用Python定义字符的方法有以下几种:使用单引号、使用双引号、使用三引号、使用chr()函数。 以下是详细描述:
一、使用单引号定义字符
在Python中,最简单的定义字符的方法是使用单引号。单引号可以用于定义单个字符或字符串。比如:
char = 'a'
在上面的例子中,我们定义了一个变量char
,它的值是字符'a'。单引号也可以用于定义包含多个字符的字符串,例如:
string = 'hello'
单引号适用于简单的字符和字符串定义,特别是在字符串中不包含单引号的情况下。
二、使用双引号定义字符
双引号与单引号的作用相同,也可以用于定义字符和字符串。比如:
char = "a"
在上面的例子中,我们定义了一个变量char
,它的值是字符'a'。双引号也可以用于定义包含多个字符的字符串,例如:
string = "hello"
使用双引号的好处是,当字符串内部包含单引号时,不需要进行转义。例如:
quote = "He said, 'Hello!'"
三、使用三引号定义字符
三引号(单引号或双引号的三重形式)通常用于定义多行字符串,但也可以用于定义单个字符或字符串。比如:
char = '''a'''
在上面的例子中,我们定义了一个变量char
,它的值是字符'a'。三引号也可以用于定义包含多个字符的字符串,例如:
string = '''hello'''
三引号的主要优势在于它可以直接包含换行符和其他特殊字符,而无需进行转义。例如:
multiline_string = """This is a
multi-line string."""
四、使用chr()函数定义字符
在Python中,chr()
函数可以用于根据Unicode码点生成相应的字符。比如:
char = chr(97)
在上面的例子中,我们定义了一个变量char
,它的值是字符'a'(97是'a'的Unicode码点)。chr()
函数特别适用于需要根据Unicode码点动态生成字符的场景。例如:
char_a = chr(97) # 'a'
char_b = chr(98) # 'b'
五、字符与字符串的区别
在Python中,字符实际上是长度为1的字符串。虽然在使用上没有太多区别,但理解这一点有助于更好地掌握Python的字符和字符串处理。例如:
char = 'a'
print(type(char)) # <class 'str'>
上面的例子表明,即使定义的是单个字符,其类型仍然是字符串(str)。
六、字符的操作与处理
Python提供了丰富的字符串操作方法,可以用于字符的处理。例如,可以使用ord()
函数获取字符的Unicode码点:
char = 'a'
code_point = ord(char) # 97
可以使用len()
函数获取字符串的长度:
string = 'hello'
length = len(string) # 5
可以使用字符串切片操作获取字符串中的单个字符:
string = 'hello'
char = string[0] # 'h'
七、字符的编码与解码
字符在内存中是以编码形式存储的,Python提供了编码与解码的方法。例如,可以使用encode()
方法将字符串编码为字节:
string = 'hello'
encoded_string = string.encode('utf-8')
可以使用decode()
方法将字节解码为字符串:
encoded_string = b'hello'
decoded_string = encoded_string.decode('utf-8')
八、字符的比较
Python支持字符的比较操作,例如,可以使用比较运算符比较两个字符的大小:
char1 = 'a'
char2 = 'b'
print(char1 < char2) # True
字符比较基于其Unicode码点的大小。
九、字符的拼接
可以使用+
运算符拼接字符和字符串:
char1 = 'a'
char2 = 'b'
combined_string = char1 + char2 # 'ab'
也可以使用join()
方法拼接多个字符和字符串:
chars = ['a', 'b', 'c']
combined_string = ''.join(chars) # 'abc'
十、字符的查找与替换
可以使用字符串的方法查找和替换字符。例如,可以使用find()
方法查找字符的位置:
string = 'hello'
position = string.find('e') # 1
可以使用replace()
方法替换字符:
string = 'hello'
new_string = string.replace('e', 'a') # 'hallo'
十一、字符的格式化
可以使用字符串的格式化方法格式化字符。例如,可以使用format()
方法格式化字符串:
char = 'a'
formatted_string = 'Character: {}'.format(char)
也可以使用f字符串进行格式化:
char = 'a'
formatted_string = f'Character: {char}'
十二、字符的判断
Python提供了多种字符串方法用于判断字符的属性。例如,可以使用isalpha()
方法判断字符是否为字母:
char = 'a'
is_alpha = char.isalpha() # True
可以使用isdigit()
方法判断字符是否为数字:
char = '1'
is_digit = char.isdigit() # True
可以使用isspace()
方法判断字符是否为空白字符:
char = ' '
is_space = char.isspace() # True
十三、字符的转换
Python提供了多种字符串方法用于转换字符的大小写。例如,可以使用upper()
方法将字符转换为大写:
char = 'a'
upper_char = char.upper() # 'A'
可以使用lower()
方法将字符转换为小写:
char = 'A'
lower_char = char.lower() # 'a'
可以使用capitalize()
方法将字符串的首字符转换为大写:
string = 'hello'
capitalized_string = string.capitalize() # 'Hello'
十四、字符的编码与解码
