Python正则匹配可以通过使用正则表达式模块 re
来提取字符串中的特定模式、使用 re.compile()
编译正则表达式、使用 re.search()
、re.match()
、re.findall()
和 re.finditer()
等方法来查找匹配项、使用捕获组来提取感兴趣的部分。 例如,使用 re.findall()
方法可以一次性返回所有匹配项,而使用 re.search()
可以找到第一个匹配项并提取子组。下面将详细介绍如何使用这些方法来提取匹配内容。
一、正则表达式基础
在开始之前,了解一下正则表达式的基础知识是非常有必要的。正则表达式是一种用来描述字符串模式的工具,可以用于字符串搜索、替换和提取。正则表达式可以由普通字符和元字符(如 .
、*
、+
、?
等)组成。
1、基本元字符
.
:匹配除换行符以外的任意字符。*
:匹配前面的字符零次或多次。+
:匹配前面的字符一次或多次。?
:匹配前面的字符零次或一次。[]
:匹配括号内的任意字符。^
:匹配字符串的开头。$
:匹配字符串的结尾。
2、预定义字符类
\d
:匹配任何数字,相当于[0-9]
。\D
:匹配任何非数字字符。\w
:匹配任何字母数字字符,相当于[a-zA-Z0-9_]
。\W
:匹配任何非字母数字字符。\s
:匹配任何空白字符。\S
:匹配任何非空白字符。
二、使用 re
模块
Python 提供了 re
模块来处理正则表达式。下面是一些常用的方法:
1、re.compile()
re.compile(pattern, flags=0)
将正则表达式模式编译成正则表达式对象,可以提高效率。flags
可以是正则表达式标志,例如 re.IGNORECASE
用于忽略大小写匹配。
import re
pattern = re.compile(r'\d+')
2、re.search()
re.search(pattern, string, flags=0)
在字符串中搜索第一次出现的正则表达式模式。返回一个匹配对象,如果没有找到匹配项,则返回 None
。
match = re.search(r'\d+', 'Sample 123 text 456')
if match:
print(match.group()) # 输出: 123
3、re.match()
re.match(pattern, string, flags=0)
从字符串的起始位置开始匹配正则表达式模式。只有在字符串的开头匹配成功时才会返回匹配对象,否则返回 None
。
match = re.match(r'Sample', 'Sample text')
if match:
print(match.group()) # 输出: Sample
4、re.findall()
re.findall(pattern, string, flags=0)
返回字符串中所有非重叠的匹配项,以列表形式返回。
matches = re.findall(r'\d+', 'Sample 123 text 456')
print(matches) # 输出: ['123', '456']
5、re.finditer()
re.finditer(pattern, string, flags=0)
返回字符串中所有非重叠的匹配项的迭代器,每个匹配项都是一个匹配对象。
matches = re.finditer(r'\d+', 'Sample 123 text 456')
for match in matches:
print(match.group()) # 输出: 123 456
三、捕获组
捕获组允许你提取匹配的一部分。捕获组用圆括号 ()
表示。
1、基本捕获组
pattern = re.compile(r'(\d+)-(\d+)-(\d+)')
match = pattern.search('Phone number: 123-456-7890')
if match:
print(match.group(0)) # 输出: 123-456-7890
print(match.group(1)) # 输出: 123
print(match.group(2)) # 输出: 456
print(match.group(3)) # 输出: 7890
2、命名捕获组
命名捕获组可以使用 (?P<name>...)
语法。
pattern = re.compile(r'(?P<area_code>\d+)-(?P<exchange>\d+)-(?P<number>\d+)')
match = pattern.search('Phone number: 123-456-7890')
if match:
print(match.group('area_code')) # 输出: 123
print(match.group('exchange')) # 输出: 456
print(match.group('number')) # 输出: 7890
四、使用替换和分割
除了匹配和提取,re
模块还提供了替换和分割字符串的方法。
1、re.sub()
re.sub(pattern, repl, string, count=0, flags=0)
用 repl
替换字符串中符合 pattern
的部分。count
表示最大替换次数,默认为 0 表示替换所有匹配项。
result = re.sub(r'\d+', 'NUMBER', 'Sample 123 text 456')
print(result) # 输出: Sample NUMBER text NUMBER
2、re.split()
re.split(pattern, string, maxsplit=0, flags=0)
根据 pattern
分割字符串,返回分割后的列表。maxsplit
表示最大分割次数,默认为 0 表示分割所有匹配项。
result = re.split(r'\d+', 'Sample 123 text 456')
print(result) # 输出: ['Sample ', ' text ', '']
五、实际应用示例
1、提取电子邮件地址
text = "Please contact us at support@example.com or sales@example.co.uk."
pattern = re.compile(r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b')
emails = pattern.findall(text)
print(emails) # 输出: ['support@example.com', 'sales@example.co.uk']
2、提取URL
text = "Visit our website at https://www.example.com or http://www.example.org."
pattern = re.compile(r'https?://[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}')
urls = pattern.findall(text)
print(urls) # 输出: ['https://www.example.com', 'http://www.example.org']
3、提取电话号码
text = "Call us at 123-456-7890 or 987.654.3210."
