Python求链表长度的方法包括遍历链表、利用递归、使用内置函数等。 其中,遍历链表是一种最常用且简单的方法,它通过遍历每个节点并计数来确定链表的长度。我们将详细描述这种方法。
遍历链表的方法如下:首先,需要创建一个指针来指向链表的头节点,同时设置一个计数器为0。然后,通过循环遍历链表中的每个节点,将指针移动到下一个节点,并在每次遍历时将计数器加1。最后,当指针指向空节点时,循环结束,计数器的值即为链表的长度。
以下是具体实现代码:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def get_length(head: ListNode) -> int:
length = 0
current_node = head
while current_node:
length += 1
current_node = current_node.next
return length
一、遍历链表求长度
遍历链表是一种简单且直接的方法来计算链表的长度。它通过逐个访问链表中的每个节点并计数来确定链表的长度。
1、创建链表节点类
首先,我们需要定义一个链表节点类。每个节点包含一个值和指向下一个节点的指针。
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
2、定义求长度函数
接下来,我们定义一个函数来遍历链表并计算其长度。
def get_length(head: ListNode) -> int:
length = 0
current_node = head
while current_node:
length += 1
current_node = current_node.next
return length
在这个函数中,head
是链表的头节点。我们初始化一个计数器length
为0,并使用current_node
指针来遍历链表。每当访问到一个节点时,计数器加1,然后将指针移动到下一个节点。当指针指向空节点时,循环结束,返回计数器的值即为链表的长度。
二、递归求链表长度
递归是一种优雅的编程技巧,通过函数自身的调用来解决问题。我们也可以使用递归来求链表的长度。
1、递归函数定义
递归函数通常包含两个部分:基准情况和递归情况。对于链表长度的递归求解,基准情况是链表为空时长度为0,递归情况是链表长度等于头节点加上剩余部分的长度。
def get_length_recursive(head: ListNode) -> int:
if not head:
return 0
return 1 + get_length_recursive(head.next)
在这个函数中,如果链表为空(即head
为None
),返回长度0。否则,返回1加上剩余链表的长度。
三、使用内置函数求链表长度
在一些情况下,我们可以将链表转换为其他数据结构(例如列表),然后使用Python的内置函数来求长度。这种方法虽然不如前两种方法高效,但在某些情况下可能会更简洁。
1、将链表转换为列表
首先,我们需要定义一个函数来将链表转换为列表。
def linked_list_to_list(head: ListNode) -> list:
result = []
current_node = head
while current_node:
result.append(current_node.val)
current_node = current_node.next
return result
2、使用内置函数求长度
然后,我们可以使用Python的内置len
函数来求长度。
def get_length_with_builtin(head: ListNode) -> int:
return len(linked_list_to_list(head))
四、总结与对比
以上介绍了三种求链表长度的方法:遍历链表、递归和使用内置函数。我们可以根据具体情况选择最合适的方法。
- 遍历链表:这种方法简单直接,时间复杂度为O(n),适用于大多数情况。
- 递归:这种方法优雅但可能会导致栈溢出,尤其是在链表较长时。
- 使用内置函数:这种方法需要先将链表转换为列表,时间复杂度较高,但代码简洁。
五、链表基础知识
在深入讨论如何求链表长度之前,我们先来回顾一下链表的基础知识。
1、链表的定义
链表是一种线性数据结构,其中的元素通过指针连接。每个节点包含一个值和指向下一个节点的指针。链表的第一个节点称为头节点,最后一个节点的指针指向空。
2、链表的类型
链表有多种类型,包括单链表、双向链表和循环链表。单链表的每个节点只有一个指针指向下一个节点;双向链表的每个节点有两个指针,分别指向前一个和下一个节点;循环链表的最后一个节点指针指向头节点,形成一个环。
3、链表的优缺点
链表的优点是动态大小,插入和删除操作快速。缺点是随机访问效率低,额外的存储空间用于指针。
六、链表的基本操作
在讨论链表长度的求解之前,我们先了解一些链表的基本操作,如插入、删除和查找。
1、插入操作
插入操作包括在链表头部、尾部和中间插入节点。
def insert_at_head(head: ListNode, val: int) -> ListNode:
new_node = ListNode(val)
new_node.next = head
return new_node
def insert_at_tail(head: ListNode, val: int) -> ListNode:
new_node = ListNode(val)
if not head:
return new_node
current_node = head
while current_node.next:
current_node = current_node.next
current_node.next = new_node
return head
def insert_at_position(head: ListNode, val: int, position: int) -> ListNode:
new_node = ListNode(val)
if position == 0:
new_node.next = head
return new_node
current_node = head
for _ in range(position - 1):
if not current_node:
raise IndexError("Position out of bounds")
current_node = current_node.next
new_node.next = current_node.next
current_node.next = new_node
return head
2、删除操作
删除操作包括删除头节点、尾节点和中间节点。
def delete_at_head(head: ListNode) -> ListNode:
if not head:
return None
return head.next
def delete_at_tail(head: ListNode) -> ListNode:
if not head:
return None
if not head.next:
return None
current_node = head
while current_node.next.next:
current_node = current_node.next
current_node.next = None
return head
def delete_at_position(head: ListNode, position: int) -> ListNode:
if position == 0:
return delete_at_head(head)
current_node = head
