Python抓取网页内容的常用方法包括使用requests库发送HTTP请求、使用BeautifulSoup库解析HTML内容、利用Selenium进行动态网页抓取等。requests库和BeautifulSoup库适用于静态网页数据抓取,Selenium则适用于需要模拟用户操作的动态网页抓取。下面将详细介绍如何使用requests和BeautifulSoup进行静态网页抓取,并对Selenium进行动态网页抓取进行阐述。
一、使用requests库发送HTTP请求
requests库是Python中一个非常流行的HTTP库,可以非常方便地发送HTTP请求,并获取响应内容。以下是使用requests库抓取网页内容的步骤:
-
安装requests库:
pip install requests
-
发送HTTP请求并获取响应内容:
import requests
url = 'http://example.com'
response = requests.get(url)
print(response.text)
在上面的代码中,我们使用requests.get()方法发送HTTP GET请求,并将响应内容存储在response对象中。response.text属性包含了网页的HTML内容。
二、使用BeautifulSoup库解析HTML内容
BeautifulSoup是一个用于解析HTML和XML文档的Python库,可以轻松地从网页中提取数据。以下是使用BeautifulSoup库解析HTML内容的步骤:
-
安装BeautifulSoup库:
pip install beautifulsoup4
-
使用BeautifulSoup解析HTML内容:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify())
在上面的代码中,我们使用BeautifulSoup将response.text解析为一个BeautifulSoup对象,并使用prettify()方法格式化输出HTML内容。接下来,我们可以使用BeautifulSoup提供的各种方法和属性从网页中提取所需的数据。
三、使用Selenium进行动态网页抓取
Selenium是一个用于自动化Web浏览器操作的工具,可以用于抓取需要模拟用户操作的动态网页内容。以下是使用Selenium进行动态网页抓取的步骤:
-
安装Selenium库和浏览器驱动(如ChromeDriver):
pip install selenium
-
使用Selenium抓取动态网页内容:
from selenium import webdriver
from selenium.webdriver.common.by import By
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
等待页面加载完成,获取所需元素
element = driver.find_element(By.XPATH, '//*[@id="element_id"]')
print(element.text)
driver.quit()
在上面的代码中,我们使用Selenium创建一个Chrome浏览器实例,并使用get()方法访问指定的URL。然后,我们使用find_element()方法找到所需的元素,并获取其文本内容。最后,我们关闭浏览器实例。
四、requests库和BeautifulSoup库的结合使用
在实际的网页抓取过程中,requests库和BeautifulSoup库通常是结合使用的。以下是一个结合使用requests和BeautifulSoup抓取网页内容的完整示例:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
提取网页中的所有链接
for link in soup.find_all('a'):
print(link.get('href'))
在上面的代码中,我们使用requests库发送HTTP请求,并使用BeautifulSoup解析响应内容。然后,我们使用find_all()方法找到网页中的所有链接,并输出每个链接的href属性值。
五、处理网页中的表单和AJAX请求
在抓取网页内容时,可能会遇到需要提交表单或处理AJAX请求的情况。以下是处理表单提交和AJAX请求的示例:
-
提交表单:
import requests
url = 'http://example.com/login'
data = {
'username': 'your_username',
'password': 'your_password'
}
response = requests.post(url, data=data)
print(response.text)
-
处理AJAX请求:
import requests
url = 'http://example.com/ajax_endpoint'
headers = {
'X-Requested-With': 'XMLHttpRequest'
}
response = requests.get(url, headers=headers)
print(response.json())
在上面的代码中,我们使用requests.post()方法提交表单数据,并使用requests.get()方法发送AJAX请求,设置了一个特殊的HTTP头部X-Requested-With: XMLHttpRequest以模拟AJAX请求。
六、处理复杂网页结构
在实际的网页抓取过程中,网页的结构可能非常复杂,包含了嵌套的标签和动态加载的内容。以下是处理复杂网页结构的一些技巧:
-
使用CSS选择器:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
使用CSS选择器提取元素
for item in soup.select('.item-class'):
print(item.text)
-
使用XPath选择器:
from lxml import html
import requests
url = 'http://example.com'
response = requests.get(url)
tree = html.fromstring(response.content)
使用XPath选择器提取元素
for item in tree.xpath('//div[@class="item-class"]'):
print(item.text)
七、处理分页和多页抓取
在抓取网页内容时,可能需要处理分页或多页抓取的情况。以下是处理分页和多页抓取的示例:
-
处理分页:
from bs4 import BeautifulSoup
import requests
base_url = 'http://example.com/page/'
for page in range(1, 11): # 假设有10页
url = base_url + str(page)
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 提取当前页的内容
for item in soup.find_all('div', class_='item-class'):
print(item.text)
-
处理多页抓取:
from bs4 import BeautifulSoup
