Python连接Elasticsearch认证的方法包括使用Elasticsearch客户端库、配置基本身份验证、配置API密钥认证以及使用OAuth 2.0认证等。其中,使用Elasticsearch客户端库是最常见的方法,下面将详细介绍如何实现这一方法。
使用官方Elasticsearch Python客户端库(Elasticsearch-py)连接Elasticsearch集群是最常见的方式。这个库提供了方便的方法来配置连接和认证信息。首先,我们需要安装Elasticsearch-py库:
pip install elasticsearch
安装完成后,可以通过以下示例代码来进行连接和认证:
from elasticsearch import Elasticsearch
使用基本身份验证连接Elasticsearch
es = Elasticsearch(
['https://localhost:9200'],
http_auth=('user', 'password'),
scheme="https",
port=9200,
)
验证连接是否成功
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
在上面的代码中,我们使用了http_auth
参数来传递用户名和密码进行基本身份验证,并指定了集群的URL和端口号。如果需要使用其他认证方式,可以参考以下内容。
一、基本认证
基本认证是最简单的认证方式,通过在HTTP请求头中传递用户名和密码来实现。在Elasticsearch-py中,可以通过http_auth
参数来配置基本认证。
from elasticsearch import Elasticsearch
使用基本身份验证连接Elasticsearch
es = Elasticsearch(
['https://localhost:9200'],
http_auth=('user', 'password'),
scheme="https",
port=9200,
)
验证连接是否成功
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
在上面的代码中,http_auth
参数接收一个元组,包含用户名和密码。这样,在每次发送请求时,客户端会自动将这些认证信息添加到请求头中。
二、API密钥认证
Elasticsearch支持使用API密钥进行认证,这种方式比基本认证更安全。我们需要首先生成一个API密钥,然后在客户端中使用这个密钥进行认证。
首先,在Elasticsearch中创建API密钥:
curl -X POST "https://localhost:9200/_security/api_key" -u user:password -H "Content-Type: application/json" -d'
{
"name": "my-api-key",
"role_descriptors": {
"my-api-key-role": {
"cluster": ["all"],
"index": [
{
"names": ["*"],
"privileges": ["all"]
}
]
}
}
}
'
这个命令将创建一个名为my-api-key
的API密钥,并授予它对所有索引的所有权限。返回的JSON响应中包含了API密钥的ID和密钥值。
然后,在Python客户端中使用API密钥进行认证:
from elasticsearch import Elasticsearch
使用API密钥认证连接Elasticsearch
es = Elasticsearch(
['https://localhost:9200'],
api_key=('api_key_id', 'api_key_value'),
scheme="https",
port=9200,
)
验证连接是否成功
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
在上面的代码中,api_key
参数接收一个元组,包含API密钥的ID和密钥值。
三、OAuth 2.0认证
OAuth 2.0是一种常见的认证授权方式,Elasticsearch也支持这种方式。我们需要首先获取一个访问令牌,然后在客户端中使用这个令牌进行认证。
假设我们已经有一个获取访问令牌的API端点:
import requests
获取访问令牌
response = requests.post('https://auth.example.com/oauth/token', data={
'grant_type': 'password',
'client_id': 'client_id',
'client_secret': 'client_secret',
'username': 'user',
'password': 'password'
})
token = response.json()['access_token']
然后,在Python客户端中使用访问令牌进行认证:
from elasticsearch import Elasticsearch
使用OAuth 2.0令牌认证连接Elasticsearch
es = Elasticsearch(
['https://localhost:9200'],
headers={'Authorization': f'Bearer {token}'},
scheme="https",
port=9200,
)
验证连接是否成功
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
