捕获timeout异常的方法包括使用try/except结构、设置timeout参数、使用信号、使用第三方库。 其中,try/except结构是最常用的方法。下面将详细展开。
使用try/except结构时,首先需要导入相关的库和模块。以socket库为例,设置超时并捕获timeout异常的代码如下:
import socket
try:
sock = socket.create_connection(('www.example.com', 80), timeout=10)
except socket.timeout:
print("Connection timed out")
except socket.error as e:
print(f"Socket error: {e}")
在这个例子中,socket.create_connection函数在尝试连接到指定的地址时,会等待最多10秒钟。如果在这段时间内未能建立连接,就会抛出一个socket.timeout异常,该异常将被捕获并处理。
接下来,将从多个方面详细介绍如何在Python中捕获timeout异常。
一、设置timeout参数
在许多网络编程库中,如socket、requests等,都可以设置timeout参数来指定等待时间。
1.1、socket库
socket库是Python标准库中的一个模块,用于进行底层网络通信。可以通过设置timeout参数来指定连接等待的时间。
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.settimeout(5) # 设置超时时间为5秒
try:
sock.connect(('www.example.com', 80))
except socket.timeout:
print("Connection timed out")
except socket.error as e:
print(f"Socket error: {e}")
finally:
sock.close()
1.2、requests库
requests库是一个强大的HTTP库,用于发送HTTP请求。可以在请求方法中设置timeout参数。
import requests
try:
response = requests.get('https://www.example.com', timeout=5)
except requests.Timeout:
print("Request timed out")
except requests.RequestException as e:
print(f"Request error: {e}")
二、使用信号
在某些情况下,可以使用Python的signal模块来设置超时信号。信号模块允许设置一个定时器,在指定时间后触发一个异常。
import signal
class TimeoutException(Exception):
pass
def timeout_handler(signum, frame):
raise TimeoutException
设置超时信号
signal.signal(signal.SIGALRM, timeout_handler)
signal.alarm(5) # 设置超时时间为5秒
try:
# 执行可能超时的操作
result = some_long_running_function()
except TimeoutException:
print("Operation timed out")
finally:
signal.alarm(0) # 取消定时器
三、使用第三方库
除了内置库,Python还有许多第三方库支持超时控制。以下是一些常用的库。
3.1、timeout-decorator库
timeout-decorator库允许为函数调用设置超时时间,并在超时时抛出异常。
import timeout_decorator
@timeout_decorator.timeout(5)
def long_running_function():
# 执行耗时操作
pass
try:
long_running_function()
except timeout_decorator.TimeoutError:
print("Function call timed out")
3.2、concurrent.futures库
concurrent.futures库提供了高层次的并发执行功能,其中的ThreadPoolExecutor和ProcessPoolExecutor都支持设置超时。
from concurrent.futures import ThreadPoolExecutor, TimeoutError
def long_running_function():
# 执行耗时操作
pass
with ThreadPoolExecutor() as executor:
future = executor.submit(long_running_function)
try:
result = future.result(timeout=5)
except TimeoutError:
print("Function call timed out")
四、使用上下文管理器
上下文管理器可以确保在代码块执行完毕后进行清理工作。可以实现一个自定义的上下文管理器来处理超时。
import time
from contextlib import contextmanager
class TimeoutException(Exception):
pass
@contextmanager
def timeout(time_limit):
def signal_handler(signum, frame):
raise TimeoutException
signal.signal(signal.SIGALRM, signal_handler)
signal.alarm(time_limit)
try:
yield
finally:
signal.alarm(0)
try:
with timeout(5):
time.sleep(10)
except TimeoutException:
print("Operation timed out")
五、使用线程
线程是Python中常用的并发编程方法。可以通过设置守护线程来控制超时。
import threading
class TimeoutException(Exception):
pass
def long_running_function():
# 执行耗时操作
pass
def run_with_timeout(func, args=(), kwargs={}, timeout_duration=5):
result = [TimeoutException('Function call timed out')]
def target():
try:
result[0] = func(*args, kwargs)
except Exception as e:
result[0] = e
thread = threading.Thread(target=target)
thread.daemon = True
thread.start()
thread.join(timeout_duration)
if isinstance(result[0], BaseException):
raise result[0]
return result[0]
try:
run_with_timeout(long_running_function, timeout_duration=5)
except TimeoutException as e:
print(e)
六、使用asyncio库
asyncio库是Python标准库中的异步编程库,可以用于编写并发代码。可以通过asyncio.wait_for函数设置超时。
import asyncio
async def long_running_function():
await asyncio.sleep(10)
async def main():
try:
await asyncio.wait_for(long_running_function(), timeout=5)
except asyncio.TimeoutError:
print("Function call timed out")
asyncio.run(main())
通过以上几种方法,可以在Python中捕获timeout异常,并根据需要进行处理。不同的方法适用于不同的场景,可以根据具体需求进行选择。
相关问答FAQs:
如何在Python中设置超时并捕获异常?
在Python中,可以使用signal
模块来设置超时并捕获异常。通过定义一个超时处理函数,可以在指定时间内执行某个操作,如果超时则抛出异常。示例代码如下:
import signal
def handler(signum, frame):
raise TimeoutError("Operation timed out")
signal.signal(signal.SIGALRM, handler)
signal.alarm(5) # 设置超时为5秒
try:
# 这里是需要执行的代码
# 例如:time.sleep(10) # 这段代码会导致超时
pass
except TimeoutError as e:
print(e)
finally:
signal.alarm(0) # 取消定时器
在Python中捕获TimeoutError时有哪些最佳实践?
捕获TimeoutError时,确保在异常处理代码中执行必要的清理操作。例如,在网络请求或文件操作中,可以关闭打开的连接或文件,避免资源泄露。同时,在捕获异常后,可以记录错误信息,便于后续的调试和分析。
是否可以使用threading
模块来处理超时问题?
可以使用threading
模块来创建一个线程执行耗时操作,并通过join
方法设置超时时间。如果主线程超时未返回,可以通过设置一个标志位来中断该线程的操作。示例代码如下:
import threading
def long_running_task():
# 模拟长时间运行的任务
pass
thread = threading.Thread(target=long_running_task)
thread.start()
thread.join(timeout=5) # 设置超时为5秒
if thread.is_alive():
print("Operation timed out")
# 可以在此处处理超时的逻辑
通过以上方法,可以灵活捕获并处理超时异常,确保程序的稳定性和资源的有效利用。
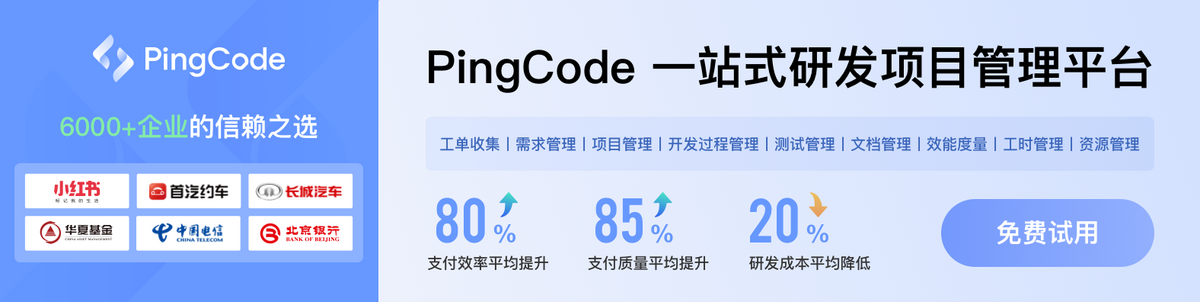