Python 提供了多种方法来操作文件,包括读取文件、写入文件、删除文件等。使用 open() 函数打开文件、使用 read() 方法读取文件内容、使用 write() 方法写入文件内容、使用 close() 方法关闭文件。其中,使用 open() 函数打开文件是最常用的操作之一。
使用 open() 函数打开文件
在 Python 中,文件操作的第一步是打开文件。可以使用内置的 open()
函数来完成这个任务。open()
函数的语法如下:
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
其中,file
参数是文件路径,mode
参数指定文件的打开模式,常用的模式有:
'r'
:读模式(默认)'w'
:写模式'a'
:追加模式'b'
:二进制模式't'
:文本模式(默认)
例如,以下代码展示了如何以读模式打开一个文件:
file = open('example.txt', 'r')
一、读文件
1、使用 read() 方法读取文件内容
read()
方法可以一次性读取整个文件的内容,适用于文件内容较小的情况。示例如下:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
使用 with
语句可以确保文件在读取完毕后自动关闭。
2、使用 readline() 方法逐行读取文件内容
readline()
方法每次只读取文件的一行内容,适用于逐行处理文件内容的情况。示例如下:
with open('example.txt', 'r') as file:
line = file.readline()
while line:
print(line, end='')
line = file.readline()
3、使用 readlines() 方法读取所有行并返回列表
readlines()
方法将文件的每一行作为一个元素,存储到列表中并返回。示例如下:
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line, end='')
二、写文件
1、使用 write() 方法写入文件内容
write()
方法可以向文件中写入字符串内容。如果文件不存在,open()
函数会创建一个新文件。示例如下:
with open('example.txt', 'w') as file:
file.write('Hello, World!')
在写入文件时,可以使用 'a'
模式以追加内容的方式写入文件,而不是覆盖原有内容。
2、使用 writelines() 方法写入多行内容
writelines()
方法可以向文件中写入一个字符串列表。示例如下:
lines = ['Hello, World!\n', 'Python is great!\n']
with open('example.txt', 'w') as file:
file.writelines(lines)
三、关闭文件
在文件操作完成后,应该关闭文件以释放系统资源。可以使用 close()
方法手动关闭文件,或者使用 with
语句自动关闭文件。示例如下:
file = open('example.txt', 'r')
content = file.read()
file.close()
四、文件操作的其他方法
1、文件指针操作
文件指针指示了当前读写位置,可以使用 seek()
方法设置文件指针的位置,使用 tell()
方法获取当前文件指针的位置。示例如下:
with open('example.txt', 'r') as file:
print(file.tell()) # 输出文件指针的初始位置
file.seek(5) # 将文件指针移动到位置 5
content = file.read()
print(content)
2、处理二进制文件
在操作二进制文件时,需要使用 'b'
模式打开文件。例如,读取一个二进制文件:
with open('example.bin', 'rb') as file:
data = file.read()
print(data)
3、使用 os 模块删除文件
可以使用 os
模块提供的 remove()
函数来删除文件。示例如下:
import os
os.remove('example.txt')
五、异常处理
在文件操作中,可能会遇到各种异常情况,如文件不存在、权限不足等。可以使用 try-except
结构来捕获并处理这些异常。示例如下:
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('文件未找到')
except PermissionError:
print('权限不足')
except Exception as e:
print(f'发生错误:{e}')
六、文件操作的一些高级应用
1、使用上下文管理器
Python 的 with
语句可以用于简化文件操作,确保文件在操作完成后自动关闭。上下文管理器不仅限于文件操作,还可以应用于其他资源管理场景。示例如下:
class ManagedFile:
def __init__(self, filename):
self.filename = filename
def __enter__(self):
self.file = open(self.filename, 'w')
return self.file
def __exit__(self, exc_type, exc_val, exc_tb):
self.file.close()
with ManagedFile('example.txt') as file:
file.write('Hello, World!')
