要用Python画树状图,可以使用多种库,如matplotlib
、networkx
、pydot
等。最常用的库是matplotlib
、networkx
和pydot
,它们各自有不同的优势。下面我将详细介绍如何使用matplotlib
库来画树状图。
使用matplotlib
库画树状图,需要先安装库并导入相关模块。接下来,定义树的节点和边,将它们绘制出来。matplotlib
库提供了丰富的图形绘制功能,但绘制树状图需要一些额外的处理,比如计算节点的位置和绘制边。以下是一个完整的示例代码:
import matplotlib.pyplot as plt
import networkx as nx
定义树的结构
edges = [
('A', 'B'),
('A', 'C'),
('B', 'D'),
('B', 'E'),
('C', 'F'),
('C', 'G')
]
创建一个有向图
G = nx.DiGraph()
G.add_edges_from(edges)
计算节点的布局
pos = nx.spring_layout(G)
绘制节点和边
nx.draw(G, pos, with_labels=True, node_size=3000, node_color="skyblue", font_size=15, font_weight="bold", arrows=True)
显示绘图
plt.show()
下面将详细介绍如何使用其他库如networkx
和pydot
来画树状图,以及针对一些复杂情况的处理方法。
一、MATPLOTLIB库
1、安装和导入
首先,确保你已经安装了matplotlib
库。可以使用以下命令安装:
pip install matplotlib
然后,在你的Python脚本中导入matplotlib
和其他必要的模块:
import matplotlib.pyplot as plt
import networkx as nx
2、定义树结构
树状图由节点和边组成。你可以使用一个列表来定义树的边,每个边用一个元组表示:
edges = [
('A', 'B'),
('A', 'C'),
('B', 'D'),
('B', 'E'),
('C', 'F'),
('C', 'G')
]
3、创建图并添加边
创建一个有向图并添加边:
G = nx.DiGraph()
G.add_edges_from(edges)
4、计算节点布局
使用networkx
库的spring_layout
函数计算节点的布局:
pos = nx.spring_layout(G)
5、绘制图形
使用networkx
库的draw
函数绘制节点和边:
nx.draw(G, pos, with_labels=True, node_size=3000, node_color="skyblue", font_size=15, font_weight="bold", arrows=True)
6、显示图形
使用matplotlib
库的show
函数显示图形:
plt.show()
二、NETWORKX库
1、安装和导入
确保你已经安装了networkx
库。可以使用以下命令安装:
pip install networkx
然后,在你的Python脚本中导入networkx
和其他必要的模块:
import networkx as nx
import matplotlib.pyplot as plt
2、定义树结构
与之前相同,使用一个列表来定义树的边:
edges = [
('A', 'B'),
('A', 'C'),
('B', 'D'),
('B', 'E'),
('C', 'F'),
('C', 'G')
]
3、创建图并添加边
创建一个有向图并添加边:
G = nx.DiGraph()
G.add_edges_from(edges)
4、计算节点布局
使用networkx
库的spring_layout
函数计算节点的布局:
pos = nx.spring_layout(G)
5、绘制图形
使用networkx
库的draw
函数绘制节点和边:
nx.draw(G, pos, with_labels=True, node_size=3000, node_color="skyblue", font_size=15, font_weight="bold", arrows=True)
6、显示图形
使用matplotlib
库的show
函数显示图形:
plt.show()
三、PYDOT库
1、安装和导入
确保你已经安装了pydot
库和graphviz
库。可以使用以下命令安装:
pip install pydot
pip install graphviz
然后,在你的Python脚本中导入pydot
和其他必要的模块:
import pydot
from IPython.display import Image, display
2、定义树结构
使用一个列表来定义树的边:
edges = [
('A', 'B'),
('A', 'C'),
('B', 'D'),
('B', 'E'),
('C', 'F'),
('C', 'G')
]
3、创建图并添加边
创建一个有向图并添加边:
graph = pydot.Dot(graph_type='digraph')
for edge in edges:
graph.add_edge(pydot.Edge(edge[0], edge[1]))
4、保存和显示图形
保存图形为图像文件并显示:
graph.write_png('tree.png')
display(Image('tree.png'))
四、复杂树状图的绘制
在处理复杂树状图时,可能需要更复杂的布局算法和更多的图形属性设置。以下是一些可以考虑的方面:
1、层次布局
对于层次结构的树状图,可以使用networkx
库的hierarchy_pos
函数来计算节点的布局:
def hierarchy_pos(G, root=None, width=1., vert_gap=0.2, vert_loc=0, xcenter=0.5):
'''
If the graph is a tree this will return the positions to plot this in a
hierarchical layout.
Based on Joel's answer at https://stackoverflow.com/a/29597209/2966723,
but with some modifications.
