Python可以通过多种方式停止其他程序,比如使用os模块、subprocess模块、psutil模块等。在实际应用中,选择合适的模块和方法对于确保程序的稳定性和安全性非常重要。下面是一些详细的介绍和代码示例,帮助你理解如何使用这些模块停止其他程序。
一、os模块
os模块提供了与操作系统进行交互的多种方法,可以使用os.kill()函数来停止其他程序。这个函数需要传递两个参数:进程ID(PID)和信号。
import os
import signal
def stop_process(pid):
try:
os.kill(pid, signal.SIGTERM)
print(f"Process {pid} has been terminated.")
except ProcessLookupError:
print(f"No process with PID {pid} was found.")
except PermissionError:
print(f"Permission denied to terminate process {pid}.")
详细描述:
os.kill()函数通过向指定的进程发送信号来终止它。常见的信号包括SIGTERM(请求进程终止)、SIGKILL(强制终止进程)等。SIGTERM是一个更友好的信号,允许进程进行清理操作,而SIGKILL则会强制终止进程。
二、subprocess模块
subprocess模块可以启动新进程、与进程进行通信并获取它们的返回码。通过调用subprocess.Popen对象的terminate()或kill()方法,可以停止进程。
import subprocess
def stop_process(process):
try:
process.terminate()
print("Process has been terminated.")
except Exception as e:
print(f"Error occurred: {e}")
Example usage
process = subprocess.Popen(["some_program"])
stop_process(process)
详细描述:
subprocess模块提供了更高层次的接口来启动和管理进程。Popen对象表示创建的子进程,terminate()方法会发送SIGTERM信号,而kill()方法会发送SIGKILL信号。
三、psutil模块
psutil是一个跨平台库,用于检索有关正在运行的进程和系统利用率(CPU、内存、磁盘、网络、传感器等)的信息。可以使用psutil.Process对象来终止进程。
import psutil
def stop_process(pid):
try:
process = psutil.Process(pid)
process.terminate()
print(f"Process {pid} has been terminated.")
except psutil.NoSuchProcess:
print(f"No process with PID {pid} was found.")
except psutil.AccessDenied:
print(f"Permission denied to terminate process {pid}.")
except Exception as e:
print(f"Error occurred: {e}")
Example usage
pid = 1234 # Replace with the actual PID of the process you want to terminate
stop_process(pid)
详细描述:
psutil模块提供了更丰富的功能来管理进程和获取系统信息。psutil.Process对象的terminate()方法会发送SIGTERM信号,而kill()方法会发送SIGKILL信号。psutil还可以处理各种异常情况,比如进程不存在或权限不足。
四、通过系统命令
在某些情况下,使用系统命令可能是最直接的方式来停止其他程序。可以使用os.system()或subprocess.run()来执行系统命令。
import os
def stop_process(pid):
command = f"kill {pid}"
os.system(command)
print(f"Command '{command}' executed.")
Example usage
pid = 1234 # Replace with the actual PID of the process you want to terminate
stop_process(pid)
详细描述:
通过os.system()执行系统命令是一个简单但不太灵活的方法。subprocess.run()提供了更强大的接口,可以捕获命令的输出和错误,并设置更多的选项。
五、跨平台考虑
在实际应用中,必须考虑到代码的跨平台兼容性。不同操作系统对进程管理有不同的机制,因此需要根据操作系统选择合适的方法。
Windows
在Windows上,可以使用os模块的TerminateProcess函数或psutil模块来停止进程。
import os
import psutil
def stop_process(pid):
try:
if os.name == 'nt':
process = psutil.Process(pid)
process.terminate()
print(f"Process {pid} has been terminated.")
else:
print("Unsupported OS.")
except psutil.NoSuchProcess:
print(f"No process with PID {pid} was found.")
except psutil.AccessDenied:
print(f"Permission denied to terminate process {pid}.")
except Exception as e:
print(f"Error occurred: {e}")
Example usage
pid = 1234 # Replace with the actual PID of the process you want to terminate
stop_process(pid)
Unix/Linux
在Unix/Linux系统上,可以使用os模块的kill函数或psutil模块来停止进程。
import os
import signal
import psutil
def stop_process(pid):
try:
if os.name != 'nt':
os.kill(pid, signal.SIGTERM)
print(f"Process {pid} has been terminated.")
else:
print("Unsupported OS.")
except ProcessLookupError:
print(f"No process with PID {pid} was found.")
except PermissionError:
print(f"Permission denied to terminate process {pid}.")
except Exception as e:
print(f"Error occurred: {e}")
Example usage
pid = 1234 # Replace with the actual PID of the process you want to terminate
stop_process(pid)
六、总结
使用os模块、subprocess模块和psutil模块是Python中停止其他程序的常用方法。每种方法都有其优缺点,选择合适的方法取决于具体的应用场景和需求。os模块提供了底层接口,适合简单任务;subprocess模块提供了更高层次的接口,适合启动和管理子进程;psutil模块提供了丰富的功能,适合需要获取进程和系统信息的复杂任务。
在实际应用中,还需要考虑跨平台兼容性,根据操作系统选择合适的方法。此外,处理异常情况(如进程不存在或权限不足)也是确保程序稳定性和安全性的重要方面。通过综合运用这些方法,可以实现对其他程序的灵活控制。
相关问答FAQs:
如何使用Python终止特定的进程?
可以使用Python的os
和signal
模块来停止特定进程。首先,需找到要终止的进程ID(PID),然后使用os.kill(pid, signal.SIGTERM)
来发送终止信号。此外,使用psutil
库也可以方便地处理进程的管理,包括查找和终止进程。
Python可以终止哪些类型的程序?
Python能够终止几乎所有在操作系统上运行的程序,只要你有足够的权限。无论是命令行工具、图形界面应用,还是后台服务,Python均可通过进程管理库进行处理。不过,某些系统进程可能需要管理员权限才能终止。
在Python中如何安全地停止一个程序?
为了安全地停止程序,建议使用try...except
语句来捕获可能发生的异常。这样可以确保在终止进程时不会影响系统的稳定性或其他正在运行的程序。此外,可以考虑使用psutil
库中的terminate()
方法,它会优雅地终止进程,允许其进行清理工作。
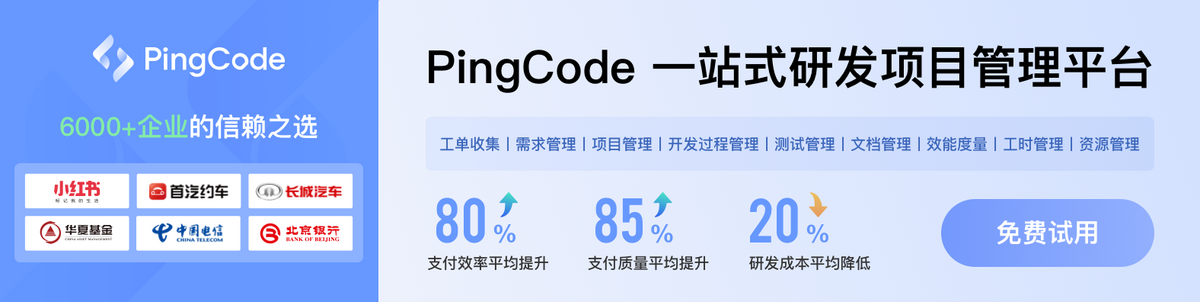