Python运行圆形轨迹的方法包括:使用数学公式计算坐标、利用图形库绘制路径、实现动画效果等。其中,利用数学公式计算圆形轨迹的坐标是最基本的方法,也是其他方法的基础。下面将详细介绍如何通过这些方法实现圆形轨迹的运行。
数学公式计算坐标
运行圆形轨迹的核心在于计算圆上各点的坐标。对于一个圆心在 (h, k),半径为 r 的圆,其参数方程可以表示为:
[ x = h + r \cos(\theta) ]
[ y = k + r \sin(\theta) ]
其中,θ 是从 0 到 2π 的角度,通过改变 θ 的值,可以得到圆上不同点的坐标。
通过这种方法,可以得到圆上所有点的坐标,并使用这些坐标来绘制圆形轨迹。
利用图形库绘制路径
Python 有多个图形库可以用来绘制圆形轨迹,例如 matplotlib、turtle 等。使用这些库,可以将之前计算的坐标点绘制出来,并实现圆形轨迹的可视化。
以下是详细介绍:
一、利用数学公式计算坐标
计算圆形轨迹的坐标是绘制和运行圆形轨迹的基础。通过圆的参数方程,可以得到圆上任意点的坐标。
1、基本计算方法
假设圆的圆心在 (h, k),半径为 r,圆的参数方程为:
[ x = h + r \cos(\theta) ]
[ y = k + r \sin(\theta) ]
通过改变 θ 的值,可以得到圆上不同点的坐标。θ 的范围是从 0 到 2π。
import math
圆心坐标
h = 0
k = 0
半径
r = 5
生成圆上点的坐标
coordinates = []
for theta in range(0, 360):
theta_rad = math.radians(theta)
x = h + r * math.cos(theta_rad)
y = k + r * math.sin(theta_rad)
coordinates.append((x, y))
print(coordinates)
在这个例子中,我们计算了圆周上 360 个点的坐标,并将它们存储在一个列表中。
2、优化计算方法
为了提高计算效率,可以使用 numpy 库进行批量计算。
import numpy as np
圆心坐标
h = 0
k = 0
半径
r = 5
生成 θ 的值
theta = np.linspace(0, 2 * np.pi, 360)
计算圆上点的坐标
x = h + r * np.cos(theta)
y = k + r * np.sin(theta)
coordinates = np.column_stack((x, y))
print(coordinates)
在这个例子中,我们使用 numpy 库生成了 θ 的值,并批量计算了圆上点的坐标。这样可以大大提高计算效率。
二、利用图形库绘制路径
Python 提供了多个图形库,可以用来绘制圆形轨迹。这里介绍两种常用的图形库:matplotlib 和 turtle。
1、使用 matplotlib 绘制圆形轨迹
matplotlib 是一个广泛使用的绘图库,可以方便地绘制各种图形。
import matplotlib.pyplot as plt
import numpy as np
圆心坐标
h = 0
k = 0
半径
r = 5
生成 θ 的值
theta = np.linspace(0, 2 * np.pi, 360)
计算圆上点的坐标
x = h + r * np.cos(theta)
y = k + r * np.sin(theta)
绘制圆形轨迹
plt.figure()
plt.plot(x, y)
plt.title('Circular Trajectory')
plt.xlabel('X')
plt.ylabel('Y')
plt.axis('equal')
plt.grid(True)
plt.show()
在这个例子中,我们使用 matplotlib 库绘制了一个圆形轨迹,并设置了标题、坐标轴标签和网格。
2、使用 turtle 绘制圆形轨迹
turtle 是一个简单的图形库,特别适合教学和简单图形的绘制。
import turtle
设置画布
screen = turtle.Screen()
screen.title('Circular Trajectory')
screen.bgcolor('white')
创建画笔
pen = turtle.Turtle()
pen.speed(0)
pen.color('blue')
圆心坐标
h = 0
k = 0
半径
r = 100
绘制圆形轨迹
pen.penup()
pen.goto(h + r, k)
pen.pendown()
pen.circle(r)
完成绘制
turtle.done()
在这个例子中,我们使用 turtle 库绘制了一个圆形轨迹,并设置了画布和画笔的属性。
三、实现动画效果
除了静态绘制圆形轨迹,还可以使用动画效果来显示圆形轨迹的运行。这里介绍如何使用 matplotlib 和 turtle 库实现动画效果。
1、使用 matplotlib 实现动画效果
matplotlib 提供了一个 animation 模块,可以用来创建动画效果。
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
圆心坐标
h = 0
k = 0
半径
r = 5
生成 θ 的值
theta = np.linspace(0, 2 * np.pi, 360)
计算圆上点的坐标
x = h + r * np.cos(theta)
y = k + r * np.sin(theta)
创建图形和轴
fig, ax = plt.subplots()
ax.set_xlim(-r - 1, r + 1)
ax.set_ylim(-r - 1, r + 1)
line, = ax.plot([], [], 'bo')
初始化函数
def init():
line.set_data([], [])
return line,
动画更新函数
def update(frame):
line.set_data(x[:frame], y[:frame])
return line,
创建动画
ani = animation.FuncAnimation(fig, update, frames=len(theta), init_func=init, blit=True)
plt.show()
