在Python中,批量复制数据的方法主要包括使用文件操作、数据库操作、Pandas库、shutil模块等。你可以根据具体需求和数据类型选择适合的方法。Pandas库是一个非常强大的数据操作库,特别适用于处理表格数据。接下来,将详细介绍如何使用Pandas库批量复制数据。
一、使用Pandas库
Pandas库是Python中一个强大的数据分析和数据处理库,广泛用于数据科学和数据分析领域。利用Pandas库,可以方便地进行数据读取、处理和写入操作。
1.1 读取数据
Pandas提供了丰富的数据读取方法,可以从CSV、Excel、SQL数据库等多种数据源中读取数据。例如:
import pandas as pd
从CSV文件读取数据
df = pd.read_csv('input_file.csv')
从Excel文件读取数据
df = pd.read_excel('input_file.xlsx')
从SQL数据库读取数据
import sqlite3
conn = sqlite3.connect('database.db')
df = pd.read_sql('SELECT * FROM table_name', conn)
1.2 复制数据
读取数据后,可以通过Pandas的各种方法对数据进行复制和操作。以下是一些常见的操作:
1.2.1 复制整个数据框
可以使用copy()
方法复制整个数据框:
df_copy = df.copy()
1.2.2 复制部分数据
可以使用loc
和iloc
方法选择并复制部分数据:
# 选择并复制特定列
df_columns_copy = df[['column1', 'column2']].copy()
选择并复制特定行
df_rows_copy = df.loc[0:10].copy() # 使用标签索引
df_rows_copy = df.iloc[0:10].copy() # 使用位置索引
1.3 写入数据
处理完数据后,可以将数据写入到不同的数据源中,例如CSV、Excel或SQL数据库:
# 写入CSV文件
df.to_csv('output_file.csv', index=False)
写入Excel文件
df.to_excel('output_file.xlsx', index=False)
写入SQL数据库
df.to_sql('table_name', conn, if_exists='replace', index=False)
二、使用shutil模块
shutil模块提供了高级的文件操作功能,可以方便地进行文件复制和移动等操作。
2.1 复制文件
使用shutil.copy()
或shutil.copy2()
方法可以复制文件:
import shutil
复制文件
shutil.copy('source_file.txt', 'destination_file.txt')
复制文件并保留元数据
shutil.copy2('source_file.txt', 'destination_file.txt')
2.2 复制目录
使用shutil.copytree()
方法可以递归地复制整个目录:
import shutil
复制目录
shutil.copytree('source_directory', 'destination_directory')
三、使用os模块
os模块提供了与操作系统进行交互的功能,可以用于文件和目录的操作。
3.1 复制文件
可以使用os模块结合shutil模块实现文件复制:
import os
import shutil
复制文件
src_file = 'source_file.txt'
dst_file = 'destination_file.txt'
shutil.copy(src_file, dst_file)
3.2 批量复制文件
可以使用os模块遍历目录并批量复制文件:
import os
import shutil
src_directory = 'source_directory'
dst_directory = 'destination_directory'
创建目标目录
if not os.path.exists(dst_directory):
os.makedirs(dst_directory)
遍历源目录并复制文件
for filename in os.listdir(src_directory):
src_file = os.path.join(src_directory, filename)
dst_file = os.path.join(dst_directory, filename)
if os.path.isfile(src_file):
shutil.copy(src_file, dst_file)
四、使用数据库操作
在进行大规模数据操作时,使用数据库是一个高效的方法。可以使用Python的数据库库,如sqlite3
、pymysql
、psycopg2
等,连接数据库并进行数据复制操作。
4.1 使用sqlite3
以下是使用sqlite3
库在SQLite数据库中复制数据的示例:
import sqlite3
连接源数据库
src_conn = sqlite3.connect('source_database.db')
src_cursor = src_conn.cursor()
连接目标数据库
dst_conn = sqlite3.connect('destination_database.db')
dst_cursor = dst_conn.cursor()
从源数据库读取数据
src_cursor.execute('SELECT * FROM table_name')
