在Python中,爬取网络数据可以使用多种工具和库,例如requests、BeautifulSoup、Scrapy、Selenium等。requests库用于发送HTTP请求、BeautifulSoup用于解析HTML文档、Scrapy是一个强大的爬虫框架、Selenium用于模拟浏览器操作。下面将详细描述如何使用这些工具和库进行数据爬取。
一、REQUESTS库
requests库是一个简单易用的HTTP库,适用于发送GET和POST请求,获取网页内容。
1. 安装requests库
首先,确保你已经安装了requests库,可以通过以下命令安装:
pip install requests
2. 发送HTTP请求
使用requests库发送HTTP请求,并获取网页内容:
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print('Failed to retrieve the webpage')
requests.get(url)发送GET请求,返回一个Response对象。response.text获取网页的HTML内容。
二、BEAUTIFULSOUP库
BeautifulSoup是一个用于解析HTML和XML文档的库,常与requests库配合使用,用于从网页中提取数据。
1. 安装BeautifulSoup库
安装BeautifulSoup库及其依赖的解析器lxml:
pip install beautifulsoup4
pip install lxml
2. 解析网页内容
使用BeautifulSoup解析网页内容,并提取所需数据:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
# 提取网页标题
title = soup.title.string
print(title)
# 提取所有链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
else:
print('Failed to retrieve the webpage')
BeautifulSoup(response.text, 'lxml')解析网页内容,soup.title.string获取网页标题,soup.find_all('a')获取所有链接。
三、SCRAPY框架
Scrapy是一个功能强大的爬虫框架,适用于构建复杂的爬虫项目,支持多种扩展和中间件。
1. 安装Scrapy框架
安装Scrapy框架:
pip install scrapy
2. 创建Scrapy项目
使用Scrapy创建一个新项目:
scrapy startproject myproject
项目结构如下:
myproject/
scrapy.cfg
myproject/
__init__.py
items.py
middlewares.py
pipelines.py
settings.py
spiders/
__init__.py
myspider.py
3. 创建Spider
在spiders目录下创建一个新的Spider:
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com']
def parse(self, response):
# 提取网页标题
title = response.xpath('//title/text()').get()
print(title)
# 提取所有链接
links = response.xpath('//a/@href').getall()
for link in links:
print(link)
4. 运行Spider
运行Spider,开始爬取数据:
scrapy crawl myspider
四、SELENIUM库
Selenium是一个用于自动化浏览器操作的工具,适用于处理动态网页内容。
1. 安装Selenium库
安装Selenium库及其依赖的浏览器驱动,例如ChromeDriver:
pip install selenium
下载并安装ChromeDriver:https://sites.google.com/a/chromium.org/chromedriver/downloads
2. 使用Selenium模拟浏览器操作
使用Selenium模拟浏览器操作,获取网页内容:
from selenium import webdriver
配置Chrome浏览器
options = webdriver.ChromeOptions()
options.add_argument('--headless')
driver = webdriver.Chrome(options=options)
url = 'http://example.com'
driver.get(url)
提取网页标题
title = driver.title
print(title)
提取所有链接
links = driver.find_elements_by_tag_name('a')
for link in links:
print(link.get_attribute('href'))
driver.quit()
webdriver.Chrome(options=options)配置并启动Chrome浏览器,driver.get(url)打开网页,driver.title获取网页标题,driver.find_elements_by_tag_name('a')获取所有链接。
五、数据存储
爬取的数据可以存储到各种格式和数据库中,例如CSV文件、JSON文件、SQLite数据库、MySQL数据库等。
1. 存储为CSV文件
使用Python内置的csv模块,将数据存储为CSV文件:
import csv
data = [['Title', 'Link'], ['Example Title', 'http://example.com']]
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
2. 存储为JSON文件
使用Python内置的json模块,将数据存储为JSON文件:
import json
data = {'title': 'Example Title', 'link': 'http://example.com'}
with open('data.json', 'w') as file:
json.dump(data, file)
3. 存储到SQLite数据库
使用Python内置的sqlite3模块,将数据存储到SQLite数据库:
import sqlite3
conn = sqlite3.connect('data.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS data (title TEXT, link TEXT)''')
c.execute('''INSERT INTO data (title, link) VALUES (?, ?)''', ('Example Title', 'http://example.com'))
conn.commit()
conn.close()
4. 存储到MySQL数据库
使用MySQL数据库时,需要安装MySQL驱动,例如mysql-connector-python:
pip install mysql-connector-python
将数据存储到MySQL数据库:
import mysql.connector
conn = mysql.connector.connect(
host='localhost',
user='yourusername',
password='yourpassword',
database='yourdatabase'
)
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS data (title VARCHAR(255), link VARCHAR(255))''')
cursor.execute('''INSERT INTO data (title, link) VALUES (%s, %s)''', ('Example Title', 'http://example.com'))
conn.commit()
conn.close()
六、处理反爬机制
在实际爬取过程中,可能会遇到反爬机制,例如IP封锁、验证码等。以下是几种常见的处理方法:
1. 使用代理
使用代理IP,避免被封锁:
import requests
proxies = {
'http': 'http://yourproxy:port',
'https': 'https://yourproxy:port'
}
response = requests.get('http://example.com', proxies=proxies)
2. 设置请求头
设置请求头,模拟浏览器请求:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get('http://example.com', headers=headers)
3. 使用Selenium
使用Selenium模拟浏览器操作,处理动态内容和验证码:
from selenium import webdriver
options = webdriver.ChromeOptions()
options.add_argument('--headless')
driver = webdriver.Chrome(options=options)
url = 'http://example.com'
driver.get(url)
处理验证码、动态内容等
...
