在Python中,如果你需要对列表、元组、字典等数据结构进行降序排序,可以使用一些内置函数和方法。使用sorted函数、使用sort方法、指定reverse参数。下面,我将详细描述其中的一种方法:使用sorted函数
使用sorted函数时,可以通过设置reverse参数为True来实现降序排序。sorted函数可以用于任何可迭代对象,并且不会修改原始数据,而是返回一个新的排序后的列表。下面是一个示例:
numbers = [1, 3, 5, 2, 4, 6]
sorted_numbers = sorted(numbers, reverse=True)
print(sorted_numbers)
在这个示例中,sorted函数对numbers列表进行了降序排序,并将结果存储在sorted_numbers中。最终输出将是:[6, 5, 4, 3, 2, 1]。这种方法特别适用于需要保留原始数据而不进行修改的情况。
一、使用sorted函数
sorted函数是Python内置的一个高效排序函数,适用于所有可迭代对象,比如列表、元组、字典、集合等。使用sorted函数进行降序排序时,只需将reverse参数设置为True。这个方法非常直观且易于理解。
1. 列表降序排序
我们以列表为例,展示如何使用sorted函数进行降序排序。
numbers = [10, 30, 20, 40, 60, 50]
sorted_numbers = sorted(numbers, reverse=True)
print(sorted_numbers) # 输出: [60, 50, 40, 30, 20, 10]
在这个例子中,sorted函数对numbers列表进行了降序排序,并且返回了一个新的排序后的列表。
2. 字典降序排序
对于字典,可以对字典的键或值进行排序。下面是一个根据字典的值进行降序排序的示例:
students = {'Alice': 88, 'Bob': 72, 'Charlie': 95}
sorted_students = sorted(students.items(), key=lambda item: item[1], reverse=True)
print(sorted_students) # 输出: [('Charlie', 95), ('Alice', 88), ('Bob', 72)]
在这个例子中,我们使用了sorted函数对students字典的项(键值对)进行了排序,并且通过lambda函数指定了排序的依据为字典的值。
3. 集合降序排序
集合(set)是无序的,但是我们可以通过sorted函数对集合进行排序,并返回一个排序后的列表。
numbers_set = {10, 30, 20, 40, 60, 50}
sorted_numbers_set = sorted(numbers_set, reverse=True)
print(sorted_numbers_set) # 输出: [60, 50, 40, 30, 20, 10]
在这个例子中,sorted函数对集合进行了排序,并且返回了一个新的排序后的列表。
二、使用sort方法
列表对象的sort方法可以对列表进行原地排序。通过设置reverse参数为True,可以实现降序排序。需要注意的是,sort方法会直接修改原列表。
1. 列表降序排序
我们以列表为例,展示如何使用sort方法进行降序排序。
numbers = [10, 30, 20, 40, 60, 50]
numbers.sort(reverse=True)
print(numbers) # 输出: [60, 50, 40, 30, 20, 10]
在这个例子中,sort方法对numbers列表进行了原地降序排序。
2. 自定义排序
如果需要根据自定义的规则进行排序,可以通过key参数指定一个函数,该函数用于从每个元素中提取用于排序的关键字。
students = [{'name': 'Alice', 'score': 88}, {'name': 'Bob', 'score': 72}, {'name': 'Charlie', 'score': 95}]
students.sort(key=lambda student: student['score'], reverse=True)
print(students) # 输出: [{'name': 'Charlie', 'score': 95}, {'name': 'Alice', 'score': 88}, {'name': 'Bob', 'score': 72}]
在这个例子中,我们通过key参数指定了一个lambda函数,该函数从每个学生字典中提取score作为排序关键字。
三、使用heapq模块
heapq模块提供了堆队列算法的实现,可以用于找到集合中的最大或最小元素。虽然heapq模块主要用于优先队列,但也可以用于排序。
1. 找到最大的n个元素
我们可以使用heapq.nlargest函数找到列表中最大的n个元素。
import heapq
numbers = [10, 30, 20, 40, 60, 50]
largest_numbers = heapq.nlargest(3, numbers)
print(largest_numbers) # 输出: [60, 50, 40]
在这个例子中,heapq.nlargest函数找到了numbers列表中最大的3个元素。
2. 找到最小的n个元素
同样的,我们可以使用heapq.nsmallest函数找到列表中最小的n个元素。
import heapq
numbers = [10, 30, 20, 40, 60, 50]