data = src_cursor.fetchall()
向目标数据库写入数据
dst_cursor.executemany('INSERT INTO table_name VALUES (?, ?, ?)', data)
提交更改并关闭连接
dst_conn.commit()
src_conn.close()
dst_conn.close()
4.2 使用pymysql
以下是使用pymysql
库在MySQL数据库中复制数据的示例:
import pymysql
连接源数据库
src_conn = pymysql.connect(host='source_host', user='user', password='password', db='source_db')
src_cursor = src_conn.cursor()
连接目标数据库
dst_conn = pymysql.connect(host='destination_host', user='user', password='password', db='destination_db')
dst_cursor = dst_conn.cursor()
从源数据库读取数据
src_cursor.execute('SELECT * FROM table_name')
data = src_cursor.fetchall()
向目标数据库写入数据
insert_query = 'INSERT INTO table_name (column1, column2, column3) VALUES (%s, %s, %s)'
dst_cursor.executemany(insert_query, data)
提交更改并关闭连接
dst_conn.commit()
src_conn.close()
dst_conn.close()
4.3 使用psycopg2
以下是使用psycopg2
库在PostgreSQL数据库中复制数据的示例:
import psycopg2
连接源数据库
src_conn = psycopg2.connect(host='source_host', dbname='source_db', user='user', password='password')
src_cursor = src_conn.cursor()
连接目标数据库
dst_conn = psycopg2.connect(host='destination_host', dbname='destination_db', user='user', password='password')
dst_cursor = dst_conn.cursor()
从源数据库读取数据
src_cursor.execute('SELECT * FROM table_name')
data = src_cursor.fetchall()
向目标数据库写入数据
insert_query = 'INSERT INTO table_name (column1, column2, column3) VALUES (%s, %s, %s)'
dst_cursor.executemany(insert_query, data)
提交更改并关闭连接
dst_conn.commit()
src_conn.close()
dst_conn.close()
五、使用多线程和多进程
在处理大规模数据复制操作时,可以使用多线程和多进程技术来提高效率。Python提供了threading
和multiprocessing
模块来实现多线程和多进程操作。
5.1 使用多线程
以下是使用threading
模块进行多线程数据复制的示例:
import threading
import shutil
def copy_file(src_file, dst_file):
shutil.copy(src_file, dst_file)
src_directory = 'source_directory'
dst_directory = 'destination_directory'
创建目标目录
if not os.path.exists(dst_directory):
os.makedirs(dst_directory)
创建线程列表
threads = []
遍历源目录并创建复制线程
for filename in os.listdir(src_directory):
src_file = os.path.join(src_directory, filename)
dst_file = os.path.join(dst_directory, filename)
if os.path.isfile(src_file):
thread = threading.Thread(target=copy_file, args=(src_file, dst_file))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
5.2 使用多进程
以下是使用multiprocessing
模块进行多进程数据复制的示例:
import multiprocessing
import shutil
def copy_file(src_file, dst_file):
shutil.copy(src_file, dst_file)
src_directory = 'source_directory'
dst_directory = 'destination_directory'
创建目标目录
if not os.path.exists(dst_directory):
