在Python中生成中间文件的方法有很多种,常见的方法包括使用临时文件、手动创建文件、使用特定库生成文件。其中,使用临时文件是一种非常常见且安全的方法,因为它能确保文件在使用完毕后被自动删除,从而避免文件污染。详细描述一下临时文件的使用方法:
使用tempfile
模块创建临时文件:
tempfile
模块是Python标准库的一部分,它提供了生成临时文件和目录的功能。使用tempfile
模块可以轻松创建一个临时文件,且无需担心文件名冲突等问题。以下是一个简单的例子:
import tempfile
创建一个临时文件
with tempfile.NamedTemporaryFile(delete=False) as temp_file:
# 向临时文件中写入内容
temp_file.write(b'This is some temporary data.')
temp_file_path = temp_file.name
print(f'Temporary file created at: {temp_file_path}')
在这个示例中,NamedTemporaryFile
函数创建了一个临时文件,并且在with
块内部操作该文件。delete=False
参数确保文件在关闭时不会被删除。可以使用temp_file.name
获取临时文件的路径,以便在后续操作中使用该文件。
一、临时文件的使用
临时文件在许多情况下非常有用,尤其是在需要确保文件不会永久留存的情境下。它们可以在数据处理中用于存储中间结果、在网络应用中用于存储下载的数据片段等。
1、使用tempfile
模块
tempfile
模块提供了多种创建临时文件和目录的方法。除了NamedTemporaryFile
,还有TemporaryFile
、mkstemp
和mkdtemp
等函数。
TemporaryFile
:创建一个临时文件,文件关闭时会自动删除。mkstemp
:创建一个临时文件并返回文件描述符和文件路径。mkdtemp
:创建一个临时目录并返回目录路径。
以下是使用这些函数的示例:
import tempfile
import os
使用TemporaryFile创建临时文件
with tempfile.TemporaryFile() as temp_file:
temp_file.write(b'This is some temporary data.')
temp_file.seek(0)
print(temp_file.read())
使用mkstemp创建临时文件
fd, path = tempfile.mkstemp()
with os.fdopen(fd, 'w') as temp_file:
temp_file.write('This is some temporary data.')
print(f'Temporary file created at: {path}')
os.remove(path) # 手动删除临时文件
使用mkdtemp创建临时目录
temp_dir = tempfile.mkdtemp()
print(f'Temporary directory created at: {temp_dir}')
os.rmdir(temp_dir) # 手动删除临时目录
2、临时文件在数据处理中的应用
在数据处理中,临时文件可以用于存储中间结果,以便后续操作使用。例如,在数据清洗过程中,可以将清洗后的数据存储在临时文件中,随后进行进一步处理。
import tempfile
import pandas as pd
生成示例数据
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
清洗数据
df_cleaned = df[df['age'] > 28]
存储清洗后的数据到临时文件
with tempfile.NamedTemporaryFile(delete=False, suffix='.csv') as temp_file:
df_cleaned.to_csv(temp_file.name, index=False)
temp_file_path = temp_file.name
print(f'Cleaned data stored at: {temp_file_path}')
二、手动创建文件
除了使用临时文件,有时我们需要手动创建文件。这种方法适用于需要持久化存储的情况。常见的方法是使用内置的open
函数。
1、使用open
函数创建文件
使用open
函数可以轻松创建和操作文件。以下是一个创建文件并写入内容的示例:
# 创建文件并写入内容
with open('example.txt', 'w') as file:
file.write('This is some example data.')
print('File created: example.txt')
在这个示例中,open
函数以写模式('w'
)打开文件,如果文件不存在则创建文件,随后写入内容。with
块确保文件在操作完成后自动关闭。
2、文件操作的常见模式
open
函数支持多种文件操作模式,包括:
'r'
:只读模式(默认)。'w'
:写模式,覆盖文件内容。'a'
:追加模式,在文件末尾添加内容。'b'
:二进制模式,与其他模式结合使用。'+'
:读写模式,与其他模式结合使用。
以下是一些常见模式的示例:
# 读模式
with open('example.txt', 'r') as file:
content = file.read()
print('File content:', content)
追加模式
with open('example.txt', 'a') as file:
file.write('\nThis is additional data.')
二进制模式
with open('example.bin', 'wb') as file:
file.write(b'\x00\x01\x02\x03')
读写模式
with open('example.txt', 'r+') as file:
content = file.read()
file.write('\nThis is read-write mode.')