for _ in range(position - 1):
if not current_node:
raise IndexError("Position out of bounds")
current_node = current_node.next
if not current_node.next:
raise IndexError("Position out of bounds")
current_node.next = current_node.next.next
return head
3、查找操作
查找操作包括根据值查找节点和根据位置查找节点。
def find_by_value(head: ListNode, val: int) -> ListNode:
current_node = head
while current_node:
if current_node.val == val:
return current_node
current_node = current_node.next
return None
def find_by_position(head: ListNode, position: int) -> ListNode:
current_node = head
for _ in range(position):
if not current_node:
return None
current_node = current_node.next
return current_node
七、链表长度求解的应用
求链表长度是链表操作中的一个基本问题,它在许多应用中都有重要作用。
1、判断链表是否为空
通过判断链表长度是否为0,可以确定链表是否为空。
def is_empty(head: ListNode) -> bool:
return get_length(head) == 0
2、判断链表是否有环
通过判断链表长度是否无限,可以确定链表是否有环。使用快慢指针法可以有效检测链表中的环。
def has_cycle(head: ListNode) -> bool:
slow = head
fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
if slow == fast:
return True
return False
3、合并两个链表
在合并两个链表时,可以使用链表长度来确定合并后的链表长度。
def merge_two_lists(l1: ListNode, l2: ListNode) -> ListNode:
dummy = ListNode()
current = dummy
while l1 and l2:
if l1.val < l2.val:
current.next = l1
l1 = l1.next
else:
current.next = l2
l2 = l2.next
current = current.next
current.next = l1 if l1 else l2
return dummy.next
八、链表长度求解的优化
在求解链表长度时,我们可以通过一些优化方法来提高效率。
1、缓存链表长度
通过在链表节点中缓存链表长度,可以避免重复遍历链表,提高求长度的效率。
class ListNodeWithLength:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
self.length = 1 if not next else next.length + 1
def get_length_with_cache(head: ListNodeWithLength) -> int:
return head.length if head else 0
2、使用双指针法
双指针法是一种常用的优化技巧,通过两个指针的相对运动来减少遍历次数。
def get_middle_node(head: ListNode) -> ListNode:
slow = head
fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
return slow
九、链表长度求解的复杂度分析
在求解链表长度时,我们需要考虑时间复杂度和空间复杂度。
1、时间复杂度
遍历链表和递归求解链表长度的时间复杂度均为O(n),其中n是链表的长度。使用内置函数求长度的时间复杂度较高,因为需要先将链表转换为列表。
2、空间复杂度
遍历链表的空间复杂度为O(1),因为只使用了常数级别的额外空间。递归求解链表长度的空间复杂度为O(n),因为需要维护递归栈。使用内置函数求长度的空间复杂度为O(n),因为需要存储链表转换后的列表。
十、链表长度求解的实际应用
链表长度的求解在实际应用中有广泛的应用场景。
1、链表反转
在反转链表时,可以通过计算链表长度来确定反转的范围。
def reverse_linked_list(head: ListNode) -> ListNode:
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
2、分割链表
在分割链表时,可以通过计算链表长度来确定分割的位置。
def split_linked_list(head: ListNode, k: int) -> list:
length = get_length(head)
part_length = length // k
extra_nodes = length % k
parts = []
current = head
for _ in range(k):
part_head = current
for _ in range(part_length + (1 if extra_nodes > 0 else 0) - 1):
if current:
current = current.next
if current:
next_part = current.next
current.next = None
current = next_part
parts.append(part_head)
if extra_nodes > 0:
extra_nodes -= 1
return parts
总结
通过以上内容,我们详细介绍了在Python中求链表长度的多种方法,包括遍历链表、递归和使用内置函数等。我们还探讨了链表的基本知识、基本操作、优化技巧、复杂度分析及实际应用。希望这些内容能够帮助读者更好地理解和掌握链表长度的求解方法,并在实际编程中灵活应用。
相关问答FAQs:
如何在Python中定义一个链表?
在Python中,链表通常通过定义一个节点类来实现,每个节点包含数据和指向下一个节点的指针。以下是一个简单的链表节点定义示例:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
在Python中计算链表长度的常用方法是什么?
计算链表长度通常涉及遍历整个链表,直到达到最后一个节点。可以使用一个计数器来记录节点的数量。以下是一个实现示例:
def get_length(linked_list):
current_node = linked_list.head
count = 0
while current_node is not None:
count += 1
current_node = current_node.next
return count
链表长度的计算在实际应用中有什么重要性?
链表长度的计算对于许多算法和数据结构操作至关重要。例如,在进行链表插入、删除或查找操作时,了解链表的长度可以帮助优化性能。此外,它在实现某些算法时,如合并两个链表或反转链表,提供了必要的信息。
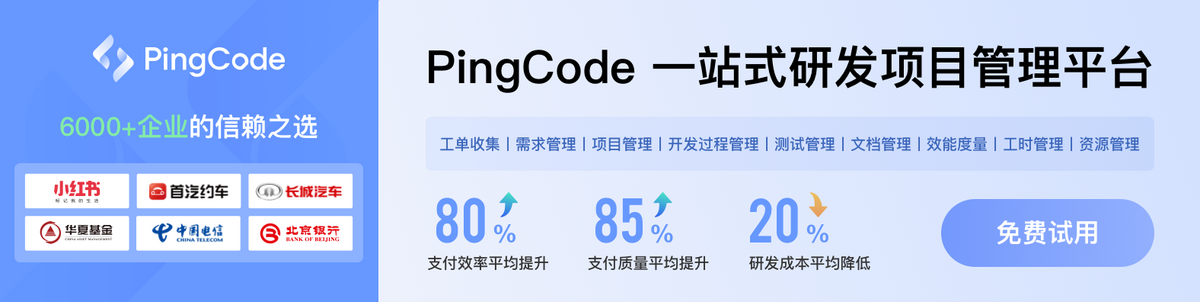