字符在内存中是以编码形式存储的,Python提供了编码与解码的方法。例如,可以使用encode()
方法将字符串编码为字节:
string = 'hello'
encoded_string = string.encode('utf-8')
可以使用decode()
方法将字节解码为字符串:
encoded_string = b'hello'
decoded_string = encoded_string.decode('utf-8')
十五、字符的输入与输出
可以使用input()
函数从用户输入中获取字符:
char = input('Enter a character: ')
可以使用print()
函数输出字符:
char = 'a'
print('Character:', char)
十六、字符的转义序列
在定义包含特殊字符的字符串时,可以使用转义序列。例如,可以使用\n
表示换行符:
string = 'Hello\nWorld'
可以使用\
表示反斜杠:
string = 'C:\\path\\to\\file'
可以使用\'
表示单引号:
string = 'It\'s a test'
可以使用\"
表示双引号:
string = "He said, \"Hello!\""
十七、字符串的分割与合并
可以使用split()
方法将字符串分割为多个子字符串:
string = 'hello world'
words = string.split() # ['hello', 'world']
可以使用join()
方法将多个子字符串合并为一个字符串:
words = ['hello', 'world']
string = ' '.join(words) # 'hello world'
十八、字符串的去除
可以使用strip()
方法去除字符串两端的空白字符:
string = ' hello '
trimmed_string = string.strip() # 'hello'
可以使用lstrip()
方法去除字符串左端的空白字符:
string = ' hello '
left_trimmed_string = string.lstrip() # 'hello '
可以使用rstrip()
方法去除字符串右端的空白字符:
string = ' hello '
right_trimmed_string = string.rstrip() # ' hello'
十九、字符串的查找与替换
可以使用find()
方法查找子字符串的位置:
string = 'hello'
position = string.find('e') # 1
可以使用replace()
方法替换子字符串:
string = 'hello'
new_string = string.replace('e', 'a') # 'hallo'
二十、字符串的格式化
可以使用format()
方法格式化字符串:
name = 'Alice'
formatted_string = 'Hello, {}'.format(name)
也可以使用f字符串进行格式化:
name = 'Alice'
formatted_string = f'Hello, {name}'
二十一、字符串的比较
可以使用比较运算符比较两个字符串的大小:
string1 = 'apple'
string2 = 'banana'
print(string1 < string2) # True
字符串比较基于其字符的Unicode码点的大小。
二十二、字符串的拼接
可以使用+
运算符拼接字符串:
string1 = 'hello'
string2 = 'world'
combined_string = string1 + ' ' + string2 # 'hello world'
也可以使用join()
方法拼接多个字符串:
words = ['hello', 'world']
combined_string = ' '.join(words) # 'hello world'
二十三、字符串的判断
可以使用字符串的方法判断字符串的属性。例如,可以使用isalpha()
方法判断字符串是否为字母:
string = 'hello'
is_alpha = string.isalpha() # True
可以使用isdigit()
方法判断字符串是否为数字:
string = '123'
is_digit = string.isdigit() # True
可以使用isspace()
方法判断字符串是否为空白字符:
string = ' '
is_space = string.isspace() # True
二十四、字符串的转换
可以使用upper()
方法将字符串转换为大写:
string = 'hello'
upper_string = string.upper() # 'HELLO'
可以使用lower()
方法将字符串转换为小写:
string = 'HELLO'
lower_string = string.lower() # 'hello'
可以使用capitalize()
方法将字符串的首字符转换为大写:
string = 'hello'
capitalized_string = string.capitalize() # 'Hello'
二十五、字符串的编码与解码
可以使用encode()
方法将字符串编码为字节:
string = 'hello'
encoded_string = string.encode('utf-8')
可以使用decode()
方法将字节解码为字符串:
encoded_string = b'hello'
decoded_string = encoded_string.decode('utf-8')
二十六、字符串的输入与输出
可以使用input()
函数从用户输入中获取字符串:
string = input('Enter a string: ')
可以使用print()
函数输出字符串:
string = 'hello'
print('String:', string)
二十七、字符串的转义序列
在定义包含特殊字符的字符串时,可以使用转义序列。例如,可以使用\n
表示换行符:
string = 'Hello\nWorld'
可以使用\
表示反斜杠:
string = 'C:\\path\\to\\file'
可以使用\'
