要学会Python面向对象编程,你需要了解类和对象、继承、多态、封装,并且通过实际项目进行练习。 面向对象编程(OOP)是一种编程范式,它使用“对象”来设计应用程序和计算机程序。这些对象可以包含数据,也可以包含代码:数据以字段的形式存在,代码以方法的形式存在。为了详细描述如何学会Python面向对象编程,我们需要从基础概念入手,然后逐步深入到更高级的特性和实际应用。
一、类和对象
1. 类的定义和对象的创建
类是对一类事物的抽象,而对象是类的实例。 在Python中,类是用class
关键字定义的。下面是一个简单的例子:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
print(f"{self.name} is barking.")
在这个例子中,Dog
类有一个构造方法__init__
,用于初始化对象的属性,还有一个方法bark
。我们可以创建Dog
类的对象并调用其方法:
my_dog = Dog("Buddy", 3)
print(my_dog.name) # 输出: Buddy
my_dog.bark() # 输出: Buddy is barking.
2. 类的属性和方法
属性是类的变量,方法是类的函数。 在类的定义中,属性定义了类的状态,而方法定义了类的行为。通过实例化类,我们可以创建对象,并通过对象访问类的属性和方法。
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def start_engine(self):
print(f"The engine of {self.year} {self.make} {self.model} is starting.")
通过这个例子,Car
类的属性包括make
、model
和year
,方法包括start_engine
。创建对象并调用其方法:
my_car = Car("Toyota", "Corolla", 2021)
print(my_car.make) # 输出: Toyota
my_car.start_engine() # 输出: The engine of 2021 Toyota Corolla is starting.
二、继承
1. 继承的基本概念
继承是面向对象编程的一大特性,它允许一个类继承另一个类的属性和方法。 通过继承,我们可以创建一个新的类,该类是现有类的扩展。新的类称为子类,现有的类称为父类或基类。
class Animal:
def __init__(self, species):
self.species = species
def eat(self):
print(f"{self.species} is eating.")
通过继承Animal
类,我们可以创建一个新的子类Dog
:
class Dog(Animal):
def __init__(self, name, age):
super().__init__("Dog")
self.name = name
self.age = age
def bark(self):
print(f"{self.name} is barking.")
在这个例子中,Dog
类继承了Animal
类的属性和方法,并增加了自己的属性和方法。
2. 重写方法
在子类中,我们可以重写父类的方法。 这意味着子类可以提供特定的实现,而不是使用父类的实现。
class Animal:
def make_sound(self):
print("Some generic animal sound")
class Dog(Animal):
def make_sound(self):
print("Woof woof!")
在这个例子中,Dog
类重写了Animal
类的make_sound
方法。
my_dog = Dog()
my_dog.make_sound() # 输出: Woof woof!
三、多态
1. 多态的基本概念
多态性是指在不同的类中调用相同的方法时,表现出不同的行为。 多态性使得程序更加灵活和可扩展。它允许我们编写能够处理不同对象的代码,而无需了解这些对象的具体类型。
class Animal:
def make_sound(self):
print("Some generic animal sound")
class Dog(Animal):
def make_sound(self):
print("Woof woof!")
class Cat(Animal):
def make_sound(self):
print("Meow meow!")
通过多态性,我们可以在处理不同类型的动物时调用相同的方法:
def animal_sound(animal):
animal.make_sound()
my_dog = Dog()
my_cat = Cat()
animal_sound(my_dog) # 输出: Woof woof!
animal_sound(my_cat) # 输出: Meow meow!
2. 使用多态性进行代码重用
多态性允许我们编写更加通用的代码,通过调用对象的通用方法,而不必担心对象的具体类型。这大大提高了代码的重用性和灵活性。
class Bird(Animal):
def make_sound(self):
print("Chirp chirp!")
def animal_sound(animal):
animal.make_sound()
my_bird = Bird()
animal_sound(my_bird) # 输出: Chirp chirp!