driver.quit()
七、多线程与分布式爬取
对于大规模数据爬取,可以使用多线程和分布式爬取技术,提高爬取效率。
1. 使用多线程
使用Python的threading模块,实现多线程爬取:
import threading
import requests
def fetch(url):
response = requests.get(url)
if response.status_code == 200:
print(response.text)
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
threads = []
for url in urls:
thread = threading.Thread(target=fetch, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2. 使用Scrapy的分布式扩展
Scrapy支持分布式爬取,可以使用Scrapy-Redis扩展,将爬取任务分配到多个节点:
pip install scrapy-redis
在Scrapy项目的settings.py中配置Scrapy-Redis:
# 使用Scrapy-Redis的调度器和去重器
SCHEDULER = "scrapy_redis.scheduler.Scheduler"
DUPEFILTER_CLASS = "scrapy_redis.dupefilter.RFPDupeFilter"
配置Redis连接
REDIS_URL = 'redis://user:pass@hostname:9001'
在Spider中使用Redis队列:
from scrapy_redis.spiders import RedisSpider
class MySpider(RedisSpider):
name = 'myspider'
redis_key = 'myspider:start_urls'
def parse(self, response):
title = response.xpath('//title/text()').get()
print(title)
八、数据清洗和预处理
爬取的数据通常需要进行清洗和预处理,以便后续分析和处理。
1. 去除HTML标签
使用BeautifulSoup去除HTML标签:
from bs4 import BeautifulSoup
html = '<p>Example <b>text</b></p>'
soup = BeautifulSoup(html, 'lxml')
text = soup.get_text()
print(text) # 输出:Example text
2. 数据格式化
使用正则表达式格式化数据:
import re
data = 'Price: $100.00'
formatted_data = re.sub(r'[^0-9.]', '', data)
print(formatted_data) # 输出:100.00
3. 处理缺失值
使用Pandas处理缺失值:
import pandas as pd
data = {'name': ['Alice', 'Bob', None], 'age': [25, None, 30]}
df = pd.DataFrame(data)
填充缺失值
df.fillna({'name': 'Unknown', 'age': 0}, inplace=True)
print(df)
九、数据分析和可视化
爬取的数据可以进行分析和可视化,获得有价值的洞见。
1. 数据分析
使用Pandas进行数据分析:
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
计算平均年龄
average_age = df['age'].mean()
print(average_age) # 输出:30.0
2. 数据可视化
使用Matplotlib进行数据可视化:
import matplotlib.pyplot as plt
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
绘制柱状图
plt.bar(df['name'], df['age'])
plt.xlabel('Name')
plt.ylabel('Age')
plt.title('Age of Individuals')
plt.show()
十、常见问题和解决方案
在爬取数据过程中,可能会遇到各种问题,以下是一些常见问题和解决方案:
1. 爬取速度慢
解决方案:
- 使用多线程或异步爬取,提高爬取效率。
- 使用分布式爬取,将任务分配到多个节点。
2. 反爬机制
解决方案:
- 使用代理IP,避免IP被封锁。
- 设置请求头,模拟浏览器请求。
- 使用Selenium模拟浏览器操作,处理动态内容和验证码。
3. 数据解析错误
解决方案:
- 使用正确的解析器,例如BeautifulSoup的lxml解析器。
- 检查HTML结构,确保XPath或CSS选择器正确。
十一、最佳实践
在实际项目中,遵循以下最佳实践,可以提高爬虫的稳定性和效率:
1. 遵守爬取规则
尊重网站的robots.txt文件,遵守爬取规则,避免对网站造成过大负担。
2. 设置合理的爬取间隔
设置合理的爬取间隔,避免频繁请求导致IP被封锁:
import time
time.sleep(1) # 每次请求后等待1秒
3. 处理异常
处理网络异常和请求失败,确保爬虫稳定运行:
import requests
from requests.exceptions import RequestException
try:
response = requests.get('http://example.com')
response.raise_for_status()
except RequestException as e:
print(f'Error: {e}')
4. 数据存储和备份
定期存储和备份爬取的数据,避免数据丢失。
十二、应用实例
以下是一个完整的应用实例,使用requests和BeautifulSoup爬取某电商网站的商品信息,并存储到CSV文件中:
import requests
from bs4 import BeautifulSoup
import csv
url = 'http://example.com/products'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
products = soup.find_all('div', class_='product')
data = [['Product Name', 'Price', 'Link']]
for product in products:
name = product.find('h2', class_='product-name').text
price = product.find('span', class_='price').text
link = product.find('a', class_='product-link')['href']
data.append([name, price, link])
with open('products.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
else:
print('Failed to retrieve the webpage')
总结
本文详细介绍了使用Python爬取网络数据的方法,包括requests、BeautifulSoup、Scrapy、Selenium等工具和库的使用,以及数据存储、处理反爬机制、多线程与分布式爬取、数据清洗和预处理、数据分析和可视化、常见问题和解决方案、最佳实践等方面的内容。希望本文能帮助你更好地理解和掌握Python爬虫技术,为实际项目提供有力支持。
相关问答FAQs:
如何选择合适的Python库来进行网络爬虫?
在进行网络爬虫时,选择合适的Python库至关重要。常用的库包括Requests和BeautifulSoup,它们分别用于发送HTTP请求和解析HTML文档。Requests库非常简单易用,适合初学者,而BeautifulSoup则能够帮助你快速提取所需数据。此外,Scrapy是一个更为强大的框架,适合处理复杂的爬虫任务。根据需求选择合适的工具,可以显著提高工作效率。
在爬取数据时需要注意哪些法律和道德问题?
进行网络爬虫时,了解并遵守相关法律法规非常重要。确保遵循网站的robots.txt文件中的爬虫规则,并避免对网站造成负担。此外,爬取敏感数据或个人信息可能涉及隐私问题,因此在设计爬虫时应尊重他人隐私及数据使用政策。确保你的行为符合道德标准,可以有效避免潜在的法律风险。
如何处理爬虫过程中遇到的反爬机制?
许多网站会采取反爬机制来阻止自动化程序的访问。应对这些机制可以采取多种策略,比如设置请求头伪装成浏览器,使用代理IP以更换访问地址,或者引入随机延时以模拟人类行为。了解并灵活运用这些策略,可以有效提高爬虫的成功率。不过,要注意合理使用,避免对目标网站造成负面影响。
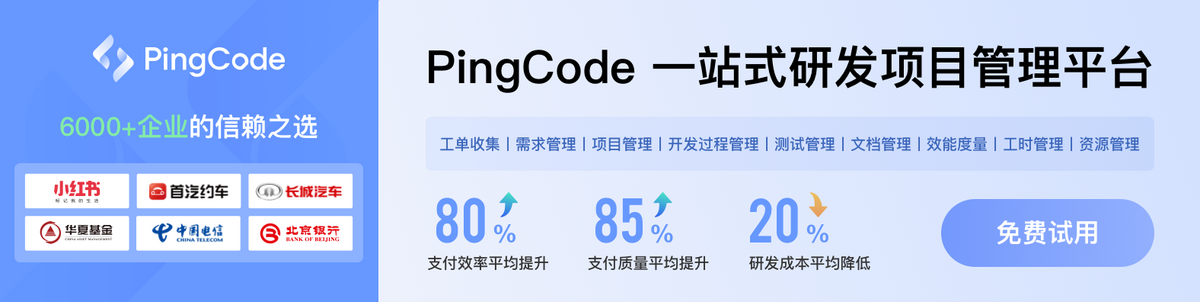