在Python中,使用pi
的主要方法是导入math
模块或numpy
模块。通过导入math
模块、通过导入numpy
模块、自己定义π的值。下面将详细描述如何通过导入math
模块来使用pi
。
一、通过导入math
模块
math
模块是Python自带的标准库之一,它包含了许多数学函数和常量。要使用pi
,只需导入math
模块,然后访问math.pi
即可。以下是具体步骤:
import math
pi_value = math.pi
print("The value of pi is:", pi_value)
在这个例子中,我们首先导入了math
模块,然后通过math.pi
获取了π的值。math.pi
提供的是一个浮点数,精确到小数点后15位。
二、通过导入numpy
模块
numpy
是一个功能强大的科学计算库,广泛应用于数值运算、线性代数等领域。numpy
也提供了对π的支持,使用numpy.pi
可以获得π的值。
import numpy as np
pi_value = np.pi
print("The value of pi is:", pi_value)
三、自己定义π的值
如果不想使用第三方库,还可以自己定义π的值。虽然这种方法不推荐,但在某些特定情况下可能会用到。
pi_value = 3.141592653589793
print("The value of pi is:", pi_value)
这种方法的灵活性在于你可以根据需要定义π的精度。
四、应用实例
1、计算圆的面积
计算圆的面积是π的一个经典应用。公式为Area = π * r^2
,其中r
是圆的半径。
import math
def calculate_circle_area(radius):
return math.pi * (radius 2)
radius = 5
area = calculate_circle_area(radius)
print(f"The area of a circle with radius {radius} is {area}")
2、计算圆的周长
计算圆的周长的公式为Circumference = 2 * π * r
。
import math
def calculate_circle_circumference(radius):
return 2 * math.pi * radius
radius = 5
circumference = calculate_circle_circumference(radius)
print(f"The circumference of a circle with radius {radius} is {circumference}")
3、计算球的体积
球体积的公式为Volume = (4/3) * π * r^3
。
import math
def calculate_sphere_volume(radius):
return (4/3) * math.pi * (radius 3)
radius = 5
volume = calculate_sphere_volume(radius)
print(f"The volume of a sphere with radius {radius} is {volume}")
4、计算圆锥的体积
圆锥体积的公式为Volume = (1/3) * π * r^2 * h
,其中h
是圆锥的高度。
import math
def calculate_cone_volume(radius, height):
return (1/3) * math.pi * (radius 2) * height
radius = 5
height = 10
volume = calculate_cone_volume(radius, height)
print(f"The volume of a cone with radius {radius} and height {height} is {volume}")
五、科学计算中的π
在科学计算中,π常常用于各种复杂的数学模型和公式中。例如,傅里叶变换、概率论和统计学中的正态分布等。
1、傅里叶变换
傅里叶变换是信号处理中的基本工具,它将时域信号转换为频域信号。公式中包含2π
的因子。
import numpy as np
def fourier_transform(signal):
n = len(signal)
frequency_domain = np.fft.fft(signal)
return frequency_domain
time_domain_signal = np.array([0, 1, 2, 3])
frequency_domain_signal = fourier_transform(time_domain_signal)
print("Frequency domain signal:", frequency_domain_signal)
2、正态分布
正态分布的概率密度函数包含1/(sqrt(2π)*σ)
,其中σ
是标准差。
import math
def normal_distribution(x, mean, std_dev):
return (1/(std_dev * math.sqrt(2 * math.pi))) * math.exp(-0.5 * ((x - mean)/std_dev) 2)
mean = 0
std_dev = 1
x = 1
probability_density = normal_distribution(x, mean, std_dev)
print(f"The probability density at x = {x} for a normal distribution with mean {mean} and standard deviation {std_dev} is {probability_density}")
六、π的精度问题
在计算机中,π的精度是有限的。Python中的math.pi
和numpy.pi
提供的精度通常已经足够满足大多数科学计算需求。然而,对于极端精度要求的应用,可以考虑使用符号计算库如SymPy
。
import sympy as sp
pi_value = sp.pi
print("The value of pi with SymPy is:", pi_value)
七、其他数学常量
除了π,math
和numpy
模块还提供了其他常用的数学常量,如e
(自然对数的底数),这些常量在科学计算中也非常重要。
import math
e_value = math.e
print("The value of e is:", e_value)
八、小结
通过导入math
或numpy
模块,使用pi
变得非常简单,适用于各种数学和科学计算。了解并掌握如何在Python中使用π,不仅能帮助你解决许多实际问题,还能加深对数学和编程的理解。
总结:
- 通过导入
math
模块 - 通过导入
numpy
模块 - 自己定义π的值
- 计算圆的面积、周长、球的体积、圆锥的体积
- 傅里叶变换、正态分布等科学计算应用
- π的精度问题
- 其他数学常量
掌握这些知识,能够在Python编程中更加高效地使用和操作π,解决各种数学和科学计算问题。
相关问答FAQs:
在Python中,如何获取圆周率π的值?
在Python中,可以通过math
模块轻松获取圆周率π的值。只需导入该模块,然后使用math.pi
即可。例如:
import math
print(math.pi)
这将输出3.141592653589793,这是π的近似值。
使用Python计算与π相关的数学公式有哪些实用示例?
通过Python,可以计算许多与π相关的数学公式。例如,计算圆的面积可以使用公式A = π * r²
。可以这样编写代码:
import math
def circle_area(radius):
return math.pi * radius ** 2
print(circle_area(5))
这个函数将返回半径为5的圆的面积,结果为78.53981633974483。
在Python中如何使用π进行科学计算?
在科学计算中,π常常与其他数学函数结合使用。使用numpy
库,你可以处理数组和矩阵,进行高效的数学计算。以下是一个示例,计算正弦函数:
import numpy as np
angles = np.array([0, np.pi/2, np.pi])
sine_values = np.sin(angles)
print(sine_values)
此代码将输出数组[0. 1. 0.],表示对应角度的正弦值。使用numpy
,可以轻松处理与π相关的复杂计算。
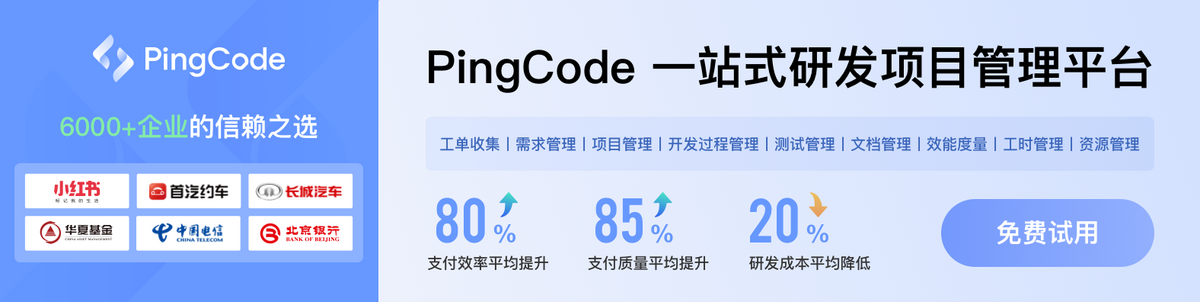