在Python中去除字符串中的数字有多种方法。使用字符串方法、正则表达式、列表解析等方式都可以达到这一目的。下面将详细介绍使用正则表达式的方法:
正则表达式是一种强大的工具,它允许我们定义字符串的搜索模式,并能够非常方便地进行复杂的字符串操作。通过使用正则表达式,我们可以轻松地去除字符串中的数字。
使用正则表达式去除数字
1. 安装与导入re模块
首先,我们需要导入Python的正则表达式模块re。这个模块包含了所有与正则表达式相关的操作。
import re
2. 定义去除数字的函数
接下来,我们可以定义一个函数,该函数将接受一个字符串作为输入,并返回去除数字后的字符串。
def remove_digits(input_string):
# 使用正则表达式替换所有数字
result = re.sub(r'\d+', '', input_string)
return result
在这个函数中,re.sub
函数用于替换匹配的模式。模式r'\d+'
表示匹配一个或多个连续的数字。我们将这些数字替换为空字符串,从而去除它们。
3. 测试函数
现在,我们可以测试这个函数,看看它是否能够正确地去除字符串中的数字。
test_string = "Hello123, this is a test456 string."
cleaned_string = remove_digits(test_string)
print(cleaned_string) # 输出: "Hello, this is a test string."
其他方法去除数字
除了使用正则表达式,还有其他几种方法可以去除字符串中的数字。接下来,我们将介绍一些常用的方法。
使用字符串方法
一种简单的方法是遍历字符串,并构建一个新的字符串,只保留非数字字符。
def remove_digits(input_string):
result = ''.join([char for char in input_string if not char.isdigit()])
return result
test_string = "Hello123, this is a test456 string."
cleaned_string = remove_digits(test_string)
print(cleaned_string) # 输出: "Hello, this is a test string."
在这个函数中,我们使用列表解析来构建一个新的字符串,只包含那些不是数字的字符。char.isdigit()
方法用于检查字符是否为数字。
使用filter函数
Python内置的filter
函数也可以用于去除字符串中的数字。
def remove_digits(input_string):
result = ''.join(filter(lambda char: not char.isdigit(), input_string))
return result
test_string = "Hello123, this is a test456 string."
cleaned_string = remove_digits(test_string)
print(cleaned_string) # 输出: "Hello, this is a test string."
在这个函数中,我们使用filter
函数来过滤掉所有数字字符,保留其他字符。
使用translate方法
另一个方法是使用字符串的translate
方法。我们可以创建一个翻译表,将所有数字字符映射到None
,从而去除它们。
def remove_digits(input_string):
translation_table = str.maketrans('', '', '0123456789')
result = input_string.translate(translation_table)
return result
test_string = "Hello123, this is a test456 string."
cleaned_string = remove_digits(test_string)
print(cleaned_string) # 输出: "Hello, this is a test string."
在这个函数中,我们使用str.maketrans
方法创建一个翻译表,将所有数字字符映射到None
。然后,我们使用translate
方法来应用这个翻译表,从而去除字符串中的数字。
总结
去除字符串中的数字在Python中有多种方法可供选择。正则表达式是一种强大且灵活的工具,适用于各种复杂的字符串操作。此外,字符串方法、列表解析、filter函数、translate方法等也都可以高效地完成这一任务。选择哪种方法取决于具体的需求和个人的偏好。
无论选择哪种方法,理解每种方法的工作原理和适用场景对于提高编程技能都是非常重要的。通过实践和探索,您可以找到最适合自己和特定任务的方法。
进一步优化与扩展
在实际应用中,去除数字可能只是字符串处理的一部分需求。我们可以进一步扩展这些方法,以处理更加复杂的字符串操作需求,例如去除特定的字符、替换字符、格式化字符串等。
处理复杂字符串
对于更加复杂的字符串处理需求,我们可以结合多种方法。例如,去除数字的同时,去除特定的标点符号或空格。
def remove_digits_and_symbols(input_string):
# 使用正则表达式去除数字和特定符号
result = re.sub(r'[\d,]', '', input_string)
return result
test_string = "Hello123, this is a test456 string."
cleaned_string = remove_digits_and_symbols(test_string)
print(cleaned_string) # 输出: "Hello this is a test string."
