使用Python进行考勤可以通过以下方式:开发考勤系统、使用现有的库、集成数据库、使用图形界面开发工具。其中,开发考勤系统是一种灵活性高且可定制的方式,可以根据具体需求设计和实现考勤功能。
开发考勤系统涉及到多个步骤,包括设计数据库结构、编写考勤记录的增删改查(CRUD)操作、实现用户身份验证和权限管理、提供友好的用户界面等。在数据库设计方面,需要考虑员工信息表、考勤记录表、假期和加班记录表等。编写代码时,可以使用Python的Flask或Django框架来构建Web应用,提供API接口供前端调用。在用户界面设计上,可以使用HTML、CSS和JavaScript,以及前端框架如React或Vue.js来实现。
接下来,让我们详细讨论如何用Python开发一个简单的考勤系统。
一、开发考勤系统
1、设计数据库结构
在设计考勤系统的数据库时,通常需要以下几张表:
- 员工信息表(Employee):存储员工的基本信息,如员工ID、姓名、部门等。
- 考勤记录表(Attendance):存储每日考勤记录,包括打卡时间、状态(如迟到、早退、正常等)。
- 假期记录表(Leave):存储员工请假信息,包括请假开始时间、结束时间、假期类型等。
- 加班记录表(Overtime):存储员工加班信息,包括加班日期、加班时长等。
示例的表结构如下:
CREATE TABLE Employee (
employee_id INT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
department VARCHAR(100) NOT NULL
);
CREATE TABLE Attendance (
attendance_id INT PRIMARY KEY,
employee_id INT NOT NULL,
date DATE NOT NULL,
check_in_time TIME,
check_out_time TIME,
status VARCHAR(50),
FOREIGN KEY (employee_id) REFERENCES Employee(employee_id)
);
CREATE TABLE Leave (
leave_id INT PRIMARY KEY,
employee_id INT NOT NULL,
start_date DATE NOT NULL,
end_date DATE NOT NULL,
leave_type VARCHAR(50),
FOREIGN KEY (employee_id) REFERENCES Employee(employee_id)
);
CREATE TABLE Overtime (
overtime_id INT PRIMARY KEY,
employee_id INT NOT NULL,
date DATE NOT NULL,
duration TIME NOT NULL,
FOREIGN KEY (employee_id) REFERENCES Employee(employee_id)
);
2、编写CRUD操作
使用Python和Flask框架来编写考勤系统的CRUD操作。首先,安装Flask:
pip install Flask
然后,创建一个Flask应用,并编写CRUD操作:
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///attendance.db'
db = SQLAlchemy(app)
class Employee(db.Model):
employee_id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(100), nullable=False)
department = db.Column(db.String(100), nullable=False)
class Attendance(db.Model):
attendance_id = db.Column(db.Integer, primary_key=True)
employee_id = db.Column(db.Integer, db.ForeignKey('employee.employee_id'), nullable=False)
date = db.Column(db.Date, nullable=False)
check_in_time = db.Column(db.Time)
check_out_time = db.Column(db.Time)
status = db.Column(db.String(50))
Create the database
db.create_all()
Add an employee
@app.route('/employee', methods=['POST'])
def add_employee():
data = request.json
new_employee = Employee(name=data['name'], department=data['department'])
db.session.add(new_employee)
db.session.commit()
return jsonify({"message": "Employee added successfully!"}), 201
Record attendance
@app.route('/attendance', methods=['POST'])
def add_attendance():
data = request.json
new_attendance = Attendance(
employee_id=data['employee_id'],
date=data['date'],
check_in_time=data.get('check_in_time'),
check_out_time=data.get('check_out_time'),
status=data.get('status')
)
db.session.add(new_attendance)
db.session.commit()
return jsonify({"message": "Attendance recorded successfully!"}), 201
if __name__ == '__main__':
app.run(debug=True)
以上代码展示了如何使用Flask和SQLAlchemy创建一个简单的考勤系统,包括添加员工和记录考勤操作。
