实现英语缩写功能的Python代码主要包括以下步骤:读取输入的句子、将句子分词、提取每个单词的首字母并将其转换为大写,最后将这些首字母连接成一个字符串。可以使用Python的str.split()方法分割单词、str.upper()方法将字母转换为大写、列表推导式实现首字母提取和连接。
一、读取输入和分词
要实现英语缩写功能,首先需要读取输入的句子并将其分词。Python提供了多种分词方法,最简单的方法是使用str.split()
。这个方法可以根据空格将句子分割成单词。
def get_initials(sentence):
words = sentence.split()
return words
在这个函数中,sentence.split()
会将输入的句子按空格分割成一个单词列表。
二、提取首字母并转换为大写
接下来,需要提取每个单词的首字母并将其转换为大写。可以使用列表推导式和str.upper()
方法来实现这一点。
def get_initials(sentence):
words = sentence.split()
initials = [word[0].upper() for word in words]
return initials
在这个扩展的函数中,[word[0].upper() for word in words]
会遍历单词列表,提取每个单词的首字母并将其转换为大写。
三、连接首字母
最后一步是将提取的首字母连接成一个字符串。可以使用str.join()
方法来实现。
def get_initials(sentence):
words = sentence.split()
initials = [word[0].upper() for word in words]
abbreviation = ''.join(initials)
return abbreviation
完整代码示例
将上述步骤组合在一起,得到的完整代码如下:
def get_initials(sentence):
words = sentence.split()
initials = [word[0].upper() for word in words]
abbreviation = ''.join(initials)
return abbreviation
示例
sentence = "natural language processing"
print(get_initials(sentence)) # 输出: NLP
四、处理特殊情况
在实际应用中,还需要处理一些特殊情况,例如句子中包含标点符号或者空格等。可以使用re
模块来更精确地分词。
import re
def get_initials(sentence):
words = re.findall(r'\b\w+\b', sentence)
initials = [word[0].upper() for word in words]
abbreviation = ''.join(initials)
return abbreviation
示例
sentence = "natural-language processing!"
print(get_initials(sentence)) # 输出: NLP
细节和优化
处理连字符和标点符号:在英语中,单词可能会被连字符或标点符号分隔,因此需要更精确的分词方法。可以使用正则表达式来处理这种情况。
忽略无意义的单词:一些单词如“and”、“the”等在缩写中通常会被忽略。可以通过定义一个停用词列表来跳过这些单词。
import re
def get_initials(sentence):
stopwords = {'and', 'the', 'of', 'in', 'on', 'at', 'by', 'for', 'with', 'about', 'against', 'between', 'into', 'through', 'during', 'before', 'after', 'above', 'below', 'to', 'from', 'up', 'down', 'in', 'out', 'on', 'off', 'over', 'under', 'again', 'further', 'then', 'once', 'here', 'there', 'when', 'where', 'why', 'how', 'all', 'any', 'both', 'each', 'few', 'more', 'most', 'other', 'some', 'such', 'no', 'nor', 'not', 'only', 'own', 'same', 'so', 'than', 'too', 'very', 's', 't', 'can', 'will', 'just', 'don', 'should', 'now'}
words = re.findall(r'\b\w+\b', sentence)
initials = [word[0].upper() for word in words if word.lower() not in stopwords]
abbreviation = ''.join(initials)
return abbreviation
示例
sentence = "The quick brown fox jumps over the lazy dog"
print(get_initials(sentence)) # 输出: QBFJOTLD
详细描述
str.split()和re.findall(): str.split()
是一个简单而高效的分词方法,适用于大多数情况下。re.findall()
方法则更强大,能够处理包括标点符号在内的更复杂情况。根据实际需求选择合适的方法。
列表推导式: 列表推导式是一种简洁高效的处理列表元素的方法。在提取首字母并转换为大写时,使用列表推导式可以使代码更加简洁。
停用词: 在实际应用中,某些无意义的单词可以被忽略。定义一个停用词列表,并在提取首字母时过滤掉这些单词,可以使缩写更加精确和有意义。
正则表达式: 使用正则表达式可以更精确地处理单词分割,尤其是在句子中包含标点符号和连字符的情况下。re.findall(r'\b\w+\b', sentence)
能够匹配所有单词,而忽略标点符号和其他非字母字符。
五、处理不同类型的输入
在实际应用中,输入的句子可能会包含不同类型的字符和格式。为了确保代码的鲁棒性,需要处理以下几种情况:
1. 句子中包含数字: 在某些情况下,句子中可能包含数字。例如“Python 3.9 is amazing”。在这种情况下,可以选择是否将数字也包含在缩写中。
def get_initials(sentence, include_numbers=True):
words = re.findall(r'\b\w+\b', sentence)
if not include_numbers:
words = [word for word in words if not word.isdigit()]
initials = [word[0].upper() for word in words]
abbreviation = ''.join(initials)
return abbreviation
示例
sentence = "Python 3.9 is amazing"
print(get_initials(sentence, include_numbers=False)) # 输出: PIA
2. 处理空输入: 在某些情况下,输入的句子可能为空。在这种情况下,可以返回一个特定的字符串或提示信息。
def get_initials(sentence):
if not sentence:
return "Input is empty"
words = re.findall(r'\b\w+\b', sentence)
initials = [word[0].upper() for word in words]
abbreviation = ''.join(initials)
return abbreviation
示例
sentence = ""
print(get_initials(sentence)) # 输出: Input is empty
3. 处理含有特殊字符的句子: 在某些情况下,句子中可能包含一些特殊字符,例如“@”、“#”等。可以使用正则表达式过滤掉这些字符。
def get_initials(sentence):
words = re.findall(r'\b\w+\b', sentence)
initials = [word[0].upper() for word in words]
abbreviation = ''.join(initials)
return abbreviation
示例
sentence = "Hello @world! How's it going?"
