使用Python抓取职位的方法有:使用请求库获取网页内容、解析HTML提取职位信息、保存数据到文件或数据库。 其中,使用请求库获取网页内容 是抓取职位信息的重要步骤。请求库(如requests
)可用于发送HTTP请求并获取网页源代码。以下是详细介绍:
使用requests
库获取网页内容是抓取职位信息的基础。通过发送HTTP请求,获取目标网页的HTML代码,然后可以使用解析库(如BeautifulSoup
)从HTML中提取所需的职位信息。以下是具体步骤:
首先,安装所需的库:
pip install requests beautifulsoup4
然后,编写代码获取网页内容:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/jobs'
response = requests.get(url)
html_content = response.content
soup = BeautifulSoup(html_content, 'html.parser')
jobs = soup.find_all('div', class_='job-listing')
for job in jobs:
title = job.find('h2').text
company = job.find('span', class_='company').text
location = job.find('span', class_='location').text
print(f'Title: {title}, Company: {company}, Location: {location}')
上述代码展示了如何获取网页内容并使用BeautifulSoup
解析HTML,提取职位信息并打印。接下来将详细介绍每个步骤及其他方法。
一、使用请求库获取网页内容
使用requests
库发送HTTP请求,可以轻松获取网页内容,这是抓取网页信息的第一步。
1、安装和导入请求库
首先需要安装并导入requests
库:
pip install requests
导入库:
import requests
2、发送HTTP请求
使用requests.get()
发送HTTP GET请求,获取网页内容:
url = 'https://example.com/jobs'
response = requests.get(url)
3、检查响应状态
检查请求是否成功,确保获取到正确的网页内容:
if response.status_code == 200:
print('Request successful!')
else:
print('Request failed:', response.status_code)
4、获取网页HTML内容
成功获取网页内容后,可以通过response.content
或response.text
获取HTML代码:
html_content = response.content
二、解析HTML提取职位信息
使用解析库(如BeautifulSoup
)从HTML中提取所需的职位信息。
1、安装和导入BeautifulSoup
首先需要安装并导入BeautifulSoup
库:
pip install beautifulsoup4
导入库:
from bs4 import BeautifulSoup
2、创建BeautifulSoup对象
将HTML代码传递给BeautifulSoup
,创建解析对象:
soup = BeautifulSoup(html_content, 'html.parser')
3、查找职位信息
使用BeautifulSoup
的查找方法,提取职位信息。例如,查找所有职位列表项:
jobs = soup.find_all('div', class_='job-listing')
4、提取职位详情
遍历职位列表,提取职位标题、公司名称和工作地点:
for job in jobs:
title = job.find('h2').text
company = job.find('span', class_='company').text
location = job.find('span', class_='location').text
print(f'Title: {title}, Company: {company}, Location: {location}')
三、保存数据到文件或数据库
提取到的职位信息可以保存到文件或数据库中,便于后续分析和处理。
1、保存到CSV文件
使用csv
库将职位信息保存到CSV文件:
import csv
with open('jobs.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['Title', 'Company', 'Location'])
for job in jobs:
title = job.find('h2').text
company = job.find('span', class_='company').text
location = job.find('span', class_='location').text
writer.writerow([title, company, location])
2、保存到数据库
使用sqlite3
库将职位信息保存到SQLite数据库:
import sqlite3
conn = sqlite3.connect('jobs.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS jobs
(title TEXT, company TEXT, location TEXT)''')
for job in jobs:
title = job.find('h2').text
company = job.find('span', class_='company').text
location = job.find('span', class_='location').text
c.execute("INSERT INTO jobs (title, company, location) VALUES (?, ?, ?)",
(title, company, location))
conn.commit()
conn.close()
四、处理动态网页
对于一些动态加载内容的网页,可以使用Selenium库模拟浏览器操作,获取完整的网页内容。
1、安装和导入Selenium
首先需要安装并导入Selenium
库:
pip install selenium