smallest_numbers = heapq.nsmallest(3, numbers)
print(smallest_numbers) # 输出: [10, 20, 30]
在这个例子中,heapq.nsmallest函数找到了numbers列表中最小的3个元素。
四、使用pandas模块
如果你在处理数据分析任务,可以使用pandas模块进行排序。pandas模块提供了DataFrame和Series两种数据结构,并且提供了强大的排序功能。
1. DataFrame降序排序
我们以DataFrame为例,展示如何使用pandas进行降序排序。
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie'], 'score': [88, 72, 95]}
df = pd.DataFrame(data)
sorted_df = df.sort_values(by='score', ascending=False)
print(sorted_df)
在这个例子中,我们使用sort_values方法对DataFrame进行了降序排序。
2. Series降序排序
对于Series数据结构,可以使用sort_values方法进行排序。
import pandas as pd
scores = pd.Series([88, 72, 95], index=['Alice', 'Bob', 'Charlie'])
sorted_scores = scores.sort_values(ascending=False)
print(sorted_scores)
在这个例子中,我们使用sort_values方法对Series进行了降序排序。
五、使用numpy模块
numpy模块是一个强大的数值计算库,提供了许多高效的数组操作功能。我们可以使用numpy模块对数组进行排序。
1. 数组降序排序
我们以numpy数组为例,展示如何使用numpy进行降序排序。
import numpy as np
numbers = np.array([10, 30, 20, 40, 60, 50])
sorted_numbers = np.sort(numbers)[::-1]
print(sorted_numbers) # 输出: [60 50 40 30 20 10]
在这个例子中,我们使用np.sort函数对数组进行了排序,然后通过[::-1]对排序结果进行了反转,实现了降序排序。
2. 多维数组降序排序
对于多维数组,可以使用numpy的argsort函数进行排序。
import numpy as np
numbers = np.array([[10, 30, 20], [40, 60, 50]])
sorted_numbers = np.argsort(-numbers, axis=1)
print(sorted_numbers)
在这个例子中,我们使用np.argsort函数对多维数组进行了排序,并且通过负号实现了降序排序。
六、使用sortedcontainers模块
sortedcontainers模块提供了高效的排序容器,比如SortedList、SortedDict、SortedSet等。我们可以使用这些容器进行排序。
1. SortedList降序排序
我们以SortedList为例,展示如何使用sortedcontainers进行降序排序。
from sortedcontainers import SortedList
numbers = SortedList([10, 30, 20, 40, 60, 50])
sorted_numbers = list(reversed(numbers))
print(sorted_numbers) # 输出: [60, 50, 40, 30, 20, 10]
在这个例子中,我们使用SortedList对列表进行了排序,然后通过reversed函数实现了降序排序。
2. SortedDict降序排序
对于SortedDict,可以通过items方法获取键值对的列表,然后进行排序。
from sortedcontainers import SortedDict
students = SortedDict({'Alice': 88, 'Bob': 72, 'Charlie': 95})
sorted_students = sorted(students.items(), key=lambda item: item[1], reverse=True)
print(sorted_students) # 输出: [('Charlie', 95), ('Alice', 88), ('Bob', 72)]
在这个例子中,我们使用sorted函数对SortedDict的项(键值对)进行了排序,并且通过lambda函数指定了排序的依据为字典的值。
七、使用operator模块
operator模块提供了一些函数,这些函数可以用于排序操作。比如,itemgetter函数可以从序列中获取特定的元素。
1. 使用itemgetter进行排序
我们以列表的列表为例,展示如何使用operator模块进行排序。
from operator import itemgetter
students = [['Alice', 88], ['Bob', 72], ['Charlie', 95]]
sorted_students = sorted(students, key=itemgetter(1), reverse=True)
print(sorted_students) # 输出: [['Charlie', 95], ['Alice', 88], ['Bob', 72]]