3、实现用户身份验证和权限管理
为了确保系统的安全性,考勤系统需要实现用户身份验证和权限管理。可以使用Flask-Login库来实现这一功能:
pip install Flask-Login
在Flask应用中,配置身份验证功能:
from flask_login import LoginManager, UserMixin, login_user, login_required, logout_user, current_user
login_manager = LoginManager()
login_manager.init_app(app)
class User(UserMixin, db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(100), unique=True)
password = db.Column(db.String(100))
@login_manager.user_loader
def load_user(user_id):
return User.query.get(int(user_id))
@app.route('/login', methods=['POST'])
def login():
data = request.json
user = User.query.filter_by(username=data['username']).first()
if user and user.password == data['password']:
login_user(user)
return jsonify({"message": "Logged in successfully!"}), 200
return jsonify({"message": "Invalid credentials!"}), 401
@app.route('/logout')
@login_required
def logout():
logout_user()
return jsonify({"message": "Logged out successfully!"}), 200
通过添加身份验证和权限管理,可以确保只有授权的用户才能访问和操作考勤系统的功能。
4、提供友好的用户界面
为了提供友好的用户界面,可以使用HTML、CSS和JavaScript以及前端框架如React或Vue.js来开发前端部分。以下是一个简单的HTML表单,用于添加员工和记录考勤:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Attendance System</title>
<style>
form {
margin-bottom: 20px;
}
</style>
</head>
<body>
<h1>Attendance System</h1>
<form id="employeeForm">
<h2>Add Employee</h2>
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="department">Department:</label>
<input type="text" id="department" name="department" required>
<button type="submit">Add Employee</button>
</form>
<form id="attendanceForm">
<h2>Record Attendance</h2>
<label for="employee_id">Employee ID:</label>
<input type="number" id="employee_id" name="employee_id" required>
<label for="date">Date:</label>
<input type="date" id="date" name="date" required>
<label for="check_in_time">Check-in Time:</label>
<input type="time" id="check_in_time" name="check_in_time">
<label for="check_out_time">Check-out Time:</label>
<input type="time" id="check_out_time" name="check_out_time">
<label for="status">Status:</label>
<input type="text" id="status" name="status">
<button type="submit">Record Attendance</button>
</form>
<script>
document.getElementById('employeeForm').addEventListener('submit', function(event) {
event.preventDefault();
const formData = new FormData(event.target);
fetch('/employee', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(Object.fromEntries(formData.entries()))
})
.then(response => response.json())
.then(data => alert(data.message));
});
document.getElementById('attendanceForm').addEventListener('submit', function(event) {
event.preventDefault();
const formData = new FormData(event.target);
fetch('/attendance', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(Object.fromEntries(formData.entries()))
})
.then(response => response.json())
.then(data => alert(data.message));
});
</script>
</body>
</html>