四、封装
1. 封装的基本概念
封装是指将数据和操作数据的方法绑定在一起,并隐藏类的内部实现细节。 这使得类的使用者不需要了解类的内部实现,只需要了解类提供的接口。封装提高了代码的可维护性和安全性。
class Account:
def __init__(self, owner, balance=0):
self.owner = owner
self.__balance = balance
def deposit(self, amount):
if amount > 0:
self.__balance += amount
print(f"Added {amount} to the balance")
else:
print("Deposit amount must be positive")
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
print(f"Withdrew {amount} from the balance")
else:
print("Invalid withdrawal amount")
def get_balance(self):
return self.__balance
在这个例子中,Account
类的__balance
属性是私有的,不能直接访问,只能通过类提供的方法访问。
2. 通过方法访问属性
封装的一个重要方面是通过方法访问和修改类的属性。 这可以确保类的内部状态只能通过受控的方式改变,从而避免意外的错误和不一致性。
my_account = Account("John", 100)
my_account.deposit(50) # 输出: Added 50 to the balance
my_account.withdraw(30) # 输出: Withdrew 30 from the balance
print(my_account.get_balance()) # 输出: 120
五、实际项目练习
1. 项目一:图书管理系统
通过实际项目练习是掌握面向对象编程的有效方法。 让我们通过一个简单的图书管理系统来练习面向对象编程的基本概念。
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
print(f"Added book: {book.title}")
def remove_book(self, isbn):
for book in self.books:
if book.isbn == isbn:
self.books.remove(book)
print(f"Removed book: {book.title}")
return
print("Book not found")
def find_book(self, isbn):
for book in self.books:
if book.isbn == isbn:
print(f"Found book: {book.title}")
return
print("Book not found")
在这个项目中,我们定义了Book
类和Library
类,并实现了添加、删除和查找图书的方法。
library = Library()
book1 = Book("1984", "George Orwell", "1234567890")
book2 = Book("To Kill a Mockingbird", "Harper Lee", "0987654321")
library.add_book(book1) # 输出: Added book: 1984
library.add_book(book2) # 输出: Added book: To Kill a Mockingbird
library.find_book("1234567890") # 输出: Found book: 1984
library.remove_book("1234567890") # 输出: Removed book: 1984
library.find_book("1234567890") # 输出: Book not found
2. 项目二:银行账户管理系统
通过另一个项目进一步练习和理解面向对象编程。 我们将创建一个银行账户管理系统,包括账户的创建、存款、取款和查询余额功能。
class Account:
def __init__(self, account_number, owner, balance=0):
self.account_number = account_number
self.owner = owner
self.__balance = balance
def deposit(self, amount):
if amount > 0:
self.__balance += amount
print(f"Deposited {amount} to account {self.account_number}")
else:
print("Deposit amount must be positive")
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
print(f"Withdrew {amount} from account {self.account_number}")
else:
print("Invalid withdrawal amount")
def get_balance(self):
return self.__balance
class Bank:
def __init__(self):
self.accounts = {}
def create_account(self, account_number, owner):
if account_number not in self.accounts:
self.accounts[account_number] = Account(account_number, owner)
print(f"Created account {account_number} for {owner}")
else:
print("Account number already exists")
def get_account(self, account_number):
return self.accounts.get(account_number, None)
通过这个项目,我们实现了银行账户的创建、存款、取款和查询余额功能。
bank = Bank()
bank.create_account("123", "Alice") # 输出: Created account 123 for Alice
bank.create_account("456", "Bob") # 输出: Created account 456 for Bob
account = bank.get_account("123")
if account:
account.deposit(1000) # 输出: Deposited 1000 to account 123
account.withdraw(500) # 输出: Withdrew 500 from account 123
print(account.get_balance()) # 输出: 500
else:
print("Account not found")
六、总结
通过理解和掌握类和对象、继承、多态、封装等面向对象编程的基本概念,并通过实际项目进行练习,你将能够熟练地使用Python进行面向对象编程。掌握这些概念不仅能提高你的编程技能,还能帮助你编写更加灵活、可维护和可扩展的代码。 继续练习和尝试更多的项目,将帮助你进一步巩固和提高你的面向对象编程能力。
相关问答FAQs:
如何开始学习Python的面向对象编程?
学习Python面向对象编程的第一步是理解基本的面向对象概念,如类、对象、继承和多态。可以通过在线课程、书籍或者视频教程来获取这些知识。建议从简单的示例代码开始,逐步尝试创建自己的类和对象,增强实践能力。
有哪些推荐的资源可以帮助理解Python面向对象?
许多资源可以帮助你深入了解Python的面向对象编程。书籍如《Python编程:从入门到实践》和《流畅的Python》都是不错的选择。此外,像Codecademy、Coursera和Udemy等平台提供的在线课程也非常有用,结合实际项目进行练习会更有效。
在学习过程中常见的错误有哪些?如何避免?
学习Python面向对象时,常见的错误包括对类和对象的理解不清、方法和属性的命名不规范、以及未能正确使用继承和多态等。为了避免这些错误,可以在编写代码时多进行代码审查,参考良好的编程规范,并时常回顾面向对象的核心原则,确保自己对概念的理解准确无误。
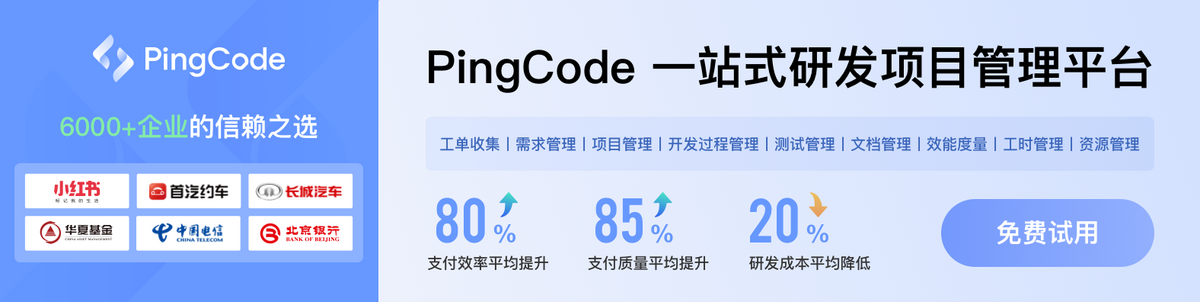