要用Python计算工资,可以采用基本工资、加班工资、税收扣除和其他扣除等因素来计算。其中,基本工资是每个员工的固定收入,加班工资是根据员工的加班时间和加班费率计算得出的,税收扣除和其他扣除项则是根据国家和公司的相关政策规定来进行扣除的。在这几个因素中,加班工资的计算是比较复杂的,需要根据加班时间和加班费率进行精确计算。
一、基本工资的计算
基本工资是员工每个月固定的收入,是计算总工资的基础。通常情况下,基本工资是由公司根据员工的职位、经验、资历等因素确定的。
def calculate_basic_salary(base_salary):
return base_salary
这个函数接受一个参数 base_salary
,表示员工的基本工资,并返回基本工资。
二、加班工资的计算
加班工资是根据员工的加班时间和加班费率计算得出的。通常情况下,加班时间和加班费率是由公司根据国家的相关政策规定和公司的实际情况确定的。
def calculate_overtime_salary(overtime_hours, overtime_rate):
return overtime_hours * overtime_rate
这个函数接受两个参数 overtime_hours
和 overtime_rate
,分别表示加班时间和加班费率,并返回加班工资。
三、税收扣除的计算
税收扣除是根据国家的相关政策规定进行扣除的。通常情况下,税收扣除是根据员工的收入情况和税收政策进行计算的。
def calculate_tax_deduction(total_income, tax_rate):
return total_income * tax_rate
这个函数接受两个参数 total_income
和 tax_rate
,分别表示员工的总收入和税率,并返回税收扣除金额。
四、其他扣除项的计算
其他扣除项是根据公司的相关政策规定进行扣除的。通常情况下,其他扣除项包括社保、公积金等。
def calculate_other_deductions(social_security, housing_fund):
return social_security + housing_fund
这个函数接受两个参数 social_security
和 housing_fund
,分别表示社保和公积金,并返回其他扣除项的总金额。
五、总工资的计算
总工资是基本工资、加班工资和其他收入的总和,再减去税收扣除和其他扣除项后的结果。
def calculate_total_salary(base_salary, overtime_hours, overtime_rate, tax_rate, social_security, housing_fund):
basic_salary = calculate_basic_salary(base_salary)
overtime_salary = calculate_overtime_salary(overtime_hours, overtime_rate)
total_income = basic_salary + overtime_salary
tax_deduction = calculate_tax_deduction(total_income, tax_rate)
other_deductions = calculate_other_deductions(social_security, housing_fund)
total_salary = total_income - tax_deduction - other_deductions
return total_salary
这个函数接受六个参数 base_salary
、overtime_hours
、overtime_rate
、tax_rate
、social_security
和 housing_fund
,分别表示基本工资、加班时间、加班费率、税率、社保和公积金,并返回总工资。
详细描述加班工资的计算
在计算加班工资时,我们需要注意几个重要因素:加班时间、加班费率、加班费率的适用范围等。加班时间是员工实际工作的加班时长,加班费率是公司根据国家和公司政策规定的加班费率。加班费率的适用范围是指加班费率适用于哪些时间段,比如平时加班、周末加班和节假日加班等。
加班时间的计算
加班时间是员工实际工作的加班时长,通常情况下,可以通过员工的上下班时间和标准工作时间来计算加班时间。
from datetime import datetime
def calculate_overtime_hours(start_time, end_time, standard_work_hours):
start_time = datetime.strptime(start_time, "%H:%M")
end_time = datetime.strptime(end_time, "%H:%M")
work_hours = (end_time - start_time).seconds / 3600
overtime_hours = max(0, work_hours - standard_work_hours)
return overtime_hours
这个函数接受三个参数 start_time
