Python连接Elasticsearch并查询数据的步骤包括安装Elasticsearch库、配置连接、构建查询请求、处理响应等步骤。
一、安装Elasticsearch库
首先,我们需要安装用于连接Elasticsearch的Python库。最常用的库是elasticsearch-py
。可以通过以下命令安装:
pip install elasticsearch
二、配置连接
接下来,我们需要配置与Elasticsearch的连接。在Python中,可以使用以下代码进行连接配置:
from elasticsearch import Elasticsearch
创建Elasticsearch客户端实例
es = Elasticsearch(
['http://localhost:9200'], # Elasticsearch服务器地址
http_auth=('user', 'password'), # 认证信息(如果有)
scheme="http",
port=9200,
)
详细描述:在上述代码中,我们使用了Elasticsearch
类来创建一个客户端实例。['http://localhost:9200']
是Elasticsearch服务器的地址和端口,http_auth
是可选的认证信息。如果你的Elasticsearch服务器没有开启认证,可以省略此参数。
三、构建查询请求
在连接成功后,我们可以使用Elasticsearch的DSL(Domain Specific Language)来构建查询请求。DSL是一种基于JSON的查询语言,非常适用于构建复杂的查询。
例如,以下代码展示了如何查询指定索引中的所有文档:
# 构建查询请求
query = {
"query": {
"match_all": {}
}
}
执行查询
response = es.search(index="my_index", body=query)
输出结果
print(response)
四、处理响应
查询Elasticsearch会返回一个响应对象,其中包含了查询结果。我们可以解析这个响应对象来获取需要的数据。
例如,以下代码展示了如何处理查询响应并提取文档:
# 从响应中提取文档
documents = response['hits']['hits']
遍历文档并打印内容
for doc in documents:
print(doc['_source'])
五、其他查询示例
除了match_all
查询之外,Elasticsearch还支持多种查询类型,例如term
查询、range
查询、bool
查询等。以下是一些常见的查询示例:
- Term查询
用于精确匹配字段值。
query = {
"query": {
"term": {
"field_name": "value"
}
}
}
response = es.search(index="my_index", body=query)
- Range查询
用于匹配数值或日期范围。
query = {
"query": {
"range": {
"date_field": {
"gte": "2023-01-01",
"lte": "2023-12-31"
}
}
}
}
response = es.search(index="my_index", body=query)
- Bool查询
用于组合多个查询条件。
query = {
"query": {
"bool": {
"must": [
{"term": {"field1": "value1"}},
{"range": {"field2": {"gte": 10}}}
],
"filter": [
{"term": {"field3": "value3"}}
]
}
}
}
response = es.search(index="my_index", body=query)
六、分页查询
在查询结果较多时,Elasticsearch支持分页查询。可以通过from
和size
参数来控制返回结果的起始位置和数量。
query = {
"query": {
"match_all": {}
},
"from": 0,
"size": 10
}
response = es.search(index="my_index", body=query)
七、聚合查询
Elasticsearch提供了强大的聚合功能,可以进行数据统计和分组。
例如,以下代码展示了如何进行聚合查询以统计文档数量:
query = {
"aggs": {
"document_count": {
"value_count": {
"field": "_id"
}
}
}
}
response = es.search(index="my_index", body=query)
输出聚合结果
print(response['aggregations']['document_count']['value'])
八、索引文档
除了查询数据,Python还可以用来向Elasticsearch索引文档。以下代码展示了如何索引一个新的文档:
document = {
"title": "Elasticsearch Guide",
"author": "John Doe",
"publish_date": "2023-10-10",
"content": "This is a guide to Elasticsearch."
}
索引文档
response = es.index(index="my_index", body=document)
输出结果
print(response)
九、更新文档
在Elasticsearch中更新文档可以使用update
方法。以下代码展示了如何更新一个文档的字段:
# 更新文档
update = {
"doc": {
"title": "Updated Elasticsearch Guide"
}
}
response = es.update(index="my_index", id="1", body=update)
输出结果
print(response)
十、删除文档
删除文档可以使用delete
方法。以下代码展示了如何删除一个文档:
# 删除文档
response = es.delete(index="my_index", id="1")
输出结果
print(response)
十一、批量操作
批量操作可以使用bulk
方法来进行。以下代码展示了如何批量索引文档:
from elasticsearch.helpers import bulk
准备批量操作的数据
actions = [
{
"_index": "my_index",
"_type": "_doc",
"_id": 1,
"_source": {
"title": "Bulk Elasticsearch Guide",
"author": "Jane Doe",
"publish_date": "2023-11-01",
"content": "This is a bulk guide to Elasticsearch."
}
},
{
"_index": "my_index",
"_type": "_doc",
"_id": 2,
"_source": {
"title": "Another Bulk Guide",
"author": "Jane Doe",
"publish_date": "2023-11-02",
"content": "This is another bulk guide to Elasticsearch."