在上面的代码中,我们使用headers
参数将访问令牌添加到HTTP请求头中,以进行OAuth 2.0认证。
四、SSL/TLS配置
在连接Elasticsearch时,启用SSL/TLS加密是非常重要的。这可以确保数据在传输过程中不会被窃听或篡改。在Elasticsearch-py中,可以通过配置SSL/TLS参数来启用加密。
首先,我们需要在Elasticsearch集群上配置SSL/TLS。具体步骤可以参考Elasticsearch官方文档。然后,在Python客户端中配置SSL/TLS参数:
from elasticsearch import Elasticsearch
配置SSL/TLS连接
es = Elasticsearch(
['https://localhost:9200'],
http_auth=('user', 'password'),
scheme="https",
port=9200,
ssl_context={
'verify_certs': True,
'ca_certs': '/path/to/ca.crt',
'client_cert': '/path/to/client.crt',
'client_key': '/path/to/client.key'
}
)
验证连接是否成功
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
在上面的代码中,ssl_context
参数接收一个包含SSL/TLS配置的字典。我们可以指定是否验证证书、CA证书路径、客户端证书和客户端密钥等。
五、使用高级配置
除了基本的连接和认证配置外,Elasticsearch-py还提供了一些高级配置选项,可以帮助我们更好地控制连接行为和性能。
1. 连接池配置
Elasticsearch-py使用连接池来管理HTTP连接。我们可以通过配置连接池参数来优化连接性能。
from elasticsearch import Elasticsearch
from elasticsearch.connection import RequestsHttpConnection
配置连接池
es = Elasticsearch(
['https://localhost:9200'],
http_auth=('user', 'password'),
scheme="https",
port=9200,
connection_class=RequestsHttpConnection,
maxsize=10, # 最大连接数
retry_on_timeout=True, # 超时重试
timeout=30 # 请求超时
)
验证连接是否成功
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
在上面的代码中,我们使用maxsize
参数配置最大连接数,retry_on_timeout
参数配置是否在超时后重试,timeout
参数配置请求超时时间。
2. 重试策略
Elasticsearch-py提供了内置的重试策略,可以帮助我们在请求失败时自动重试。
from elasticsearch import Elasticsearch
from elasticsearch.transport import Transport
配置重试策略
es = Elasticsearch(
['https://localhost:9200'],
http_auth=('user', 'password'),
scheme="https",
port=9200,
transport_class=Transport,
retry_on_status=[500, 502, 503, 504], # 重试的HTTP状态码
max_retries=3 # 最大重试次数
)
验证连接是否成功
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
在上面的代码中,我们使用retry_on_status
参数配置需要重试的HTTP状态码,max_retries
参数配置最大重试次数。
六、处理认证错误
在实际应用中,我们需要处理各种可能的认证错误。以下是一些常见的认证错误及其处理方法:
1. 认证失败
如果用户名或密码错误,Elasticsearch会返回401 Unauthorized错误。我们可以捕获这个错误并进行处理。
from elasticsearch import Elasticsearch
from elasticsearch.exceptions import AuthenticationException
try:
es = Elasticsearch(
['https://localhost:9200'],
http_auth=('user', 'wrong_password'),
scheme="https",
port=9200,
)
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
except AuthenticationException as e:
print("Authentication failed:", e)
2. API密钥无效
如果API密钥无效,Elasticsearch会返回403 Forbidden错误。我们可以捕获这个错误并进行处理。
from elasticsearch import Elasticsearch
from elasticsearch.exceptions import AuthorizationException
try:
es = Elasticsearch(
