Python中消除停用词的主要方法包括使用NLTK库、使用spaCy库、手动定义停用词列表、使用gensim库。其中,使用NLTK库是一种非常流行且简单的方法。NLTK(Natural Language Toolkit)是一个强大的Python库,包含了文本处理和自然语言处理的各种工具。通过NLTK库,我们可以很方便地加载停用词列表,并在文本处理中将这些停用词移除,从而达到消除停用词的目的。
下面我将详细介绍如何使用NLTK库来消除停用词:
首先,确保您已经安装了NLTK库。可以使用以下命令来安装:
pip install nltk
接下来,下载停用词数据集。NLTK库提供了一组预定义的停用词列表,可以通过以下代码进行下载:
import nltk
nltk.download('stopwords')
下载完成后,便可以加载这些停用词并进行处理:
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
加载停用词列表
stop_words = set(stopwords.words('english'))
示例文本
text = "This is a sample sentence, showing off the stop words filtration."
分词
words = word_tokenize(text)
移除停用词
filtered_words = [word for word in words if word.lower() not in stop_words]
print("Original Text:", text)
print("Filtered Text:", " ".join(filtered_words))
一、NLTK库
NLTK库是Python中最广泛使用的自然语言处理工具之一。它提供了各种文本处理功能,包括分词、标注、语法解析、语义分析等。在NLTK库中,已经预定义了一组常见的停用词,这些停用词可以直接使用,从而简化了文本处理的过程。
- 加载停用词
要使用NLTK库中的停用词列表,首先需要导入相关模块并下载停用词数据集。以下代码展示了如何加载和使用NLTK库中的停用词列表:
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
下载停用词数据集
nltk.download('stopwords')
加载停用词列表
stop_words = set(stopwords.words('english'))
- 分词与移除停用词
在加载了停用词列表后,可以将文本进行分词,并移除其中的停用词。以下代码展示了如何进行这一过程:
# 示例文本
text = "This is a sample sentence, showing off the stop words filtration."
分词
words = word_tokenize(text)
移除停用词
filtered_words = [word for word in words if word.lower() not in stop_words]
print("Original Text:", text)
print("Filtered Text:", " ".join(filtered_words))
使用NLTK库的优势在于其简单易用和功能丰富。除了停用词处理之外,NLTK还提供了许多其他自然语言处理工具,使其成为进行文本分析和处理的强大工具。
二、spaCy库
spaCy是另一个流行的自然语言处理库,具有高效、快速和易于使用的特点。与NLTK类似,spaCy也提供了预定义的停用词列表,可以用来处理文本中的停用词。
- 安装和加载spaCy
首先,需要安装spaCy库和相关的语言模型:
pip install spacy
python -m spacy download en_core_web_sm
- 使用spaCy处理停用词
以下代码展示了如何使用spaCy库加载停用词列表,并对文本进行处理:
import spacy
加载spaCy的英语模型
nlp = spacy.load('en_core_web_sm')
示例文本
text = "This is a sample sentence, showing off the stop words filtration."
处理文本
doc = nlp(text)
移除停用词
filtered_words = [token.text for token in doc if not token.is_stop]
print("Original Text:", text)
print("Filtered Text:", " ".join(filtered_words))
spaCy库的优势在于其高效性和易用性。spaCy不仅提供了停用词处理功能,还包含了命名实体识别、依存解析等高级自然语言处理功能。
三、手动定义停用词列表
有时,您可能需要根据具体需求定义自己的停用词列表。这种方法灵活性更高,可以根据具体应用场景调整停用词列表。
- 定义停用词列表
首先,定义一个包含常见停用词的列表:
custom_stop_words = ["is", "a", "the", "of", "and", "to", "in"]
- 分词与移除停用词
使用手动定义的停用词列表进行分词和停用词移除:
from nltk.tokenize import word_tokenize
示例文本
text = "This is a sample sentence, showing off the stop words filtration."
分词
words = word_tokenize(text)
移除停用词
filtered_words = [word for word in words if word.lower() not in custom_stop_words]
print("Original Text:", text)
print("Filtered Text:", " ".join(filtered_words))
手动定义停用词列表的优势在于灵活性。可以根据具体应用场景和需求,动态调整停用词列表,避免了一些不必要的词被移除。
四、gensim库
gensim是一个专门用于主题建模和文档相似度计算的Python库。gensim也提供了停用词处理功能,可以方便地在文本处理中移除停用词。
- 安装gensim
首先,安装gensim库:
pip install gensim
- 使用gensim处理停用词
以下代码展示了如何使用gensim库加载停用词列表,并对文本进行处理:
from gensim.parsing.preprocessing import remove_stopwords
示例文本
text = "This is a sample sentence, showing off the stop words filtration."
