在Python中训练语料库可以通过多种方法实现,使用NLP库(如NLTK、spaCy)、数据预处理技术、机器学习算法(如TF-IDF、Word2Vec),以及深度学习模型(如LSTM、Transformer)等。以下将详细描述如何使用这些方法来训练语料库。
一、NLP库的使用
1、NLTK
NLTK(Natural Language Toolkit)是Python最流行的自然语言处理工具包之一,提供了丰富的语料库和工具,适用于文本处理和分析。
安装NLTK
pip install nltk
导入必要模块并下载语料库
import nltk
nltk.download('punkt') # Tokenizer
nltk.download('averaged_perceptron_tagger') # POS Tagging
nltk.download('stopwords') # Stopwords
示例:文本分词、词性标注和去除停用词
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
from nltk import pos_tag
text = "NLTK is a leading platform for building Python programs to work with human language data."
分词
tokens = word_tokenize(text)
print("Tokens:", tokens)
词性标注
tagged = pos_tag(tokens)
print("POS Tags:", tagged)
去除停用词
stop_words = set(stopwords.words('english'))
filtered_tokens = [word for word in tokens if word.lower() not in stop_words]
print("Filtered Tokens:", filtered_tokens)
2、spaCy
spaCy是一个用于高级自然语言处理的库,具有极高的性能和灵活性,适用于信息提取、自然语言理解等任务。
安装spaCy
pip install spacy
python -m spacy download en_core_web_sm
导入spaCy并进行基本处理
import spacy
nlp = spacy.load("en_core_web_sm")
text = "spaCy is an open-source software library for advanced natural language processing in Python."
解析文本
doc = nlp(text)
分词、词性标注和命名实体识别
for token in doc:
print(token.text, token.pos_, token.lemma_)
for ent in doc.ents:
print(ent.text, ent.label_)
二、数据预处理技术
1、文本清理
文本清理是自然语言处理中的重要步骤,涉及去除标点符号、转换为小写、去除特殊字符等。
示例:文本清理
import re
text = "Hello World! Welcome to the realm of Natural Language Processing. Let's clean this text."
转换为小写
text = text.lower()
去除标点符号
text = re.sub(r'[^\w\s]', '', text)
去除多余空格
text = re.sub(r'\s+', ' ', text).strip()
print("Cleaned Text:", text)
2、词干提取和词形还原
词干提取和词形还原是将单词还原为其基本形式的技术,有助于减少词汇量,提升模型性能。
示例:词干提取和词形还原
from nltk.stem import PorterStemmer, WordNetLemmatizer
from nltk.corpus import wordnet
词干提取
stemmer = PorterStemmer()
stemmed_words = [stemmer.stem(word) for word in word_tokenize(text)]
print("Stemmed Words:", stemmed_words)
词形还原
lemmatizer = WordNetLemmatizer()
lemmatized_words = [lemmatizer.lemmatize(word, pos=wordnet.VERB) for word in word_tokenize(text)]
print("Lemmatized Words:", lemmatized_words)
三、机器学习算法
1、TF-IDF
TF-IDF(Term Frequency-Inverse Document Frequency)是一种用于文本挖掘的统计方法,评估词语在文档集中的重要性。
示例:TF-IDF
from sklearn.feature_extraction.text import TfidfVectorizer
corpus = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?"
]
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(corpus)
print("TF-IDF Matrix:\n", X.toarray())
2、Word2Vec
Word2Vec是一种将词语转换为向量的技术,能够捕捉词语间的语义关系。
安装gensim
pip install gensim
示例:Word2Vec
from gensim.models import Word2Vec
from nltk.tokenize import sent_tokenize, word_tokenize
text = "Word2Vec is a group of related models that are used to produce word embeddings. These models are shallow, two-layer neural networks that are trained to reconstruct linguistic contexts of words."
分句和分词
sentences = [word_tokenize(sent) for sent in sent_tokenize(text)]
训练Word2Vec模型
model = Word2Vec(sentences, vector_size=100, window=5, min_count=1, workers=4)
查看词语的向量表示
print("Word Vector:", model.wv['Word2Vec'])
四、深度学习模型
1、LSTM
LSTM(长短期记忆网络)是一种特殊的RNN,适用于处理和预测时间序列中间隔和延迟相对较长的重要事件。
安装TensorFlow
pip install tensorflow
示例:LSTM
import tensorflow as tf
from tensorflow.keras.preprocessing.text import Tokenizer
from tensorflow.keras.preprocessing.sequence import pad_sequences
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Embedding, LSTM, Dense
数据预处理
sentences = [
"I love programming.",
"Python is my favorite language.",
"Deep learning models are powerful."
