Python读写文件操作主要包括打开文件、读取文件、写入文件、关闭文件等步骤。具体来说,使用open()
函数打开文件、使用read()
或write()
方法进行读写、使用close()
方法关闭文件,操作模式包括读模式、写模式、追加模式等。 例如,在读取文件时,可以使用read()
方法将整个文件内容读取为一个字符串,或者使用readline()
逐行读取文件内容。此外,还可以使用with
语句来确保文件被正确关闭。
一、打开文件
在Python中,打开文件可以使用open()
函数。该函数有两个主要参数:文件名和模式。模式决定了文件的打开方式,如只读、写入、追加等。
file = open("example.txt", "r") # 以读模式打开文件
常见的文件打开模式有:
"r"
: 只读模式,文件必须存在。"w"
: 写入模式,文件不存在则创建,存在则清空内容。"a"
: 追加模式,文件不存在则创建,存在则在文件末尾追加内容。"b"
: 二进制模式,与其他模式结合使用,如"rb"
表示以二进制读模式打开文件。
二、读取文件
读取文件的方法主要有三种:read()
, readline()
和 readlines()
。
1. 使用 read()
方法
read()
方法一次性读取文件的全部内容,并返回一个字符串。
with open("example.txt", "r") as file:
content = file.read()
print(content)
2. 使用 readline()
方法
readline()
方法每次读取一行,通常用于逐行处理文件内容。
with open("example.txt", "r") as file:
line = file.readline()
while line:
print(line, end='')
line = file.readline()
3. 使用 readlines()
方法
readlines()
方法读取文件的所有行,并返回一个包含每行内容的列表。
with open("example.txt", "r") as file:
lines = file.readlines()
for line in lines:
print(line, end='')
三、写入文件
写入文件的方法主要有两种:write()
和 writelines()
。
1. 使用 write()
方法
write()
方法用于将字符串写入文件。
with open("example.txt", "w") as file:
file.write("Hello, World!\n")
file.write("This is a new line.")
2. 使用 writelines()
方法
writelines()
方法用于将一个字符串列表写入文件。
lines = ["Hello, World!\n", "This is a new line.\n"]
with open("example.txt", "w") as file:
file.writelines(lines)
四、关闭文件
在进行文件操作后,务必关闭文件以释放系统资源。使用 close()
方法可以关闭文件。不过更推荐使用 with
语句,因为它会自动关闭文件,即使在发生异常时也能确保文件被正确关闭。
file = open("example.txt", "r")
content = file.read()
file.close()
推荐使用 with
语句:
with open("example.txt", "r") as file:
content = file.read()
五、文件指针
文件指针用于标记文件中当前位置。tell()
方法返回文件指针的当前位置,seek()
方法用于移动文件指针。
with open("example.txt", "r") as file:
print(file.tell()) # 输出当前文件指针位置
file.seek(5) # 将文件指针移动到文件的第6个字节(从0开始计数)
print(file.read()) # 从文件指针位置开始读取文件内容
六、处理二进制文件
处理二进制文件时,需要在模式中添加 b
。例如,读取二进制文件可以使用 rb
模式,写入二进制文件可以使用 wb
模式。
# 读取二进制文件
with open("example.bin", "rb") as file:
binary_content = file.read()
写入二进制文件
with open("example.bin", "wb") as file:
file.write(b'\x00\x01\x02\x03')
七、文件与路径操作
除了读写文件内容,Python的 os
和 os.path
模块还提供了丰富的文件与路径操作功能。
1. 检查文件是否存在
使用 os.path.exists()
方法可以检查文件或目录是否存在。
import os
if os.path.exists("example.txt"):
print("File exists")
else:
print("File does not exist")
2. 获取文件大小
使用 os.path.getsize()
方法可以获取文件的大小(以字节为单位)。
file_size = os.path.getsize("example.txt")
print(f"File size: {file_size} bytes")
3. 创建和删除文件或目录
使用 os.mkdir()
创建目录,使用 os.rmdir()
删除目录,使用 os.remove()
删除文件。
# 创建目录
os.mkdir("example_dir")
删除目录
os.rmdir("example_dir")
删除文件
os.remove("example.txt")
4. 列出目录内容
使用 os.listdir()
列出指定目录中的文件和子目录。
directory_contents = os.listdir(".")