表示单引号:
string = 'It\'s a test'
可以使用\"
表示双引号:
string = "He said, \"Hello!\""
二十八、字符串的分割与合并
可以使用split()
方法将字符串分割为多个子字符串:
string = 'hello world'
words = string.split() # ['hello', 'world']
可以使用join()
方法将多个子字符串合并为一个字符串:
words = ['hello', 'world']
string = ' '.join(words) # 'hello world'
二十九、字符串的去除
可以使用strip()
方法去除字符串两端的空白字符:
string = ' hello '
trimmed_string = string.strip() # 'hello'
可以使用lstrip()
方法去除字符串左端的空白字符:
string = ' hello '
left_trimmed_string = string.lstrip() # 'hello '
可以使用rstrip()
方法去除字符串右端的空白字符:
string = ' hello '
right_trimmed_string = string.rstrip() # ' hello'
三十、字符串的查找与替换
可以使用find()
方法查找子字符串的位置:
string = 'hello'
position = string.find('e') # 1
可以使用replace()
方法替换子字符串:
string = 'hello'
new_string = string.replace('e', 'a') # 'hallo'
三十一、字符串的格式化
可以使用format()
方法格式化字符串:
name = 'Alice'
formatted_string = 'Hello, {}'.format(name)
也可以使用f字符串进行格式化:
name = 'Alice'
formatted_string = f'Hello, {name}'
三十二、字符串的比较
可以使用比较运算符比较两个字符串的大小:
string1 = 'apple'
string2 = 'banana'
print(string1 < string2) # True
字符串比较基于其字符的Unicode码点的大小。
三十三、字符串的拼接
可以使用+
运算符拼接字符串:
string1 = 'hello'
string2 = 'world'
combined_string = string1 + ' ' + string2 # 'hello world'
也可以使用join()
方法拼接多个字符串:
words = ['hello', 'world']
combined_string = ' '.join(words) # 'hello world'
三十四、字符串的判断
可以使用字符串的方法判断字符串的属性。例如,可以使用isalpha()
方法判断字符串是否为字母:
string = 'hello'
is_alpha = string.isalpha() # True
可以使用isdigit()
方法判断字符串是否为数字:
string = '123'
is_digit = string.isdigit() # True
可以使用isspace()
方法判断字符串是否为空白字符:
string = ' '
is_space = string.isspace() # True
三十五、字符串的转换
可以使用upper()
方法将字符串转换为大写:
string = 'hello'
upper_string = string.upper() # 'HELLO'
可以使用lower()
方法将字符串转换为小写:
string = 'HELLO'
lower_string = string.lower() # 'hello'
可以使用capitalize()
方法将字符串的首字符转换为大写:
string = 'hello'
capitalized_string = string.capitalize() # 'Hello'
三十六、字符串的编码与解码
可以使用encode()
方法将字符串编码为字节:
string = 'hello'
encoded_string = string.encode('utf-8')
可以使用decode()
方法将字节解码为字符串:
encoded_string = b'hello'
decoded_string = encoded_string.decode('utf-8')
三十七、字符串的输入与输出
可以使用input()
函数从用户输入中获取字符串:
string = input('Enter a string: ')
可以使用print()
函数输出字符串:
string = 'hello'
print('String:', string)
三十八、字符串的转义序列
在定义包含特殊字符的字符串时,可以使用转义序列。例如,可以使用\n
表示换行符:
string = 'Hello\nWorld'
可以使用\
表示反斜杠:
string = 'C:\\path\\to\\file'
可以使用\'
表示单引号:
string = 'It\'s a test'
``
相关问答FAQs:
如何在Python中创建和使用字符串?
在Python中,字符串可以通过单引号(')、双引号(")或三重引号('''或""")来定义。三重引号特别适合于多行字符串。创建字符串后,可以使用各种字符串方法进行操作,比如upper()
、lower()
和replace()
等。
Python中字符串的常用操作有哪些?
Python提供了丰富的字符串操作方法,例如拼接字符串使用+
运算符,重复字符串使用*
运算符。可以通过索引访问字符,也可以使用切片提取子字符串。此外,len()
函数可用于获取字符串的长度。
如何在Python中处理字符串中的特殊字符?
在Python字符串中,特殊字符可以通过转义字符(如\n
表示换行,\t
表示制表符)进行处理。使用原始字符串(在字符串前加上r
)可以避免转义,使得字符串中的反斜杠按字面意义处理。这对于处理文件路径或正则表达式特别有用。
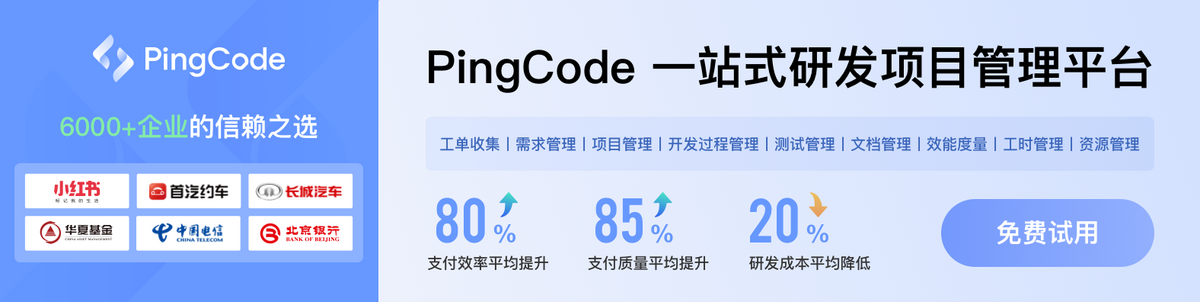