实现Python中的非阻塞操作可以通过多种方式来完成,主要包括多线程、多进程、异步编程、使用非阻塞I/O模块等。本文将详细介绍这些方法中的每一种,并提供实际示例,以帮助你更好地理解和应用它们。
一、多线程
多线程是实现非阻塞操作的一种常用方法。在Python中,可以使用threading
模块来创建和管理线程。通过将不同的任务分配给不同的线程来实现并发执行,从而达到非阻塞的效果。
1.1、创建线程
import threading
import time
def task():
print("Task started")
time.sleep(2)
print("Task finished")
创建线程
thread = threading.Thread(target=task)
启动线程
thread.start()
print("Main thread continues to run while the task runs in the background")
以上代码示例中,通过创建并启动一个新线程来运行task
函数,从而使主线程继续运行而不被阻塞。
1.2、线程同步
在多线程编程中,线程之间可能需要共享数据资源,这时需要考虑线程同步问题。可以使用threading.Lock
来实现线程同步,避免数据竞争。
import threading
shared_resource = 0
lock = threading.Lock()
def increment():
global shared_resource
with lock:
for _ in range(100000):
shared_resource += 1
threads = [threading.Thread(target=increment) for _ in range(10)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print("Final value of shared resource:", shared_resource)
二、多进程
多进程是另一种实现非阻塞操作的方法,尤其适用于CPU密集型任务。在Python中,可以使用multiprocessing
模块来创建和管理进程。
2.1、创建进程
import multiprocessing
import time
def task():
print("Task started")
time.sleep(2)
print("Task finished")
创建进程
process = multiprocessing.Process(target=task)
启动进程
process.start()
print("Main process continues to run while the task runs in the background")
2.2、进程同步
与多线程类似,多进程编程中也需要考虑进程之间的同步问题。可以使用multiprocessing.Lock
来实现进程同步。
import multiprocessing
shared_resource = 0
lock = multiprocessing.Lock()
def increment():
global shared_resource
with lock:
for _ in range(100000):
shared_resource += 1
processes = [multiprocessing.Process(target=increment) for _ in range(10)]
for process in processes:
process.start()
for process in processes:
process.join()
print("Final value of shared resource:", shared_resource)
三、异步编程
异步编程是一种实现非阻塞操作的现代方法,特别适合I/O密集型任务。在Python中,可以使用asyncio
模块来实现异步编程。
3.1、异步函数
import asyncio
async def task():
print("Task started")
await asyncio.sleep(2)
print("Task finished")
async def main():
await task()
print("Main function continues to run after the task finishes")
运行异步函数
asyncio.run(main())
3.2、并发任务
可以使用asyncio.gather
或asyncio.create_task
来并发执行多个异步任务,从而实现非阻塞操作。
import asyncio
async def task(id):
print(f"Task {id} started")
await asyncio.sleep(2)
print(f"Task {id} finished")
async def main():
tasks = [asyncio.create_task(task(i)) for i in range(5)]
await asyncio.gather(*tasks)
print("All tasks finished")
运行异步函数
asyncio.run(main())
四、非阻塞I/O模块
Python提供了一些非阻塞I/O模块,如selectors
和asyncore
,用于实现非阻塞网络通信和文件操作。
4.1、使用selectors模块
selectors
模块提供了一个高层次的I/O多路复用接口,支持非阻塞的文件和网络操作。
import selectors
import socket
sel = selectors.DefaultSelector()
def accept(sock, mask):
conn, addr = sock.accept()
print('Accepted connection from', addr)
conn.setblocking(False)
sel.register(conn, selectors.EVENT_READ, read)
def read(conn, mask):
data = conn.recv(1000)
if data:
print('Received data:', data)
else:
print('Closing connection')
sel.unregister(conn)
conn.close()
sock = socket.socket()
sock.bind(('localhost', 12345))
sock.listen()
sock.setblocking(False)
sel.register(sock, selectors.EVENT_READ, accept)
while True:
events = sel.select()
for key, mask in events:
callback = key.data
callback(key.fileobj, mask)
4.2、使用asyncore模块
asyncore
模块提供了一个异步套接字服务框架,适用于实现高效的网络服务器和客户端。
import asyncore
import socket
class EchoHandler(asyncore.dispatcher_with_send):
def handle_read(self):
data = self.recv(1024)
if data:
self.send(data)
class EchoServer(asyncore.dispatcher):
def __init__(self, host, port):
super().__init__()
self.create_socket(socket.AF_INET, socket.SOCK_STREAM)
self.bind((host, port))
self.listen(5)
def handle_accepted(self, sock, addr):
print('Accepted connection from', addr)
handler = EchoHandler(sock)
server = EchoServer('localhost', 12345)
asyncore.loop()
结论
通过多线程、多进程、异步编程和非阻塞I/O模块,可以在Python中实现非阻塞操作。不同的方法适用于不同类型的任务:多线程适合I/O密集型任务,多进程适合CPU密集型任务,异步编程适合需要高并发的I/O操作,非阻塞I/O模块则适合网络通信和文件操作。根据具体需求选择合适的方法,可以有效提高程序的并发性能和响应速度。
相关问答FAQs:
如何在Python中实现非阻塞IO?
在Python中,可以使用asyncio
库实现非阻塞IO。通过使用异步函数和await
关键字,可以在执行IO操作时不阻塞主线程。此外,使用asyncio.run()
可以启动事件循环,从而实现非阻塞的执行。
非阻塞编程的优点是什么?
非阻塞编程的主要优点包括提高程序的性能和响应速度。当一个任务在执行IO操作时,程序可以继续执行其他任务,而不必等待IO操作完成。这在处理大量并发连接时,尤其是网络应用程序中,可以显著提升效率。
在Python中,如何处理多线程与非阻塞IO?
在Python中,可以使用threading
模块创建多线程应用,同时结合非阻塞IO实现更高效的任务处理。通过在不同线程中执行IO操作,可以避免主线程被阻塞。然而,需要注意线程安全问题,确保共享资源的正确访问和修改。
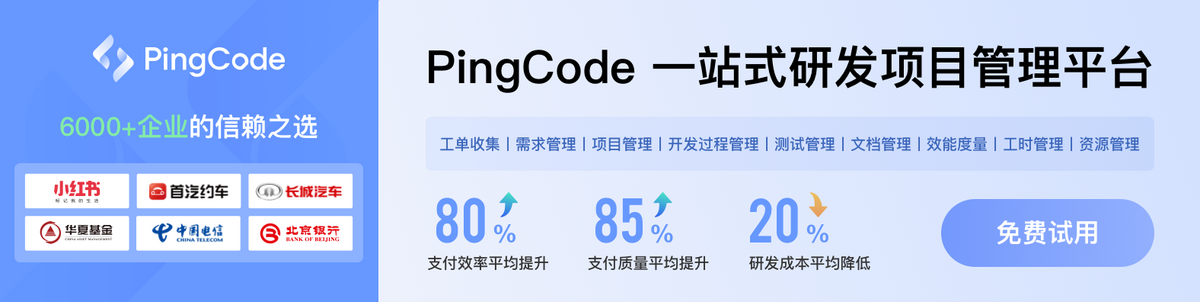