Python可以通过多种方式自动标记星号,如字符串操作、正则表达式、文本处理库等。其中一种常用的方法是使用字符串操作,通过遍历字符串并对字符进行处理,将需要标记的字符替换为星号。以下是这种方法的详细描述:
字符串操作方法:可以通过遍历字符串中的每个字符,检查是否符合需要标记的条件,然后将符合条件的字符替换为星号。比如,可以将所有的元音字母标记为星号。代码示例如下:
def replace_vowels_with_star(text):
vowels = "AEIOUaeiou"
result = ""
for char in text:
if char in vowels:
result += "*"
else:
result += char
return result
text = "Hello World"
print(replace_vowels_with_star(text))
在上面的代码中,我们定义了一个函数 replace_vowels_with_star
,它将输入字符串中的所有元音字母替换为星号。通过遍历字符串中的每个字符,检查是否为元音字母,如果是,则用星号替换,否则保留原字符。
接下来,我们将详细探讨其他几种方法:
一、字符串操作
字符串操作是最基本的文本处理方式,通过遍历字符串并对字符进行处理,可以实现各种标记星号的需求。
1. 替换特定字符
我们可以使用字符串的 replace
方法来替换特定字符为星号。举个例子,假设我们需要将字符串中的所有字母 'a' 替换为星号:
def replace_a_with_star(text):
return text.replace('a', '*')
text = "A cat and a hat."
print(replace_a_with_star(text))
在这个例子中,replace
方法将字符串中的所有 'a' 替换为星号。输出结果为 A c*t *nd * h*t.
。
2. 替换特定模式
有时我们需要替换特定模式的字符为星号,比如将所有数字替换为星号。我们可以使用字符串操作结合条件判断来实现这一点:
def replace_numbers_with_star(text):
result = ""
for char in text:
if char.isdigit():
result += "*"
else:
result += char
return result
text = "My phone number is 123-456-7890."
print(replace_numbers_with_star(text))
在这个例子中,我们遍历字符串中的每个字符,检查是否为数字字符,如果是,则用星号替换,否则保留原字符。输出结果为 My phone number is <strong>*-</strong>*-<strong></strong>.
。
二、正则表达式
正则表达式是一种强大的文本处理工具,可以用来匹配复杂的字符模式,并进行替换操作。Python 中的 re
模块提供了对正则表达式的支持。
1. 替换特定模式
使用正则表达式,我们可以更方便地替换特定模式的字符。比如,我们可以使用正则表达式来替换字符串中的所有数字为星号:
import re
def replace_numbers_with_star(text):
return re.sub(r'\d', '*', text)
text = "My phone number is 123-456-7890."
print(replace_numbers_with_star(text))
在这个例子中,re.sub
函数使用正则表达式 \d
匹配所有数字,并将其替换为星号。输出结果为 My phone number is <strong>*-</strong>*-<strong></strong>.
。
2. 替换复杂模式
正则表达式可以用来匹配更复杂的模式,比如替换所有单词中的元音字母为星号:
import re
def replace_vowels_with_star(text):
return re.sub(r'[AEIOUaeiou]', '*', text)
text = "Hello World"
print(replace_vowels_with_star(text))
在这个例子中,re.sub
函数使用正则表达式 [AEIOUaeiou]
匹配所有元音字母,并将其替换为星号。输出结果为 H*ll* W*rld
。
三、文本处理库
Python 有许多强大的文本处理库,可以用来处理复杂的文本标记需求。比如,我们可以使用 nltk
库来标记文本中的特定单词。
1. 使用 NLTK 标记单词
nltk
(Natural Language Toolkit)是一个用于处理自然语言文本的库。我们可以使用 nltk
来标记文本中的特定单词。
首先,我们需要安装 nltk
库:
pip install nltk
然后,我们可以使用 nltk
来标记文本中的特定单词:
import nltk
from nltk.tokenize import word_tokenize
nltk.download('punkt')
def replace_specific_words_with_star(text, words_to_replace):
tokens = word_tokenize(text)
result = []
for token in tokens:
if token in words_to_replace:
result.append("*" * len(token))
else:
result.append(token)
return " ".join(result)
text = "Hello World"
words_to_replace = ["Hello"]
print(replace_specific_words_with_star(text, words_to_replace))
在这个例子中,我们使用 nltk
的 word_tokenize
函数将文本分割为单词,然后遍历每个单词,检查是否为需要标记的单词,如果是,则用星号替换,否则保留原单词。输出结果为 <strong></strong>* World
。
2. 使用 SpaCy 标记实体
spacy
是另一个强大的自然语言处理库,可以用来标记文本中的命名实体。
首先,我们需要安装 spacy
库:
pip install spacy
python -m spacy download en_core_web_sm
然后,我们可以使用 spacy
来标记文本中的命名实体:
import spacy
nlp = spacy.load("en_core_web_sm")
def replace_entities_with_star(text):
doc = nlp(text)
result = text
for ent in doc.ents:
result = result.replace(ent.text, "*" * len(ent.text))
return result
text = "Barack Obama was born in Hawaii."
