编写Python模块的关键步骤包括:定义功能、组织代码、使用命名空间、添加文档字符串和测试模块。其中,组织代码是确保模块易于理解和维护的关键。
一、定义功能
在编写Python模块之前,首先需要明确模块的功能和用途。考虑模块将解决的问题、提供的功能以及如何与其他模块或应用程序交互。这一步骤有助于确定模块的结构和内容。
示例:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
二、组织代码
组织代码是指将代码分成独立且相关的部分,使其更易于理解和维护。这包括将函数和类分组、使用适当的命名空间以及确保代码具有良好的可读性。
示例:
class Calculator:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
三、使用命名空间
命名空间有助于避免命名冲突,特别是在大型项目中。通过将代码组织到类或模块中,可以创建独立的命名空间。
示例:
class MathOperations:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
四、添加文档字符串
文档字符串是对代码的说明,帮助其他开发者理解代码的功能和使用方法。每个模块、类和函数都应该包含文档字符串。
示例:
class MathOperations:
"""
This class provides basic mathematical operations.
"""
def add(self, a, b):
"""
Add two numbers.
Parameters:
a (int, float): The first number.
b (int, float): The second number.
Returns:
int, float: The sum of the two numbers.
"""
return a + b
def subtract(self, a, b):
"""
Subtract two numbers.
Parameters:
a (int, float): The first number.
b (int, float): The second number.
Returns:
int, float: The difference between the two numbers.
"""
return a - b
五、测试模块
测试是确保模块功能正确的关键步骤。编写单元测试可以帮助发现和修复错误,并确保代码在修改后仍然正确。
示例:
import unittest
from math_operations import MathOperations
class TestMathOperations(unittest.TestCase):
def setUp(self):
self.calc = MathOperations()
def test_add(self):
self.assertEqual(self.calc.add(2, 3), 5)
self.assertEqual(self.calc.add(-1, 1), 0)
def test_subtract(self):
self.assertEqual(self.calc.subtract(5, 3), 2)
self.assertEqual(self.calc.subtract(3, 5), -2)
if __name__ == '__main__':
unittest.main()
模块的命名和结构
模块的命名和结构对代码的可读性和可维护性有很大影响。选择清晰、描述性的名称,并遵循Python的命名约定。模块名称应全部小写,并使用下划线分隔单词。
示例:
# 文件名:math_operations.py
class MathOperations:
pass
导入和使用模块
在使用模块时,可以使用import语句将模块导入到其他脚本或模块中。Python提供了多种导入方式,如导入整个模块、导入特定函数或类等。
示例:
# 导入整个模块
import math_operations
calc = math_operations.MathOperations()
print(calc.add(2, 3))
导入特定类
from math_operations import MathOperations
calc = MathOperations()
print(calc.add(2, 3))
模块的发布和分发
当模块开发完成并经过测试后,可以考虑将其发布和分发给其他开发者使用。Python提供了多种分发方法,包括手动分发和使用Python包管理工具(如pip)。
示例:
# setup.py
from setuptools import setup, find_packages
setup(
name='math_operations',
version='0.1',
packages=find_packages(),
description='A module for basic mathematical operations',
author='Your Name',
author_email='your.email@example.com',
url='https://github.com/yourusername/math_operations',
)
模块的优化和维护
模块的优化和维护是一个持续的过程。在使用和分发模块后,可能会发现一些需要改进的地方。通过定期审查和更新代码,可以提高模块的性能和可用性。
示例:
# 优化代码
class MathOperations:
def add(self, *args):
return sum(args)
def subtract(self, a, b):
return a - b
处理错误和异常
在编写模块时,处理错误和异常是确保代码健壮性的关键。通过捕获和处理异常,可以提高模块的可靠性,并提供有意义的错误信息。
示例:
class MathOperations:
def add(self, a, b):
try:
return a + b
except TypeError:
raise ValueError("Both arguments must be numbers")
def subtract(self, a, b):
try:
return a - b
except TypeError:
raise ValueError("Both arguments must be numbers")
模块的文档和示例
提供详细的文档和示例,可以帮助其他开发者快速理解和使用模块。文档应包括模块的功能描述、使用方法和示例代码。
示例:
# MathOperations Module
## Description
This module provides basic mathematical operations.
## Installation
```bash
pip install math_operations
Usage
from math_operations import MathOperations
calc = MathOperations()
print(calc.add(2, 3))
print(calc.subtract(5, 3))
## 模块的版本控制
使用版本控制系统(如Git)管理模块的开发和维护,可以方便地跟踪代码的变化,并与其他开发者协作。
<strong>示例:</strong>
```bash
初始化Git仓库
git init
添加文件到Git仓库
git add .