在这个例子中,我们使用itemgetter函数从每个子列表中获取第二个元素(即分数),然后进行排序。
2. 使用attrgetter进行排序
对于对象列表,可以使用attrgetter函数获取对象的属性进行排序。
from operator import attrgetter
class Student:
def __init__(self, name, score):
self.name = name
self.score = score
students = [Student('Alice', 88), Student('Bob', 72), Student('Charlie', 95)]
sorted_students = sorted(students, key=attrgetter('score'), reverse=True)
for student in sorted_students:
print(student.name, student.score)
在这个例子中,我们使用attrgetter函数从每个Student对象中获取score属性,然后进行排序。
八、使用functools模块
functools模块提供了一些高阶函数,这些函数可以用于排序操作。比如,cmp_to_key函数可以将比较函数转换为键函数。
1. 使用cmp_to_key进行排序
我们以列表为例,展示如何使用functools模块进行排序。
from functools import cmp_to_key
def compare(x, y):
return y - x
numbers = [10, 30, 20, 40, 60, 50]
sorted_numbers = sorted(numbers, key=cmp_to_key(compare))
print(sorted_numbers) # 输出: [60, 50, 40, 30, 20, 10]
在这个例子中,我们使用cmp_to_key函数将compare函数转换为了键函数,然后进行了排序。
2. 自定义比较函数
我们可以定义一个自定义的比较函数,然后使用cmp_to_key进行排序。
from functools import cmp_to_key
def compare(x, y):
if x[1] > y[1]:
return -1
elif x[1] < y[1]:
return 1
else:
return 0
students = [['Alice', 88], ['Bob', 72], ['Charlie', 95]]
sorted_students = sorted(students, key=cmp_to_key(compare))
print(sorted_students) # 输出: [['Charlie', 95], ['Alice', 88], ['Bob', 72]]
在这个例子中,我们定义了一个自定义的比较函数compare,然后使用cmp_to_key进行排序。
九、总结
在Python中,有多种方法可以实现降序排序,包括使用sorted函数、使用sort方法、使用heapq模块、使用pandas模块、使用numpy模块、使用sortedcontainers模块、使用operator模块、使用functools模块等。每种方法都有其适用的场景和优势。根据具体需求选择合适的方法,可以提高代码的可读性和效率。在选择排序方法时,应考虑数据类型、数据量以及具体需求。通过合理选择和应用这些方法,可以轻松实现数据的降序排序。
相关问答FAQs:
在Python中,如何对列表进行降序排序?
在Python中,可以使用内置的sort()
方法或sorted()
函数来对列表进行降序排序。对于sort()
方法,可以通过设置reverse=True
参数来实现。例如:
my_list = [5, 2, 9, 1]
my_list.sort(reverse=True)
print(my_list) # 输出: [9, 5, 2, 1]
而使用sorted()
函数也可以实现同样的效果,示例如下:
my_list = [5, 2, 9, 1]
sorted_list = sorted(my_list, reverse=True)
print(sorted_list) # 输出: [9, 5, 2, 1]
在Python中,如何对字典按值进行降序排序?
要对字典按值进行降序排序,可以使用sorted()
函数结合items()
方法。通过设置key
参数为字典的值,并将reverse
设为True
,就可以实现。例如:
my_dict = {'a': 3, 'b': 1, 'c': 2}
sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1], reverse=True))
print(sorted_dict) # 输出: {'a': 3, 'c': 2, 'b': 1}
在Python中,如何对字符串列表进行降序排序?
对于字符串列表的降序排序,sort()
和sorted()
同样适用。默认情况下,字符串的排序是按字母顺序的,因此通过设置reverse=True
就能得到降序排列。示例代码如下:
string_list = ["apple", "orange", "banana"]
string_list.sort(reverse=True)
print(string_list) # 输出: ['orange', 'banana', 'apple']
使用sorted()
函数也能实现类似的效果。
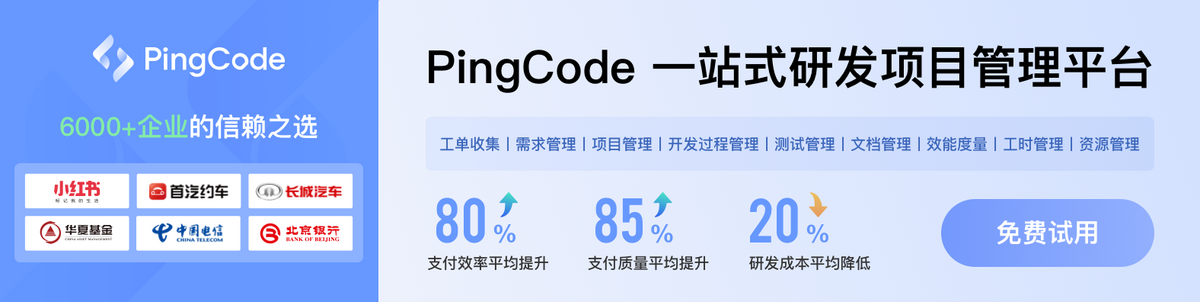