在Python中实现卷积神经网络(CNN),你可以使用深度学习框架如TensorFlow和Keras。通过使用这些框架,可以方便地构建和训练卷积神经网络、提取特征、进行图像分类等任务。 其中,Keras是一个高层API,构建在TensorFlow之上,非常适合快速构建和实验深度学习模型。接下来,我将详细描述如何使用Keras来实现一个简单的卷积神经网络。
一、准备工作
在开始之前,你需要确保已安装TensorFlow和Keras。如果还没有安装,可以使用以下命令进行安装:
pip install tensorflow
二、导入必要的库
在构建CNN模型之前,我们需要导入一些必要的库:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
from tensorflow.keras.preprocessing.image import ImageDataGenerator
三、数据准备
在训练CNN模型之前,我们需要准备数据。我们将使用Keras自带的MNIST数据集作为示例,它包含手写数字的图像。你也可以使用自己的数据集,通过ImageDataGenerator进行数据预处理。
# 加载MNIST数据集
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
数据预处理
x_train = x_train.reshape(-1, 28, 28, 1).astype('float32') / 255
x_test = x_test.reshape(-1, 28, 28, 1).astype('float32') / 255
将标签转换为one-hot编码
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
四、构建CNN模型
接下来,我们将构建一个简单的CNN模型。这个模型包含卷积层、池化层、全连接层和Dropout层。
model = Sequential()
第一个卷积层
model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)))
model.add(MaxPooling2D(pool_size=(2, 2)))
第二个卷积层
model.add(Conv2D(64, kernel_size=(3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
第三个卷积层
model.add(Conv2D(128, kernel_size=(3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
全连接层
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(10, activation='softmax'))
五、编译模型
在构建好模型之后,我们需要编译模型,指定损失函数、优化器和评估指标。
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
六、训练模型
接下来,我们开始训练模型。我们可以使用fit方法来训练模型,并指定训练数据、验证数据、批次大小和训练的轮数。
model.fit(x_train, y_train, batch_size=128, epochs=10, validation_data=(x_test, y_test))
七、评估模型
在训练完成后,我们可以使用evaluate方法来评估模型在测试数据上的表现。
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f'Test accuracy: {test_acc}')
八、保存和加载模型
为了方便以后使用,我们可以将训练好的模型保存到文件中,并在需要时加载。
# 保存模型
model.save('mnist_cnn_model.h5')
加载模型
model = tf.keras.models.load_model('mnist_cnn_model.h5')
九、预测新数据
我们可以使用训练好的模型来预测新的图像数据。
import numpy as np
假设new_image是一个新的手写数字图像
new_image = ...
预处理图像
new_image = new_image.reshape(-1, 28, 28, 1).astype('float32') / 255
进行预测
predictions = model.predict(new_image)
predicted_class = np.argmax(predictions, axis=1)
print(f'Predicted class: {predicted_class}')
十、数据增强
为了提升模型的泛化能力,我们可以使用数据增强技术。Keras提供了ImageDataGenerator类,方便我们进行数据增强。
datagen = ImageDataGenerator(
rotation_range=10,
zoom_range=0.1,
width_shift_range=0.1,
height_shift_range=0.1
)
重新训练模型时,使用数据增强生成的数据
model.fit(datagen.flow(x_train, y_train, batch_size=128), epochs=10, validation_data=(x_test, y_test))
十一、模型的优化
在实际项目中,训练一个CNN模型可能需要一些优化技巧来提高模型的性能和训练速度。
