使用Python筛选新闻网页的方法包括:使用网络爬虫抓取网页内容、使用自然语言处理技术分析文本、使用正则表达式提取关键信息、使用机器学习分类器进行筛选。 其中,使用网络爬虫抓取网页内容是最基础的步骤,下面将详细介绍这一点。
网络爬虫是用来自动抓取网页内容的工具。Python中常用的网络爬虫库包括requests和BeautifulSoup。requests库用于发送HTTP请求获取网页内容,而BeautifulSoup库用于解析HTML文档并提取需要的数据。以下是一个简单的示例代码,展示如何使用requests和BeautifulSoup抓取网页内容:
import requests
from bs4 import BeautifulSoup
发送HTTP请求获取网页内容
url = 'https://example.com/news'
response = requests.get(url)
web_content = response.text
使用BeautifulSoup解析HTML文档
soup = BeautifulSoup(web_content, 'html.parser')
查找并提取新闻标题和链接
news_items = soup.find_all('h2', class_='news-title')
for item in news_items:
title = item.text
link = item.find('a')['href']
print(f'Title: {title}\nLink: {link}\n')
通过这段代码,我们可以抓取到网页中的新闻标题和链接。接下来我们将详细介绍使用Python筛选新闻网页的完整流程。
一、使用网络爬虫抓取网页内容
1、安装和导入必要的库
在开始编写代码之前,需要安装和导入必要的库。使用pip工具安装requests和BeautifulSoup库:
pip install requests beautifulsoup4
然后在Python脚本中导入这些库:
import requests
from bs4 import BeautifulSoup
2、发送HTTP请求获取网页内容
使用requests库发送HTTP请求获取网页内容。以下是一个示例代码:
url = 'https://example.com/news'
response = requests.get(url)
web_content = response.text
3、解析HTML文档
使用BeautifulSoup库解析HTML文档,并提取需要的数据。以下是一个示例代码:
soup = BeautifulSoup(web_content, 'html.parser')
news_items = soup.find_all('h2', class_='news-title')
4、提取新闻标题和链接
通过解析后的HTML文档,查找并提取新闻标题和链接。以下是一个示例代码:
for item in news_items:
title = item.text
link = item.find('a')['href']
print(f'Title: {title}\nLink: {link}\n')
二、使用自然语言处理技术分析文本
1、安装和导入必要的库
在开始编写代码之前,需要安装和导入必要的库。使用pip工具安装nltk库:
pip install nltk
然后在Python脚本中导入这些库:
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
2、文本预处理
对文本进行预处理,包括去除停用词、标点符号和特殊字符。以下是一个示例代码:
text = 'This is an example of news text.'
stop_words = set(stopwords.words('english'))
word_tokens = word_tokenize(text)
filtered_text = [word for word in word_tokens if word.lower() not in stop_words]
print(filtered_text)
3、关键词提取
使用TF-IDF算法提取文本中的关键词。以下是一个示例代码:
from sklearn.feature_extraction.text import TfidfVectorizer
documents = ['This is the first news text.', 'This is the second news text.']
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(documents)
feature_names = vectorizer.get_feature_names_out()
for doc_index, doc in enumerate(tfidf_matrix):
print(f'Document {doc_index + 1}:')
for term_index, term in enumerate(doc.toarray()[0]):
print(f'{feature_names[term_index]}: {term}')
三、使用正则表达式提取关键信息
1、安装和导入必要的库
在开始编写代码之前,需要导入re库:
import re
2、编写正则表达式
编写正则表达式,用于匹配新闻标题和链接。以下是一个示例代码:
pattern = re.compile(r'<h2 class="news-title"><a href="(.*?)">(.*?)</a></h2>')
matches = pattern.findall(web_content)
for match in matches:
link, title = match
print(f'Title: {title}\nLink: {link}\n')
3、提取关键信息
使用正则表达式匹配网页内容,并提取关键信息。以下是一个示例代码:
for match in matches:
link, title = match
print(f'Title: {title}\nLink: {link}\n')
四、使用机器学习分类器进行筛选
1、安装和导入必要的库
在开始编写代码之前,需要安装和导入必要的库。使用pip工具安装scikit-learn库:
pip install scikit-learn
然后在Python脚本中导入这些库:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
2、准备数据集
准备一个包含新闻文本和标签的数据集。以下是一个示例代码:
documents = ['This is the first news text.', 'This is the second news text.']