移除停用词
filtered_text = remove_stopwords(text)
print("Original Text:", text)
print("Filtered Text:", filtered_text)
gensim库的优势在于其专注于主题建模和文档相似度计算。使用gensim库,可以方便地进行文本预处理,并将处理后的文本用于进一步的主题建模和分析。
总结
在Python中,消除停用词的方法有很多,选择适合自己需求的方法非常重要。NLTK库、spaCy库、手动定义停用词列表、gensim库都是常见的选择。NLTK库功能强大且易于使用,适合各种文本处理任务;spaCy库高效且功能丰富,适合需要快速处理大规模文本的场景;手动定义停用词列表灵活性高,适合特定需求;gensim库专注于主题建模和文档相似度计算,适合文本分析任务。
在实际应用中,可以根据具体需求选择合适的方法,并结合其他文本处理技术,提高文本处理和分析的效果。无论选择哪种方法,都需要根据具体应用场景进行调整和优化,以确保处理结果的准确性和有效性。
五、示例应用
为了更好地理解如何在实际应用中消除停用词,下面我们将展示一个完整的示例应用,结合多种方法进行文本处理。
- 加载示例文本
首先,加载一个示例文本:
example_text = """
Natural language processing (NLP) is a sub-field of artificial intelligence (AI) that focuses on the interaction between computers and humans through natural language. The ultimate goal of NLP is to enable computers to understand, interpret, and generate human languages in a way that is both meaningful and useful. NLP is used in a variety of applications, such as language translation, sentiment analysis, speech recognition, and text summarization.
"""
- 使用NLTK库处理停用词
使用NLTK库对示例文本进行处理:
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
nltk.download('punkt')
nltk.download('stopwords')
加载停用词列表
stop_words = set(stopwords.words('english'))
分词
words = word_tokenize(example_text)
移除停用词
filtered_words_nltk = [word for word in words if word.lower() not in stop_words]
print("Filtered Text using NLTK:", " ".join(filtered_words_nltk))
- 使用spaCy库处理停用词
使用spaCy库对示例文本进行处理:
import spacy
nlp = spacy.load('en_core_web_sm')
处理文本
doc = nlp(example_text)
移除停用词
filtered_words_spacy = [token.text for token in doc if not token.is_stop]
print("Filtered Text using spaCy:", " ".join(filtered_words_spacy))
- 手动定义停用词列表处理
使用手动定义的停用词列表对示例文本进行处理:
custom_stop_words = ["is", "a", "the", "of", "and", "to", "in", "that", "on", "between", "through"]
分词
words = word_tokenize(example_text)
移除停用词
filtered_words_custom = [word for word in words if word.lower() not in custom_stop_words]
print("Filtered Text using Custom Stop Words:", " ".join(filtered_words_custom))
- 使用gensim库处理停用词
使用gensim库对示例文本进行处理:
from gensim.parsing.preprocessing import remove_stopwords
移除停用词
filtered_text_gensim = remove_stopwords(example_text)
print("Filtered Text using gensim:", filtered_text_gensim)
六、总结与对比
通过以上示例应用,我们可以看到不同方法在处理停用词时的效果和差异。具体来说:
- NLTK库:提供了丰富的停用词列表,处理结果较为完整,但需要额外下载数据集。
- spaCy库:高效且功能强大,处理结果与NLTK类似,但处理速度更快。
- 手动定义停用词列表:灵活性高,可以根据具体需求调整停用词列表,适合特定应用场景。
- gensim库:专注于主题建模和文档相似度计算,处理结果简洁高效,但功能相对单一。
在实际应用中,可以根据具体需求选择合适的方法。例如,对于一般的文本处理任务,可以使用NLTK或spaCy库;对于特定需求,可以手动定义停用词列表;对于主题建模和文本分析任务,可以使用gensim库。
无论选择哪种方法,消除停用词都是文本预处理中的重要一步,有助于提高文本分析和处理的效果。通过合理选择和使用这些工具,可以更好地完成文本处理任务,达到预期的效果。
相关问答FAQs:
如何识别和处理停用词?
停用词是指在文本中出现频率高但对内容意义贡献较小的词汇,如“的”、“是”、“在”等。可以使用Python的自然语言处理库(如NLTK、spaCy)来识别和处理这些词。通过加载预定义的停用词列表,用户可以轻松过滤掉文本中的停用词,从而提高文本分析的效率。
在Python中有哪些库可以帮助消除停用词?
Python中有多种库可以用于消除停用词。最常用的包括NLTK(自然语言工具包)和spaCy。NLTK提供了丰富的停用词列表和处理工具,用户可以自定义和扩展停用词。而spaCy则通过其高效的处理速度和简洁的API,使得停用词处理变得更加便捷。
消除停用词后,文本的分析效果如何?
去除停用词后,文本的分析效果通常会显著提升。停用词的消除可以帮助突出文本中更有意义的关键词,从而改善信息检索、情感分析和主题建模等任务的准确性。此过程有助于减少数据的噪声,使得后续的分析和机器学习算法能够更有效地捕捉到文本的核心信息。
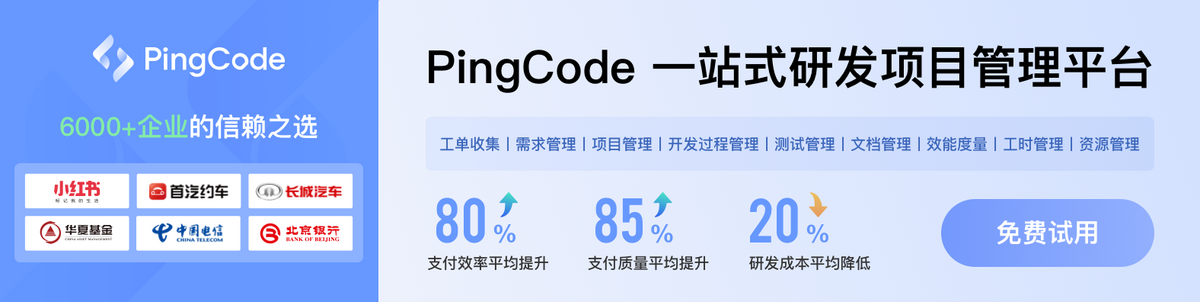