三、使用特定库生成文件
在某些情况下,特定的库可以帮助我们生成特定类型的文件。这些库通常提供了更高层次的抽象,使得文件生成过程更加简便。
1、使用pandas
生成CSV文件
pandas
库提供了强大的数据处理和文件生成功能。以下是生成CSV文件的示例:
import pandas as pd
生成示例数据
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
存储数据到CSV文件
df.to_csv('example.csv', index=False)
print('CSV file created: example.csv')
2、使用openpyxl
生成Excel文件
openpyxl
库允许我们创建和操作Excel文件。以下是生成Excel文件的示例:
import openpyxl
创建一个新的Excel工作簿
wb = openpyxl.Workbook()
ws = wb.active
写入数据
ws.append(['Name', 'Age'])
ws.append(['Alice', 25])
ws.append(['Bob', 30])
ws.append(['Charlie', 35])
保存工作簿到文件
wb.save('example.xlsx')
print('Excel file created: example.xlsx')
3、使用reportlab
生成PDF文件
reportlab
库允许我们创建PDF文件。以下是生成PDF文件的示例:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
创建一个新的PDF文件
pdf_file = 'example.pdf'
c = canvas.Canvas(pdf_file, pagesize=letter)
写入数据
c.drawString(100, 750, 'This is an example PDF file.')
c.drawString(100, 730, 'Generated using reportlab.')
保存PDF文件
c.save()
print('PDF file created: example.pdf')
四、生成图像文件
在某些应用场景中,我们需要生成图像文件。Python提供了多个库来实现这一目标,包括Pillow
和matplotlib
。
1、使用Pillow
生成图像文件
Pillow
是Python Imaging Library(PIL)的一个分支,提供了强大的图像处理功能。以下是生成图像文件的示例:
from PIL import Image, ImageDraw, ImageFont
创建一个新的图像
image = Image.new('RGB', (200, 100), color=(255, 255, 255))
draw = ImageDraw.Draw(image)
绘制文本
font = ImageFont.load_default()
draw.text((10, 10), 'Hello, World!', fill=(0, 0, 0), font=font)
保存图像到文件
image.save('example.png')
print('Image file created: example.png')
2、使用matplotlib
生成图像文件
matplotlib
是一个强大的绘图库,常用于生成图表和图像文件。以下是生成图像文件的示例:
import matplotlib.pyplot as plt
生成示例数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
创建图表
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Example Plot')
保存图表到文件
plt.savefig('example_plot.png')
print('Plot image file created: example_plot.png')
五、生成JSON文件
在现代应用中,JSON(JavaScript Object Notation)文件广泛用于数据交换。Python标准库提供了json
模块来处理JSON数据。
1、使用json
模块生成JSON文件
以下是一个生成JSON文件的示例:
import json
生成示例数据
data = {
'name': 'Alice',
'age': 25,
'city': 'Wonderland'
}
存储数据到JSON文件
with open('example.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
print('JSON file created: example.json')
2、读取和写入复杂的JSON数据
json
模块还支持读取和写入复杂的JSON数据,例如嵌套结构和列表。以下是一个示例:
import json
生成复杂的示例数据
data = {
'name': 'Alice',
'age': 25,
'address': {
'street': '123 Wonderland Ave',
'city': 'Wonderland',
'zipcode': '12345'
},
'hobbies': ['reading', 'gardening', 'painting']
}
存储数据到JSON文件
with open('complex_example.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
print('Complex JSON file created: complex_example.json')
六、生成XML文件
XML(eXtensible Markup Language)是另一种常用于数据交换的格式。Python提供了多个库来处理XML数据,包括xml.etree.ElementTree
和lxml
。
1、使用xml.etree.ElementTree
生成XML文件
xml.etree.ElementTree
是Python标准库的一部分,提供了生成和解析XML文件的功能。以下是生成XML文件的示例:
import xml.etree.ElementTree as ET
创建根元素
root = ET.Element('person')
创建子元素
name = ET.SubElement(root, 'name')
name.text = 'Alice'
age = ET.SubElement(root, 'age')
age.text = '25'
创建XML树
tree = ET.ElementTree(root)
保存XML到文件
tree.write('example.xml')
print('XML file created: example.xml')
2、使用lxml
生成XML文件
lxml
是一个功能强大的XML处理库,提供了更高效和灵活的XML生成和解析功能。以下是一个生成XML文件的示例:
from lxml import etree
创建根元素
root = etree.Element('person')
创建子元素
name = etree.SubElement(root, 'name')
name.text = 'Alice'
age = etree.SubElement(root, 'age')
age.text = '25'
创建XML树
tree = etree.ElementTree(root)
保存XML到文件
tree.write('example_lxml.xml', pretty_print=True, xml_declaration=True, encoding='UTF-8')
print('XML file created: example_lxml.xml')
七、生成HTML文件
HTML(HyperText Markup Language)文件用于创建网页。在Python中,生成HTML文件的方法有很多,包括使用html
模块、Jinja2
模板引擎等。
1、使用html
模块生成HTML文件
html
模块是Python标准库的一部分,提供了生成和转义HTML内容的功能。以下是生成HTML文件的示例:
import html
生成示例HTML内容
html_content = '''
<!DOCTYPE html>
<html>
<head>
<title>Example HTML</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is an example HTML file.</p>
</body>
</html>
'''
存储HTML内容到文件
with open('example.html', 'w') as html_file:
html_file.write(html_content)
print('HTML file created: example.html')
2、使用Jinja2
模板引擎生成HTML文件
Jinja2
是一个现代的模板引擎,常用于生成HTML文件。以下是一个使用Jinja2
生成HTML文件的示例:
from jinja2 import Template
生成示例数据
data = {
'title': 'Example HTML',
'heading': 'Hello, World!',
'paragraph': 'This is an example HTML file generated using Jinja2.'
