Python猜数字游戏的玩法包括:随机生成一个目标数字、用户输入猜测数字、提示用户大或小、重复猜测直到猜对。下面详细描述其中一个步骤,即随机生成一个目标数字。
在Python中,随机生成一个目标数字可以使用random
模块。首先需要导入random
模块,然后使用random.randint(a, b)
函数,该函数生成一个在a
和b
之间的随机整数(包括a
和b
)。例如,random.randint(1, 100)
会生成一个1到100之间的随机整数。这个数字将作为用户需要猜的目标数字。
下面,我将详细介绍如何创建一个完整的Python猜数字游戏,涵盖游戏的各个方面:
一、导入必要的模块
import random
random
模块在游戏中用于生成随机数,这个模块是Python标准库的一部分。
二、设置游戏参数
游戏需要设定一些基本参数,例如猜测的次数限制和数字的范围。
MAX_TRIES = 10
RANGE_START = 1
RANGE_END = 100
这些参数可以根据需求进行调整。
三、生成目标数字
使用random.randint
函数生成一个在指定范围内的随机整数。
target_number = random.randint(RANGE_START, RANGE_END)
四、主游戏循环
这个循环将控制游戏的主要逻辑,包括用户输入、判断和提示。
获取用户输入
使用input
函数获取用户的猜测数字,并确保输入的是一个有效的整数。
def get_user_guess():
while True:
try:
guess = int(input(f"Please enter your guess (between {RANGE_START} and {RANGE_END}): "))
if guess < RANGE_START or guess > RANGE_END:
raise ValueError(f"Number must be between {RANGE_START} and {RANGE_END}")
return guess
except ValueError as e:
print(e)
判断和提示
根据用户的猜测数字与目标数字的比较结果,提供相应的提示。
def give_feedback(guess, target):
if guess < target:
print("Your guess is too low.")
elif guess > target:
print("Your guess is too high.")
else:
print("Congratulations! You've guessed the right number!")
游戏主逻辑
将所有部分结合在一起,构建游戏的主循环。
def main():
target_number = random.randint(RANGE_START, RANGE_END)
print("Welcome to the Guess the Number Game!")
for attempt in range(1, MAX_TRIES + 1):
print(f"Attempt {attempt} of {MAX_TRIES}:")
guess = get_user_guess()
if guess == target_number:
print("Congratulations! You've guessed the right number!")
break
else:
give_feedback(guess, target_number)
else:
print(f"Sorry, you've used all {MAX_TRIES} attempts. The correct number was {target_number}.")
if __name__ == "__main__":
main()
五、改进游戏体验
添加难度选择
可以增加游戏的难度选择功能,让用户决定数字范围和猜测次数。
def select_difficulty():
print("Select difficulty level:")
print("1. Easy (1-50, 15 attempts)")
print("2. Medium (1-100, 10 attempts)")
print("3. Hard (1-200, 5 attempts)")
while True:
choice = input("Enter your choice (1, 2, or 3): ")
if choice == '1':
return 1, 50, 15
elif choice == '2':
return 1, 100, 10
elif choice == '3':
return 1, 200, 5
else:
print("Invalid choice. Please choose again.")
def main():
range_start, range_end, max_tries = select_difficulty()
target_number = random.randint(range_start, range_end)
print("Welcome to the Guess the Number Game!")
for attempt in range(1, max_tries + 1):
print(f"Attempt {attempt} of {max_tries}:")
guess = get_user_guess()
if guess == target_number:
print("Congratulations! You've guessed the right number!")
break
else:
give_feedback(guess, target_number)
else:
print(f"Sorry, you've used all {max_tries} attempts. The correct number was {target_number}.")
if __name__ == "__main__":
main()
添加游戏重玩功能
游戏结束后,可以询问用户是否想要再玩一次。
def main():
while True:
range_start, range_end, max_tries = select_difficulty()
target_number = random.randint(range_start, range_end)
print("Welcome to the Guess the Number Game!")
for attempt in range(1, max_tries + 1):
print(f"Attempt {attempt} of {max_tries}:")
guess = get_user_guess()
if guess == target_number:
print("Congratulations! You've guessed the right number!")
break
else:
give_feedback(guess, target_number)
else:
print(f"Sorry, you've used all {max_tries} attempts. The correct number was {target_number}.")
play_again = input("Would you like to play again? (yes/no): ").strip().lower()
if play_again != 'yes':
break
if __name__ == "__main__":
main()
六、总结
通过上述步骤,你可以创建一个功能完备的Python猜数字游戏。这个游戏包括生成随机数字、获取用户输入、判断和提示、以及提供重玩选项。通过添加难度选择和重玩功能,游戏的体验更加丰富和有趣。希望这些内容对你有所帮助,祝你编程愉快!
相关问答FAQs:
如何设置python猜数字游戏的范围?
在python猜数字游戏中,通常会设定一个数字范围,例如1到100。玩家需要在这个范围内猜测一个随机生成的数字。你可以通过修改代码中的范围参数来调整难度,比如选择1到50或1到1000。
游戏中如何提示玩家猜测的数字是过高还是过低?
在游戏进行中,程序会根据玩家输入的数字进行判断。如果玩家猜的数字高于目标数字,程序会提示“太高了”;如果猜的数字低于目标数字,程序会提示“太低了”。这种反馈机制帮助玩家缩小猜测范围,从而更快找到正确答案。
如何记录玩家的猜测次数以提高游戏的趣味性?
可以在代码中添加一个计数器变量,用于记录玩家的每一次猜测。在每次玩家输入猜测后,计数器加一,程序将显示当前的猜测次数。这不仅增加了游戏的挑战性,还能让玩家尝试在更少的猜测次数内找到正确的数字。
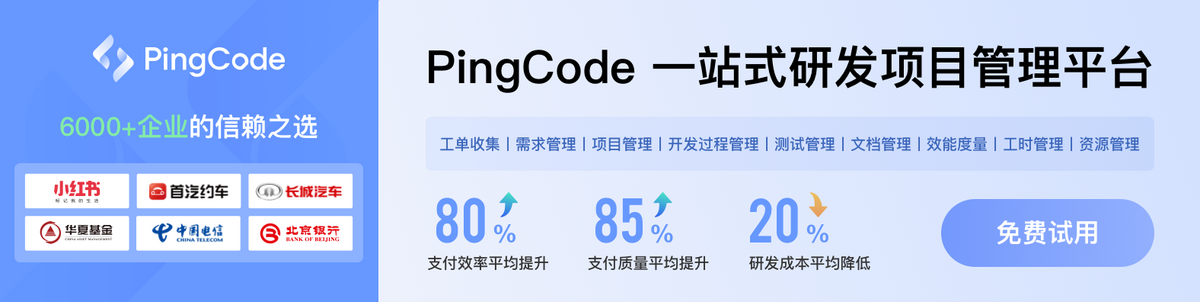