Python限制操作次数的方法包括使用循环计数器、设置函数调用次数限制、使用装饰器、利用类属性等。 可以通过设定计数器、使用装饰器,或者利用类属性来限制某个操作的次数。 其中,利用装饰器的方法非常灵活且易于维护,只需在需要限制次数的函数上添加装饰器即可。
一、使用循环计数器
循环计数器是最简单也是最直观的方法之一。可以通过一个计数器来跟踪某个操作的执行次数,并在达到预设次数后停止该操作。
示例代码:
MAX_ATTEMPTS = 5
for attempt in range(MAX_ATTEMPTS):
print(f"Attempt {attempt + 1}")
# 这里放置需要限制操作次数的代码块
这种方法适用于循环结构中需要限制操作次数的场景。
二、设置函数调用次数限制
在某些情况下,可能需要限制函数的调用次数。可以通过在函数内部维护一个计数器来实现这一点。
示例代码:
def limited_function(max_calls):
calls = 0
def inner_function():
nonlocal calls
if calls >= max_calls:
raise Exception("Function call limit exceeded")
calls += 1
print(f"Function called {calls} times")
return inner_function
limited_func = limited_function(3)
limited_func()
limited_func()
limited_func()
limited_func() # 这次调用会抛出异常
这种方法适用于需要限制某个函数调用次数的场景。
三、使用装饰器
装饰器是一种非常强大的工具,能够为函数添加额外的功能。可以利用装饰器来限制函数的调用次数。
示例代码:
def limit_calls(max_calls):
def decorator(func):
calls = 0
def wrapper(*args, kwargs):
nonlocal calls
if calls >= max_calls:
raise Exception("Function call limit exceeded")
calls += 1
return func(*args, kwargs)
return wrapper
return decorator
@limit_calls(3)
def my_function():
print("Function executed")
my_function()
my_function()
my_function()
my_function() # 这次调用会抛出异常
装饰器方法非常灵活,适用于需要在多个函数上应用相同限制的场景。
四、利用类属性
对于面向对象编程,可以利用类属性来限制方法的调用次数。
示例代码:
class LimitedClass:
def __init__(self, max_calls):
self.max_calls = max_calls
self.calls = 0
def limited_method(self):
if self.calls >= self.max_calls:
raise Exception("Method call limit exceeded")
self.calls += 1
print(f"Method called {self.calls} times")
obj = LimitedClass(3)
obj.limited_method()
obj.limited_method()
obj.limited_method()
obj.limited_method() # 这次调用会抛出异常
这种方法适用于需要在类的多个实例中应用相同限制的场景。
五、使用全局变量
在某些情况下,可以使用全局变量来限制函数或方法的调用次数。
示例代码:
MAX_CALLS = 3
global_calls = 0
def global_limited_function():
global global_calls
if global_calls >= MAX_CALLS:
raise Exception("Global function call limit exceeded")
global_calls += 1
print(f"Global function called {global_calls} times")
global_limited_function()
global_limited_function()
global_limited_function()
global_limited_function() # 这次调用会抛出异常
这种方法适用于需要在整个程序范围内应用相同限制的场景。
六、使用队列
在某些高级应用场景中,可以使用队列来限制操作次数。队列可以用来跟踪操作的执行顺序和次数。
示例代码:
from collections import deque
class OperationLimiter:
def __init__(self, max_operations):
self.max_operations = max_operations
self.operations = deque(maxlen=max_operations)
def perform_operation(self):
if len(self.operations) >= self.max_operations:
raise Exception("Operation limit exceeded")
self.operations.append("Operation")
print(f"Operation performed {len(self.operations)} times")
limiter = OperationLimiter(3)
limiter.perform_operation()
limiter.perform_operation()
limiter.perform_operation()
limiter.perform_operation() # 这次调用会抛出异常
这种方法适用于需要跟踪操作执行顺序和次数的场景。
七、使用缓存
在某些情况下,可以使用缓存来限制操作次数。缓存可以用来存储操作的执行结果,并限制操作的执行次数。
示例代码:
from functools import lru_cache
@lru_cache(maxsize=3)
def cached_function(x):
print(f"Function executed with argument {x}")