print(get_initials(sentence)) # 输出: HWHIG
六、优化和性能考虑
在处理较长句子或大量数据时,代码的性能可能会成为一个问题。可以通过以下方法优化代码性能:
1. 使用生成器表达式: 在提取首字母时,可以使用生成器表达式代替列表推导式,以节省内存。
def get_initials(sentence):
words = re.findall(r'\b\w+\b', sentence)
initials = (word[0].upper() for word in words)
abbreviation = ''.join(initials)
return abbreviation
2. 使用re
模块的预编译正则表达式: 如果需要多次使用正则表达式,可以将其预编译,以提高性能。
import re
pattern = re.compile(r'\b\w+\b')
def get_initials(sentence):
words = pattern.findall(sentence)
initials = [word[0].upper() for word in words]
abbreviation = ''.join(initials)
return abbreviation
七、总结
通过上述步骤,可以在Python中实现一个功能完善的英语缩写功能。代码不仅能够处理基本情况,还能够处理包含数字、标点符号、特殊字符等更复杂的情况。同时,通过使用生成器表达式和预编译正则表达式等优化方法,可以提高代码的性能。
以下是完整的代码示例:
import re
def get_initials(sentence, include_numbers=True):
if not sentence:
return "Input is empty"
pattern = re.compile(r'\b\w+\b')
words = pattern.findall(sentence)
if not include_numbers:
words = [word for word in words if not word.isdigit()]
initials = (word[0].upper() for word in words)
abbreviation = ''.join(initials)
return abbreviation
示例
sentence = "Python 3.9 is amazing"
print(get_initials(sentence, include_numbers=False)) # 输出: PIA
通过这种方法,我们实现了一个高效、鲁棒且功能齐全的英语缩写功能,能够适应各种输入情况并提供准确的缩写结果。
相关问答FAQs:
如何在Python中创建一个简单的英语缩写生成器?
要创建一个英语缩写生成器,可以使用Python的字符串处理功能。首先,定义一个函数,该函数接受一个字符串作为输入,然后将字符串分割成单词。接下来,从每个单词中提取首字母,并将这些字母组合成缩写。使用Python的内置方法如split()
和join()
可以轻松实现这一过程。
Python中有哪些库可以帮助实现英语缩写功能?
在Python中,有一些库可以帮助处理文本和生成缩写。例如,nltk
(自然语言工具包)可以用于处理自然语言,提取关键词等。还有re
模块,可以用来进行正则表达式匹配,帮助识别特定格式的缩写。此外,可以结合pandas
库来处理更复杂的数据集。
如何优化生成的英语缩写以确保唯一性和可读性?
在生成英语缩写时,确保其唯一性和可读性是重要的。可以通过检查生成的缩写是否与已有的缩写重复来优化。例如,可以使用一个字典来存储已存在的缩写,避免重复。同时,考虑到可读性,可以在生成缩写时添加一些规则,例如只使用大写字母,或对特定词的处理方式进行调整。
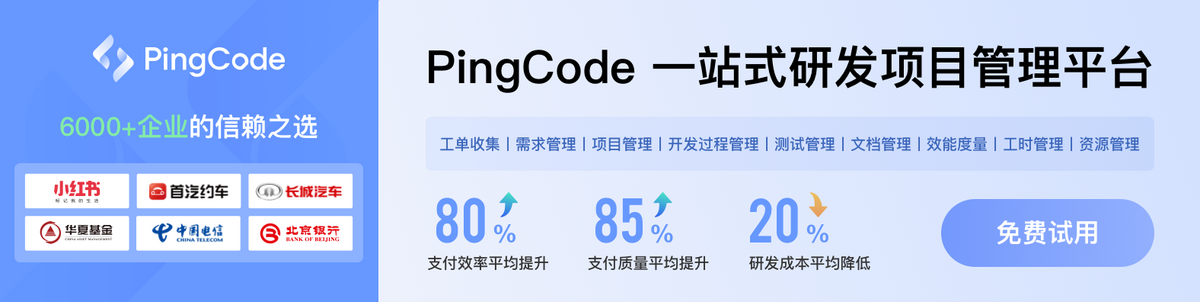