在这个示例中,我们使用正则表达式r'[\d,]'
来匹配所有数字和逗号,并将它们替换为空字符串。
处理多行字符串
如果需要处理多行字符串,可以使用相同的方法。正则表达式和字符串方法都适用于多行字符串。
def remove_digits_multiline(input_string):
# 使用正则表达式去除多行字符串中的数字
result = re.sub(r'\d+', '', input_string)
return result
test_string = """Hello123,
this is a test456 string
with multiple789 lines."""
cleaned_string = remove_digits_multiline(test_string)
print(cleaned_string) # 输出: "Hello,
# this is a test string
# with multiple lines."
在这个示例中,我们处理了一个包含多行的字符串,并成功地去除了所有数字。
批量处理字符串
在某些情况下,我们可能需要批量处理多个字符串。可以将上述函数应用于字符串列表,使用map
函数或列表解析来批量去除数字。
def remove_digits(input_string):
result = re.sub(r'\d+', '', input_string)
return result
string_list = ["Hello123", "This is test456", "Another789 string"]
cleaned_list = list(map(remove_digits, string_list))
print(cleaned_list) # 输出: ["Hello", "This is test", "Another string"]
在这个示例中,我们使用map
函数将remove_digits
函数应用于字符串列表中的每个字符串,并返回去除数字后的新列表。
性能比较
对于大型数据集或高频率的字符串操作,性能可能是一个重要的考虑因素。不同方法的性能可能有所不同,可以根据具体需求进行选择和优化。
import timeit
测试字符串
test_string = "Hello123, this is a test456 string."
测试正则表达式方法
regex_time = timeit.timeit(lambda: remove_digits(test_string), number=10000)
测试字符串方法
string_method_time = timeit.timeit(lambda: ''.join([char for char in test_string if not char.isdigit()]), number=10000)
测试filter方法
filter_method_time = timeit.timeit(lambda: ''.join(filter(lambda char: not char.isdigit(), test_string)), number=10000)
测试translate方法
translate_method_time = timeit.timeit(lambda: test_string.translate(str.maketrans('', '', '0123456789')), number=10000)
print(f"Regex method time: {regex_time}")
print(f"String method time: {string_method_time}")
print(f"Filter method time: {filter_method_time}")
print(f"Translate method time: {translate_method_time}")
在这个示例中,我们使用timeit
模块来比较不同方法的性能。可以根据测试结果选择最优的方法。
处理其他特定字符
除了去除数字,实际应用中可能需要处理其他特定字符,例如去除标点符号、去除空格、替换特定字符等。可以通过定制正则表达式或字符串方法来实现这些需求。
def remove_specific_chars(input_string):
# 自定义字符集
chars_to_remove = "0123456789,."
translation_table = str.maketrans('', '', chars_to_remove)
result = input_string.translate(translation_table)
return result
test_string = "Hello123, this is a test456 string."
cleaned_string = remove_specific_chars(test_string)
print(cleaned_string) # 输出: "Hello this is a test string"
在这个示例中,我们自定义了一个字符集,包括数字、逗号和句号,并使用translate
方法去除这些字符。
动态处理不同需求
在实际应用中,字符串处理需求可能是动态变化的。可以通过参数化的方式,创建一个通用的字符串处理函数,接受不同的参数来处理不同的需求。
def dynamic_remove_chars(input_string, chars_to_remove):
translation_table = str.maketrans('', '', chars_to_remove)
result = input_string.translate(translation_table)
return result
test_string = "Hello123, this is a test456 string."