import requests
start_url = 'http://example.com'
next_page = start_url
while next_page:
response = requests.get(next_page)
soup = BeautifulSoup(response.text, 'html.parser')
# 提取当前页的内容
for item in soup.find_all('div', class_='item-class'):
print(item.text)
# 查找下一页链接
next_page = soup.find('a', {'rel': 'next'})
if next_page:
next_page = next_page.get('href')
else:
next_page = None
八、处理反爬虫机制
在抓取网页内容时,可能会遇到网站的反爬虫机制。以下是一些应对反爬虫机制的技巧:
-
模拟浏览器请求:
import requests
url = 'http://example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get(url, headers=headers)
print(response.text)
-
使用代理IP:
import requests
url = 'http://example.com'
proxies = {
'http': 'http://your_proxy_ip:port',
'https': 'http://your_proxy_ip:port'
}
response = requests.get(url, proxies=proxies)
print(response.text)
-
设置请求间隔:
import time
import requests
url = 'http://example.com'
for _ in range(10):
response = requests.get(url)
print(response.text)
time.sleep(2) # 设置请求间隔,避免被检测为爬虫
九、处理JavaScript渲染的内容
在抓取网页内容时,可能会遇到JavaScript渲染的内容。以下是处理JavaScript渲染内容的方法:
-
使用Selenium模拟浏览器操作:
from selenium import webdriver
from selenium.webdriver.common.by import By
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
等待页面加载完成,获取所需元素
element = driver.find_element(By.XPATH, '//*[@id="element_id"]')
print(element.text)
driver.quit()
-
使用Pyppeteer(Python版Puppeteer):
import asyncio
from pyppeteer import launch
async def main():
browser = await launch()
page = await browser.newPage()
await page.goto('http://example.com')
content = await page.content()
print(content)
await browser.close()
asyncio.get_event_loop().run_until_complete(main())
在上面的代码中,我们使用Pyppeteer模拟浏览器操作,并获取JavaScript渲染后的页面内容。
十、保存抓取的数据
在抓取网页内容后,通常需要将数据保存到文件或数据库中。以下是保存抓取数据的方法:
-
保存到文件:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
with open('data.txt', 'w', encoding='utf-8') as file:
for item in soup.find_all('div', class_='item-class'):
file.write(item.text + '\n')
-
保存到CSV文件:
import csv
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
with open('data.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['Item'])
for item in soup.find_all('div', class_='item-class'):
writer.writerow([item.text])
-
保存到数据库:
import sqlite3
from bs4 import BeautifulSoup
import requests
conn = sqlite3.connect('data.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS items (id INTEGER PRIMARY KEY, item TEXT)''')
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for item in soup.find_all('div', class_='item-class'):
cursor.execute('INSERT INTO items (item) VALUES (?)', (item.text,))
conn.commit()
conn.close()
以上是Python抓取网页内容的常用方法和技巧。根据具体的需求和网页结构,可以灵活运用requests库、BeautifulSoup库、Selenium和其他工具进行数据抓取。希望这些内容对你有所帮助,能够顺利完成网页数据抓取任务。
相关问答FAQs:
如何使用Python抓取动态加载的网页内容?
在抓取动态加载的网页内容时,传统的requests库可能无法获取到所需的数据。可以考虑使用Selenium或Playwright等工具,它们可以模拟浏览器行为,加载JavaScript生成的内容。通过这些工具,可以等待特定元素加载完成后,再抓取所需信息。
抓取网页内容后如何进行数据清洗和处理?
抓取到的网页内容通常包含许多冗余信息。使用BeautifulSoup库可以轻松解析HTML文档,提取所需的数据。通过正则表达式、字符串处理或Pandas等数据处理库,可以对数据进行清洗,去除多余的空格、HTML标签以及其他无关信息,确保数据的准确性和可用性。
在抓取网页内容时,如何避免被网站封禁?
为了避免被网站封禁,可以采取一些措施,如设置请求头(如User-Agent)以伪装成普通用户,控制请求频率,避免短时间内发送大量请求。此外,使用代理IP可以有效分散请求来源,降低被识别为爬虫的风险。遵循网站的robots.txt文件中的抓取规则也是良好的实践。
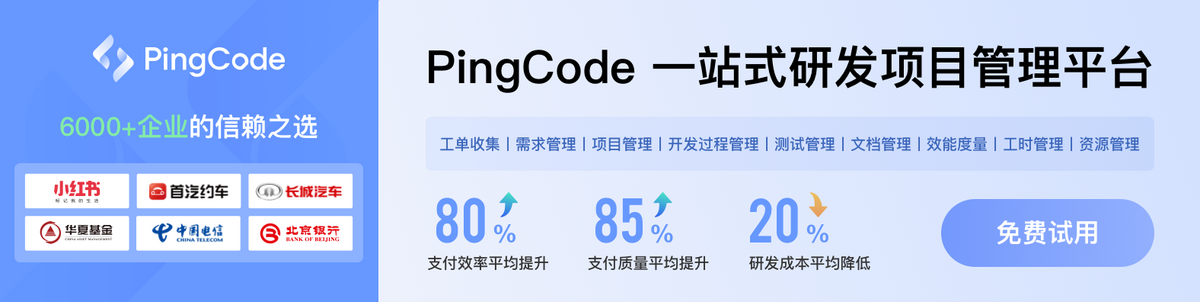