labels = [0, 1] # 0: Not relevant, 1: Relevant
3、特征提取
使用TF-IDF算法提取文本特征。以下是一个示例代码:
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(documents)
4、划分训练集和测试集
将数据集划分为训练集和测试集。以下是一个示例代码:
X_train, X_test, y_train, y_test = train_test_split(X, labels, test_size=0.2, random_state=42)
5、训练分类器
使用朴素贝叶斯分类器训练模型。以下是一个示例代码:
classifier = MultinomialNB()
classifier.fit(X_train, y_train)
6、评估模型
使用测试集评估模型的性能。以下是一个示例代码:
y_pred = classifier.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy * 100:.2f}%')
五、综合示例
综合以上步骤,编写一个完整的示例代码,用于抓取新闻网页、提取新闻标题和链接、分析文本、提取关键词、并使用机器学习分类器进行筛选。以下是一个示例代码:
import requests
from bs4 import BeautifulSoup
import re
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
发送HTTP请求获取网页内容
url = 'https://example.com/news'
response = requests.get(url)
web_content = response.text
使用BeautifulSoup解析HTML文档
soup = BeautifulSoup(web_content, 'html.parser')
news_items = soup.find_all('h2', class_='news-title')
提取新闻标题和链接
news_data = []
for item in news_items:
title = item.text
link = item.find('a')['href']
news_data.append({'title': title, 'link': link})
文本预处理
stop_words = set(stopwords.words('english'))
for news in news_data:
word_tokens = word_tokenize(news['title'])
filtered_text = [word for word in word_tokens if word.lower() not in stop_words]
news['filtered_title'] = ' '.join(filtered_text)
关键词提取
documents = [news['filtered_title'] for news in news_data]
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(documents)
feature_names = vectorizer.get_feature_names_out()
for doc_index, doc in enumerate(tfidf_matrix):
print(f'Document {doc_index + 1}:')
for term_index, term in enumerate(doc.toarray()[0]):
print(f'{feature_names[term_index]}: {term}')
准备数据集
labels = [1 if 'relevant keyword' in news['filtered_title'] else 0 for news in news_data]
划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(tfidf_matrix, labels, test_size=0.2, random_state=42)
训练分类器
classifier = MultinomialNB()
classifier.fit(X_train, y_train)
评估模型
y_pred = classifier.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy * 100:.2f}%')
通过以上步骤和示例代码,我们可以使用Python筛选新闻网页,包括抓取网页内容、分析文本、提取关键信息和使用机器学习分类器进行筛选。这些方法和技术可以帮助我们高效地处理和筛选大量的新闻网页内容。
相关问答FAQs:
如何使用Python自动抓取新闻网页上的信息?
使用Python抓取新闻网页信息通常依赖于库如Beautiful Soup和Requests。首先,使用Requests库发送HTTP请求获取网页内容,接着利用Beautiful Soup解析HTML文档。你可以提取特定的标签和类名,获取标题、发布时间、正文等信息,最终将其存储到CSV文件或数据库中以便后续分析。
在筛选新闻网页时,有哪些常用的库和工具推荐?
在进行新闻网页筛选时,常用的库包括Beautiful Soup(用于HTML解析)、Requests(用于发送网络请求)、Scrapy(一个强大的爬虫框架)和Pandas(用于数据处理与分析)。这些工具能够帮助你轻松获取和处理新闻数据,使得信息提取过程更加高效。
如何处理抓取到的新闻数据以便于分析?
抓取到的新闻数据可以使用Pandas库进行处理。你可以将数据转换为DataFrame格式,方便进行数据清洗、筛选和统计分析。常用的处理方法包括去除重复项、筛选特定时间段的新闻、按关键词过滤等。此外,数据可视化工具如Matplotlib或Seaborn可以帮助你更直观地展示分析结果。
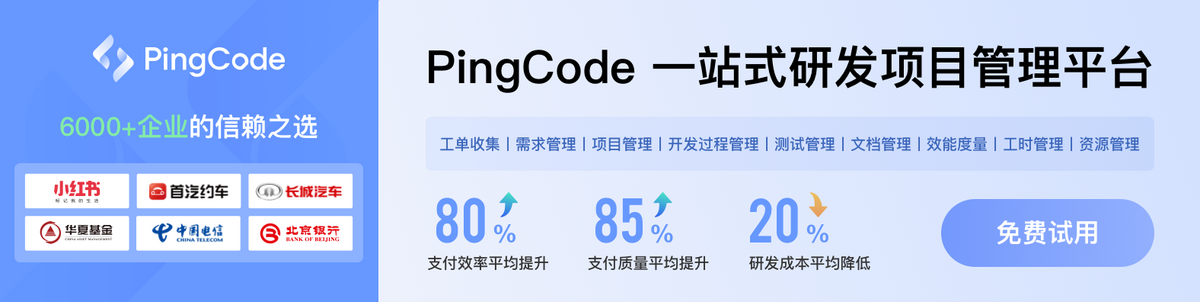