、end_time
和 standard_work_hours
,分别表示上下班时间和标准工作时间,并返回加班时间。
加班费率的计算
加班费率是公司根据国家和公司政策规定的加班费率,通常情况下,不同时间段的加班费率是不同的,比如平时加班、周末加班和节假日加班等。
def calculate_overtime_rate(day_of_week, is_holiday):
if is_holiday:
return 3.0
elif day_of_week in [6, 7]:
return 2.0
else:
return 1.5
这个函数接受两个参数 day_of_week
和 is_holiday
,分别表示星期几和是否节假日,并返回加班费率。
综合加班工资的计算
综合加班工资的计算需要结合加班时间和加班费率,计算出总的加班工资。
def calculate_total_overtime_salary(start_time, end_time, standard_work_hours, base_salary, day_of_week, is_holiday):
overtime_hours = calculate_overtime_hours(start_time, end_time, standard_work_hours)
overtime_rate = calculate_overtime_rate(day_of_week, is_holiday)
overtime_salary = calculate_overtime_salary(overtime_hours, base_salary * overtime_rate / standard_work_hours)
return overtime_salary
这个函数综合了加班时间和加班费率的计算,接受六个参数 start_time
、end_time
、standard_work_hours
、base_salary
、day_of_week
和 is_holiday
,分别表示上下班时间、标准工作时间、基本工资、星期几和是否节假日,并返回总的加班工资。
详细描述税收扣除的计算
税收扣除是根据国家的相关政策规定进行扣除的,通常情况下,税收扣除是根据员工的收入情况和税收政策进行计算的。
税率表的定义
税率表是根据国家的税收政策规定的不同收入区间的税率和速算扣除数。
tax_brackets = [
(3000, 0.03, 0),
(12000, 0.1, 210),
(25000, 0.2, 1410),
(35000, 0.25, 2660),
(55000, 0.3, 4410),
(80000, 0.35, 7160),
(float('inf'), 0.45, 15160)
]
税率表是一个列表,每个元素是一个元组,包含三个值,分别表示收入区间、税率和速算扣除数。
税收扣除的计算
税收扣除是根据员工的总收入和税率表进行计算的。
def calculate_tax_deduction(total_income):
for bracket in tax_brackets:
if total_income <= bracket[0]:
return total_income * bracket[1] - bracket[2]
return 0
这个函数接受一个参数 total_income
,表示员工的总收入,并返回税收扣除金额。
详细描述其他扣除项的计算
其他扣除项是根据公司的相关政策规定进行扣除的,通常情况下,其他扣除项包括社保、公积金等。
社保和公积金的计算
社保和公积金是根据公司的相关政策规定进行扣除的,通常情况下,社保和公积金是根据员工的收入情况和公司政策进行计算的。
def calculate_social_security(base_salary, social_security_rate):
return base_salary * social_security_rate
def calculate_housing_fund(base_salary, housing_fund_rate):
return base_salary * housing_fund_rate
这两个函数分别接受两个参数 base_salary
和 social_security_rate
、housing_fund_rate
,表示基本工资和社保、公积金的费率,并返回社保和公积金的扣除金额。
综合其他扣除项的计算
综合其他扣除项的计算需要结合社保和公积金的计算,计算出总的其他扣除项。
def calculate_other_deductions(base_salary, social_security_rate, housing_fund_rate):
social_security = calculate_social_security(base_salary, social_security_rate)
housing_fund = calculate_housing_fund(base_salary, housing_fund_rate)
return social_security + housing_fund
这个函数综合了社保和公积金的计算,接受三个参数 base_salary