print(directory_contents)
八、文件权限
在文件操作中,设置文件权限也是一个重要方面。os.chmod()
方法可以更改文件权限。
import os
import stat
将文件设置为只读
os.chmod("example.txt", stat.S_IREAD)
将文件设置为可读可写
os.chmod("example.txt", stat.S_IREAD | stat.S_IWRITE)
九、文件路径操作
os.path
模块提供了多种路径操作方法,如获取文件名、目录名、文件扩展名等。
1. 获取文件名和目录名
使用 os.path.basename()
获取文件名,使用 os.path.dirname()
获取目录名。
file_path = "/path/to/example.txt"
file_name = os.path.basename(file_path)
print(f"File name: {file_name}")
directory_name = os.path.dirname(file_path)
print(f"Directory name: {directory_name}")
2. 分离文件路径和文件名
使用 os.path.split()
将文件路径和文件名分离。
file_path = "/path/to/example.txt"
directory, file_name = os.path.split(file_path)
print(f"Directory: {directory}")
print(f"File name: {file_name}")
3. 获取文件扩展名
使用 os.path.splitext()
获取文件扩展名。
file_path = "example.txt"
file_name, file_extension = os.path.splitext(file_path)
print(f"File name: {file_name}")
print(f"File extension: {file_extension}")
十、文件复制与移动
Python的 shutil
模块提供了文件复制与移动的功能。
1. 复制文件
使用 shutil.copy()
方法可以复制文件。
import shutil
shutil.copy("example.txt", "example_copy.txt")
2. 移动文件
使用 shutil.move()
方法可以移动文件。
shutil.move("example.txt", "new_directory/example.txt")
十一、文件压缩与解压
Python的 zipfile
模块提供了文件压缩与解压功能。
1. 压缩文件
使用 zipfile.ZipFile()
创建压缩文件,并使用 write()
方法添加文件。
import zipfile
with zipfile.ZipFile("example.zip", "w") as zipf:
zipf.write("example.txt")
2. 解压文件
使用 zipfile.ZipFile()
打开压缩文件,并使用 extractall()
方法解压文件。
with zipfile.ZipFile("example.zip", "r") as zipf:
zipf.extractall("extracted_files")
十二、文件读取与写入的进阶操作
对于大文件的读取与写入,可以使用分块读取和写入的方法,以避免内存占用过大。
1. 分块读取文件
可以使用 iter()
函数和 functools.partial()
函数分块读取文件。
import functools
with open("example.txt", "r") as file:
for chunk in iter(functools.partial(file.read, 1024), ''):
print(chunk)
2. 分块写入文件
分块写入文件可以将数据分成小块逐块写入文件。
data = "This is a large amount of data." * 1000
with open("example_large.txt", "w") as file:
for i in range(0, len(data), 1024):
file.write(data[i:i+1024])
十三、使用第三方库进行文件操作
除了Python标准库,许多第三方库也提供了高级文件操作功能。例如,pandas
库可以方便地读取和写入CSV文件,openpyxl
库可以操作Excel文件。
1. 使用 pandas
读取和写入CSV文件
import pandas as pd
读取CSV文件
df = pd.read_csv("example.csv")
print(df)
写入CSV文件
df.to_csv("example_output.csv", index=False)
2. 使用 openpyxl
操作Excel文件
import openpyxl
创建一个新的Excel文件
wb = openpyxl.Workbook()
ws = wb.active
ws['A1'] = "Hello, World!"
wb.save("example.xlsx")
读取Excel文件
wb = openpyxl.load_workbook("example.xlsx")
ws = wb.active
print(ws['A1'].value)
十四、文件系统监控
Python的 watchdog
库可以用于监控文件系统的变化,如文件创建、修改、删除等。
1. 安装 watchdog
库
pip install watchdog
2. 使用 watchdog
监控文件系统
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class MyHandler(FileSystemEventHandler):
def on_modified(self, event):
print(f"File modified: {event.src_path}")
def on_created(self, event):
print(f"File created: {event.src_path}")
def on_deleted(self, event):
print(f"File deleted: {event.src_path}")
event_handler = MyHandler()
observer = Observer()
observer.schedule(event_handler, path='.', recursive=False)
observer.start()
try:
while True:
time.sleep(1)
except KeyboardInterrupt:
observer.stop()
observer.join()
十五、总结
Python提供了丰富的文件操作功能,从基本的文件读写到高级的文件系统监控和压缩解压操作。这些功能使得Python在处理文件和目录时非常灵活和高效。在实际应用中,选择合适的方法和库可以大大提高工作效率和代码质量。通过熟练掌握这些文件操作技巧,你可以更轻松地处理各种文件操作任务。
相关问答FAQs:
如何在Python中打开一个文件进行读取?
在Python中,可以使用内置的open()
函数来打开文件。读取文件时,通常使用'r'
模式打开文件。例如,with open('example.txt', 'r') as file:
这段代码将以读取模式打开名为example.txt
的文件。使用with
语句可以确保文件在读取完成后自动关闭,避免资源泄露。读取内容可以通过file.read()
、file.readline()
或file.readlines()
等方法实现。
Python中如何写入文件并确保数据保存?
要在Python中写入文件,使用open()
函数并指定'w'
或'a'
模式。'w'
模式会覆盖已有内容,而'a'
模式则会在文件末尾追加内容。例如,使用with open('output.txt', 'w') as file:
可以创建或覆盖output.txt
文件。写入内容时,可以使用file.write('文本内容')
或file.writelines(['行1\n', '行2\n'])
方法。确保使用with
语句,这样数据将被自动保存并且文件会在操作完成后关闭。
如何处理Python文件操作中的异常?
在进行文件读写操作时,可能会遇到各种异常,如文件未找到或权限不足等。为了处理这些异常,可以使用try
和except
语句。例如,使用try:
来尝试打开文件,若出现FileNotFoundError
或IOError
等异常,则在except
中进行处理。这样可以确保程序在遇到问题时不会崩溃,并且能够提供适当的错误信息或进行其他处理。
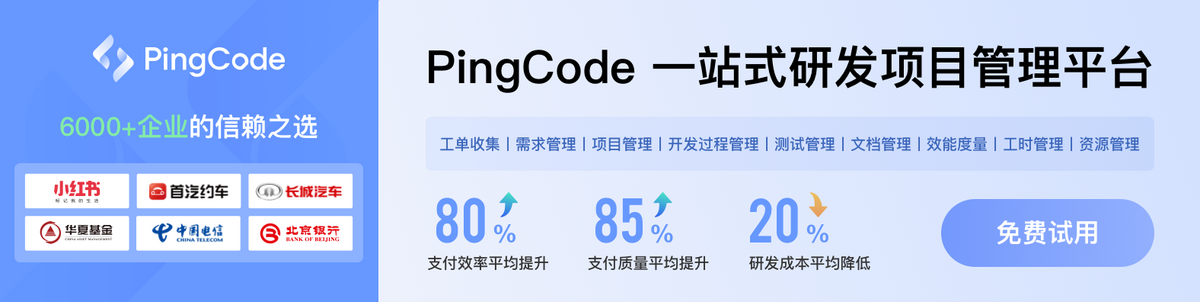