字典转换为JSON格式。首先,定义一个字典,然后使用json.dump()
函数将其写入文件。例如:
import json
data = {'name': 'Alice', 'age': 30, 'city': 'New York'}
with open('data.json', 'w') as json_file:
json.dump(data, json_file)
这段代码会创建一个名为data.json
的文件,并将字典内容以JSON格式保存。
如何读取JSON文件并将其转换为Python对象?
读取JSON文件也非常简单。使用json.load()
函数可以将JSON文件内容解析为Python对象。示例代码如下:
import json
with open('data.json', 'r') as json_file:
data = json.load(json_file)
print(data)
执行后,data
将包含从文件中读取的字典内容。
在Python中如何处理JSON数据的异常情况?
在处理JSON数据时,可能会遇到格式错误或编码问题。可以使用try-except
块来捕获这些异常。例如:
import json
try:
with open('data.json', 'r') as json_file:
data = json.load(json_file)
except json.JSONDecodeError:
print("文件格式错误,无法解析JSON数据。")
except FileNotFoundError:
print("指定的文件未找到。")
这种方式可以确保程序在处理JSON数据时的稳定性,避免因错误导致程序崩溃。
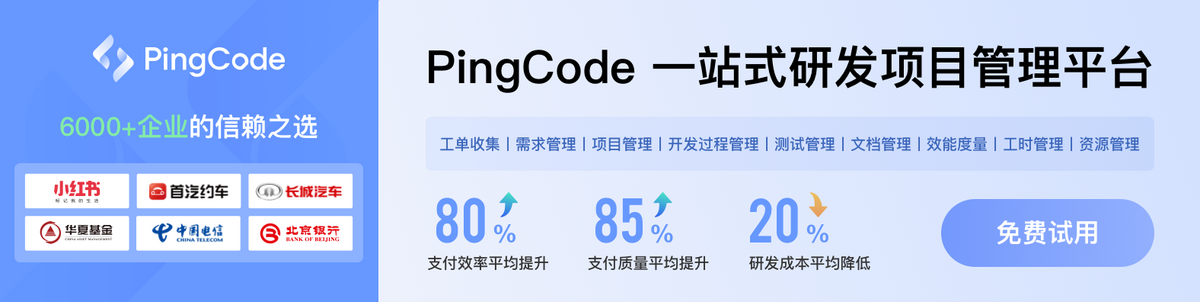