在Python中书写配置文件有多种方式,常见的有使用配置文件模块、使用JSON格式、使用YAML格式、使用环境变量。其中,使用配置文件模块是最常见且灵活的一种方式。下面将详细描述如何使用配置文件模块来书写和读取配置文件。
一、使用配置文件模块
Python提供了一个内置的配置文件模块configparser
,专门用于处理配置文件。
1. 创建配置文件
首先,我们需要创建一个配置文件,例如config.ini
:
[DEFAULT]
ServerAliveInterval = 45
Compression = yes
CompressionLevel = 9
ForwardX11 = yes
[bitbucket.org]
User = hg
[topsecret.server.com]
Host Port = 50022
ForwardX11 = no
2. 读取配置文件
接下来,我们使用configparser
模块来读取配置文件:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
读取默认值
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
print(server_alive_interval)
读取特定部分的值
user = config['bitbucket.org']['User']
print(user)
host_port = config['topsecret.server.com']['Host Port']
print(host_port)
3. 写入配置文件
我们还可以使用configparser
模块来写入或更新配置文件:
import configparser
config = configparser.ConfigParser()
config['DEFAULT'] = {
'ServerAliveInterval': '45',
'Compression': 'yes',
'CompressionLevel': '9',
'ForwardX11': 'yes'
}
config['bitbucket.org'] = {'User': 'hg'}
config['topsecret.server.com'] = {'Host Port': '50022', 'ForwardX11': 'no'}
with open('config.ini', 'w') as configfile:
config.write(configfile)
二、使用JSON格式
JSON格式是一种轻量级的数据交换格式,非常适合配置文件的书写和读取。
1. 创建配置文件
首先,我们创建一个JSON格式的配置文件,例如config.json
:
{
"DEFAULT": {
"ServerAliveInterval": 45,
"Compression": "yes",
"CompressionLevel": 9,
"ForwardX11": "yes"
},
"bitbucket.org": {
"User": "hg"
},
"topsecret.server.com": {
"Host Port": 50022,
"ForwardX11": "no"
}
}
2. 读取配置文件
接下来,我们使用json
模块来读取JSON格式的配置文件:
import json
with open('config.json', 'r') as configfile:
config = json.load(configfile)
读取默认值
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
print(server_alive_interval)
读取特定部分的值
user = config['bitbucket.org']['User']
print(user)
host_port = config['topsecret.server.com']['Host Port']
print(host_port)
3. 写入配置文件
我们还可以使用json
模块来写入或更新JSON格式的配置文件:
import json
config = {
"DEFAULT": {
"ServerAliveInterval": 45,
"Compression": "yes",
"CompressionLevel": 9,
"ForwardX11": "yes"
},
"bitbucket.org": {
"User": "hg"
},
"topsecret.server.com": {
"Host Port": 50022,
"ForwardX11": "no"
}
}
with open('config.json', 'w') as configfile:
json.dump(config, configfile, indent=4)
三、使用YAML格式
YAML是一种人类可读的数据序列化标准,可以用来书写配置文件。
1. 创建配置文件
首先,我们创建一个YAML格式的配置文件,例如config.yaml
:
DEFAULT:
ServerAliveInterval: 45
Compression: yes
CompressionLevel: 9
ForwardX11: yes
bitbucket.org:
User: hg
topsecret.server.com:
Host Port: 50022
ForwardX11: no
2. 读取配置文件
接下来,我们使用PyYAML
库来读取YAML格式的配置文件:
import yaml
with open('config.yaml', 'r') as configfile:
config = yaml.safe_load(configfile)
读取默认值
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
