在 Python 中删除字符串中的双引号,可以使用 replace() 方法、strip() 方法、或者正则表达式等方法。其中,最常用的是 replace() 方法,它可以将字符串中的特定子字符串替换为另一个子字符串。我们可以利用它将双引号替换为空字符串,从而达到删除的效果。
例如,使用 replace() 方法删除字符串中的双引号:
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = string_with_quotes.replace('"', '')
print(string_without_quotes) # Output: He said, Hello, World!
此外,还可以使用 strip() 方法和正则表达式来删除字符串中的双引号。下面将详细介绍这些方法的使用。
一、使用 replace() 方法
replace() 方法是 Python 字符串对象的一个内置方法,可以用来将字符串中的某个子字符串替换为另一个子字符串。通过将双引号替换为空字符串,就可以实现删除双引号的效果。
# 示例代码
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = string_with_quotes.replace('"', '')
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,replace('"', '')
将字符串中的所有双引号替换为空字符串,从而删除了双引号。
二、使用 strip() 方法
strip() 方法可以删除字符串开头和结尾的指定字符。默认情况下,它删除空白字符,但我们可以指定删除的字符集。如果我们只想删除字符串开头和结尾的双引号,可以使用 strip() 方法。
# 示例代码
string_with_quotes = '"Hello, World!"'
string_without_quotes = string_with_quotes.strip('"')
print(string_without_quotes) # Output: Hello, World!
在上面的代码中,strip('"')
删除了字符串开头和结尾的双引号。
三、使用正则表达式
Python 的 re 模块提供了对正则表达式的支持。我们可以使用正则表达式来匹配和删除字符串中的双引号。
import re
示例代码
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = re.sub(r'"', '', string_with_quotes)
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,re.sub(r'"', '', string_with_quotes)
使用正则表达式将字符串中的所有双引号替换为空字符串,从而删除了双引号。
四、使用 split() 和 join() 方法
我们还可以使用 split() 和 join() 方法来删除字符串中的双引号。首先使用 split() 方法将字符串拆分成一个列表,然后使用 join() 方法将这些列表元素重新连接成一个字符串。
# 示例代码
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = ''.join(string_with_quotes.split('"'))
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,split('"')
将字符串拆分成一个列表,然后 join()
方法将列表元素重新连接成一个字符串,从而删除了双引号。
五、使用 translate() 方法
translate() 方法和 maketrans() 方法可以结合使用来删除字符串中的特定字符。首先使用 maketrans() 方法创建一个字符映射表,然后使用 translate() 方法应用该映射表。
# 示例代码
string_with_quotes = 'He said, "Hello, World!"'
translation_table = str.maketrans('', '', '"')
string_without_quotes = string_with_quotes.translate(translation_table)
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,str.maketrans('', '', '"')
创建了一个字符映射表,将双引号映射为空字符串,然后 translate()
方法应用该映射表,从而删除了双引号。
六、使用 list 和 for 循环
我们还可以使用 list 和 for 循环来逐个检查字符串中的每个字符,并构建一个不包含双引号的新字符串。
# 示例代码
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = ''.join([char for char in string_with_quotes if char != '"'])
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,列表推导式逐个检查字符串中的每个字符,并构建一个不包含双引号的列表,然后使用 join()
方法将列表元素重新连接成一个字符串。
七、使用递归函数
使用递归函数也可以删除字符串中的双引号。递归函数逐个检查字符串中的每个字符,如果当前字符是双引号,则跳过该字符并继续处理剩余的字符串。
# 示例代码
def remove_quotes(string):
if not string:
return ''
