Python通过图像判断年龄的方法包括:深度学习模型、预处理数据、训练模型、测试与评估。其中,深度学习模型是最关键的一步,通过构建和训练一个深度学习模型,可以实现对图像中人物年龄的预测。接下来我们详细介绍这一点。
深度学习模型:在图像年龄预测中,常用的深度学习模型有卷积神经网络(CNN)以及一些预训练模型如ResNet、VGG等。这些模型能够从图像中提取特征,并通过训练数据学习如何将这些特征映射到具体的年龄值。需要有大量的标注数据进行训练,并且通过不断调整模型参数和训练策略来提升模型的准确性。
一、数据预处理
在进行图像年龄预测之前,数据预处理是非常关键的一步。它包括图像的读取、尺寸调整、归一化处理等。
图像读取
首先,需要读取图像数据。可以使用Python中的PIL库或OpenCV库来进行图像读取。PIL库是Python图像处理库,使用简单方便,而OpenCV则是一个功能强大的计算机视觉库,支持多种图像处理操作。
from PIL import Image
import cv2
import numpy as np
使用PIL库读取图像
image = Image.open('path_to_image.jpg')
使用OpenCV读取图像
image_cv = cv2.imread('path_to_image.jpg')
尺寸调整
为了适应深度学习模型的输入要求,通常需要将图像调整到固定的尺寸。例如,ResNet模型通常需要输入224×224的图像。
# 使用PIL库调整图像尺寸
image_resized = image.resize((224, 224))
使用OpenCV调整图像尺寸
image_resized_cv = cv2.resize(image_cv, (224, 224))
归一化处理
归一化处理是将图像像素值缩放到0到1之间,这样可以加速模型的训练过程,并提升模型的准确性。
# 将图像转换为numpy数组
image_array = np.array(image_resized) / 255.0
对于OpenCV读取的图像,同样需要转换为numpy数组并归一化
image_array_cv = image_resized_cv / 255.0
二、构建深度学习模型
构建深度学习模型是图像年龄预测的核心步骤。我们可以使用Keras或PyTorch等深度学习框架来构建模型。
使用Keras构建模型
Keras是一个高层神经网络API,易于使用,适合快速构建和训练模型。下面是一个简单的卷积神经网络模型示例。
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(224, 224, 3)),
MaxPooling2D(pool_size=(2, 2)),
Dropout(0.25),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Dropout(0.25),
Flatten(),
Dense(128, activation='relu'),
Dropout(0.5),
Dense(1, activation='linear')
])
model.compile(optimizer='adam', loss='mean_squared_error', metrics=['mae'])
model.summary()
使用预训练模型
预训练模型如ResNet、VGG等已经在大规模数据集上进行过训练,具有很强的特征提取能力。我们可以使用这些模型,并在其基础上进行微调(fine-tuning)。
from tensorflow.keras.applications import ResNet50
from tensorflow.keras.models import Model
from tensorflow.keras.layers import GlobalAveragePooling2D, Dense
base_model = ResNet50(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(128, activation='relu')(x)
predictions = Dense(1, activation='linear')(x)
model = Model(inputs=base_model.input, outputs=predictions)
for layer in base_model.layers:
layer.trainable = False
model.compile(optimizer='adam', loss='mean_squared_error', metrics=['mae'])
model.summary()
三、训练模型
在数据预处理和模型构建完成后,我们需要使用训练数据对模型进行训练。训练数据通常包括大量的带有年龄标签的图像。
数据准备
在进行训练之前,需要将训练数据转换为适合模型输入的格式。可以使用Keras的ImageDataGenerator来生成训练数据和验证数据。
from tensorflow.keras.preprocessing.image import ImageDataGenerator
train_datagen = ImageDataGenerator(rescale=1.0/255.0, validation_split=0.2)
train_generator = train_datagen.flow_from_directory(
'path_to_train_data',
target_size=(224, 224),
batch_size=32,
class_mode='sparse',
subset='training'
)
validation_generator = train_datagen.flow_from_directory(
'path_to_train_data',
target_size=(224, 224),
batch_size=32,
class_mode='sparse',
subset='validation'
)
训练过程
使用fit方法进行模型训练,并指定训练数据和验证数据。
history = model.fit(
train_generator,
epochs=50,
validation_data=validation_generator
)
四、模型测试与评估
在模型训练完成后,我们需要对模型进行测试和评估,以确定其在未见过的数据上的性能。
模型测试
可以使用单张图像进行测试,查看模型的预测结果。
test_image = Image.open('path_to_test_image.jpg')
test_image_resized = test_image.resize((224, 224))
test_image_array = np.array(test_image_resized) / 255.0
test_image_array = np.expand_dims(test_image_array, axis=0)
predicted_age = model.predict(test_image_array)
print(f'Predicted Age: {predicted_age[0][0]}')
模型评估
使用测试数据集对模型进行评估,查看模型的平均绝对误差(MAE)等指标。
test_datagen = ImageDataGenerator(rescale=1.0/255.0)
test_generator = test_datagen.flow_from_directory(
'path_to_test_data',
target_size=(224, 224),
batch_size=32,
