Python判断文件类型的方法有多种:通过文件扩展名判断、使用MIME类型库、通过二进制数据头部信息判断。 其中,通过文件扩展名判断的方法最为简单,但不够准确。使用MIME类型库是比较常见和可靠的方法,可以通过库获取文件的MIME类型。通过二进制数据头部信息判断的方法最为复杂,但精确度较高,适用于对文件类型判断有较高要求的场合。
下面将详细介绍使用MIME类型库的方法:
使用Python的mimetypes
库可以轻松判断文件类型。首先,需要导入mimetypes
库,然后使用mimetypes.guess_type
函数来获取文件的MIME类型。MIME类型是用于表示文件类型的一种互联网标准,可以非常准确地判断文件的类型。下面是一个示例代码:
import mimetypes
def get_file_type(file_path):
mime_type, encoding = mimetypes.guess_type(file_path)
if mime_type:
return mime_type
else:
return 'Unknown'
file_path = 'example.txt'
file_type = get_file_type(file_path)
print(f'The MIME type of the file is: {file_type}')
在这个例子中,我们定义了一个函数get_file_type
,该函数接收一个文件路径作为参数,并返回文件的MIME类型。如果无法判断文件类型,则返回'Unknown'。通过这种方法,我们可以轻松判断文件类型并进行相应的处理。
一、通过文件扩展名判断
通过文件扩展名判断文件类型的方法非常简单,只需要提取文件名中的扩展名即可。但这种方法的准确性较低,因为文件扩展名可能被随意更改。
import os
def get_file_extension(file_path):
_, file_extension = os.path.splitext(file_path)
return file_extension
file_path = 'example.txt'
file_extension = get_file_extension(file_path)
print(f'The file extension is: {file_extension}')
在这个示例中,使用os.path.splitext
函数获取文件的扩展名。虽然这种方法非常简单,但是只能作为辅助判断手段。
二、使用MIME类型库
1. mimetypes
库
mimetypes
库是Python标准库的一部分,可以用来判断文件的MIME类型。mimetypes.guess_type
函数可以根据文件名或URL来猜测文件类型。
import mimetypes
def get_file_type(file_path):
mime_type, encoding = mimetypes.guess_type(file_path)
return mime_type
file_path = 'example.pdf'
file_type = get_file_type(file_path)
print(f'The MIME type of the file is: {file_type}')
2. python-magic
库
python-magic
库是一个更强大的工具,可以读取文件的内容来判断文件类型。它支持更多的文件类型,适用于对判断准确性要求较高的场合。
import magic
def get_file_type(file_path):
mime = magic.Magic(mime=True)
mime_type = mime.from_file(file_path)
return mime_type
file_path = 'example.pdf'
file_type = get_file_type(file_path)
print(f'The MIME type of the file is: {file_type}')
在这个示例中,使用python-magic
库中的Magic
类来获取文件的MIME类型。需要注意的是,这个库可能需要安装额外的依赖项。
三、通过二进制数据头部信息判断
通过二进制数据头部信息判断文件类型的方法可以获得较高的准确性。许多文件格式都有特定的二进制头部信息(magic number),可以通过读取文件的前几个字节来判断文件类型。
def get_file_type(file_path):
with open(file_path, 'rb') as file:
header = file.read(4)
if header.startswith(b'\x25\x50\x44\x46'):
return 'application/pdf'
elif header.startswith(b'\x50\x4B\x03\x04'):
return 'application/zip'
else:
return 'Unknown'
file_path = 'example.pdf'
file_type = get_file_type(file_path)
print(f'The MIME type of the file is: {file_type}')
在这个示例中,通过读取文件的前4个字节来判断文件类型。虽然这种方法的准确性较高,但需要对各种文件格式的二进制头部信息有一定了解。
四、结合多种方法
在实际应用中,为了提高判断文件类型的准确性,可以结合多种方法。例如,可以先通过文件扩展名进行初步判断,然后再通过MIME类型库或二进制数据头部信息进行进一步确认。
import os
import mimetypes
import magic
def get_file_extension(file_path):
_, file_extension = os.path.splitext(file_path)
return file_extension
def get_mime_type(file_path):
mime_type, encoding = mimetypes.guess_type(file_path)
return mime_type
def get_magic_type(file_path):
mime = magic.Magic(mime=True)
mime_type = mime.from_file(file_path)
return mime_type
def get_file_type(file_path):
file_extension = get_file_extension(file_path)
mime_type = get_mime_type(file_path)
magic_type = get_magic_type(file_path)
if mime_type == magic_type:
return mime_type
else:
return 'Inconclusive'
file_path = 'example.pdf'
file_type = get_file_type(file_path)
print(f'The MIME type of the file is: {file_type}')
在这个示例中,我们结合了文件扩展名、mimetypes
库和python-magic
库来判断文件类型。如果mimetypes
库和python-magic
库判断的结果一致,则返回该MIME类型,否则返回'Inconclusive'。
五、实际应用案例
1. 文件上传系统
在文件上传系统中,判断文件类型是非常重要的一步,可以防止恶意文件的上传。可以结合前面介绍的方法,对上传的文件进行类型判断,并根据判断结果决定是否接收文件。
from flask import Flask, request
import os