通过上述代码,可以实现一个简单的考勤系统的前端界面,用户可以通过表单添加员工和记录考勤信息。
二、使用现有的库
1、Pandas库
Pandas是一个强大的数据处理和分析库,可以用来处理考勤数据。以下是一个使用Pandas处理考勤数据的示例:
import pandas as pd
创建一个考勤数据的DataFrame
data = {
'employee_id': [1, 2, 3, 1, 2, 3],
'date': ['2023-01-01', '2023-01-01', '2023-01-01', '2023-01-02', '2023-01-02', '2023-01-02'],
'check_in_time': ['09:00', '09:15', '09:30', '09:00', '09:15', '09:30'],
'check_out_time': ['17:00', '17:15', '17:30', '17:00', '17:15', '17:30'],
'status': ['正常', '迟到', '迟到', '正常', '迟到', '迟到']
}
df = pd.DataFrame(data)
计算每个员工的出勤天数和迟到次数
attendance_summary = df.groupby('employee_id')['status'].value_counts().unstack().fillna(0)
attendance_summary['出勤天数'] = attendance_summary.sum(axis=1)
attendance_summary['迟到次数'] = attendance_summary['迟到']
print(attendance_summary)
使用Pandas可以方便地对考勤数据进行各种统计分析和处理,如计算出勤天数、迟到次数等。
2、OpenCV库
OpenCV是一个强大的计算机视觉库,可以用来实现考勤系统中的人脸识别功能。以下是一个使用OpenCV和Python实现人脸识别考勤的示例:
import cv2
加载预训练的人脸检测模型
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
打开摄像头
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.imshow('Face Detection', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
以上代码展示了如何使用OpenCV实现一个简单的人脸检测功能,可以进一步扩展为人脸识别考勤系统。
三、集成数据库
考勤系统通常需要存储大量的考勤记录和员工信息,可以使用关系型数据库如MySQL、PostgreSQL或NoSQL数据库如MongoDB来存储这些数据。
1、使用MySQL
以下是一个使用MySQL存储考勤数据的示例:
import mysql.connector
连接到MySQL数据库
conn = mysql.connector.connect(
host='localhost',
user='root',
password='password',
database='attendance'
)
cursor = conn.cursor()
插入考勤记录
sql = "INSERT INTO Attendance (employee_id, date, check_in_time, check_out_time, status) VALUES (%s, %s, %s, %s, %s)"
val = (1, '2023-01-01', '09:00', '17:00', '正常')
cursor.execute(sql, val)
conn.commit()
查询考勤记录
cursor.execute("SELECT * FROM Attendance")
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
通过上述代码,可以将考勤数据存储到MySQL数据库中,并进行查询操作。
2、使用MongoDB
以下是一个使用MongoDB存储考勤数据的示例:
from pymongo import MongoClient
连接到MongoDB数据库
client = MongoClient('mongodb://localhost:27017/')
db = client['attendance']
插入考勤记录
attendance = {
'employee_id': 1,
'date': '2023-01-01',
'check_in_time': '09:00',
'check_out_time': '17:00',
'status': '正常'
}
db.attendance.insert_one(attendance)
查询考勤记录
for record in db.attendance.find():
print(record)
通过上述代码,可以将考勤数据存储到MongoDB数据库中,并进行查询操作。
四、使用图形界面开发工具
为了提供更好的用户体验,可以使用图形界面开发工具如Tkinter、PyQt或Kivy来开发考勤系统的图形界面。
1、使用Tkinter
以下是一个使用Tkinter开发的简单考勤系统图形界面:
import tkinter as tk
from tkinter import messagebox
def add_employee():
name = name_entry.get()
department = department_entry.get()
messagebox.showinfo("Info", f"Employee {name} from {department} added successfully!")
def record_attendance():
employee_id = employee_id_entry.get()
date = date_entry.get()
check_in_time = check_in_time_entry.get()
check_out_time = check_out_time_entry.get()
status = status_entry.get()
messagebox.showinfo("Info", f"Attendance for Employee ID {employee_id} on {date} recorded successfully!")