}
生成HTML模板
template = Template('''
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ heading }}</h1>
<p>{{ paragraph }}</p>
</body>
</html>
''')
渲染模板
html_content = template.render(data)
存储HTML内容到文件
with open('example_jinja2.html', 'w') as html_file:
html_file.write(html_content)
print('HTML file created: example_jinja2.html')
八、生成日志文件
日志文件用于记录应用程序的运行状态和错误信息。在Python中,生成日志文件的常用方法是使用logging
模块。
1、使用logging
模块生成日志文件
logging
模块是Python标准库的一部分,提供了强大的日志记录功能。以下是生成日志文件的示例:
import logging
配置日志记录
logging.basicConfig(filename='example.log', level=logging.DEBUG,
format='%(asctime)s - %(levelname)s - %(message)s')
记录日志信息
logging.debug('This is a debug message.')
logging.info('This is an info message.')
logging.warning('This is a warning message.')
logging.error('This is an error message.')
logging.critical('This is a critical message.')
print('Log file created: example.log')
2、日志记录的高级配置
logging
模块支持高级配置,例如使用RotatingFileHandler
实现日志文件轮转。以下是一个示例:
import logging
from logging.handlers import RotatingFileHandler
配置日志记录
handler = RotatingFileHandler('example_rotating.log', maxBytes=2000, backupCount=5)
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s', handlers=[handler])
记录日志信息
for i in range(100):
logging.debug(f'This is debug message {i}')
print('Rotating log file created: example_rotating.log')
总结
生成中间文件在数据处理、网络应用、日志记录等众多领域都有广泛的应用。在Python中,常见的方法包括使用临时文件、手动创建文件、使用特定库生成文件。不同的方法适用于不同的应用场景,通过合理选择和使用这些方法,可以高效地生成所需的中间文件。
相关问答FAQs:
如何在Python中创建和写入中间文件?
在Python中,可以使用内置的open()
函数创建和写入中间文件。首先,指定文件名和模式(例如,'w'表示写入模式)。接下来,使用write()
方法将数据写入文件。完成后,务必使用close()
方法关闭文件,或者使用with
语句自动管理文件的打开和关闭。例如:
with open('intermediate_file.txt', 'w') as file:
file.write('这是一个中间文件的示例。')
Python支持哪些文件格式的中间文件生成?
Python支持多种文件格式的中间文件生成,包括文本文件(.txt)、CSV文件(.csv)、JSON文件(.json)以及Excel文件(.xlsx)等。您可以使用相应的库,例如csv
、json
或pandas
来处理不同格式的数据,确保生成的中间文件符合您的需求。
如何处理生成的中间文件以提高性能?
在处理生成的中间文件时,可以采取一些优化措施来提高性能。考虑使用缓冲区(例如,在open()
时指定buffering
参数),以减少磁盘I/O操作的次数。此外,使用with
语句管理文件操作可以确保资源的有效使用,避免内存泄漏等问题。对于大型数据集,考虑分块写入文件,保持内存使用在可控范围内。
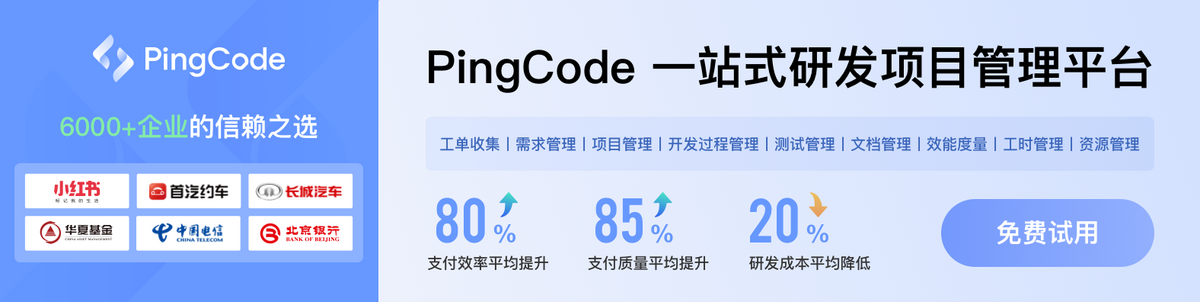