在Python中,设置主索引主要通过Pandas库来实现。使用Pandas库的set_index
方法、使用reset_index
方法、使用index_col
参数是设置主索引的常用方法。下面我们将详细介绍其中的一种方法。
一、使用Pandas库的set_index
方法:
set_index
方法可以将DataFrame的某一列或多列设置为索引,这样可以更方便地进行数据操作和分析。以下是使用set_index
方法的步骤:
- 首先,需要导入Pandas库并创建一个示例数据集:
import pandas as pd
创建一个示例数据集
data = {
'id': [1, 2, 3, 4],
'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40]
}
df = pd.DataFrame(data)
- 使用
set_index
方法将'id'列设置为索引:
df.set_index('id', inplace=True)
print(df)
此时,id
列已经成为了DataFrame的索引。
二、使用Pandas库的reset_index
方法:
reset_index
方法可以将索引重置为默认的整型索引,并将原来的索引列重新变回DataFrame的列。以下是使用reset_index
方法的步骤:
- 继续使用上面的示例数据集,首先查看当前的DataFrame:
print(df)
- 使用
reset_index
方法将索引重置为默认整型索引:
df.reset_index(inplace=True)
print(df)
此时,id
列已经重新变回了DataFrame的列。
三、使用Pandas库的index_col
参数:
在读取数据时,可以直接通过index_col
参数将某一列设置为索引。以下是使用index_col
参数的步骤:
- 创建一个CSV文件作为示例数据源:
import csv
data = [
['id', 'name', 'age'],
[1, 'Alice', 25],
[2, 'Bob', 30],
[3, 'Charlie', 35],
[4, 'David', 40]
]
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
- 使用
index_col
参数读取CSV文件并将'id'列设置为索引:
df = pd.read_csv('example.csv', index_col='id')
print(df)
此时,id
列已经作为索引读取进DataFrame。
四、索引的操作和应用:
-
选择数据:
索引可以大大简化数据的选择和切片操作。例如:
# 通过索引选择单行数据
row = df.loc[1]
print(row)
通过索引选择多行数据
rows = df.loc[[1, 3]]
print(rows)
-
重新索引:
可以使用
reindex
方法对DataFrame进行重新索引。new_index = [1, 2, 3, 4, 5]
df_reindexed = df.reindex(new_index)
print(df_reindexed)
-
多级索引:
Pandas还支持多级索引(MultiIndex),这对于处理分层数据非常有用。
arrays = [
['bar', 'bar', 'baz', 'baz', 'foo', 'foo', 'qux', 'qux'],
['one', 'two', 'one', 'two', 'one', 'two', 'one', 'two']
]
index = pd.MultiIndex.from_arrays(arrays, names=['first', 'second'])
df_multi = pd.DataFrame({'A': range(8)}, index=index)
print(df_multi)
-
索引操作:
可以对索引进行各种操作,如排序、合并等。
# 排序索引
df_sorted = df.sort_index()
print(df_sorted)
合并索引
df1 = pd.DataFrame({'A': [1, 2, 3]}, index=[1, 2, 3])
df2 = pd.DataFrame({'B': [4, 5, 6]}, index=[2, 3, 4])
df_merged = df1.join(df2, how='outer')
print(df_merged)
五、索引的性能优化:
-
避免重复索引:
重复索引会影响性能,确保索引是唯一的。
df = df.set_index('id', verify_integrity=True)
-
使用适当的数据类型:
使用适当的数据类型(如分类数据类型)可以提高索引操作的性能。
df['name'] = df['name'].astype('category')
-
分区索引:
对于大数据集,可以考虑使用分区索引来提高性能。Pandas本身不支持分区索引,但可以结合Dask等库来实现。
import dask.dataframe as dd
ddf = dd.from_pandas(df, npartitions=3)
-
索引缓存:
对于频繁使用的索引,可以考虑缓存以提高访问速度。
通过以上内容,可以看出索引在数据操作和分析中的重要性。在实际应用中,根据具体需求选择合适的索引操作方法和优化策略,可以显著提高数据处理的效率和性能。
相关问答FAQs:
如何在Python中创建主索引?
在Python中,创建主索引通常涉及使用Pandas库来处理数据框。您可以使用set_index()
方法将某一列设置为主索引。例如,df.set_index('column_name')
将指定列设置为索引,便于数据的快速访问和操作。
在使用Pandas时,如何选择合适的列作为主索引?
选择主索引时,需考虑该列的唯一性和稳定性。理想情况下,主索引应该是唯一的,以确保每一行数据都有一个唯一的标识符。避免使用会频繁变动的列,如时间戳或状态信息,以维持数据的完整性和准确性。
如何查看和重置Pandas数据框的主索引?
要查看当前的主索引,您可以使用df.index
来输出当前索引。如果您需要重置主索引,可以使用df.reset_index()
方法,这将恢复默认的数值索引,并将原索引列添加为数据框的新列。
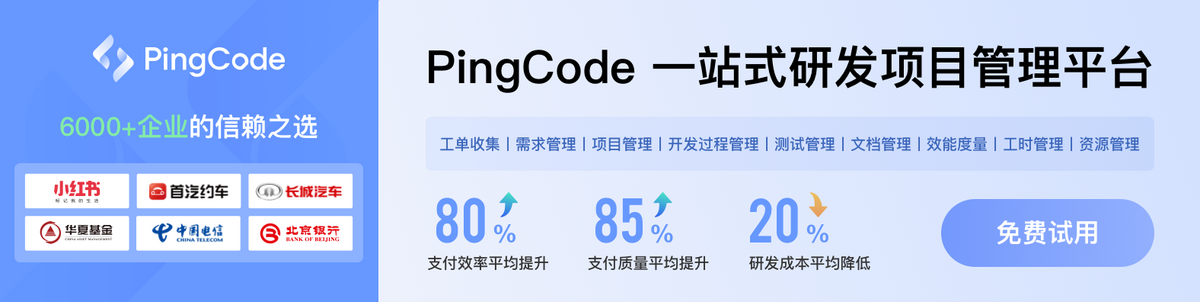