]
tokenizer = Tokenizer()
tokenizer.fit_on_texts(sentences)
sequences = tokenizer.texts_to_sequences(sentences)
padded_sequences = pad_sequences(sequences, padding='post')
构建LSTM模型
model = Sequential([
Embedding(input_dim=50, output_dim=16, input_length=10),
LSTM(32),
Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
model.summary()
2、Transformer
Transformer是一种基于注意力机制的模型,极大地提升了自然语言处理任务的性能。
安装transformers库
pip install transformers
示例:Transformer
from transformers import BertTokenizer, TFBertForSequenceClassification
import tensorflow as tf
加载预训练的BERT模型和分词器
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = TFBertForSequenceClassification.from_pretrained('bert-base-uncased')
数据预处理
inputs = tokenizer("I love programming with Python.", return_tensors="tf")
labels = tf.constant([1]) # 假设标签为1
训练模型
with tf.GradientTape() as tape:
outputs = model(inputs, labels=labels)
loss = outputs.loss
logits = outputs.logits
gradients = tape.gradient(loss, model.trainable_variables)
optimizer = tf.keras.optimizers.Adam(learning_rate=5e-5)
optimizer.apply_gradients(zip(gradients, model.trainable_variables))
print("Loss:", loss.numpy())
五、总结
训练语料库是自然语言处理中的关键步骤,通过使用NLP库(如NLTK、spaCy)、数据预处理技术、机器学习算法(如TF-IDF、Word2Vec),以及深度学习模型(如LSTM、Transformer),可以有效地处理和分析文本数据。每种方法都有其独特的优点和适用场景,可以根据具体需求选择合适的技术。希望本文能够帮助你更好地理解如何在Python中训练语料库,并应用到实际项目中。
相关问答FAQs:
如何选择合适的语料库进行训练?
在训练语料库时,选择合适的数据集至关重要。应根据你的项目需求来挑选语料库。例如,如果你在处理自然语言处理任务,像维基百科或新闻文章这样的通用语料库可能适合。而如果你的目标是特定领域(如医学或法律),则应考虑使用专业的语料库。这将确保模型在特定领域的表现更佳。
有什么方法可以提高训练过程的效率?
提高训练效率可以通过多个方式实现。使用更高效的算法和框架(如TensorFlow或PyTorch)是一个选择。此外,可以使用GPU加速训练,利用批量训练和数据增强技术。此外,适当的超参数调整和选择合适的模型架构也能显著缩短训练时间。
如何评估训练后的语料库效果?
评估训练后的模型效果可以通过多种指标实现。常见的评估指标包括准确率、召回率、F1分数和混淆矩阵等。还可以通过交叉验证和留出法来确保模型的泛化能力。此外,使用可视化工具(如TensorBoard)来监控训练过程中的损失和准确率变化,也能帮助理解模型的表现。
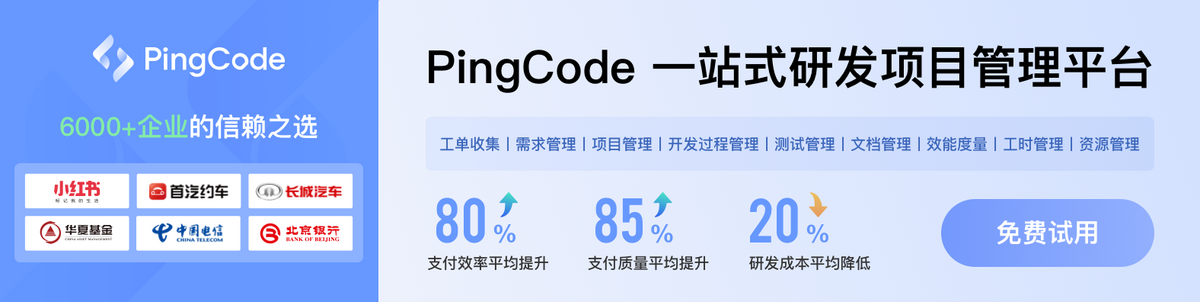