if string[0] == '"':
return remove_quotes(string[1:])
return string[0] + remove_quotes(string[1:])
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = remove_quotes(string_with_quotes)
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,递归函数 remove_quotes
逐个检查字符串中的每个字符,并构建一个不包含双引号的新字符串。
八、使用 lambda 表达式
我们还可以使用 lambda 表达式和 filter() 函数来删除字符串中的双引号。lambda 表达式是一个匿名函数,filter() 函数可以用来过滤掉不需要的字符。
# 示例代码
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = ''.join(filter(lambda x: x != '"', string_with_quotes))
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,lambda 表达式 lambda x: x != '"'
用来检查字符是否不是双引号,filter() 函数过滤掉所有双引号,然后使用 join()
方法将过滤后的字符重新连接成一个字符串。
九、使用 map() 函数
我们还可以使用 map() 函数和 filter() 函数的组合来删除字符串中的双引号。map() 函数可以用来逐个处理字符串中的每个字符,filter() 函数可以用来过滤掉不需要的字符。
# 示例代码
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = ''.join(filter(lambda x: x, map(lambda x: x if x != '"' else '', string_with_quotes)))
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,map() 函数逐个检查字符串中的每个字符,如果当前字符不是双引号,则保留该字符,否则将其替换为空字符串,然后 filter() 函数过滤掉所有空字符串,最后使用 join()
方法将过滤后的字符重新连接成一个字符串。
十、使用列表推导式
列表推导式是一种简洁的语法,可以用来创建列表。我们可以使用列表推导式来逐个检查字符串中的每个字符,并构建一个不包含双引号的新字符串。
# 示例代码
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = ''.join([char for char in string_with_quotes if char != '"'])
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,列表推导式逐个检查字符串中的每个字符,并构建一个不包含双引号的列表,然后使用 join()
方法将列表元素重新连接成一个字符串。
十一、使用生成器表达式
生成器表达式和列表推导式类似,但生成器表达式返回的是一个生成器对象,而不是一个列表。生成器表达式可以用来逐个检查字符串中的每个字符,并构建一个不包含双引号的新字符串。
# 示例代码
string_with_quotes = 'He said, "Hello, World!"'
string_without_quotes = ''.join(char for char in string_with_quotes if char != '"')
print(string_without_quotes) # Output: He said, Hello, World!
在上面的代码中,生成器表达式逐个检查字符串中的每个字符,并构建一个不包含双引号的生成器对象,然后使用 join()
方法将生成器对象的元素重新连接成一个字符串。
总结
通过上述方法,我们可以在 Python 中删除字符串中的双引号。replace() 方法、strip() 方法、正则表达式、split() 和 join() 方法、translate() 方法、递归函数、lambda 表达式、map() 和 filter() 函数、列表推导式、生成器表达式等方法都可以实现这一需求。根据具体情况选择合适的方法,以达到最佳的效果。
相关问答FAQs:
在Python中,如何去除字符串中的双引号?
可以使用字符串的replace()
方法来去除双引号。例如,my_string.replace('"', '')
将返回一个没有双引号的新字符串。你也可以使用strip()
方法去除字符串开头和结尾的双引号,但这只适用于去掉边界的引号。
有没有其他方法可以删除字符串中的双引号?
除了replace()
方法,使用正则表达式也是一种有效的方式。通过import re
后,可以使用re.sub(r'"', '', my_string)
来替换所有的双引号为一个空字符串,从而实现删除。
在处理文本文件时,如何去掉读取内容中的双引号?
在读取文本文件时,可以在读取每一行后立即使用replace()
方法来去掉双引号。例如,在读取文件时,可以这样处理:
with open('file.txt', 'r') as f:
for line in f:
cleaned_line = line.replace('"', '')
# 继续处理cleaned_line
这种方式确保每次读取时都能去掉双引号,便于后续的文本处理。
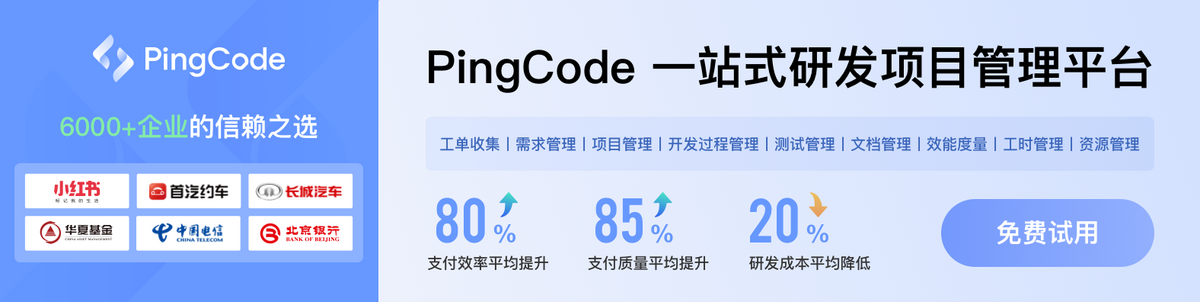