1、调整超参数
超参数的选择在模型性能中起着至关重要的作用。常见的超参数包括学习率、批次大小、优化器类型等。你可以通过网格搜索或随机搜索的方法来调整这些超参数。
from sklearn.model_selection import GridSearchCV
from tensorflow.keras.wrappers.scikit_learn import KerasClassifier
def create_model(optimizer='adam'):
model = Sequential()
model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
model.compile(loss='categorical_crossentropy', optimizer=optimizer, metrics=['accuracy'])
return model
model = KerasClassifier(build_fn=create_model)
param_grid = {'batch_size': [32, 64, 128], 'epochs': [10, 20], 'optimizer': ['adam', 'sgd']}
grid = GridSearchCV(estimator=model, param_grid=param_grid, n_jobs=-1, cv=3)
grid_result = grid.fit(x_train, y_train)
print(f'Best: {grid_result.best_score_} using {grid_result.best_params_}')
2、使用预训练模型
在某些情况下,使用预训练模型可以提高性能并减少训练时间。Keras提供了一些常用的预训练模型,如VGG、ResNet等,可以直接加载并微调这些模型。
from tensorflow.keras.applications import VGG16
加载预训练的VGG16模型,不包含顶层全连接层
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
添加自定义的全连接层
model = Sequential()
model.add(base_model)
model.add(Flatten())
model.add(Dense(256, activation='relu'))
model.add(Dense(10, activation='softmax'))
冻结预训练模型的卷积层
for layer in base_model.layers:
layer.trainable = False
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
假设x_train和y_train已被调整为适合VGG16输入的形状和大小
model.fit(x_train, y_train, batch_size=32, epochs=10, validation_data=(x_test, y_test))
十二、模型评估和可视化
为了更好地理解模型的表现,我们可以绘制训练过程中的损失和准确率曲线,或使用混淆矩阵来评估分类结果。
1、绘制训练曲线
import matplotlib.pyplot as plt
history = model.fit(x_train, y_train, batch_size=128, epochs=10, validation_data=(x_test, y_test))
绘制训练和验证损失曲线
plt.plot(history.history['loss'], label='train_loss')
plt.plot(history.history['val_loss'], label='val_loss')
plt.legend()
plt.show()
绘制训练和验证准确率曲线
plt.plot(history.history['accuracy'], label='train_accuracy')
plt.plot(history.history['val_accuracy'], label='val_accuracy')
plt.legend()
plt.show()
2、混淆矩阵
from sklearn.metrics import confusion_matrix
import seaborn as sns
预测测试集
y_pred = model.predict(x_test)
y_pred_classes = np.argmax(y_pred, axis=1)
y_true = np.argmax(y_test, axis=1)
计算混淆矩阵
conf_matrix = confusion_matrix(y_true, y_pred_classes)
绘制混淆矩阵
sns.heatmap(conf_matrix, annot=True, fmt='d', cmap='Blues')
plt.xlabel('Predicted')
plt.ylabel('True')
plt.show()
十三、模型推理优化
在模型推理阶段,优化模型的推理速度是非常重要的。可以使用TensorFlow Lite来优化模型并部署到移动设备或嵌入式设备上。
1、转换为TensorFlow Lite模型
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
保存TensorFlow Lite模型