print(server_alive_interval)
读取特定部分的值
user = config['bitbucket.org']['User']
print(user)
host_port = config['topsecret.server.com']['Host Port']
print(host_port)
3. 写入配置文件
我们还可以使用PyYAML
库来写入或更新YAML格式的配置文件:
import yaml
config = {
'DEFAULT': {
'ServerAliveInterval': 45,
'Compression': 'yes',
'CompressionLevel': 9,
'ForwardX11': 'yes'
},
'bitbucket.org': {
'User': 'hg'
},
'topsecret.server.com': {
'Host Port': 50022,
'ForwardX11': 'no'
}
}
with open('config.yaml', 'w') as configfile:
yaml.dump(config, configfile)
四、使用环境变量
环境变量是一种存储在操作系统环境中的变量,可以在Python程序中读取和写入。
1. 设置环境变量
我们可以在操作系统中设置环境变量,例如在Linux中:
export ServerAliveInterval=45
export Compression=yes
export CompressionLevel=9
export ForwardX11=yes
export User=hg
export HostPort=50022
2. 读取环境变量
我们可以使用os
模块来读取环境变量:
import os
server_alive_interval = os.getenv('ServerAliveInterval')
print(server_alive_interval)
compression = os.getenv('Compression')
print(compression)
compression_level = os.getenv('CompressionLevel')
print(compression_level)
forward_x11 = os.getenv('ForwardX11')
print(forward_x11)
user = os.getenv('User')
print(user)
host_port = os.getenv('HostPort')
print(host_port)
3. 写入环境变量
我们可以使用os
模块来写入环境变量:
import os
os.environ['ServerAliveInterval'] = '45'
os.environ['Compression'] = 'yes'
os.environ['CompressionLevel'] = '9'
os.environ['ForwardX11'] = 'yes'
os.environ['User'] = 'hg'
os.environ['HostPort'] = '50022'
五、总结
在Python中书写配置文件有多种方式,常见的有使用配置文件模块、使用JSON格式、使用YAML格式、使用环境变量。使用配置文件模块是最常见且灵活的一种方式,它可以处理多种格式的配置文件,并且提供了方便的读取和写入方法。使用JSON格式和YAML格式也非常常见,它们都是轻量级的数据交换格式,适合配置文件的书写和读取。使用环境变量也是一种常见的方式,它将配置存储在操作系统环境中,可以在Python程序中方便地读取和写入。根据实际需求选择合适的方式来书写和读取配置文件,可以提高代码的可维护性和可读性。
相关问答FAQs:
如何在Python中创建和读取配置文件?
在Python中,您可以使用configparser
模块来创建和读取配置文件。首先,您需要创建一个以.ini
为后缀的文本文件,并使用configparser
模块来读取该文件。以下是一个简单的示例:
import configparser
# 创建配置文件
config = configparser.ConfigParser()
config['DEFAULT'] = {'Server': 'localhost', 'Port': '8080'}
config['DATABASE'] = {'User': 'admin', 'Password': 'password123'}
with open('config.ini', 'w') as configfile:
config.write(configfile)
# 读取配置文件
config.read('config.ini')
print(config['DATABASE']['User']) # 输出: admin
Python配置文件的常见格式有哪些?
在Python中,最常见的配置文件格式是.ini
格式和.json
格式。.ini
格式使用键值对和节(section)来组织数据,非常适合存储简单的配置信息。而.json
格式则更加灵活,支持嵌套结构,适合复杂配置。根据需要选择合适的格式可以使配置文件更易于管理。
使用Python管理配置文件时,如何处理错误和异常?
在处理配置文件时,您可能会遇到文件缺失、格式错误等问题。使用try-except
语句可以帮助您捕获和处理这些异常。例如,在读取配置文件时,可以这样处理:
try:
config.read('config.ini')
except FileNotFoundError:
print("配置文件未找到,请检查路径。")
except configparser.Error as e:
print(f"配置文件格式错误: {e}")
通过这种方式,您可以确保程序在遇到配置文件问题时不会崩溃,并能给出友好的提示。
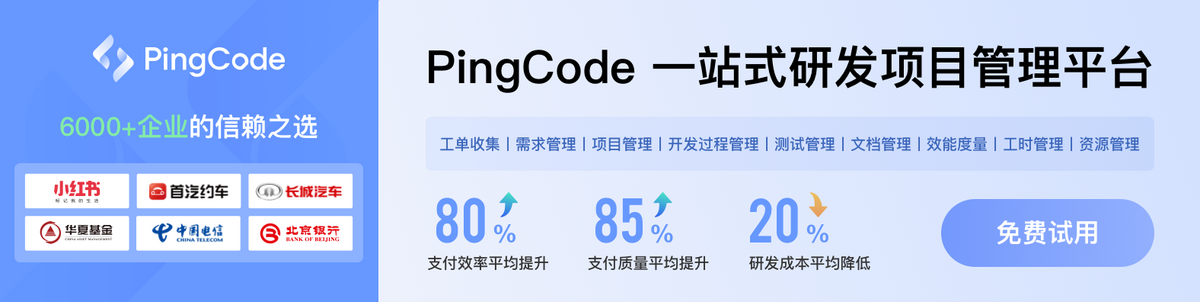