root = tk.Tk()
root.title("Attendance System")
tk.Label(root, text="Add Employee").grid(row=0, column=0, columnspan=2)
tk.Label(root, text="Name:").grid(row=1, column=0)
name_entry = tk.Entry(root)
name_entry.grid(row=1, column=1)
tk.Label(root, text="Department:").grid(row=2, column=0)
department_entry = tk.Entry(root)
department_entry.grid(row=2, column=1)
tk.Button(root, text="Add Employee", command=add_employee).grid(row=3, column=0, columnspan=2)
tk.Label(root, text="Record Attendance").grid(row=4, column=0, columnspan=2)
tk.Label(root, text="Employee ID:").grid(row=5, column=0)
employee_id_entry = tk.Entry(root)
employee_id_entry.grid(row=5, column=1)
tk.Label(root, text="Date:").grid(row=6, column=0)
date_entry = tk.Entry(root)
date_entry.grid(row=6, column=1)
tk.Label(root, text="Check-in Time:").grid(row=7, column=0)
check_in_time_entry = tk.Entry(root)
check_in_time_entry.grid(row=7, column=1)
tk.Label(root, text="Check-out Time:").grid(row=8, column=0)
check_out_time_entry = tk.Entry(root)
check_out_time_entry.grid(row=8, column=1)
tk.Label(root, text="Status:").grid(row=9, column=0)
status_entry = tk.Entry(root)
status_entry.grid(row=9, column=1)
tk.Button(root, text="Record Attendance", command=record_attendance).grid(row=10, column=0, columnspan=2)
root.mainloop()
通过上述代码,可以实现一个简单的考勤系统图形界面,用户可以通过界面添加员工和记录考勤信息。
2、使用PyQt
以下是一个使用PyQt开发的简单考勤系统图形界面:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QFormLayout, QLineEdit, QPushButton, QMessageBox
class AttendanceSystem(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle("Attendance System")
layout = QVBoxLayout()
form_layout = QFormLayout()
self.name_input = QLineEdit()
self.department_input = QLineEdit()
form_layout.addRow("Name:", self.name_input)
form_layout.addRow("Department:", self.department_input)
add_employee_button = QPushButton("Add Employee")
add_employee_button.clicked.connect(self.add_employee)
layout.addLayout(form_layout)
layout.addWidget(add_employee_button)
form_layout = QFormLayout()
self.employee_id_input = QLineEdit()
相关问答FAQs:
如何使用Python进行考勤管理系统的开发?
要开发一个考勤管理系统,您可以使用Python的Flask或Django框架来创建网页应用,使用SQLite或MySQL作为数据库存储考勤记录。您需要设计数据库表结构,包括用户信息表和考勤记录表,并实现用户登录、签到、签退等功能。同时,可以利用Python的pandas库进行数据分析,生成考勤统计报表。
Python考勤系统是否可以与其他工具集成?
是的,Python考勤系统可以与多种工具集成。例如,您可以将考勤数据导出为Excel文件,使用pandas库轻松实现。还可以与邮件服务API集成,自动发送考勤报告给相关人员。此外,使用API与其他HR管理系统进行数据同步也是一种常见集成方式。
如何确保考勤数据的安全性和准确性?
确保考勤数据的安全性可以通过多种方法实现。首先,实施用户身份验证和授权机制,确保只有授权用户才能访问考勤系统。其次,定期备份数据库以防数据丢失。此外,数据的准确性可以通过设定规则和程序进行核对,例如限制签到和签退时间,避免用户随意修改考勤记录。使用日志记录功能也有助于追踪数据变更。
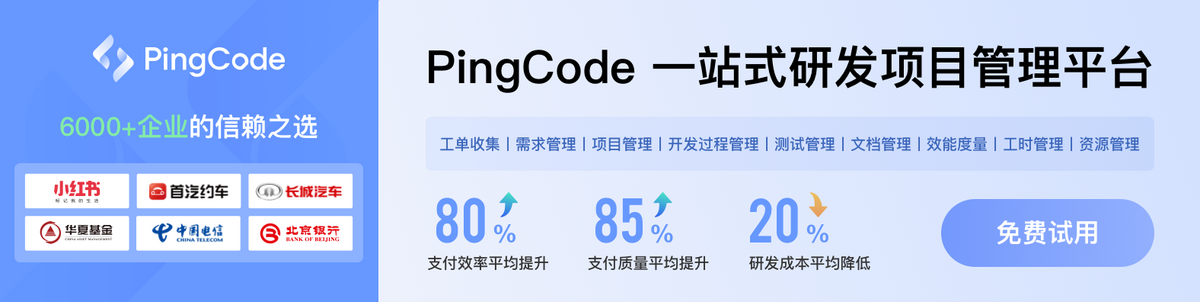