['https://localhost:9200'],
api_key=('invalid_api_key_id', 'invalid_api_key_value'),
scheme="https",
port=9200,
)
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
except AuthorizationException as e:
print("Authorization failed:", e)
3. 令牌过期
如果OAuth 2.0访问令牌过期,Elasticsearch会返回401 Unauthorized错误。我们可以捕获这个错误并刷新令牌。
from elasticsearch import Elasticsearch
from elasticsearch.exceptions import AuthenticationException
import requests
def get_access_token():
response = requests.post('https://auth.example.com/oauth/token', data={
'grant_type': 'password',
'client_id': 'client_id',
'client_secret': 'client_secret',
'username': 'user',
'password': 'password'
})
return response.json()['access_token']
try:
token = get_access_token()
es = Elasticsearch(
['https://localhost:9200'],
headers={'Authorization': f'Bearer {token}'},
scheme="https",
port=9200,
)
if es.ping():
print("Connected to Elasticsearch")
else:
print("Connection failed")
except AuthenticationException as e:
print("Authentication failed:", e)
# 刷新令牌
token = get_access_token()
es = Elasticsearch(
['https://localhost:9200'],
headers={'Authorization': f'Bearer {token}'},
scheme="https",
port=9200,
)
if es.ping():
print("Connected to Elasticsearch with new token")
else:
print("Connection failed")
在上面的代码中,我们定义了一个get_access_token
函数来获取新的访问令牌,并在捕获到认证错误后刷新令牌并重试连接。
七、总结
本文详细介绍了Python连接Elasticsearch认证的几种方法,包括基本认证、API密钥认证、OAuth 2.0认证以及SSL/TLS配置。我们还介绍了一些高级配置选项,如连接池配置和重试策略,并讨论了如何处理常见的认证错误。
通过这些方法和配置,我们可以确保在连接Elasticsearch集群时具有更高的安全性和可靠性。希望本文能对您在使用Python连接Elasticsearch时有所帮助。
相关问答FAQs:
如何在Python中设置Elasticsearch的连接认证?
在Python中连接Elasticsearch时,您需要使用Elasticsearch库的Elasticsearch
类,并在连接时提供认证信息。这通常包括用户名和密码。示例代码如下:
from elasticsearch import Elasticsearch
es = Elasticsearch(
['http://localhost:9200'],
http_auth=('username', 'password')
)
确保将username
和password
替换为您的实际认证信息。此代码片段会创建一个与Elasticsearch服务器的连接,并使用提供的凭证进行身份验证。
在使用Python连接Elasticsearch时,如何处理SSL证书?
如果您的Elasticsearch服务器使用了SSL证书,您需要在连接时指定use_ssl=True
和verify_certs=True
。这会确保连接的安全性。如果您使用的是自签名证书,可能还需要提供证书文件的路径。示例代码如下:
es = Elasticsearch(
['https://localhost:9200'],
http_auth=('username', 'password'),
use_ssl=True,
verify_certs=False # 对于自签名证书,可以设置为False
)
在生产环境中,建议将verify_certs
设置为True,并提供有效的证书以确保安全连接。
如果连接Elasticsearch时遇到认证失败,应该如何排查问题?
认证失败通常是由于用户名或密码错误,或者用户没有足够的权限。首先,确保您输入的凭证是正确的。其次,检查用户是否具备访问Elasticsearch的权限。您可以通过Elasticsearch的角色管理功能来查看用户权限。此外,查看Elasticsearch的日志文件也可以提供有用的信息,帮助您找到问题的根源。
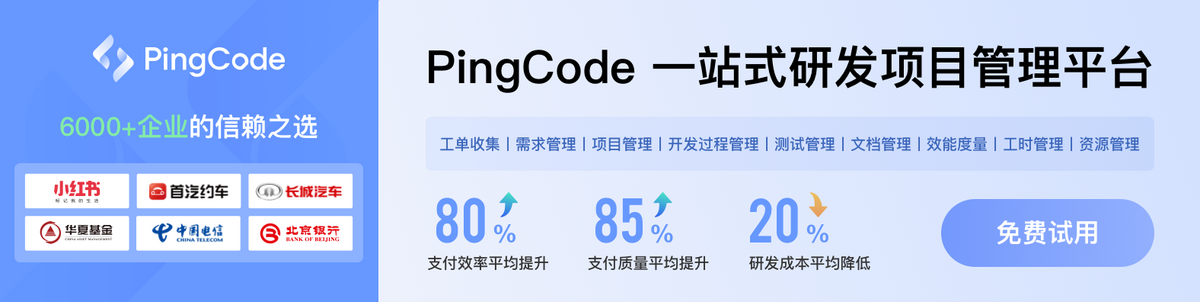