、social_security_rate
和 housing_fund_rate
,表示基本工资、社保和公积金的费率,并返回总的其他扣除项。
完整的工资计算示例
下面是一个完整的工资计算示例,综合了基本工资、加班工资、税收扣除和其他扣除项的计算。
def calculate_total_salary(base_salary, start_time, end_time, standard_work_hours, day_of_week, is_holiday, social_security_rate, housing_fund_rate):
basic_salary = calculate_basic_salary(base_salary)
overtime_salary = calculate_total_overtime_salary(start_time, end_time, standard_work_hours, base_salary, day_of_week, is_holiday)
total_income = basic_salary + overtime_salary
tax_deduction = calculate_tax_deduction(total_income)
other_deductions = calculate_other_deductions(base_salary, social_security_rate, housing_fund_rate)
total_salary = total_income - tax_deduction - other_deductions
return total_salary
示例
base_salary = 5000
start_time = "09:00"
end_time = "20:00"
standard_work_hours = 8
day_of_week = 3 # 星期三
is_holiday = False
social_security_rate = 0.105
housing_fund_rate = 0.07
total_salary = calculate_total_salary(base_salary, start_time, end_time, standard_work_hours, day_of_week, is_holiday, social_security_rate, housing_fund_rate)
print(f"总工资: {total_salary:.2f} 元")
这个示例综合了基本工资、加班工资、税收扣除和其他扣除项的计算,并返回总工资。通过这个示例,我们可以看到如何使用Python进行工资的计算。
总结起来,要用Python计算工资,可以采用基本工资、加班工资、税收扣除和其他扣除等因素来计算,其中加班工资的计算是比较复杂的,需要根据加班时间和加班费率进行精确计算。通过定义函数和调用函数,我们可以很方便地计算出员工的总工资。
相关问答FAQs:
如何使用Python编写计算工资的程序?
要编写一个计算工资的程序,您可以使用Python的基本输入输出功能和简单的数学运算。首先,您需要获取员工的基本信息,例如小时工资和工作小时数。接着,您可以通过将小时工资乘以工作小时数来计算总工资。以下是一个简单的示例代码:
hourly_wage = float(input("请输入每小时工资: "))
hours_worked = float(input("请输入工作小时数: "))
total_salary = hourly_wage * hours_worked
print(f"总工资是: {total_salary}")
在Python中如何处理加班工资的计算?
加班工资的计算通常涉及到一个更高的工资率。一般情况下,加班时间是超过40小时的部分。您可以在计算总工资时添加一个条件,若工作小时数超过40小时,则为超出部分计算加班工资。例如:
regular_hours = 40
overtime_rate = 1.5
if hours_worked > regular_hours:
overtime_hours = hours_worked - regular_hours
total_salary = (hourly_wage * regular_hours) + (hourly_wage * overtime_rate * overtime_hours)
else:
total_salary = hourly_wage * hours_worked
如何在Python中处理税收和扣款计算?
在计算工资时,税收和其他扣款也是需要考虑的因素。您可以在计算总工资后,根据税率或固定的扣款金额进行调整。举例来说,假设税率为20%,可以在总工资的基础上进行如下计算:
tax_rate = 0.2
net_salary = total_salary - (total_salary * tax_rate)
print(f"扣税后的净工资是: {net_salary}")
通过这种方式,您可以创建一个全面的工资计算器,帮助用户了解他们的实际收入。
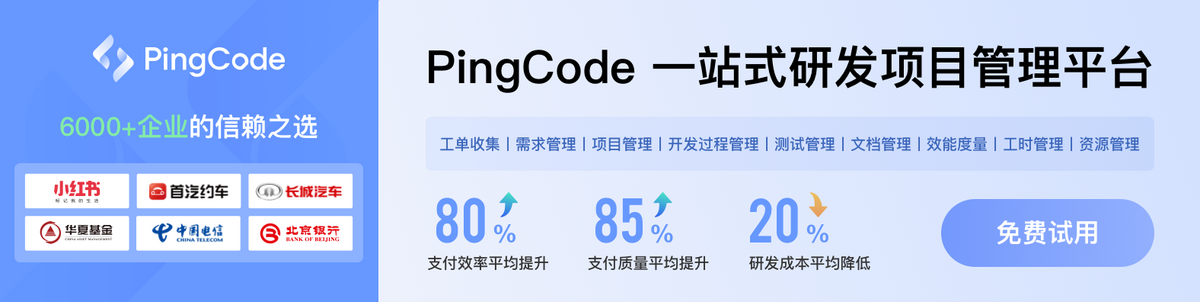