with open('mnist_cnn_model.tflite', 'wb') as f:
f.write(tflite_model)
2、加载并运行TensorFlow Lite模型
import tensorflow as tf
加载TensorFlow Lite模型
interpreter = tf.lite.Interpreter(model_path='mnist_cnn_model.tflite')
interpreter.allocate_tensors()
获取输入输出张量
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
预处理图像并设置输入张量
new_image = new_image.reshape(1, 28, 28, 1).astype('float32') / 255
interpreter.set_tensor(input_details[0]['index'], new_image)
运行模型
interpreter.invoke()
获取预测结果
output_data = interpreter.get_tensor(output_details[0]['index'])
predicted_class = np.argmax(output_data, axis=1)
print(f'Predicted class: {predicted_class}')
十四、模型解释性
理解模型的决策过程对于实际应用非常重要。我们可以使用Grad-CAM等技术来可视化模型的注意力区域,从而解释模型的预测结果。
1、Grad-CAM可视化
import cv2
import numpy as np
import matplotlib.pyplot as plt
def grad_cam(model, img, layer_name):
grad_model = tf.keras.models.Model([model.inputs], [model.get_layer(layer_name).output, model.output])
with tf.GradientTape() as tape:
conv_outputs, predictions = grad_model(img)
loss = predictions[:, np.argmax(predictions[0])]
grads = tape.gradient(loss, conv_outputs)[0]
pooled_grads = tf.reduce_mean(grads, axis=(0, 1, 2))
conv_outputs = conv_outputs[0]
for i in range(pooled_grads.shape[-1]):
conv_outputs[:, :, i] *= pooled_grads[i]
heatmap = np.mean(conv_outputs, axis=-1)
heatmap = np.maximum(heatmap, 0)
heatmap /= np.max(heatmap)
return heatmap
假设new_image是预处理后的输入图像
heatmap = grad_cam(model, new_image, 'conv2d_2')
可视化热力图
plt.matshow(heatmap)
plt.show()
将热力图叠加到原始图像上
heatmap = cv2.resize(heatmap, (new_image.shape[1], new_image.shape[2]))
heatmap = np.uint8(255 * heatmap)
heatmap = cv2.applyColorMap(heatmap, cv2.COLORMAP_JET)
superimposed_img = heatmap * 0.4 + new_image[0]
plt.imshow(superimposed_img)
plt.show()
通过这些步骤,你可以在Python中实现一个简单但功能强大的卷积神经网络。从数据准备、模型构建、训练到评估和优化,每一步都至关重要。希望这个详细的指南能帮助你更好地理解和实现CNN模型。
相关问答FAQs:
如何在Python中构建一个基本的CNN模型?
在Python中构建一个卷积神经网络(CNN)通常使用深度学习框架,如TensorFlow或PyTorch。首先,您需要安装相应的库。接下来,您可以定义网络结构,包括卷积层、池化层和全连接层。使用Keras API,您可以通过Sequential模型轻松构建CNN。例如,可以通过Conv2D
层添加卷积操作,接着使用MaxPooling2D
进行池化,最后通过Dense
层实现分类。
在Python中实现CNN需要哪些数据准备步骤?
在构建CNN之前,数据的准备是至关重要的。首先,您需要收集并清洗数据,确保其格式适合模型输入。通常,图像数据需要调整大小到一致的尺寸,并进行归一化处理。此外,数据集可以划分为训练集和验证集,以便评估模型性能。数据增强技术也可以应用于训练集,以提高模型的泛化能力。
如何评估和优化Python中CNN模型的性能?
评估CNN模型的性能可以通过多种指标实现,例如准确率、损失值等。可以在验证集上计算这些指标,以判断模型的有效性。此外,优化模型性能的方法包括调整超参数(如学习率、批量大小等)、使用不同的优化器、增加或减少层数以及使用正则化技术来防止过拟合。使用交叉验证也可以帮助确认模型的稳健性。
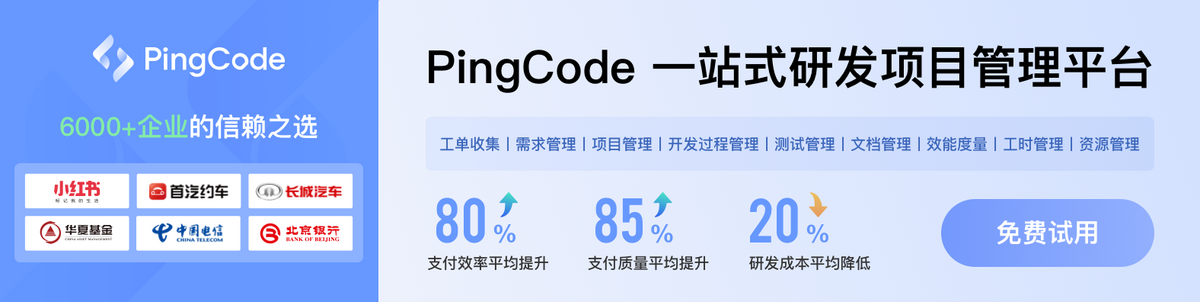