}
}
]
执行批量操作
response = bulk(es, actions)
输出结果
print(response)
十二、管理索引
Elasticsearch允许我们通过API管理索引,例如创建、删除和更新索引设置。
- 创建索引
可以使用indices.create
方法来创建一个新的索引:
# 创建索引
response = es.indices.create(index="new_index")
输出结果
print(response)
- 删除索引
可以使用indices.delete
方法来删除一个索引:
# 删除索引
response = es.indices.delete(index="new_index")
输出结果
print(response)
- 更新索引设置
可以使用indices.put_settings
方法来更新索引设置:
# 更新索引设置
settings = {
"index": {
"number_of_replicas": 2
}
}
response = es.indices.put_settings(index="my_index", body=settings)
输出结果
print(response)
十三、监控与调试
在开发过程中,监控和调试是必不可少的。Elasticsearch提供了多种监控和调试工具,例如cluster health
和node stats
。
- 集群健康
可以使用cluster.health
方法来获取集群的健康状态:
# 获取集群健康状态
response = es.cluster.health()
输出结果
print(response)
- 节点统计
可以使用nodes.stats
方法来获取节点的统计信息:
# 获取节点统计信息
response = es.nodes.stats()
输出结果
print(response)
十四、安全和认证
在生产环境中,确保Elasticsearch的安全性非常重要。可以通过以下方式实现安全和认证:
- 使用HTTPS
确保通信使用HTTPS而非HTTP:
es = Elasticsearch(
['https://localhost:9200'],
http_auth=('user', 'password'),
scheme="https",
port=9200,
)
- 使用API密钥
使用API密钥进行认证:
es = Elasticsearch(
['https://localhost:9200'],
api_key=('id', 'api_key'),
scheme="https",
port=9200,
)
通过以上步骤,您可以使用Python与Elasticsearch进行连接并查询数据。无论是基本的CRUD操作还是复杂的查询和聚合,Elasticsearch都提供了丰富的功能来满足各种需求。希望这篇文章对您有所帮助!
相关问答FAQs:
如何使用Python连接Elasticsearch并查询数据?
要使用Python连接Elasticsearch,您可以使用官方的Elasticsearch客户端库。首先,安装elasticsearch-py库,然后使用以下代码示例连接到您的Elasticsearch实例并查询数据:
from elasticsearch import Elasticsearch
# 创建Elasticsearch实例
es = Elasticsearch(['http://localhost:9200'])
# 检查连接
if es.ping():
print("连接成功!")
else:
print("连接失败!")
# 查询数据
response = es.search(index="您的索引名称", body={"query": {"match_all": {}}})
print(response)
确保替换“您的索引名称”为实际使用的索引名称。
在Python中如何处理Elasticsearch查询结果?
查询结果通常以JSON格式返回,您可以使用Python的json库或直接处理返回的字典对象来提取数据。以下是一个简单示例,展示如何遍历查询结果并提取特定字段:
for hit in response['hits']['hits']:
print(hit['_source']) # 打印每个文档的源数据
通过这种方式,您可以根据需要操作和分析查询到的数据。
如何确保Python与Elasticsearch之间的连接安全?
为了确保连接的安全性,您可以考虑以下措施:
- 使用HTTPS连接而不是HTTP,这样可以加密传输的数据。
- 如果Elasticsearch启用了身份验证,确保在连接时提供用户名和密码。
- 配置网络安全组或防火墙,以限制对Elasticsearch服务的访问,仅允许可信的IP地址连接。
通过采取以上措施,您可以增强Python与Elasticsearch之间连接的安全性。
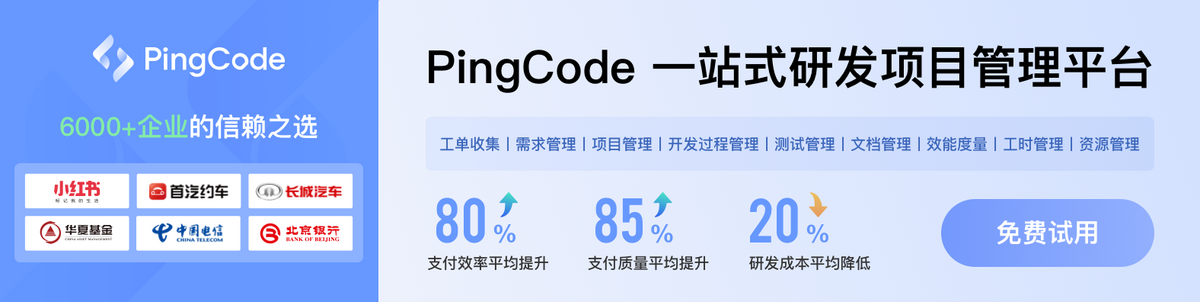