2、读取大文件
在处理大文件时,一次性读取整个文件可能会导致内存不足。可以使用迭代器逐行读取文件,避免内存占用过大。示例如下:
with open('large_file.txt', 'r') as file:
for line in file:
process(line) # 自定义处理函数
3、CSV 文件操作
CSV 文件是一种常见的数据存储格式,可以使用 csv
模块读写 CSV 文件。示例如下:
import csv
读取 CSV 文件
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
写入 CSV 文件
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age'])
writer.writerow(['Alice', 30])
writer.writerow(['Bob', 25])
4、JSON 文件操作
JSON 文件是一种常见的数据交换格式,可以使用 json
模块读写 JSON 文件。示例如下:
import json
读取 JSON 文件
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
写入 JSON 文件
data = {'Name': 'Alice', 'Age': 30}
with open('example.json', 'w') as file:
json.dump(data, file)
七、文件和目录操作
1、创建和删除目录
可以使用 os
模块提供的 mkdir()
函数创建目录,使用 rmdir()
函数删除目录。示例如下:
import os
创建目录
os.mkdir('example_dir')
删除目录
os.rmdir('example_dir')
2、遍历目录
可以使用 os
模块提供的 listdir()
函数列出目录中的所有文件和子目录。示例如下:
import os
for item in os.listdir('.'):
print(item)
3、获取文件和目录的属性
可以使用 os
模块提供的 stat()
函数获取文件和目录的属性。示例如下:
import os
stats = os.stat('example.txt')
print(f'Size: {stats.st_size} bytes')
print(f'Last modified: {stats.st_mtime}')
八、文件路径操作
1、路径拼接
可以使用 os.path
模块提供的 join()
函数拼接路径,确保路径的正确性。示例如下:
import os
path = os.path.join('example_dir', 'example.txt')
print(path)
2、获取文件名和目录名
可以使用 os.path
模块提供的 basename()
和 dirname()
函数获取文件名和目录名。示例如下:
import os
path = 'example_dir/example.txt'
print(os.path.basename(path)) # 输出 'example.txt'
print(os.path.dirname(path)) # 输出 'example_dir'
3、检查文件或目录是否存在
可以使用 os.path
模块提供的 exists()
函数检查文件或目录是否存在。示例如下:
import os
if os.path.exists('example.txt'):
print('文件存在')
else:
print('文件不存在')
九、文件压缩和解压
1、使用 zipfile 模块
可以使用 zipfile
模块创建和解压 ZIP 文件。示例如下:
import zipfile
创建 ZIP 文件
with zipfile.ZipFile('example.zip', 'w') as zipf:
zipf.write('example.txt')
解压 ZIP 文件
with zipfile.ZipFile('example.zip', 'r') as zipf:
zipf.extractall('extracted_files')
2、使用 tarfile 模块
可以使用 tarfile
模块创建和解压 TAR 文件。示例如下:
import tarfile
创建 TAR 文件
with tarfile.open('example.tar', 'w') as tarf:
tarf.add('example.txt')
解压 TAR 文件
with tarfile.open('example.tar', 'r') as tarf:
tarf.extractall('extracted_files')
十、日志文件操作
1、使用 logging 模块
可以使用 logging
模块记录日志信息到文件。示例如下:
import logging
logging.basicConfig(filename='example.log', level=logging.INFO)
logging.info('This is an informational message.')
logging.error('This is an error message.')
2、配置日志格式
可以配置日志的输出格式和日志级别,示例如下:
import logging
logging.basicConfig(filename='example.log', level=logging.INFO,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s')
logger = logging.getLogger('example_logger')
logger.info('This is an informational message.')
logger.error('This is an error message.')
