Python调用shell命令有多种方法:os.system()、subprocess.run()、os.popen()、subprocess.Popen()。其中最常用且推荐的是subprocess模块,因为它更强大且灵活。subprocess模块提供了多种方法来启动新进程、连接它们的输入/输出/错误管道,并获取它们的返回码。下面我将详细介绍subprocess模块的使用。
一、os.system()
os.system()是最简单的方法之一,但它的功能相对有限。它用于运行shell命令并将命令的输出打印到标准输出。
import os
os.system('ls -l')
尽管os.system()方法很简单,但它没有提供捕获命令输出的功能,这使得它在很多情况下不太适用。
二、subprocess.run()
subprocess.run()是subprocess模块中最常用的方法之一,适用于Python 3.5及以上版本。它执行命令并等待命令完成,返回一个包含执行结果的CompletedProcess实例。
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
- capture_output=True:捕获标准输出和标准错误。
- text=True:将输出解码为字符串。
这种方法不仅能捕获命令的输出,还能处理标准错误输出,非常适合需要捕获和处理shell命令输出的场景。
三、os.popen()
os.popen()方法用于执行shell命令并获取其输出。尽管功能较为强大,但在Python 3.5及以上版本中已经不再推荐使用。
import os
output = os.popen('ls -l').read()
print(output)
四、subprocess.Popen()
subprocess.Popen()提供了更多的控制,适用于需要复杂进程控制和管道的场景。它可以实现异步进程执行、实时获取命令输出等功能。
import subprocess
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print(stdout)
- subprocess.PIPE:用于将标准输出和标准错误输出管道化。
- communicate():与进程交互并等待命令完成,返回输出和错误。
五、捕获命令输出和错误
使用subprocess模块可以方便地捕获命令输出和错误。例如,使用subprocess.run()方法:
import subprocess
result = subprocess.run(['ls', '-l', '/nonexistent'], capture_output=True, text=True)
if result.returncode == 0:
print("Command succeeded:", result.stdout)
else:
print("Command failed:", result.stderr)
六、传递输入到命令
有时需要向命令传递输入,这可以使用subprocess.Popen()方法来实现:
import subprocess
process = subprocess.Popen(['grep', 'pattern'], stdin=subprocess.PIPE, stdout=subprocess.PIPE, text=True)
stdout, stderr = process.communicate(input='input text')
print(stdout)
七、使用Shell模式
尽管不推荐,但有时需要在shell中运行命令,可以使用subprocess.run()或subprocess.Popen()的shell=True参数:
import subprocess
result = subprocess.run('ls -l', shell=True, capture_output=True, text=True)
print(result.stdout)
需要注意的是,使用shell=True时要小心命令注入攻击,特别是在处理用户输入时。
八、设置超时
在某些情况下,命令可能会长时间运行,这时可以设置超时:
import subprocess
try:
result = subprocess.run(['sleep', '10'], timeout=5)
except subprocess.TimeoutExpired:
print("Command timed out")
九、使用环境变量
可以通过传递env参数来设置子进程的环境变量:
import subprocess
import os
env = os.environ.copy()
env['MY_VAR'] = 'value'
result = subprocess.run(['printenv', 'MY_VAR'], env=env, capture_output=True, text=True)
print(result.stdout)
十、处理复杂命令
对于需要使用管道或重定向的复杂命令,可以使用shell=True并将整个命令作为字符串传递:
import subprocess
result = subprocess.run('ls -l | grep py', shell=True, capture_output=True, text=True)
print(result.stdout)
十一、异步执行命令
可以使用subprocess.Popen()来异步执行命令,并在后台运行:
import subprocess
process = subprocess.Popen(['sleep', '5'])
print("Command is running in background")
process.wait()
print("Command completed")
十二、跨平台兼容性
在编写脚本时需要考虑到不同操作系统的兼容性。例如,Windows和Unix系统的命令语法有所不同:
import subprocess
import platform
if platform.system() == 'Windows':
command = 'dir'
else:
command = 'ls -l'
result = subprocess.run(command, shell=True, capture_output=True, text=True)
print(result.stdout)
十三、总结
subprocess模块是Python中调用shell命令的推荐方式,它提供了丰富的功能来控制和管理子进程。使用subprocess.run()方法可以方便地执行命令并获取输出,而subprocess.Popen()方法则适用于需要更复杂进程控制的场景。无论是捕获输出、处理错误、传递输入、设置超时还是使用环境变量,subprocess模块都提供了灵活的解决方案。
通过合理选择和使用subprocess模块的方法,可以在Python脚本中高效、可靠地调用和管理shell命令,从而实现更复杂和强大的功能。
相关问答FAQs:
在Python中如何执行shell命令?
Python可以通过多种方式执行shell命令,最常用的方法包括使用subprocess
模块。您可以使用subprocess.run()
来运行命令并获取输出。示例如下:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
这种方式不仅能够执行命令,还可以捕获标准输出和错误信息。
使用Python调用shell命令时,有哪些注意事项?
在使用Python调用shell命令时,确保您传递的参数是安全的,尤其是当这些参数来自用户输入时。使用subprocess
模块可以有效避免命令注入风险。推荐使用列表形式传参而非字符串,以减少错误和安全隐患。
如何处理Python中shell命令的错误?
可以通过subprocess.run()
中的check=True
参数来处理错误。如果命令执行失败,将抛出subprocess.CalledProcessError
异常。您还可以访问stderr
属性来获取错误信息。例如:
try:
subprocess.run(['ls', 'non_existent_file'], check=True)
except subprocess.CalledProcessError as e:
print(f"命令执行失败: {e.stderr}")
这种方式使得错误处理更加明确和有效。
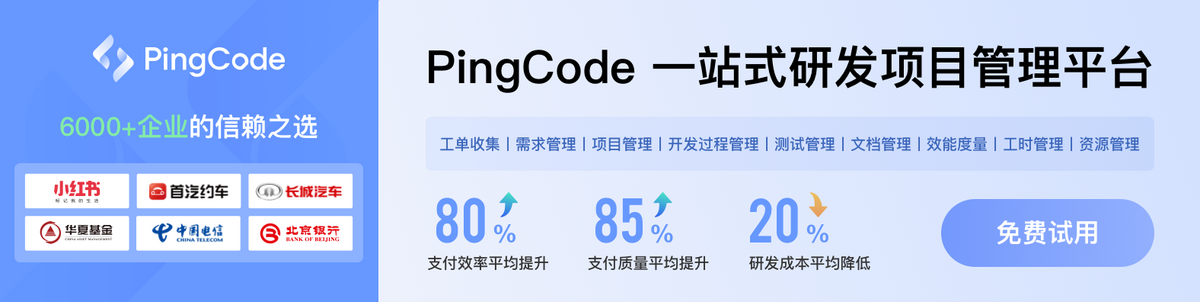