print(replace_entities_with_star(text))
在这个例子中,我们使用 spacy
的 nlp
对象处理文本,将文本中的命名实体标记为星号。输出结果为 <strong></strong><strong> </strong><strong>* was born in </strong><strong></strong>.
。
四、结合多种方法
在实际应用中,我们可以结合多种方法来实现复杂的文本标记需求。下面是一个结合字符串操作和正则表达式的方法示例:
import re
def replace_vowels_and_numbers_with_star(text):
text = re.sub(r'[AEIOUaeiou]', '*', text)
text = re.sub(r'\d', '*', text)
return text
text = "Hello World 123"
print(replace_vowels_and_numbers_with_star(text))
在这个例子中,我们首先使用正则表达式将所有元音字母替换为星号,然后使用正则表达式将所有数字替换为星号。输出结果为 H*ll* W*rld *
。
五、处理大规模文本
对于大规模文本处理,我们可以使用并行计算技术来提高效率。Python 的 multiprocessing
模块提供了对并行计算的支持。
1. 使用 multiprocessing 模块
我们可以使用 multiprocessing
模块来并行处理大规模文本。下面是一个示例:
import re
from multiprocessing import Pool
def replace_vowels_with_star(text):
return re.sub(r'[AEIOUaeiou]', '*', text)
def process_texts(texts):
with Pool() as pool:
results = pool.map(replace_vowels_with_star, texts)
return results
texts = ["Hello World", "Python is fun", "Multiprocessing is powerful"]
print(process_texts(texts))
在这个例子中,我们定义了一个 replace_vowels_with_star
函数来替换文本中的元音字母,并使用 multiprocessing
模块的 Pool
对象来并行处理多个文本。输出结果为 ['H*ll* W*rld', 'Pyth*n *s f*n', 'M*lt*pr*c*ss*ng *s p*w*rf*l']
。
通过以上几种方法,我们可以灵活地实现各种文本标记需求。根据具体需求选择合适的方法,可以提高文本处理的效率和准确性。
相关问答FAQs:
如何使用Python自动为文本添加星号?
在Python中,可以通过字符串操作和正则表达式来为特定文本添加星号。使用str.replace()
方法可以替换特定字符或单词为带星号的形式,或者使用re
模块进行更复杂的匹配和替换。例如,可以将每个出现的“重要”一词替换为“重要”。
Python中有没有现成的库可以帮助我自动标星号?
是的,有一些第三方库可以简化这个过程。比如,nltk
(自然语言工具包)可以用来处理文本,识别关键词并自动标记星号。通过设置自定义规则,可以快速对文本进行处理,自动添加星号。
在Python中如何批量处理文件并为内容添加星号?
可以利用Python的文件操作功能来读取多个文件的内容,并对每个文件进行处理。通过os
模块遍历文件夹中的文件,结合字符串操作方法,可以为每个文件中的特定内容添加星号。处理后,再将结果写入新文件或覆盖原文件,以完成批量处理。
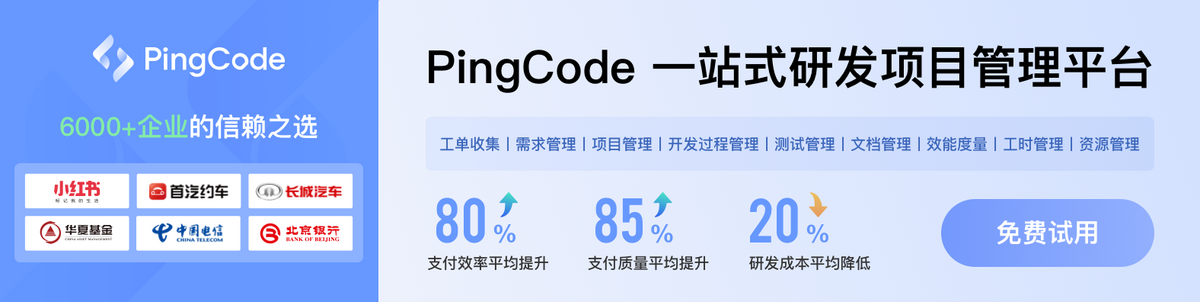