import mimetypes
import magic
app = Flask(__name__)
def get_file_extension(file_path):
_, file_extension = os.path.splitext(file_path)
return file_extension
def get_mime_type(file_path):
mime_type, encoding = mimetypes.guess_type(file_path)
return mime_type
def get_magic_type(file_path):
mime = magic.Magic(mime=True)
mime_type = mime.from_file(file_path)
return mime_type
def get_file_type(file_path):
file_extension = get_file_extension(file_path)
mime_type = get_mime_type(file_path)
magic_type = get_magic_type(file_path)
if mime_type == magic_type:
return mime_type
else:
return 'Inconclusive'
@app.route('/upload', methods=['POST'])
def upload_file():
file = request.files['file']
file_path = os.path.join('/tmp', file.filename)
file.save(file_path)
file_type = get_file_type(file_path)
if file_type == 'application/pdf':
return 'File uploaded successfully'
else:
return 'Invalid file type', 400
if __name__ == '__main__':
app.run(debug=True)
在这个示例中,我们使用Flask框架实现了一个简单的文件上传系统。在上传文件后,通过结合文件扩展名、mimetypes
库和python-magic
库判断文件类型。如果文件类型为application/pdf
,则接收文件,否则返回错误信息。
2. 文件管理系统
在文件管理系统中,判断文件类型可以帮助我们对文件进行分类和处理。例如,可以根据文件类型将文件存储在不同的目录中,或者对不同类型的文件执行不同的操作。
import os
import mimetypes
import magic
import shutil
def get_file_extension(file_path):
_, file_extension = os.path.splitext(file_path)
return file_extension
def get_mime_type(file_path):
mime_type, encoding = mimetypes.guess_type(file_path)
return mime_type
def get_magic_type(file_path):
mime = magic.Magic(mime=True)
mime_type = mime.from_file(file_path)
return mime_type
def get_file_type(file_path):
file_extension = get_file_extension(file_path)
mime_type = get_mime_type(file_path)
magic_type = get_magic_type(file_path)
if mime_type == magic_type:
return mime_type
else:
return 'Inconclusive'
def organize_files(source_dir, target_dir):
for root, dirs, files in os.walk(source_dir):
for file in files:
file_path = os.path.join(root, file)
file_type = get_file_type(file_path)
if file_type.startswith('image'):
target_path = os.path.join(target_dir, 'images', file)
elif file_type.startswith('video'):
target_path = os.path.join(target_dir, 'videos', file)
elif file_type.startswith('application/pdf'):
target_path = os.path.join(target_dir, 'documents', file)
else:
target_path = os.path.join(target_dir, 'others', file)
os.makedirs(os.path.dirname(target_path), exist_ok=True)
shutil.move(file_path, target_path)
source_dir = '/path/to/source'
target_dir = '/path/to/target'
organize_files(source_dir, target_dir)
在这个示例中,我们实现了一个简单的文件管理系统,将源目录中的文件根据文件类型分类存储到目标目录中。通过这种方式,可以方便地对不同类型的文件进行管理和处理。
总结
通过本文的介绍,我们了解了Python判断文件类型的多种方法,包括通过文件扩展名判断、使用MIME类型库、通过二进制数据头部信息判断等。结合这些方法,可以在实际应用中准确地判断文件类型,提高系统的安全性和稳定性。
在文件上传系统和文件管理系统中,判断文件类型是非常重要的一步。通过合理地结合多种判断方法,可以提高文件类型判断的准确性和可靠性,确保系统的安全性和稳定性。希望本文的内容能够对大家在实际应用中有所帮助。
相关问答FAQs:
如何在Python中获取文件的扩展名?
在Python中,可以使用os.path
模块的splitext
函数来获取文件的扩展名。只需提供文件路径,函数将返回文件名和扩展名的元组。例如:
import os
file_path = 'example.txt'
file_name, file_extension = os.path.splitext(file_path)
print(f'文件扩展名: {file_extension}')
如何判断文件内容类型而不仅仅是扩展名?
为了判断文件的实际内容类型,可以使用mimetypes
模块或magic
库。mimetypes
模块可以根据文件扩展名返回MIME类型,而magic
库则可以通过读取文件内容来确定类型。使用magic
库的示例:
import magic
file_path = 'example.pdf'
file_type = magic.from_file(file_path, mime=True)
print(f'文件内容类型: {file_type}')
在Python中如何处理不同类型的文件?
处理不同类型的文件需要根据文件的实际类型采取不同的操作。可以使用上述的方式来判断文件类型后,结合相应的库进行处理。例如,使用PIL
库处理图像文件,使用pandas
读取CSV文件。根据文件类型选择合适的库和方法,可以提高代码的灵活性和可维护性。
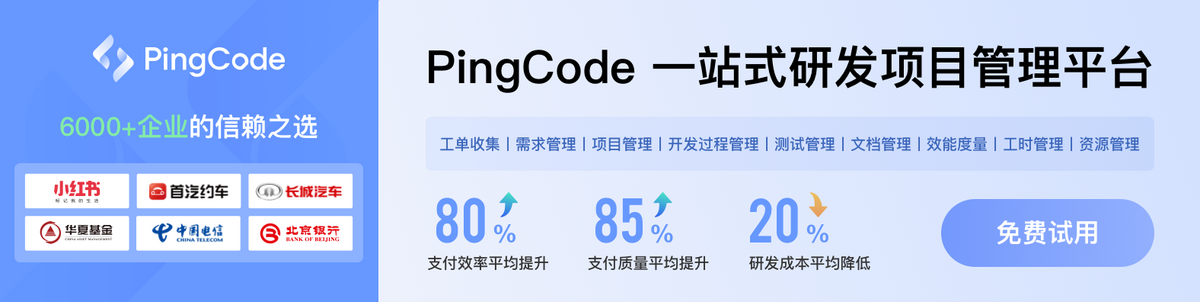