在Python中实现搜索的方法包括:使用内置的字符串方法、使用正则表达式、使用内置数据结构如列表和字典、以及使用外部库如whoosh
或elasticsearch
。 其中,使用内置字符串方法最为简单和直接,例如可以使用str.find()
或str.index()
来查找子字符串的位置。使用正则表达式则可以处理更复杂的匹配模式。列表和字典提供了灵活的数据存储和查找的功能。最后,外部库如whoosh
和elasticsearch
则适用于更加复杂和大规模的搜索需求。
下面将详细展开使用内置字符串方法来实现搜索。
一、使用内置字符串方法
Python提供了多种内置的字符串方法来实现搜索,包括find()
、index()
、startswith()
、endswith()
等。
1. find()
方法
find()
方法用于返回子字符串在字符串中的最低索引,如果子字符串不在字符串中,则返回-1。例如:
text = "Hello, welcome to the world of Python"
sub_text = "Python"
position = text.find(sub_text)
print(position) # 输出:29
2. index()
方法
与find()
方法类似,但如果子字符串不在字符串中,会抛出一个ValueError
异常。例如:
text = "Hello, welcome to the world of Python"
sub_text = "Python"
try:
position = text.index(sub_text)
print(position) # 输出:29
except ValueError:
print("Substring not found")
3. startswith()
方法
检查字符串是否以指定的子字符串开头,返回布尔值。例如:
text = "Hello, welcome to the world of Python"
result = text.startswith("Hello")
print(result) # 输出:True
4. endswith()
方法
检查字符串是否以指定的子字符串结尾,返回布尔值。例如:
text = "Hello, welcome to the world of Python"
result = text.endswith("Python")
print(result) # 输出:True
二、使用正则表达式
正则表达式是一种强大的字符串匹配工具,可以处理复杂的搜索模式。Python的re
模块提供了实现正则表达式功能的多种方法。
1. re.search()
re.search()
方法扫描整个字符串,返回匹配的第一个对象,如果没有匹配则返回None
。例如:
import re
text = "Hello, welcome to the world of Python"
pattern = r"world"
match = re.search(pattern, text)
if match:
print("Found:", match.group()) # 输出:Found: world
else:
print("Not found")
2. re.match()
re.match()
方法从字符串的起始位置进行匹配。如果起始位置匹配成功,返回匹配对象,否则返回None
。例如:
import re
text = "Hello, welcome to the world of Python"
pattern = r"Hello"
match = re.match(pattern, text)
if match:
print("Found:", match.group()) # 输出:Found: Hello
else:
print("Not found")
3. re.findall()
re.findall()
方法返回所有非重叠的匹配项,作为字符串列表。如果没有匹配项,则返回空列表。例如:
import re
text = "Hello, welcome to the world of Python. Python is powerful."
pattern = r"Python"
matches = re.findall(pattern, text)
print(matches) # 输出:['Python', 'Python']
4. re.finditer()
re.finditer()
方法返回一个迭代器,产生匹配对象。可以通过遍历迭代器来获取所有匹配项。例如:
import re
text = "Hello, welcome to the world of Python. Python is powerful."
pattern = r"Python"
matches = re.finditer(pattern, text)
for match in matches:
print("Found:", match.group(), "at position", match.start())
输出:
Found: Python at position 29
Found: Python at position 38
三、使用内置数据结构
Python的内置数据结构如列表和字典也提供了高效的搜索功能。
1. 列表搜索
列表是Python中常用的数据结构,提供了简单的搜索方法。例如:
fruits = ["apple", "banana", "cherry", "date"]
if "banana" in fruits:
print("Banana found") # 输出:Banana found
else:
print("Banana not found")
2. 字典搜索
字典是键值对的数据结构,提供了高效的查找操作。例如:
person = {"name": "Alice", "age": 25, "city": "New York"}
if "age" in person:
print("Age found:", person["age"]) # 输出:Age found: 25
else:
print("Age not found")
四、使用外部库
对于更复杂和大规模的搜索需求,可以使用外部库如whoosh
和elasticsearch
。
1. Whoosh
Whoosh是一个快速的纯Python文本索引和搜索库,适用于中小规模的文本搜索。安装Whoosh:
pip install Whoosh
使用Whoosh进行搜索的基本步骤如下:
from whoosh.index import create_in
from whoosh.fields import Schema, TEXT
from whoosh.qparser import QueryParser
定义Schema
schema = Schema(title=TEXT(stored=True), content=TEXT)
创建索引
import os
if not os.path.exists("index"):
os.mkdir("index")
ix = create_in("index", schema)
添加文档
writer = ix.writer()
writer.add_document(title="First document", content="Hello, this is the first document.")
