使用Python更改文件类型的方法有多种,例如重命名文件扩展名、使用文件转换库进行格式转换、通过读取和写入不同格式来实现。其中,重命名文件扩展名是最简单的方法,只需修改文件名的扩展名即可。以下是详细描述:读取文件内容并写入新文件格式是一种常见且有效的方法。通过读取文件的内容,再按照目标格式的要求将内容写入新的文件中,从而完成文件类型的转换。
一、重命名文件扩展名
重命名文件扩展名是更改文件类型最简单的方法之一。这种方法适用于文件内容不需要改变,只需更改文件名的扩展名。例如,将一个文本文件从 .txt
重命名为 .md
。使用 Python,可以使用 os
模块中的 rename
函数来完成这个任务。
import os
def rename_file_extension(file_path, new_extension):
base = os.path.splitext(file_path)[0]
new_file_path = f"{base}.{new_extension}"
os.rename(file_path, new_file_path)
return new_file_path
示例
old_file = "example.txt"
new_extension = "md"
new_file = rename_file_extension(old_file, new_extension)
print(f"文件已重命名为: {new_file}")
二、使用文件转换库进行格式转换
有些文件类型之间的转换可以通过使用专门的文件转换库来实现。这些库可以处理复杂的文件格式并自动执行转换操作。例如,可以使用 Pillow
库将图像从一种格式转换为另一种格式。
from PIL import Image
def convert_image_format(input_file, output_file):
with Image.open(input_file) as img:
img.save(output_file)
示例
input_file = "example.jpg"
output_file = "example.png"
convert_image_format(input_file, output_file)
print(f"图像已转换为: {output_file}")
三、读取文件内容并写入新文件格式
这种方法适用于需要根据内容进行格式转换的场景。通过读取源文件的内容,然后按照目标格式的要求写入新的文件中,可以完成文件类型的转换。
def txt_to_csv(txt_file, csv_file):
with open(txt_file, 'r') as infile, open(csv_file, 'w') as outfile:
for line in infile:
csv_line = ','.join(line.split())
outfile.write(csv_line + '\n')
示例
txt_file = "example.txt"
csv_file = "example.csv"
txt_to_csv(txt_file, csv_file)
print(f"文件已转换为: {csv_file}")
四、使用第三方服务或API进行文件转换
有些文件转换任务可以通过调用第三方服务或API来完成。这些服务通常提供强大的文件转换功能,可以处理多种文件格式。以下是一个使用 requests
库调用在线文件转换API的示例。
import requests
def convert_file_online(api_url, input_file, output_file, params):
with open(input_file, 'rb') as infile:
response = requests.post(api_url, files={'file': infile}, data=params)
response.raise_for_status()
with open(output_file, 'wb') as outfile:
outfile.write(response.content)
示例
api_url = "https://api.example.com/convert"
input_file = "example.docx"
output_file = "example.pdf"
params = {"target_format": "pdf"}
convert_file_online(api_url, input_file, output_file, params)
print(f"文件已通过在线服务转换为: {output_file}")
五、音视频文件格式转换
音频和视频文件的格式转换通常需要使用专门的多媒体处理库,如 ffmpeg
或 moviepy
。这些库提供了丰富的功能,可以处理各种多媒体格式和编解码器。
import moviepy.editor as mp
def convert_video_format(input_file, output_file):
clip = mp.VideoFileClip(input_file)
clip.write_videofile(output_file)
示例
input_file = "example.mp4"
output_file = "example.avi"
convert_video_format(input_file, output_file)
print(f"视频已转换为: {output_file}")
六、处理文档文件格式转换
文档文件的格式转换(如从 .docx
转换为 .pdf
)通常需要使用专门的文档处理库,如 python-docx
或 reportlab
。这些库可以处理复杂的文档结构和格式要求。
from docx import Document
from fpdf import FPDF
def docx_to_pdf(docx_file, pdf_file):
doc = Document(docx_file)
pdf = FPDF()
pdf.add_page()
pdf.set_auto_page_break(auto=True, margin=15)
pdf.set_font("Arial", size=12)
for paragraph in doc.paragraphs:
pdf.multi_cell(0, 10, paragraph.text)
pdf.output(pdf_file)
示例
docx_file = "example.docx"
pdf_file = "example.pdf"
docx_to_pdf(docx_file, pdf_file)
print(f"文档已转换为: {pdf_file}")
七、使用Pandas进行数据文件格式转换
对于数据文件(如 .csv
和 .xlsx
),可以使用 pandas
库进行格式转换。pandas
提供了丰富的数据处理功能,可以轻松读取和写入多种数据格式。
import pandas as pd
def convert_csv_to_excel(csv_file, excel_file):
df = pd.read_csv(csv_file)
df.to_excel(excel_file, index=False)
示例
csv_file = "example.csv"
excel_file = "example.xlsx"
convert_csv_to_excel(csv_file, excel_file)
print(f"数据已转换为: {excel_file}")
八、总结
在Python中,更改文件类型的方法多种多样,适用于不同的应用场景。无论是简单的重命名文件扩展名,还是使用专门的库进行复杂的文件转换,都可以通过合理选择和组合这些方法来实现。掌握这些方法,可以有效应对日常开发和数据处理中的文件转换需求。
相关问答FAQs:
如何在Python中将文件从一种格式转换为另一种格式?
在Python中,转换文件格式通常依赖于文件的类型。例如,使用Pandas库可以轻松地将CSV文件转换为Excel格式,使用PIL库可以将图片格式从JPEG转换为PNG。具体方法是打开原文件,读取数据,然后以目标格式保存。例如,使用pd.read_csv()
读取CSV文件,再使用to_excel()
方法保存为Excel文件。
如果我不知道文件的当前类型,如何检查文件类型?
可以使用Python的mimetypes
模块来识别文件类型。这个模块可以根据文件的扩展名返回其MIME类型。你可以通过调用mimetypes.guess_type('filename')
来获取文件的类型信息。这将帮助你确定如何进行格式转换。
使用Python更改文件类型时有哪些常见错误需要注意?
转换文件类型时,常见错误包括文件路径不正确、文件格式不支持以及数据丢失等。在进行转换前,确保文件路径是正确的,并且目标格式能够处理原文件的数据。例如,尝试将一个文本文件直接转换为图像格式可能会导致无法读取的文件。此外,建议在转换前备份原文件,以防出现意外情况导致数据丢失。
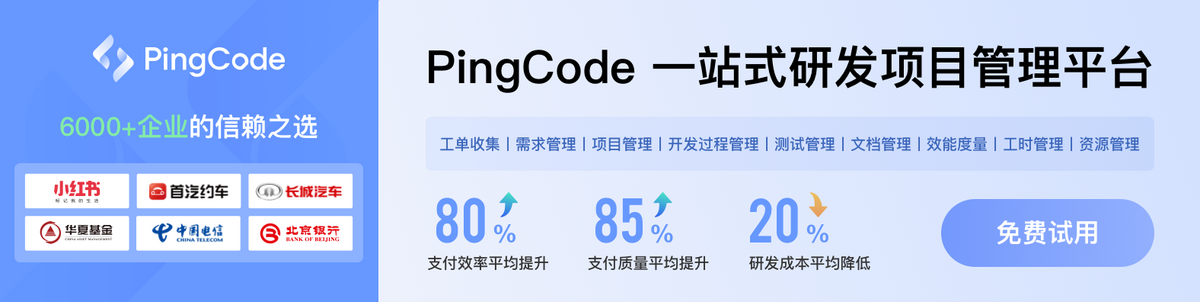