要用Python计算利润,你需要了解一些基本的财务概念,并使用Python编程语言来处理这些数据。计算利润的基本公式是:利润 = 总收入 – 总成本。在实际应用中,你可能会需要处理更多的细节,比如不同类型的成本、税收、折旧等。以下是一些具体步骤和示例代码来帮助你实现这一目标。
一、定义总收入和总成本
首先,你需要定义总收入和总成本。总收入是你通过销售产品或服务获得的总金额。总成本包括生产这些产品或服务的所有费用,如材料成本、劳动力成本、运输成本等。
# 定义总收入和总成本
total_revenue = 100000 # 总收入
total_cost = 75000 # 总成本
二、计算基本利润
使用基本公式来计算利润:
# 计算利润
profit = total_revenue - total_cost
print(f"基本利润: {profit}")
三、考虑额外的成本和收益
在实际情况中,你可能需要考虑额外的成本和收益,比如折旧、税收、利息等。以下是一个更复杂的示例:
# 定义额外的成本和收益
depreciation = 5000 # 折旧费用
tax = 10000 # 税收费用
interest = 2000 # 利息费用
other_income = 3000 # 其他收入
计算净利润
net_profit = total_revenue - total_cost - depreciation - tax - interest + other_income
print(f"净利润: {net_profit}")
四、使用函数封装计算过程
为了提高代码的可读性和复用性,可以将计算过程封装到一个函数中:
def calculate_profit(total_revenue, total_cost, depreciation=0, tax=0, interest=0, other_income=0):
return total_revenue - total_cost - depreciation - tax - interest + other_income
调用函数计算净利润
net_profit = calculate_profit(100000, 75000, 5000, 10000, 2000, 3000)
print(f"净利润: {net_profit}")
五、处理不同类型的数据
在实际应用中,数据可能来自不同的来源,比如用户输入、文件、数据库等。以下是一个处理用户输入的示例:
# 从用户输入获取数据
total_revenue = float(input("请输入总收入: "))
total_cost = float(input("请输入总成本: "))
depreciation = float(input("请输入折旧费用: "))
tax = float(input("请输入税收费用: "))
interest = float(input("请输入利息费用: "))
other_income = float(input("请输入其他收入: "))
计算净利润
net_profit = calculate_profit(total_revenue, total_cost, depreciation, tax, interest, other_income)
print(f"净利润: {net_profit}")
六、从文件读取数据
如果你的数据存储在文件中,可以使用Python的文件操作功能读取数据:
# 读取文件数据
with open('financial_data.txt', 'r') as file:
lines = file.readlines()
total_revenue = float(lines[0].strip())
total_cost = float(lines[1].strip())
depreciation = float(lines[2].strip())
tax = float(lines[3].strip())
interest = float(lines[4].strip())
other_income = float(lines[5].strip())
计算净利润
net_profit = calculate_profit(total_revenue, total_cost, depreciation, tax, interest, other_income)
print(f"净利润: {net_profit}")
七、从数据库读取数据
如果你的数据存储在数据库中,可以使用Python的数据库连接库(如sqlite3、pymysql等)读取数据:
import sqlite3
连接到数据库
conn = sqlite3.connect('financial_data.db')
cursor = conn.cursor()
执行查询
cursor.execute("SELECT total_revenue, total_cost, depreciation, tax, interest, other_income FROM financials WHERE id=1")
data = cursor.fetchone()
关闭连接
conn.close()
提取数据
total_revenue, total_cost, depreciation, tax, interest, other_income = data
计算净利润
net_profit = calculate_profit(total_revenue, total_cost, depreciation, tax, interest, other_income)
print(f"净利润: {net_profit}")
八、使用Pandas处理数据