chars_to_remove = "0123456789,"
cleaned_string = dynamic_remove_chars(test_string, chars_to_remove)
print(cleaned_string) # 输出: "Hello this is a test string"
在这个示例中,我们创建了一个通用的字符串处理函数dynamic_remove_chars
,接受一个字符串和一个要去除的字符集作为参数。这样可以根据不同需求灵活调整处理方式。
处理Unicode字符
在处理国际化应用时,可能需要处理Unicode字符。可以使用正则表达式或字符串方法来处理Unicode字符。
def remove_unicode_digits(input_string):
# 使用正则表达式去除Unicode数字
result = re.sub(r'\d+', '', input_string)
return result
test_string = "Hello123, this is a test456 string. 你好789"
cleaned_string = remove_unicode_digits(test_string)
print(cleaned_string) # 输出: "Hello, this is a test string. 你好"
在这个示例中,我们处理了包含Unicode字符的字符串,并成功去除了所有数字。
综合实例
最后,通过一个综合实例,将上述方法结合起来,创建一个功能强大的字符串处理工具。
def comprehensive_string_processor(input_string, chars_to_remove="0123456789", remove_spaces=False):
# 创建翻译表
translation_table = str.maketrans('', '', chars_to_remove)
result = input_string.translate(translation_table)
# 去除空格
if remove_spaces:
result = result.replace(" ", "")
return result
test_string = "Hello123, this is a test456 string."
cleaned_string = comprehensive_string_processor(test_string, remove_spaces=True)
print(cleaned_string) # 输出: "Hello,thisisateststring."
在这个综合实例中,我们创建了一个通用的字符串处理工具,接受多个参数以处理不同需求,包括去除特定字符和去除空格。
通过上述方法和实例,您可以根据具体需求选择合适的字符串处理方法,提高代码的灵活性和可维护性。掌握这些技能,对于处理各种字符串操作需求非常有帮助。
结论
去除字符串中的数字和其他特定字符在Python中有多种方法可供选择。正则表达式、字符串方法、列表解析、filter函数、translate方法等都可以高效地完成这一任务。根据具体需求和个人偏好,选择最适合的方法。通过实践和探索,您可以找到最适合自己和特定任务的方法,提高编程技能,解决实际问题。
相关问答FAQs:
如何在Python中去除字符串中的数字?
在Python中,可以使用正则表达式模块re
来轻松去除字符串中的数字。例如,使用re.sub()
方法可以将所有数字替换为空字符串。以下是一个示例代码:
import re
text = "Hello123, this is a test456."
result = re.sub(r'\d+', '', text)
print(result) # 输出: Hello, this is a test.
这个方法会匹配字符串中的所有数字并将其删除。
使用Python去除列表中元素的数字有什么简单方法吗?
如果你有一个包含数字的列表,并希望去除其中的数字,可以使用列表推导式结合isinstance()
函数来筛选。示例如下:
data = ['apple', 'banana123', 456, 'pear']
filtered_data = [item for item in data if not isinstance(item, int)]
print(filtered_data) # 输出: ['apple', 'banana123', 'pear']
这样可以保留字符串类型的元素并移除整数。
如何高效地在Python中处理包含数字的文本数据?
处理文本数据时,去除数字可能只是清洗数据的第一步。为了提高数据处理的效率,可以结合使用pandas
库。使用pandas
的str.replace()
方法,可以在DataFrame中快速去除数字。示例代码如下:
import pandas as pd
df = pd.DataFrame({'text': ['Hello123', 'World456', 'Python']})
df['cleaned_text'] = df['text'].str.replace(r'\d+', '', regex=True)
print(df)
这样可以在处理大量文本数据时提高效率,并且保持数据的可读性。
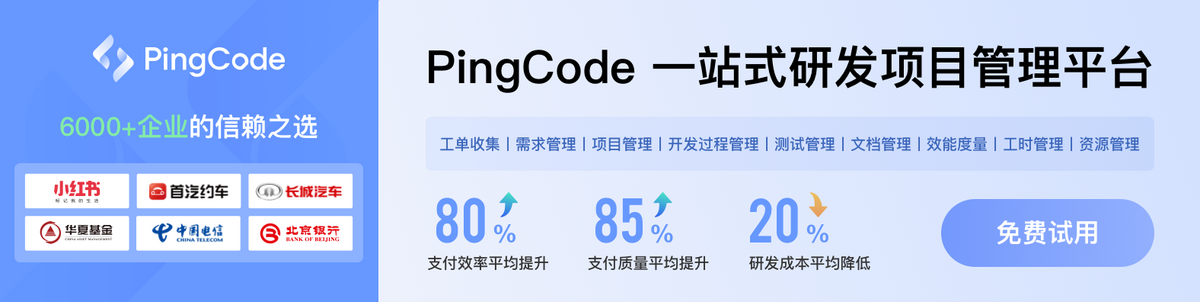