在Python中,从文件中挑选数据的方法有很多种,具体方法取决于文件的格式和你需要挑选的数据类型。常用的方法包括使用内置的open
函数、Pandas库、CSV模块、以及正则表达式等。本文将详细介绍这些方法,并提供一些实用的代码示例。
一、使用内置open
函数读取文件
使用Python的内置open
函数可以读取文本文件,并根据需要挑选出特定的数据。以下是一些常见的操作:
1. 逐行读取文件
逐行读取文件是最常见的操作之一,可以很方便地处理大文件。
# 打开文件
with open('example.txt', 'r') as file:
for line in file:
# 处理每一行
if 'keyword' in line:
print(line)
2. 读取整个文件并进行处理
有时候,我们需要一次性读取整个文件,然后进行处理。
# 打开文件
with open('example.txt', 'r') as file:
content = file.read()
lines = content.splitlines()
for line in lines:
# 处理每一行
if 'keyword' in line:
print(line)
二、使用Pandas库读取文件
Pandas是一个强大的数据分析库,非常适合处理结构化数据,如CSV文件、Excel文件等。
1. 读取CSV文件
Pandas提供了方便的read_csv
函数来读取CSV文件。
import pandas as pd
读取CSV文件
df = pd.read_csv('example.csv')
挑选特定的行或列
selected_rows = df[df['column_name'] == 'desired_value']
print(selected_rows)
2. 读取Excel文件
Pandas还提供了read_excel
函数来读取Excel文件。
import pandas as pd
读取Excel文件
df = pd.read_excel('example.xlsx')
挑选特定的行或列
selected_rows = df[df['column_name'] == 'desired_value']
print(selected_rows)
三、使用CSV模块读取文件
Python的CSV模块提供了处理CSV文件的简单方法。
1. 读取CSV文件
使用CSV模块读取CSV文件,并挑选特定的数据。
import csv
打开CSV文件
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
# 处理每一行
if 'keyword' in row:
print(row)
2. 使用DictReader读取CSV文件
DictReader可以将每一行数据转换为字典,更方便数据处理。
import csv
打开CSV文件
with open('example.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
# 处理每一行
if row['column_name'] == 'desired_value':
print(row)
四、使用正则表达式挑选数据
正则表达式提供了强大的文本匹配功能,适合处理复杂的文本数据。
1. 使用re模块进行正则匹配
使用Python的re模块,可以根据正则表达式进行匹配和挑选数据。
import re
打开文件
with open('example.txt', 'r') as file:
content = file.read()
使用正则表达式匹配
pattern = re.compile(r'\bkeyword\b')
matches = pattern.findall(content)
print(matches)
五、处理大文件的技巧
处理大文件时,需要注意内存使用和效率。以下是一些技巧:
1. 使用生成器逐行处理
使用生成器可以避免一次性读取大文件到内存中。
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
for line in read_large_file('large_file.txt'):
if 'keyword' in line:
print(line)
2. 分块读取文件
分块读取文件可以有效地处理大文件。
def read_in_chunks(file_path, chunk_size=1024):
with open(file_path, 'r') as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
yield chunk
for chunk in read_in_chunks('large_file.txt'):
if 'keyword' in chunk:
print(chunk)