os.makedirs(dst_directory)
创建进程池
pool = multiprocessing.Pool()
遍历源目录并向进程池提交任务
for filename in os.listdir(src_directory):
src_file = os.path.join(src_directory, filename)
dst_file = os.path.join(dst_directory, filename)
if os.path.isfile(src_file):
pool.apply_async(copy_file, args=(src_file, dst_file))
关闭进程池并等待所有进程完成
pool.close()
pool.join()
六、使用第三方库
除了Pandas、shutil和os模块,Python还有许多第三方库可以用于批量复制数据。例如,ftplib
库用于FTP文件传输,boto3
库用于操作AWS S3等。
6.1 使用ftplib库
以下是使用ftplib
库从FTP服务器下载文件的示例:
from ftplib import FTP
ftp = FTP('ftp_server_address')
ftp.login(user='username', passwd='password')
ftp.cwd('source_directory')
filenames = ftp.nlst()
for filename in filenames:
local_filename = os.path.join('destination_directory', filename)
with open(local_filename, 'wb') as local_file:
ftp.retrbinary('RETR ' + filename, local_file.write)
ftp.quit()
6.2 使用boto3库
以下是使用boto3
库从AWS S3下载文件的示例:
import boto3
s3 = boto3.client('s3')
bucket_name = 'source_bucket'
prefix = 'source_directory/'
response = s3.list_objects_v2(Bucket=bucket_name, Prefix=prefix)
for obj in response['Contents']:
key = obj['Key']
local_filename = os.path.join('destination_directory', os.path.basename(key))
s3.download_file(bucket_name, key, local_filename)
结论
在Python中,批量复制数据的方法有很多,选择合适的方法取决于具体的需求和数据类型。Pandas库是处理表格数据的强大工具,shutil模块适用于文件和目录操作,os模块提供了与操作系统交互的功能,数据库操作适用于大规模数据操作,多线程和多进程技术可以提高效率,第三方库如ftplib
和boto3
可以用于特定场景下的数据复制。希望本文能够帮助你更好地理解和实现Python中的批量数据复制操作。
相关问答FAQs:
如何使用Python批量复制文件或数据?
在Python中,可以使用shutil
库来批量复制文件和目录。通过shutil.copy()
可以复制单个文件,而shutil.copytree()
可以用来复制整个目录。首先,确保你有目标路径和源路径,并使用适当的函数进行操作。例如:
import shutil
# 复制单个文件
shutil.copy('source_file.txt', 'destination_file.txt')
# 复制整个目录
shutil.copytree('source_directory', 'destination_directory')
在复制数据时,如何处理文件冲突?
在批量复制时,如果目标路径已经存在同名文件,可能会导致文件冲突。可以选择在复制前检查目标文件是否存在,并根据需求决定是否覆盖或重命名。使用os.path.exists()
函数来检查文件是否存在。例如:
import os
source = 'source_file.txt'
destination = 'destination_file.txt'
if os.path.exists(destination):
print("文件已存在,选择覆盖或重命名。")
else:
shutil.copy(source, destination)
Python是否支持从数据库批量复制数据?
是的,Python可以通过各种库(如pandas
、SQLAlchemy
等)批量处理和复制数据库中的数据。使用pandas
的to_sql()
方法可以将DataFrame内容写入数据库表中,方便高效。例如:
import pandas as pd
from sqlalchemy import create_engine
# 创建数据库连接
engine = create_engine('mysql+pymysql://user:password@host/dbname')
# 读取数据到DataFrame
df = pd.read_csv('data.csv')
# 将数据写入数据库
df.to_sql('table_name', con=engine, index=False, if_exists='append')
这种方式可以轻松实现数据的批量复制与迁移。
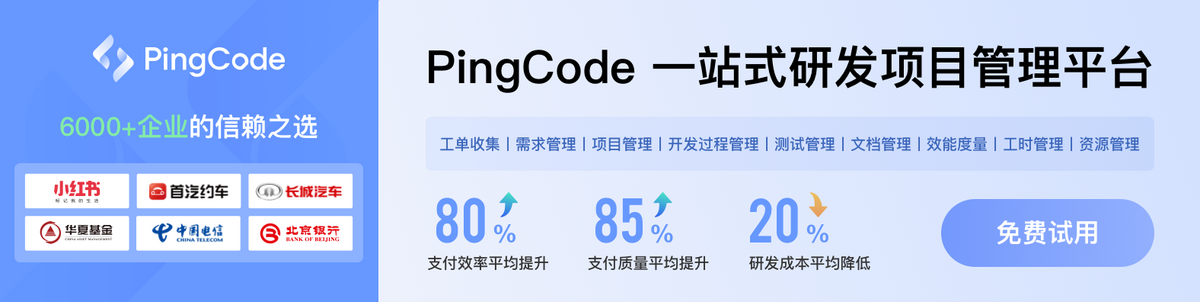