十一、文件的权限操作
1、修改文件权限
可以使用 os
模块提供的 chmod()
函数修改文件的权限。示例如下:
import os
设置文件为只读
os.chmod('example.txt', 0o444)
2、查看文件权限
可以使用 os.stat()
函数查看文件的权限。示例如下:
import os
stats = os.stat('example.txt')
print(f'Permissions: {oct(stats.st_mode)[-3:]}')
十二、文件的时间戳操作
1、修改文件的时间戳
可以使用 os
模块提供的 utime()
函数修改文件的访问时间和修改时间。示例如下:
import os
import time
修改文件的访问时间和修改时间为当前时间
os.utime('example.txt', (time.time(), time.time()))
2、查看文件的时间戳
可以使用 os.stat()
函数查看文件的访问时间、修改时间和创建时间。示例如下:
import os
stats = os.stat('example.txt')
print(f'Access time: {time.ctime(stats.st_atime)}')
print(f'Modify time: {time.ctime(stats.st_mtime)}')
print(f'Creation time: {time.ctime(stats.st_ctime)}')
十三、文件的复制和移动
1、使用 shutil 模块复制文件
可以使用 shutil
模块提供的 copy()
函数复制文件。示例如下:
import shutil
shutil.copy('example.txt', 'copy_of_example.txt')
2、使用 shutil 模块移动文件
可以使用 shutil
模块提供的 move()
函数移动文件。示例如下:
import shutil
shutil.move('example.txt', 'new_directory/example.txt')
通过本文的详细介绍,我们了解了Python中操作文件的多种方法和技巧,包括文件的读取、写入、关闭、删除、异常处理以及高级应用。掌握这些技巧可以帮助我们在实际项目中更加高效地操作文件和目录,提高开发效率。
相关问答FAQs:
如何在Python中读取文件的内容?
要读取文件内容,您可以使用内置的open()
函数。通过指定文件路径及模式(如'r'表示只读),然后使用read()
或readlines()
方法读取文件的所有内容或逐行读取。例如:
with open('example.txt', 'r') as file:
content = file.read() # 读取整个文件
这种方法会确保文件在读取后自动关闭,避免资源浪费。
如何在Python中写入文件?
写入文件同样使用open()
函数,但这次需要使用写入模式(如'w'表示写入,'a'表示追加)。使用write()
方法可以将字符串写入文件。示例代码如下:
with open('example.txt', 'w') as file:
file.write('Hello, World!') # 将内容写入文件
确保在写入模式下,文件中的现有内容会被替换,而追加模式则会在文件末尾添加新内容。
如何处理文件操作中的异常?
在进行文件操作时,文件可能不存在或无法访问,因此使用try-except
结构来捕捉异常是明智的选择。这样可以避免程序因错误而崩溃。示例代码如下:
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查文件路径。")
except IOError:
print("文件操作时发生错误。")
这种方式确保了即使出现问题,程序也能提供友好的错误提示。
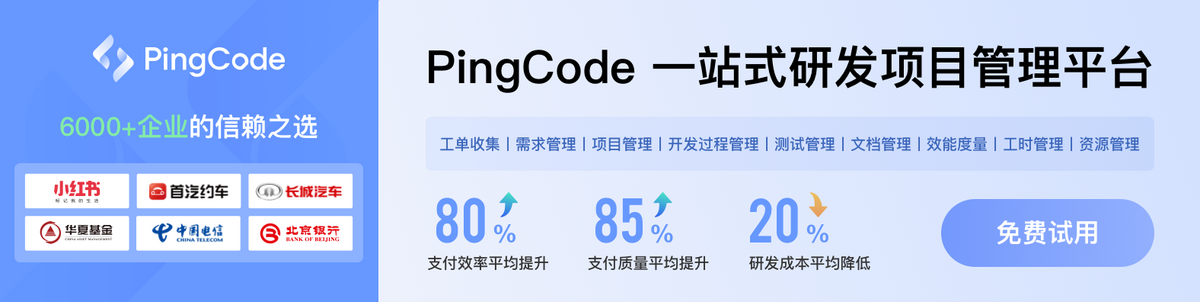