六、处理特殊格式的文件
有时候,我们需要处理特殊格式的文件,如JSON、XML等。
1. 读取JSON文件
使用Python的json
模块可以方便地处理JSON文件。
import json
打开JSON文件
with open('example.json', 'r') as file:
data = json.load(file)
挑选特定的数据
if 'desired_key' in data:
print(data['desired_key'])
2. 读取XML文件
使用Python的xml
模块可以处理XML文件。
import xml.etree.ElementTree as ET
解析XML文件
tree = ET.parse('example.xml')
root = tree.getroot()
挑选特定的数据
for element in root.findall('.//desired_tag'):
print(element.text)
七、结合多种方法提高数据挑选效率
有时候,我们需要结合多种方法来提高数据挑选的效率和准确性。
1. 结合Pandas和正则表达式
结合Pandas和正则表达式,可以处理复杂的结构化数据。
import pandas as pd
import re
读取CSV文件
df = pd.read_csv('example.csv')
使用正则表达式匹配
pattern = re.compile(r'\bkeyword\b')
matches = df[df['column_name'].apply(lambda x: bool(pattern.search(x)))]
print(matches)
2. 使用多线程处理大文件
使用多线程可以提高处理大文件的效率。
import threading
def process_chunk(chunk):
for line in chunk:
if 'keyword' in line:
print(line)
def read_in_chunks(file_path, chunk_size=1024):
with open(file_path, 'r') as file:
while True:
chunk = file.readlines(chunk_size)
if not chunk:
break
yield chunk
threads = []
for chunk in read_in_chunks('large_file.txt'):
thread = threading.Thread(target=process_chunk, args=(chunk,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
八、总结
通过本文的介绍,我们了解了Python中从文件中挑选数据的多种方法,包括使用内置open
函数、Pandas库、CSV模块、正则表达式等。同时,我们还介绍了一些处理大文件的技巧和结合多种方法提高数据挑选效率的策略。选择合适的方法和工具,可以大大提高数据处理的效率和准确性。希望本文能对你有所帮助,在实际工作中能够灵活运用这些方法处理文件和数据。
相关问答FAQs:
如何使用Python从文本文件中提取特定行或内容?
在Python中,可以使用内置的文件操作函数来读取文件内容,结合条件语句来挑选特定行或内容。例如,可以使用with open()
语句打开文件,逐行读取并使用if
语句检查是否满足特定条件。以下是一个简单的代码示例:
with open('文件名.txt', 'r') as file:
for line in file:
if '特定内容' in line:
print(line)
这种方法适用于小型文件处理,若需处理大文件,考虑使用更高效的方法,如pandas
库。
如何从CSV文件中筛选数据?
使用pandas
库可以轻松地从CSV文件中筛选出所需的数据。首先,通过pandas.read_csv()
读取文件,然后使用条件过滤来挑选数据。以下是相关代码示例:
import pandas as pd
data = pd.read_csv('文件名.csv')
filtered_data = data[data['列名'] == '特定值']
print(filtered_data)
这种方式高效且易于处理大数据集,支持多种复杂的筛选条件。
有没有推荐的库或工具可以帮助高效地从文件中挑选数据?
除了Python的内置文件操作外,pandas
库是处理数据文件的最佳选择,尤其适合大型数据集。对于文本文件,可以使用re
库进行正则表达式匹配,从而实现更灵活的筛选。此外,csv
库也是处理CSV文件时的一个不错的选择,提供了简单易用的接口。如果需要处理Excel文件,可以考虑使用openpyxl
或xlsxwriter
等库。
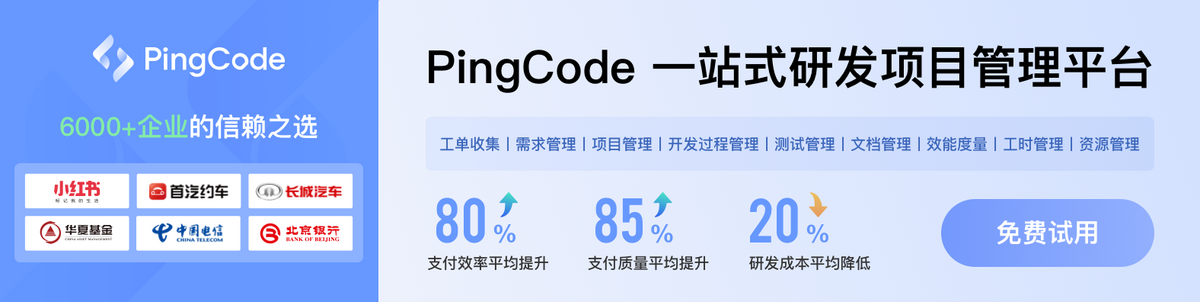