在这个例子中,我们使用 matplotlib 的 animation 模块创建了一个动画效果,显示了圆形轨迹的运行过程。
2、使用 turtle 实现动画效果
turtle 库也可以用来创建简单的动画效果。
import turtle
import math
设置画布
screen = turtle.Screen()
screen.title('Circular Trajectory Animation')
screen.bgcolor('white')
创建画笔
pen = turtle.Turtle()
pen.speed(0)
pen.color('blue')
圆心坐标
h = 0
k = 0
半径
r = 100
绘制圆形轨迹的动画
for theta in range(0, 360):
theta_rad = math.radians(theta)
x = h + r * math.cos(theta_rad)
y = k + r * math.sin(theta_rad)
pen.goto(x, y)
完成绘制
turtle.done()
在这个例子中,我们使用 turtle 库创建了一个简单的动画效果,显示了圆形轨迹的运行过程。
四、综合应用
通过上述方法,可以实现圆形轨迹的计算、绘制和动画效果。下面是一个综合应用的例子,展示如何使用这些方法实现一个完整的圆形轨迹动画。
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
import turtle
import math
def calculate_coordinates(h, k, r, num_points=360):
theta = np.linspace(0, 2 * np.pi, num_points)
x = h + r * np.cos(theta)
y = k + r * np.sin(theta)
return x, y
def plot_circle(x, y):
plt.figure()
plt.plot(x, y)
plt.title('Circular Trajectory')
plt.xlabel('X')
plt.ylabel('Y')
plt.axis('equal')
plt.grid(True)
plt.show()
def animate_circle(x, y):
fig, ax = plt.subplots()
ax.set_xlim(min(x) - 1, max(x) + 1)
ax.set_ylim(min(y) - 1, max(y) + 1)
line, = ax.plot([], [], 'bo')
def init():
line.set_data([], [])
return line,
def update(frame):
line.set_data(x[:frame], y[:frame])
return line,
ani = animation.FuncAnimation(fig, update, frames=len(x), init_func=init, blit=True)
plt.show()
def turtle_circle_animation(h, k, r):
screen = turtle.Screen()
screen.title('Circular Trajectory Animation')
screen.bgcolor('white')
pen = turtle.Turtle()
pen.speed(0)
pen.color('blue')
pen.penup()
pen.goto(h + r, k)
pen.pendown()
for theta in range(0, 360):
theta_rad = math.radians(theta)
x = h + r * math.cos(theta_rad)
y = k + r * math.sin(theta_rad)
pen.goto(x, y)
turtle.done()
圆心坐标
h = 0
k = 0
半径
r = 5
计算圆上点的坐标
x, y = calculate_coordinates(h, k, r)
绘制圆形轨迹
plot_circle(x, y)
动画效果
animate_circle(x, y)
Turtle 动画效果
turtle_circle_animation(h, k, r)
这个例子展示了如何计算圆形轨迹的坐标,使用 matplotlib 绘制圆形轨迹,并实现动画效果,以及使用 turtle 库实现简单的动画效果。通过这些方法,可以更好地理解和实现圆形轨迹的运行。
相关问答FAQs:
如何在Python中创建圆形轨迹的动画?
在Python中,可以使用多个库来创建圆形轨迹的动画,例如Matplotlib和Pygame。使用Matplotlib,你可以通过绘制一系列点形成圆形,并利用其动画功能使其动态展示。通过设置圆心坐标和半径,可以生成相应的圆形轨迹。Pygame则允许你创建更复杂的动画效果,适合游戏开发等场景。
Python中如何计算圆形轨迹上的点?
计算圆形轨迹上的点可以使用简单的三角函数。给定圆的中心坐标和半径,可以使用以下公式计算点的坐标:
- x = center_x + radius * cos(angle)
- y = center_y + radius * sin(angle)
其中,angle以弧度为单位。通过循环遍历不同的角度值,可以得到圆形轨迹上的所有点。
如何使用Python中的库来实现圆形轨迹的图形绘制?
可以使用Matplotlib的plot
函数来绘制圆形轨迹。首先,计算圆上点的坐标,然后将这些点传递给plot
函数。示例代码如下:
import numpy as np
import matplotlib.pyplot as plt
theta = np.linspace(0, 2*np.pi, 100)
x = np.cos(theta)
y = np.sin(theta)
plt.plot(x, y)
plt.axis('equal') # 确保比例相等
plt.title('Circle Trajectory')
plt.show()
这样就可以在图形窗口中看到一个完整的圆形轨迹。
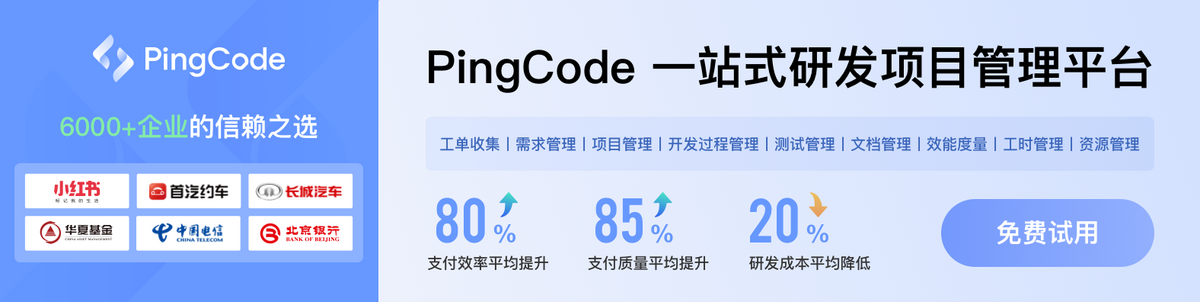