writer.add_document(title="Second document", content="Hello, this is the second document.")
writer.commit()
搜索文档
searcher = ix.searcher()
query = QueryParser("content", ix.schema).parse("first")
results = searcher.search(query)
for result in results:
print(result["title"])
输出:First document
2. Elasticsearch
Elasticsearch是一个分布式、RESTful风格的搜索和分析引擎,适用于大规模数据搜索。安装Elasticsearch的Python客户端:
pip install elasticsearch
使用Elasticsearch进行搜索的基本步骤如下:
from elasticsearch import Elasticsearch
连接到Elasticsearch
es = Elasticsearch([{'host': 'localhost', 'port': 9200}])
创建索引
index_body = {
"mappings": {
"properties": {
"title": {"type": "text"},
"content": {"type": "text"}
}
}
}
es.indices.create(index='documents', body=index_body)
添加文档
doc1 = {"title": "First document", "content": "Hello, this is the first document."}
doc2 = {"title": "Second document", "content": "Hello, this is the second document."}
es.index(index='documents', body=doc1)
es.index(index='documents', body=doc2)
搜索文档
search_body = {
"query": {
"match": {
"content": "first"
}
}
}
res = es.search(index='documents', body=search_body)
for hit in res['hits']['hits']:
print(hit['_source']['title'])
输出:First document
结论
在Python中实现搜索的方法有很多,从简单的内置字符串方法到复杂的分布式搜索引擎,每种方法都有其适用的场景。选择合适的方法取决于具体的搜索需求和数据规模。对于简单的小规模搜索,内置方法和正则表达式已经足够;对于涉及数据存储和快速查找的需求,使用列表和字典是不错的选择;而对于需要高性能、大规模的搜索,使用外部库如Whoosh和Elasticsearch则是最佳选择。
相关问答FAQs:
如何在Python中实现基本的搜索功能?
在Python中,可以使用多种方法来实现搜索功能。最常见的方式是使用列表或字典数据结构,并结合循环或条件语句来查找元素。例如,对于一个列表,可以使用in
关键字来检查元素是否存在,或者使用index()
方法来获取元素的位置。如果要在字典中查找值,可以直接使用键来访问对应的值。
Python中有哪些库可以帮助实现更复杂的搜索?
对于更复杂的搜索需求,Python提供了许多强大的库。例如,Whoosh
和Elasticsearch
可以用于文本搜索,支持索引和查询功能。如果需要处理大量数据,pandas
库中的DataFrame
对象也可以实现快速的搜索和筛选操作,使数据分析变得更高效。
在Python中如何实现模糊搜索?
模糊搜索可以通过字符串匹配来实现。可以使用fuzzywuzzy
库,它提供了字符串相似度计算功能,能够识别相似的字符串。此外,正则表达式也是一种强大的工具,可以用来实现复杂模式的搜索。通过使用re
模块,开发者可以定义自定义的搜索模式,匹配各种字符串格式。
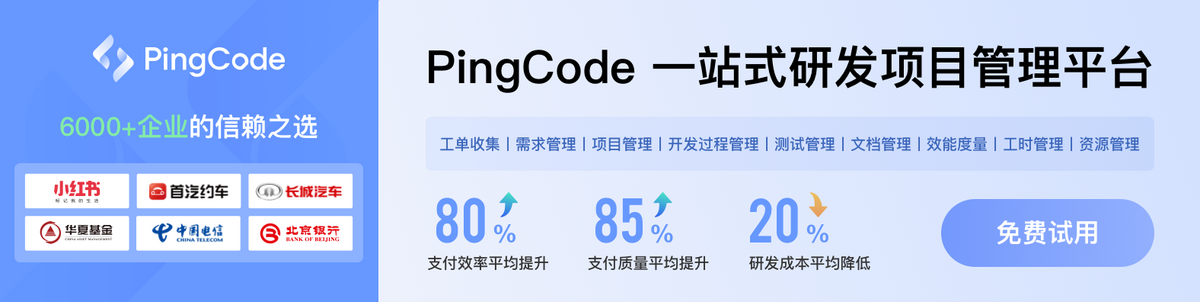