导入库:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
2、配置WebDriver
配置WebDriver,使用Chrome浏览器获取网页内容:
chrome_options = Options()
chrome_options.add_argument("--headless") # 无头模式
service = Service('path/to/chromedriver') # 替换为ChromeDriver的路径
driver = webdriver.Chrome(service=service, options=chrome_options)
3、获取动态网页内容
使用WebDriver获取网页内容,等待所有动态加载的内容加载完成:
url = 'https://example.com/jobs'
driver.get(url)
等待动态内容加载完成(具体等待时间根据实际情况调整)
driver.implicitly_wait(10)
html_content = driver.page_source
driver.quit()
soup = BeautifulSoup(html_content, 'html.parser')
jobs = soup.find_all('div', class_='job-listing')
五、处理反爬虫措施
许多网站会实施反爬虫措施,限制或阻止自动化访问。以下是一些常见的反爬虫措施及应对方法。
1、设置请求头
通过设置请求头,模拟正常的浏览器请求,避免被检测为爬虫:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.198 Safari/537.36'
}
response = requests.get(url, headers=headers)
2、使用代理IP
使用代理IP,避免因频繁请求被封禁:
proxies = {
'http': 'http://your_proxy_ip:port',
'https': 'https://your_proxy_ip:port'
}
response = requests.get(url, headers=headers, proxies=proxies)
3、增加请求间隔
通过增加请求间隔,降低请求频率,避免触发反爬虫机制:
import time
for job in jobs:
title = job.find('h2').text
company = job.find('span', class_='company').text
location = job.find('span', class_='location').text
print(f'Title: {title}, Company: {company}, Location: {location}')
time.sleep(2) # 等待2秒
4、随机请求头和时间间隔
通过随机化请求头和时间间隔,进一步降低被检测为爬虫的风险:
import random
user_agents = [
'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.198 Safari/537.36',
'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36'
]
for job in jobs:
headers = {'User-Agent': random.choice(user_agents)}
response = requests.get(url, headers=headers)
time.sleep(random.uniform(1, 3)) # 随机等待1到3秒
六、处理复杂网页结构
对于一些复杂的网页结构,需要更高级的解析方法,例如使用XPath解析或结合正则表达式提取信息。
1、使用lxml和XPath
安装并导入lxml
库,使用XPath解析复杂网页结构:
pip install lxml
from lxml import etree
html_content = response.content
tree = etree.HTML(html_content)
job_titles = tree.xpath('//div[@class="job-listing"]/h2/text()')
job_companies = tree.xpath('//div[@class="job-listing"]/span[@class="company"]/text()')
job_locations = tree.xpath('//div[@class="job-listing"]/span[@class="location"]/text()')
for title, company, location in zip(job_titles, job_companies, job_locations):
print(f'Title: {title}, Company: {company}, Location: {location}')
2、结合正则表达式
使用正则表达式从HTML中提取所需的职位信息:
import re
html_content = response.text
job_titles = re.findall(r'<h2>(.*?)</h2>', html_content)
job_companies = re.findall(r'<span class="company">(.*?)</span>', html_content)
job_locations = re.findall(r'<span class="location">(.*?)</span>', html_content)
for title, company, location in zip(job_titles, job_companies, job_locations):
print(f'Title: {title}, Company: {company}, Location: {location}')
七、处理分页内容
许多职位网站会将职位信息分页展示,需要处理分页内容,获取所有职位信息。
1、识别分页链接
通过分析网页结构,识别分页链接的URL模式:
page_urls = [f'https://example.com/jobs?page={i}' for i in range(1, 6)]
2、循环请求分页内容
循环请求每一页的内容,提取职位信息:
for page_url in page_urls:
response = requests.get(page_url, headers=headers)
html_content = response.content
soup = BeautifulSoup(html_content, 'html.parser')
jobs = soup.find_all('div', class_='job-listing')
for job in jobs:
title = job.find('h2').text
company = job.find('span', class_='company').text
location = job.find('span', class_='location').text
print(f'Title: {title}, Company: {company}, Location: {location}')
八、使用API获取职位信息
有些职位网站提供API接口,可以直接通过API获取职位信息,避免HTML解析的复杂性。
1、查找API文档
查找职位网站的API文档,获取API的使用方法和参数说明。
2、发送API请求
使用requests
库发送API请求,获取职位信息:
api_url = 'https://api.example.com/jobs'
params = {'location': 'New York', 'type': 'full-time'}
response = requests.get(api_url, params=params, headers=headers)
data = response.json()
for job in data['jobs']:
title = job['title']
company = job['company']
location = job['location']
print(f'Title: {title}, Company: {company}, Location: {location}')
3、处理API响应
解析API响应中的职位信息,保存到文件或数据库中:
with open('jobs_from_api.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['Title', 'Company', 'Location'])
for job in data['jobs']:
title = job['title']
company = job['company']
location = job['location']
writer.writerow([title, company, location])
九、自动化爬虫调度
为了定期更新职位信息,可以使用定时任务或爬虫调度框架实现自动化爬虫调度。
1、使用cron定时任务
在Linux系统中,可以使用cron定时任务定期运行爬虫脚本:
crontab -e
添加如下定时任务,每天凌晨2点运行爬虫脚本
0 2 * * * /usr/bin/python3 /path/to/your_script.py
2、使用爬虫调度框架
使用如Scrapy
的爬虫调度框架,可以更灵活地管理和调度爬虫任务:
import scrapy
class JobSpider(scrapy.Spider):
name = 'job_spider'
start_urls = ['https://example.com/jobs']
def parse(self, response):
for job in response.css('div.job-listing'):
yield {
'title': job.css('h2::text').get(),
'company': job.css('span.company::text').get(),
'location': job.css('span.location::text').get(),
}
next_page = response.css('a.next::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
通过运行Scrapy爬虫,可以自动处理分页内容并提取职位信息。
十、常见问题与解决方案
在使用Python抓取职位信息的过程中,可能会遇到一些常见问题,以下是几个典型问题及其解决方案。
1、反爬虫机制
一些网站会检测并阻止自动化请求,常见的反爬虫机制有验证码、IP封禁、频率限制等。应对方法包括使用代理、增加请求间隔、模拟浏览器请求等。
2、动态加载内容
对于动态加载内容的网页,需要使用Selenium模拟浏览器操作,获取完整的网页内容。确保等待所有动态内容加载完成后,再进行解析。
3、复杂网页结构
对于复杂的网页结构,使用XPath解析、正则表达式提取信息,或者结合不同的方法,提高解析的准确性。
4、API限流
使用API获取职位信息时,可能会遇到API限流的问题。可以通过增加请求间隔、使用API密钥、请求分页数据等方式,减少限流影响。
5、数据存储
提取的职位信息可以保存到文件(如CSV、JSON)或数据库(如SQLite、MySQL)中,选择合适的存储方式,便于后续分析和处理。
通过上述方法和技巧,可以使用Python高效地抓取职位信息。根据具体需求,灵活选择和组合不同的方法,确保抓取过程顺利、稳定。
相关问答FAQs:
如何使用Python抓取特定职位信息?
使用Python抓取职位信息通常涉及使用一些流行的库,如BeautifulSoup和Requests。首先,您需要确定目标网站,并分析其HTML结构,以确定所需数据的位置。接下来,您可以使用Requests库发送HTTP请求获取页面内容,然后通过BeautifulSoup解析HTML,提取职位名称、公司、地点等信息。最后,将这些数据存储到CSV文件或数据库中,以便后续分析。
抓取职位信息时需要注意哪些法律和道德问题?
在抓取职位信息时,务必遵循网站的使用条款和隐私政策。一些网站可能在其robots.txt文件中禁止爬虫抓取特定页面。此外,过于频繁的请求可能会导致IP被封禁,因此建议设置合适的请求间隔和使用代理IP。同时,确保不抓取个人隐私信息,以遵守数据保护法规。
有哪些Python库适合用于职位信息抓取?
Python有多个库可以帮助抓取职位信息。其中,Requests库用于发送HTTP请求获取网页内容,BeautifulSoup用于解析HTML文档并提取所需数据。Scrapy是一个功能强大的框架,适合进行大规模抓取。Selenium也可以用来抓取动态加载的页面,尤其适合需要模拟用户行为的网站。这些库各有特点,可以根据具体需求选择使用。
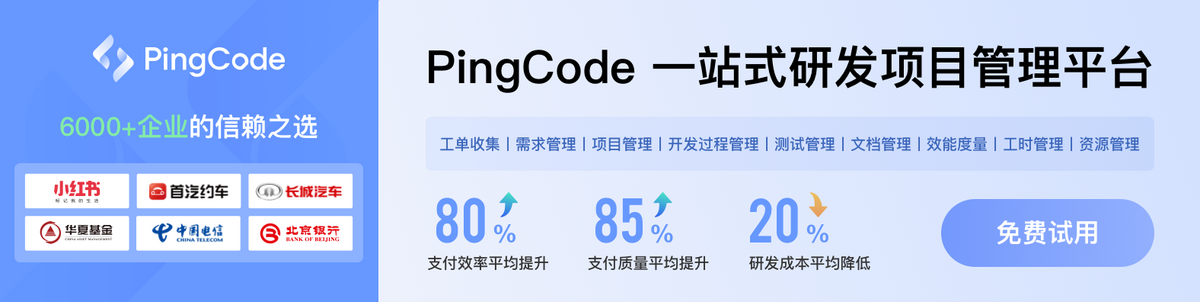