class_mode='sparse'
)
test_loss, test_mae = model.evaluate(test_generator)
print(f'Test MAE: {test_mae}')
五、模型优化与改进
在模型测试与评估后,我们可以根据结果对模型进行优化与改进,以提升其预测精度。
调整模型结构
可以尝试调整模型的层数、神经元数量、激活函数等,以找到最优的模型结构。
model = Sequential([
Conv2D(64, (3, 3), activation='relu', input_shape=(224, 224, 3)),
MaxPooling2D(pool_size=(2, 2)),
Dropout(0.25),
Conv2D(128, (3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Dropout(0.25),
Flatten(),
Dense(256, activation='relu'),
Dropout(0.5),
Dense(1, activation='linear')
])
model.compile(optimizer='adam', loss='mean_squared_error', metrics=['mae'])
model.summary()
数据增强
数据增强可以增加训练数据的多样性,提升模型的泛化能力。可以使用Keras的ImageDataGenerator进行数据增强。
train_datagen = ImageDataGenerator(
rescale=1.0/255.0,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
validation_split=0.2
)
调整训练策略
可以尝试不同的优化器、学习率、批次大小等,找到最优的训练策略。
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=0.001), loss='mean_squared_error', metrics=['mae'])
使用迁移学习
迁移学习可以利用预训练模型的特征提取能力,提升模型的预测精度。可以尝试使用不同的预训练模型,并对其进行微调。
from tensorflow.keras.applications import InceptionV3
base_model = InceptionV3(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(128, activation='relu')(x)
predictions = Dense(1, activation='linear')(x)
model = Model(inputs=base_model.input, outputs=predictions)
for layer in base_model.layers:
layer.trainable = False
model.compile(optimizer='adam', loss='mean_squared_error', metrics=['mae'])
model.summary()
六、模型部署与应用
在模型优化与改进后,可以将模型部署到实际应用中,实现实时的图像年龄预测。
模型保存与加载
在完成训练后,可以将模型保存到文件中,以便后续加载和使用。
model.save('age_prediction_model.h5')
在需要使用时,可以加载模型。
from tensorflow.keras.models import load_model
model = load_model('age_prediction_model.h5')
部署到Web应用
可以将模型部署到Web应用中,实现在线的图像年龄预测。可以使用Flask或Django等Web框架。
from flask import Flask, request, jsonify
from tensorflow.keras.models import load_model
from PIL import Image
import numpy as np
app = Flask(__name__)
model = load_model('age_prediction_model.h5')
@app.route('/predict', methods=['POST'])
def predict():
file = request.files['image']
image = Image.open(file)
image_resized = image.resize((224, 224))
image_array = np.array(image_resized) / 255.0
image_array = np.expand_dims(image_array, axis=0)
predicted_age = model.predict(image_array)
return jsonify({'predicted_age': float(predicted_age[0][0])})
if __name__ == '__main__':
app.run(debug=True)
部署到移动应用
可以将模型转换为TensorFlow Lite格式,并部署到移动应用中,实现离线的图像年龄预测。
import tensorflow as tf
将模型转换为TensorFlow Lite格式
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
保存TensorFlow Lite模型
with open('age_prediction_model.tflite', 'wb') as f:
f.write(tflite_model)
七、总结
通过本文的介绍,我们了解了如何使用Python通过图像判断年龄的全过程。首先进行了数据预处理,包括图像读取、尺寸调整和归一化处理。然后构建了深度学习模型,可以选择使用Keras构建自己的模型,或者使用预训练模型。接下来进行了模型训练,通过准备训练数据并使用fit方法进行训练。然后进行了模型测试与评估,使用单张图像进行测试,并使用测试数据集进行评估。接着进行了模型优化与改进,包括调整模型结构、进行数据增强、调整训练策略和使用迁移学习。最后介绍了如何将模型部署到实际应用中,包括保存与加载模型,部署到Web应用和移动应用。
希望通过本文的介绍,能够帮助你更好地理解和实现图像年龄预测,并应用到实际项目中。
相关问答FAQs:
如何使用Python进行年龄预测的图像处理?
在Python中,使用深度学习库(如TensorFlow或PyTorch)结合预训练的卷积神经网络(CNN)模型,可以有效地从图像中提取特征,并进行年龄预测。首先,准备好年龄标注的数据集,然后通过数据预处理、模型训练和评估,最终实现对新图像的年龄判断。
需要哪些库和工具来实现图像年龄判断?
实现图像年龄判断通常需要一些关键的Python库,例如OpenCV用于图像处理,NumPy用于数值计算,Pandas用于数据处理,以及Keras或PyTorch用于构建和训练深度学习模型。此外,Matplotlib可以用来可视化结果,帮助分析模型的表现。
如何提高图像中年龄预测的准确性?
提高年龄预测的准确性可以通过多种方式实现。首先,使用高质量和多样化的数据集进行训练;其次,调整模型的超参数,例如学习率和批量大小;此外,可以尝试数据增强技术,通过旋转、缩放、裁剪等方式增加训练数据的多样性。最后,选择合适的损失函数和优化器也是提升准确性的关键因素。
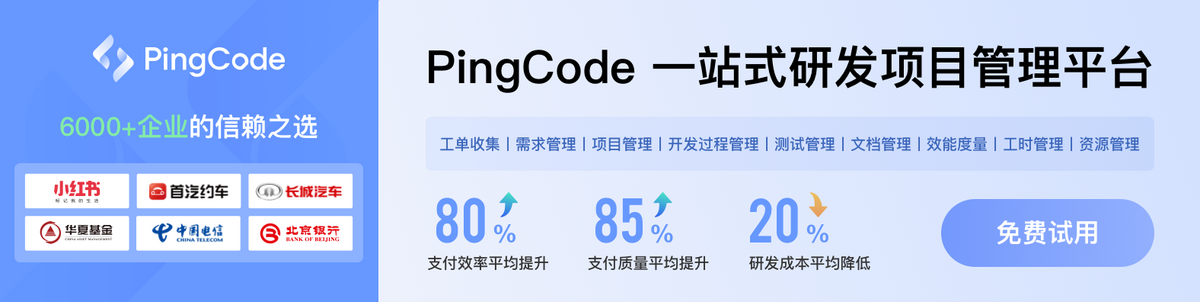