提交更改
git commit -m "Initial commit"
推送到远程仓库
git remote add origin https://github.com/yourusername/math_operations.git
git push -u origin master
模块的依赖管理
管理模块的依赖是确保模块在不同环境中正常工作的关键。使用依赖管理工具(如pipenv或poetry)可以简化依赖的安装和管理。
示例:
# 使用pipenv管理依赖
pipenv install
使用poetry管理依赖
poetry install
模块的测试和持续集成
通过编写自动化测试和设置持续集成(CI)管道,可以确保模块在开发和维护过程中始终保持高质量。使用CI工具(如GitHub Actions或Travis CI)可以自动运行测试,并在代码更改时提供反馈。
示例:
# GitHub Actions配置文件(.github/workflows/ci.yml)
name: CI
on:
push:
branches:
- master
pull_request:
branches:
- master
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.8
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
python -m unittest discover
模块的性能优化
在某些情况下,性能是模块的关键因素。通过分析和优化代码,可以提高模块的运行速度和效率。使用性能分析工具(如cProfile或line_profiler)可以帮助识别和优化性能瓶颈。
示例:
import cProfile
import pstats
from math_operations import MathOperations
calc = MathOperations()
def test_add():
for _ in range(100000):
calc.add(1, 2)
cProfile.run('test_add()', 'restats')
p = pstats.Stats('restats')
p.sort_stats('cumulative').print_stats(10)
模块的安全性
确保模块的安全性是开发过程中的一个重要方面。通过审查代码、使用静态分析工具(如Bandit或SonarQube)和遵循安全编码实践,可以提高模块的安全性。
示例:
# 使用Bandit进行静态分析
bandit -r math_operations.py
模块的国际化和本地化
如果模块需要支持多种语言,可以考虑进行国际化和本地化。使用gettext库可以简化字符串的翻译和管理。
示例:
import gettext
_ = gettext.gettext
class MathOperations:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
def main():
gettext.install('math_operations', localedir='locale')
print(_("Addition: "), MathOperations().add(1, 2))
print(_("Subtraction: "), MathOperations().subtract(2, 1))
if __name__ == '__main__':
main()
模块的元数据和包管理
在发布和分发模块时,添加模块的元数据可以帮助用户了解模块的信息。使用setup.py文件可以定义模块的名称、版本、作者、描述等信息。
示例:
# setup.py
from setuptools import setup, find_packages
setup(
name='math_operations',
version='0.1',
packages=find_packages(),
description='A module for basic mathematical operations',
author='Your Name',
author_email='your.email@example.com',
url='https://github.com/yourusername/math_operations',
classifiers=[
'Programming Language :: Python :: 3',
'License :: OSI Approved :: MIT License',
'Operating System :: OS Independent',
],
)
模块的社区和支持
建立模块的社区和提供支持可以帮助用户解决问题,并推动模块的发展。通过创建文档、示例代码、FAQ和讨论论坛,可以提供有价值的资源和支持。
示例:
# MathOperations Module
## Community and Support
Join our community on [GitHub](https://github.com/yourusername/math_operations) to report issues, ask questions, and contribute to the development of the module.
## FAQ
<strong>Q: How do I install the module?</strong>
A: Use the following command to install the module:
```bash
pip install math_operations
Q: How do I report a bug?
A: Open an issue on our GitHub repository with a detailed description of the bug.
通过以上步骤和实践,您可以编写、组织、测试、发布和维护一个高质量的Python模块。无论是简单的工具函数还是复杂的库,遵循这些最佳实践可以帮助您创建高效、可维护和可扩展的模块。
相关问答FAQs:
如何开始创建一个Python模块?
创建Python模块的第一步是定义一个Python文件,其中包含您希望重用的功能或类。文件名应以.py
结尾,并且可以包含函数、类和变量等。为了使模块可用,您只需将此文件放在您的项目目录中,或确保其路径在Python的搜索路径中。使用import
语句即可在其他Python文件中引入该模块。
Python模块的最佳实践是什么?
在编写Python模块时,遵循一些最佳实践会使您的代码更加可读和可维护。首先,确保模块的功能单一,避免将多个不相关的功能放入一个模块中。其次,使用有意义的命名,确保函数和类的名称能清晰地描述其功能。此外,添加适当的文档字符串(docstrings)为每个函数和类提供说明,可以帮助用户理解您的模块的用法。
如何管理Python模块的依赖关系?
管理模块的依赖关系是确保代码能够顺利运行的重要步骤。可以使用requirements.txt
文件列出项目所需的所有外部库和模块版本。在开发过程中,使用虚拟环境(如venv
或conda
)可以帮助隔离项目依赖,避免不同项目间的冲突。通过pip install -r requirements.txt
命令,可以轻松安装所需的依赖。
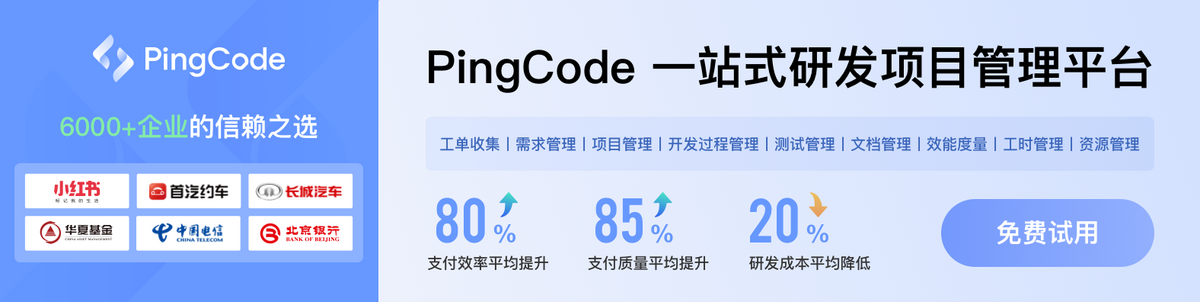