pattern = re.compile(r'\d{3}[-.]\d{3}[-.]\d{4}')
phone_numbers = pattern.findall(text)
print(phone_numbers) # 输出: ['123-456-7890', '987.654.3210']
六、结合其他模块使用
正则表达式在实际应用中常常与其他Python模块结合使用,以下是几个例子:
1、结合 pandas
提取数据
import pandas as pd
data = {'text': ['Sample 123 text 456', 'Another 789 text 012']}
df = pd.DataFrame(data)
df['numbers'] = df['text'].apply(lambda x: re.findall(r'\d+', x))
print(df)
输出:
text numbers
0 Sample 123 text 456 [123, 456]
1 Another 789 text 012 [789, 012]
2、结合 json
提取数据
import json
json_data = '{"name": "John", "email": "john.doe@example.com"}'
data = json.loads(json_data)
email_pattern = re.compile(r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b')
email = email_pattern.search(data['email']).group()
print(email) # 输出: john.doe@example.com
七、性能优化
使用正则表达式时需要注意性能问题,以下是一些优化建议:
1、编译正则表达式
将正则表达式编译成模式对象可以提高重复使用时的效率。
pattern = re.compile(r'\d+')
for text in texts:
pattern.findall(text)
2、使用合适的匹配方法
根据需求选择合适的匹配方法,如 re.search()
和 re.match()
只需要找到第一个匹配项,而 re.findall()
和 re.finditer()
会查找所有匹配项。
3、避免过度使用捕获组
捕获组的使用会增加正则表达式的复杂性和匹配时间,只有在需要提取子组时才使用捕获组。
八、常见问题和解决方案
1、匹配多个行
默认情况下,正则表达式中的 .
不匹配换行符。使用 re.DOTALL
标志可以使 .
匹配包括换行符在内的任意字符。
text = "First line.\nSecond line."
pattern = re.compile(r'.+', re.DOTALL)
match = pattern.search(text)
print(match.group()) # 输出: First line.\nSecond line.
2、忽略大小写匹配
使用 re.IGNORECASE
标志可以忽略大小写进行匹配。
text = "Sample Text"
pattern = re.compile(r'sample', re.IGNORECASE)
match = pattern.search(text)
print(match.group()) # 输出: Sample
3、非贪婪匹配
默认情况下,正则表达式的量词是贪婪的,会尽可能多地匹配。使用 ?
可以将量词转换为非贪婪。
text = "<tag>content</tag>"
pattern = re.compile(r'<.*?>')
match = pattern.search(text)
print(match.group()) # 输出: <tag>
通过以上内容,我们详细介绍了Python正则匹配的基础知识、如何使用 re
模块来提取匹配项、捕获组的使用方法、替换和分割字符串的技巧以及实际应用示例。此外,还提供了一些优化建议和常见问题的解决方案。掌握这些技能将帮助你在日常编程中更高效地处理字符串匹配和提取任务。
相关问答FAQs:
如何使用Python正则表达式提取特定模式的字符串?
在Python中,使用re
模块可以轻松提取特定模式的字符串。你可以使用re.search()
、re.match()
或re.findall()
等方法来寻找匹配项。例如,使用re.findall()
可以返回所有匹配的结果,适用于提取多个相似的字符串。确保在定义正则表达式时使用合适的模式,以匹配你需要提取的内容。
在Python中,正则表达式的常见应用场景有哪些?
正则表达式在数据清洗、文本分析和信息提取等方面非常有用。常见的应用场景包括从日志文件中提取IP地址、从用户输入中验证电子邮件格式、以及从网页内容中提取特定信息(如标题、链接等)。这些场景中,灵活使用正则表达式能够大大提高工作效率。
如何调试和优化Python中的正则表达式?
调试和优化正则表达式可以通过在线正则表达式测试工具实现,这些工具可以实时显示匹配结果和解释。使用原子组和非捕获组等技巧可以提升性能和可读性。此外,保持正则表达式的简洁性和可维护性也是优化的重要方面,避免复杂和冗长的表达式会使后续维护更加容易。
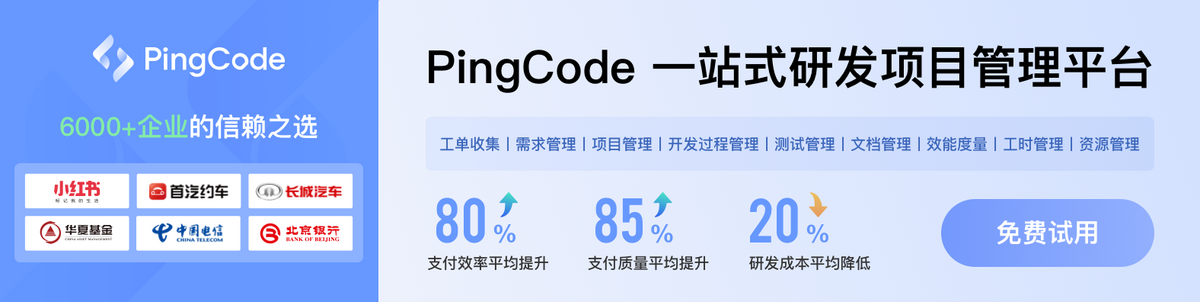