在Python中消除重复元素可以通过多种方法实现,主要包括使用集合、列表推导式、字典以及Pandas等方法。这些方法各有优缺点,适用于不同的场景。下面我将详细描述其中一种方法,即使用集合来消除重复元素。
使用集合来消除重复元素时,主要是利用集合的特性,即集合中的元素是唯一的,这样可以很方便地去除重复元素。具体的实现方法如下:
# 原始列表
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
使用集合消除重复元素
unique_elements = list(set(list_with_duplicates))
print(unique_elements)
输出: [1, 2, 3, 4, 5]
在这个方法中,我们将列表转换为集合,集合会自动去除重复元素,然后再将集合转换回列表。这种方法简单高效,适用于大多数场景。
接下来,我们将详细介绍其他几种常用方法,分别是列表推导式、字典以及Pandas,并探讨它们各自的优缺点和适用场景。
一、使用集合消除重复元素
集合(Set)是Python中的一种数据结构,它的特点是无序且不重复,因此非常适合用于去除重复元素。
1. 使用集合去重
如前所述,使用集合去重非常简单,只需将列表转换为集合,然后再转换回列表即可:
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
unique_elements = list(set(list_with_duplicates))
print(unique_elements)
优点:代码简洁,效率高。
缺点:不能保持原有的元素顺序。
2. 保持顺序的集合去重
如果需要保持原有的元素顺序,可以使用collections.OrderedDict
或dict.fromkeys
:
from collections import OrderedDict
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
unique_elements = list(OrderedDict.fromkeys(list_with_duplicates))
print(unique_elements)
或者使用dict.fromkeys
:
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
unique_elements = list(dict.fromkeys(list_with_duplicates))
print(unique_elements)
优点:可以保持原有的元素顺序。
缺点:代码相对复杂一些。
二、使用列表推导式消除重复元素
列表推导式是一种简洁的构造列表的方法,可以结合条件判断来去除重复元素。
1. 列表推导式去重
可以使用一个辅助列表来记录已经出现的元素:
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
seen = set()
unique_elements = [x for x in list_with_duplicates if x not in seen and not seen.add(x)]
print(unique_elements)
优点:代码简洁,可以保持原有的元素顺序。
缺点:需要额外的存储空间来记录已经出现的元素。
三、使用字典消除重复元素
Python 3.7以后,字典保持插入顺序,因此可以利用这一特性来去除重复元素。
1. 使用字典去重
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
unique_elements = list(dict.fromkeys(list_with_duplicates))
print(unique_elements)
优点:可以保持原有的元素顺序,代码简洁。
缺点:需要Python 3.7及以上版本。
四、使用Pandas消除重复元素
Pandas是一个强大的数据分析库,适用于处理复杂的数据结构和大规模数据集。如果数据存储在Pandas的DataFrame或Series中,可以使用Pandas提供的方法去除重复元素。
1. 使用Pandas去重
首先,需要安装Pandas库:
pip install pandas
然后,使用Pandas来去重:
import pandas as pd
使用Series去重
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
unique_elements = pd.Series(list_with_duplicates).drop_duplicates().tolist()
print(unique_elements)
使用DataFrame去重
df = pd.DataFrame({'col': [1, 2, 2, 3, 4, 4, 5]})
unique_elements = df['col'].drop_duplicates().tolist()
print(unique_elements)
优点:适用于复杂数据结构和大规模数据集。
缺点:需要依赖Pandas库,代码相对复杂。
五、其他方法
除了上述几种常用方法,还有一些其他方法可以用于去除重复元素,例如:
1. 使用循环去重
使用循环遍历列表,并将不重复的元素添加到新的列表中:
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
unique_elements = []
for elem in list_with_duplicates:
if elem not in unique_elements:
unique_elements.append(elem)
print(unique_elements)
优点:易于理解,可以保持原有的元素顺序。
缺点:效率较低,适用于小规模数据集。
2. 使用函数封装去重
可以将去重操作封装成一个函数,方便在不同场景中复用:
def remove_duplicates(input_list):
seen = set()
return [x for x in input_list if x not in seen and not seen.add(x)]
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
unique_elements = remove_duplicates(list_with_duplicates)
print(unique_elements)
优点:代码复用性强,可以保持原有的元素顺序。
缺点:需要额外的存储空间来记录已经出现的元素。
结论
通过本文的介绍,我们了解了多种在Python中消除重复元素的方法,包括使用集合、列表推导式、字典以及Pandas等。每种方法都有其优缺点和适用场景,可以根据具体需求选择合适的方法。
总的来说,使用集合去重是最简单高效的方法,适用于大多数场景,但如果需要保持原有的元素顺序,可以考虑使用字典或列表推导式。对于复杂的数据结构和大规模数据集,使用Pandas去重是一个不错的选择。希望本文能对你在处理重复元素时有所帮助。
相关问答FAQs:
如何在Python中有效地去除列表中的重复元素?
在Python中,有多种方法可以消除列表中的重复元素。最常用的方式是利用集合(set)来自动去重。将列表转换为集合后,再转换回列表即可。例如:
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(set(my_list))
这种方法简单且快速,但请注意,集合会打乱原有的元素顺序。如果需要保持顺序,可以使用列表推导式结合集合来实现。
使用哪些库可以帮助在Python中去重?
Python标准库中的collections
模块提供了OrderedDict
,可以用来保持元素的顺序并消除重复元素。通过将列表转换为OrderedDict
,可以轻松去重:
from collections import OrderedDict
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(OrderedDict.fromkeys(my_list))
这种方法既去除了重复元素,又保持了原有顺序。
在处理大型数据集时,有哪些优化去重的方法?
对于大型数据集,可以考虑使用pandas
库。pandas
提供了drop_duplicates()
函数,可以有效地处理重复数据。首先需要将列表转换为DataFrame
,然后使用该函数去重:
import pandas as pd
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = pd.Series(my_list).drop_duplicates().tolist()
这种方法在处理复杂数据时不仅高效,还能提供丰富的数据操作功能。
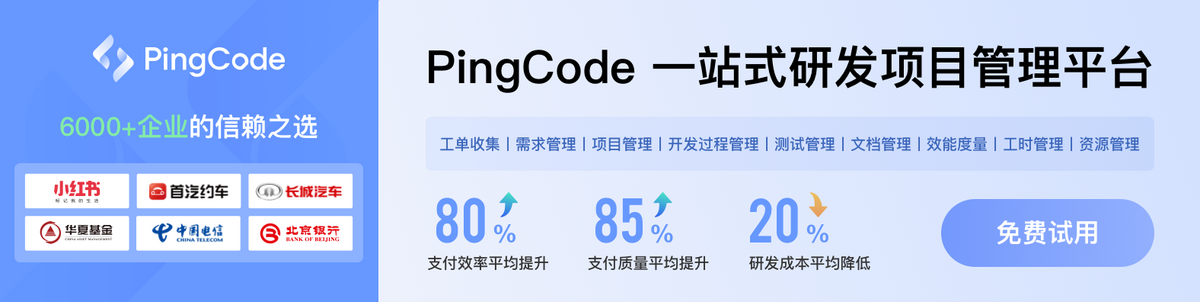