'''
if not nx.is_tree(G):
raise TypeError('cannot use hierarchy_pos on a graph that is not a tree')
if root is None:
root = next(iter(nx.topological_sort(G))) # allows for non-unique root
def _hierarchy_pos(G, root, width=1., vert_gap=0.2, vert_loc=0, xcenter=0.5, pos=None, parent=None, parsed=[]):
if pos is None:
pos = {root: (xcenter, vert_loc)}
else:
pos[root] = (xcenter, vert_loc)
children = list(G.neighbors(root))
if not isinstance(G, nx.DiGraph) and parent is not None:
children.remove(parent)
if len(children) != 0:
dx = width / len(children)
nextx = xcenter - width / 2 - dx / 2
for child in children:
nextx += dx
pos = _hierarchy_pos(G, child, width=dx, vert_gap=vert_gap, vert_loc=vert_loc - vert_gap, xcenter=nextx,
pos=pos, parent=root, parsed=parsed)
return pos
return _hierarchy_pos(G, root, width, vert_gap, vert_loc, xcenter)
使用 hierarchy_pos 计算节点的布局
pos = hierarchy_pos(G)
nx.draw(G, pos=pos, with_labels=True, node_size=3000, node_color="skyblue", font_size=15, font_weight="bold", arrows=True)
plt.show()
2、树的宽度和深度
确保你的树状图在宽度和深度上合理分布,可以避免节点之间的重叠。你可以调整spring_layout
或其他布局算法的参数来优化布局。
3、节点和边的样式
使用networkx
库的draw
函数提供的参数来调整节点和边的样式:
nx.draw(G, pos, with_labels=True, node_size=3000, node_color="skyblue", font_size=15, font_weight="bold", arrows=True,
edge_color='gray', style='dashed')
4、添加标签和注释
为节点和边添加标签和注释,使树状图更具信息性:
labels = {node: node for node in G.nodes()}
nx.draw(G, pos, labels=labels, with_labels=True, node_size=3000, node_color="skyblue", font_size=15, font_weight="bold", arrows=True)
edge_labels = {edge: edge[1] for edge in G.edges()}
nx.draw_networkx_edge_labels(G, pos, edge_labels=edge_labels, font_size=12)
五、总结
通过上述方法,你可以使用Python中的matplotlib
、networkx
和pydot
库绘制树状图。每个库都有其独特的功能和优势,根据具体需求选择合适的库和方法。在处理复杂树状图时,合理调整布局算法和图形属性可以使图形更加美观和信息丰富。希望通过本文的详细介绍,能够帮助你更好地绘制树状图。
相关问答FAQs:
如何使用Python绘制树状图的基本步骤是什么?
在Python中绘制树状图通常可以使用多个库,如Matplotlib、Seaborn和Plotly等。首先,您需要安装相关的库,例如通过命令pip install matplotlib seaborn
进行安装。接着,您可以准备数据,通常以层级结构的形式存在。最后,使用相应的函数来绘制树状图,比如matplotlib
中的plot
函数,或seaborn
中的clustermap
函数。
有哪些Python库可以帮助绘制树状图?
Python中有几个流行的库可以帮助您绘制树状图。Matplotlib
是最常用的绘图库,它提供了灵活的绘图功能。Seaborn
在Matplotlib的基础上进行了封装,适合快速创建统计图形。Plotly
则允许用户创建交互式的图形,适合需要展示的场景。Scikit-learn
也提供了一些树状图的绘制功能,特别是在机器学习模型的可视化方面。
如何自定义树状图的外观以提高可读性?
自定义树状图的外观可以通过调整图形的颜色、标签、字体大小等多个方面来实现。在Matplotlib
中,您可以使用set_title()
、set_xlabel()
和set_ylabel()
等函数来设置标题和标签的样式。使用plt.xticks()
和plt.yticks()
可以调整坐标轴的刻度和标签样式。添加网格线、改变线条样式和颜色也能显著提高图形的可读性和美观性。
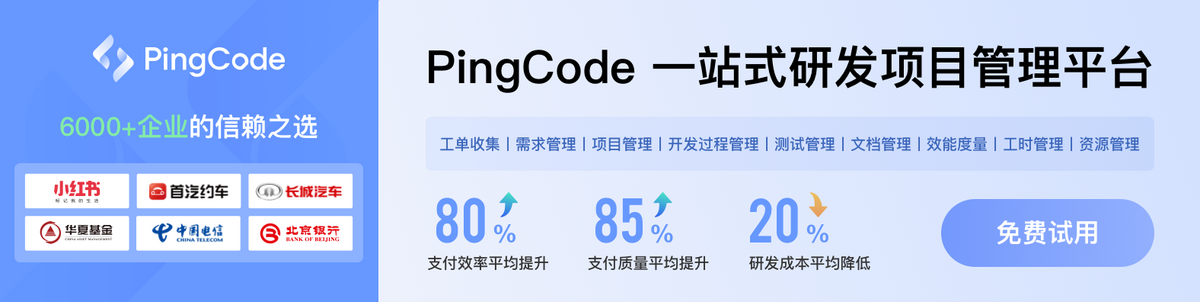