如果你需要处理复杂的数据集,Pandas是一个非常有用的工具。以下是一个使用Pandas读取CSV文件并计算利润的示例:
import pandas as pd
读取CSV文件
df = pd.read_csv('financial_data.csv')
计算净利润
df['net_profit'] = df['total_revenue'] - df['total_cost'] - df['depreciation'] - df['tax'] - df['interest'] + df['other_income']
输出结果
print(df[['total_revenue', 'total_cost', 'net_profit']])
九、数据可视化
为了更好地理解和展示利润数据,可以使用Matplotlib或Seaborn进行数据可视化:
import matplotlib.pyplot as plt
import seaborn as sns
创建数据
revenue_data = [100000, 120000, 130000, 110000, 105000]
cost_data = [75000, 80000, 85000, 70000, 72000]
profit_data = [r - c for r, c in zip(revenue_data, cost_data)]
创建数据框
data = pd.DataFrame({
'Revenue': revenue_data,
'Cost': cost_data,
'Profit': profit_data
})
绘制图表
sns.lineplot(data=data, palette="tab10", linewidth=2.5)
plt.title('Financial Data Over Time')
plt.xlabel('Time Period')
plt.ylabel('Amount')
plt.show()
十、自动化报告生成
你可能需要定期生成财务报告,可以使用Python生成Excel或PDF报告:
import pandas as pd
创建数据框
data = {
'Revenue': [100000, 120000, 130000, 110000, 105000],
'Cost': [75000, 80000, 85000, 70000, 72000],
'Profit': [25000, 40000, 45000, 40000, 33000]
}
df = pd.DataFrame(data)
导出到Excel
df.to_excel('financial_report.xlsx', index=False)
导出到PDF (需要安装相关库,如matplotlib和fpdf)
from fpdf import FPDF
class PDF(FPDF):
def header(self):
self.set_font('Arial', 'B', 12)
self.cell(0, 10, 'Financial Report', 0, 1, 'C')
def chapter_title(self, title):
self.set_font('Arial', 'B', 12)
self.cell(0, 10, title, 0, 1, 'L')
def chapter_body(self, body):
self.set_font('Arial', '', 12)
self.multi_cell(0, 10, body)
pdf = PDF()
pdf.add_page()
pdf.chapter_title('Financial Data Over Time')
pdf.chapter_body(df.to_string(index=False))
pdf.output('financial_report.pdf')
通过这些示例和步骤,你可以使用Python计算利润,并根据需要处理和展示你的财务数据。无论你的数据来源是用户输入、文件、数据库,还是其他形式,Python都可以提供灵活和强大的工具来帮助你完成这些任务。
相关问答FAQs:
如何使用Python编写计算利润的程序?
要计算利润,您可以创建一个简单的Python程序。首先,需要定义收入和成本的变量。可以通过输入函数让用户输入这些值,然后使用公式利润 = 收入 – 成本来计算。以下是一个简单示例代码:
收入 = float(input("请输入收入:"))
成本 = float(input("请输入成本:"))
利润 = 收入 - 成本
print("利润为:", 利润)
这个程序会提示用户输入收入和成本,并输出计算得出的利润。
在计算利润时,如何考虑税费和其他费用?
在计算利润时,应将所有相关费用纳入考虑,包括税费、运输费、折旧等。可以在计算利润的公式中加入这些费用。例如,利润可以重新定义为:利润 = 收入 – (成本 + 税费 + 其他费用)。在Python程序中,您只需添加相应的输入和计算即可。
如何在Python中处理多个产品的利润计算?
如果需要计算多个产品的利润,可以使用循环来处理。您可以创建一个列表来存储各个产品的收入和成本,并使用循环迭代每个产品进行计算。以下是一个处理多个产品的示例:
产品数量 = int(input("请输入产品数量:"))
总利润 = 0
for _ in range(产品数量):
收入 = float(input("请输入该产品的收入:"))
成本 = float(input("请输入该产品的成本:"))
总利润 += 收入 - 成本
print("总利润为:", 总利润)
这个程序将计算所有输入产品的总利润,非常适合需要处理多种产品情况的场景。
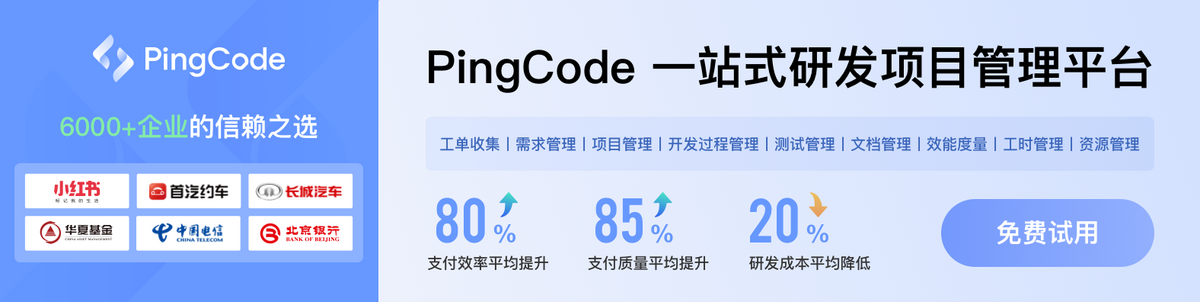