return x * 2
print(cached_function(1))
print(cached_function(2))
print(cached_function(3))
print(cached_function(4)) # 这次调用会抛出缓存的结果
这种方法适用于需要缓存操作结果并限制操作执行次数的场景。
八、使用信号量
信号量是一种同步原语,可以用来限制操作的并发执行次数。
示例代码:
import threading
class SemaphoreLimiter:
def __init__(self, max_concurrent_operations):
self.semaphore = threading.Semaphore(max_concurrent_operations)
def perform_operation(self):
with self.semaphore:
print("Operation performed")
limiter = SemaphoreLimiter(3)
threads = [threading.Thread(target=limiter.perform_operation) for _ in range(5)]
for t in threads:
t.start()
for t in threads:
t.join()
这种方法适用于需要限制操作并发执行次数的场景。
九、使用时间窗口
在某些情况下,可以使用时间窗口来限制操作次数。时间窗口可以用来限制某个时间段内的操作次数。
示例代码:
import time
class TimeWindowLimiter:
def __init__(self, max_operations, time_window):
self.max_operations = max_operations
self.time_window = time_window
self.operations = []
def perform_operation(self):
current_time = time.time()
self.operations = [t for t in self.operations if current_time - t < self.time_window]
if len(self.operations) >= self.max_operations:
raise Exception("Operation limit exceeded in time window")
self.operations.append(current_time)
print(f"Operation performed {len(self.operations)} times in time window")
limiter = TimeWindowLimiter(3, 10) # 10秒内最多3次操作
limiter.perform_operation()
time.sleep(1)
limiter.perform_operation()
time.sleep(1)
limiter.perform_operation()
limiter.perform_operation() # 这次调用会抛出异常
这种方法适用于需要限制某个时间段内操作次数的场景。
十、使用令牌桶算法
令牌桶算法是一种流量控制算法,可以用来限制操作的执行速率。
示例代码:
import time
import threading
class TokenBucket:
def __init__(self, capacity, refill_rate):
self.capacity = capacity
self.refill_rate = refill_rate
self.tokens = capacity
self.lock = threading.Lock()
self.last_refill = time.time()
def acquire(self):
with self.lock:
current_time = time.time()
elapsed_time = current_time - self.last_refill
self.tokens = min(self.capacity, self.tokens + elapsed_time * self.refill_rate)
self.last_refill = current_time
if self.tokens < 1:
raise Exception("Token bucket is empty")
self.tokens -= 1
print(f"Token acquired, remaining tokens: {self.tokens}")
bucket = TokenBucket(3, 1) # 容量为3,填充速率为1个令牌/秒
bucket.acquire()
bucket.acquire()
bucket.acquire()
bucket.acquire() # 这次调用会抛出异常
time.sleep(1)
bucket.acquire()
这种方法适用于需要限制操作执行速率的场景。
总结
通过上述十种方法,可以在不同的场景下限制操作次数。具体选择哪种方法取决于实际需求和应用场景。在编写代码时,应该根据具体情况选择最合适的方法,以确保代码的可读性和维护性。
限制操作次数的实现方法多种多样,通过合理选择和组合这些方法,可以有效地控制代码的执行次数,避免潜在的资源浪费和性能问题。
相关问答FAQs:
如何在Python中实现操作次数的限制?
在Python中,可以通过使用计数器来限制特定操作的次数。可以创建一个函数,在每次调用时增加计数器,当计数器达到设定的最大值时,函数将不再执行操作。例如,可以使用一个全局变量或类属性来跟踪调用次数,并在达到限制后抛出异常或返回特定消息。
如何在Python中利用装饰器限制函数调用次数?
装饰器是Python中的一种强大功能,可以用来限制函数的调用次数。通过定义一个装饰器,可以包装原始函数,并在每次调用时检查调用次数。如果超出限制,装饰器可以阻止函数执行并返回相应的提示信息。这样,用户可以轻松地将限制应用于多个函数。
在Python中,如何处理函数调用限制后的异常情况?
当函数调用次数达到限制时,可以设计一个机制来处理异常情况。例如,可以使用try-except语句来捕获自定义异常,并根据需要记录日志或提示用户。此外,可以提供重置计数的功能,以便在某些条件下重新允许调用操作。这样,用户体验将更加友好。
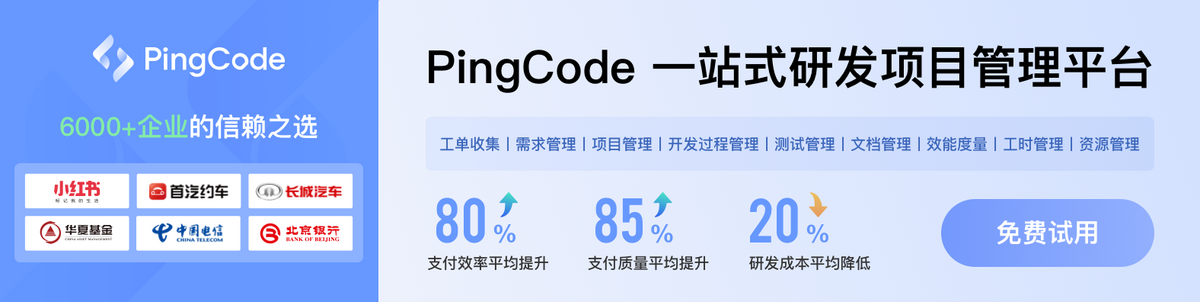