在Python 3中,继承函数可以通过类的继承来实现。在类中定义一个函数、在子类中重写该函数、使用super()调用父类的函数。这些步骤可以帮助你在子类中继承和扩展父类的函数。下面将详细描述其中的一个核心观点:使用super()
调用父类的函数。
使用super()
可以在子类中调用父类的方法。这样可以在子类中扩展父类的方法,而不必完全重写。super()
函数返回一个代理对象,该对象将方法调用委托给父类。这样可以确保代码的复用和扩展性。
一、继承函数的基本概念
在面向对象编程中,继承是一种机制,允许一个类(子类)从另一个类(父类)中继承属性和方法。子类可以直接使用父类的方法,也可以重写这些方法来实现不同的行为。Python 支持多重继承,即一个子类可以继承多个父类。
继承的基本语法
首先,我们需要了解继承的基本语法。假设我们有一个父类 Parent
和一个子类 Child
。
class Parent:
def some_method(self):
print("Parent method")
class Child(Parent):
pass
在这个例子中,Child
类继承了 Parent
类。因此,Child
类也具有 Parent
类中的 some_method
方法。
示例代码
class Parent:
def some_method(self):
print("Parent method")
class Child(Parent):
pass
c = Child()
c.some_method() # 输出: Parent method
二、重写父类方法
有时候,我们希望在子类中修改或扩展父类的方法。这可以通过重写父类的方法来实现。
重写方法的基本语法
我们可以在子类中定义一个与父类中同名的方法,这样子类中的方法就会覆盖父类中的方法。
class Parent:
def some_method(self):
print("Parent method")
class Child(Parent):
def some_method(self):
print("Child method")
示例代码
class Parent:
def some_method(self):
print("Parent method")
class Child(Parent):
def some_method(self):
print("Child method")
c = Child()
c.some_method() # 输出: Child method
三、使用super()调用父类方法
在子类中调用父类的方法,可以使用 super()
函数。super()
函数返回一个代理对象,该对象将方法调用委托给父类。
使用super()的基本语法
class Parent:
def some_method(self):
print("Parent method")
class Child(Parent):
def some_method(self):
super().some_method()
print("Child method")
在这个例子中,Child
类中的 some_method
方法首先调用了 Parent
类中的 some_method
方法,然后再执行自己的代码。
示例代码
class Parent:
def some_method(self):
print("Parent method")
class Child(Parent):
def some_method(self):
super().some_method()
print("Child method")
c = Child()
c.some_method()
输出:
Parent method
Child method
四、继承构造函数
在Python中,构造函数是通过 __init__
方法定义的。我们可以在子类中继承父类的构造函数,并使用 super()
调用父类的构造函数。
继承构造函数的基本语法
class Parent:
def __init__(self, value):
self.value = value
class Child(Parent):
def __init__(self, value, extra_value):
super().__init__(value)
self.extra_value = extra_value
示例代码
class Parent:
def __init__(self, value):
self.value = value
class Child(Parent):
def __init__(self, value, extra_value):
super().__init__(value)
self.extra_value = extra_value
c = Child(10, 20)
print(c.value) # 输出: 10
print(c.extra_value) # 输出: 20
五、示例项目:银行账户系统
为了更好地理解如何在实际项目中使用函数继承,我们可以创建一个简单的银行账户系统。这个系统包括一个父类 Account
和两个子类 SavingsAccount
和 CheckingAccount
。
创建父类Account
class Account:
def __init__(self, owner, balance=0):
self.owner = owner
self.balance = balance
def deposit(self, amount):
self.balance += amount
print(f"{amount} deposited. New balance is {self.balance}")
def withdraw(self, amount):
if amount > self.balance:
print("Insufficient funds")
else:
self.balance -= amount
print(f"{amount} withdrawn. New balance is {self.balance}")
创建子类SavingsAccount
class SavingsAccount(Account):
def __init__(self, owner, balance=0, interest_rate=0.01):
super().__init__(owner, balance)
self.interest_rate = interest_rate
def add_interest(self):
interest = self.balance * self.interest_rate
self.balance += interest
print(f"Interest added. New balance is {self.balance}")
创建子类CheckingAccount
class CheckingAccount(Account):
def __init__(self, owner, balance=0, overdraft_limit=0):
super().__init__(owner, balance)
self.overdraft_limit = overdraft_limit
def withdraw(self, amount):
if amount > self.balance + self.overdraft_limit:
print("Overdraft limit exceeded")
else:
self.balance -= amount
print(f"{amount} withdrawn. New balance is {self.balance}")
示例代码
# 创建储蓄账户
savings = SavingsAccount("Alice", 1000)
savings.deposit(500)
savings.add_interest()
savings.withdraw(300)
创建支票账户
checking = CheckingAccount("Bob", 500, 200)
checking.deposit(200)
checking.withdraw(800)
checking.withdraw(900)
六、使用抽象基类
有时候,我们需要确保子类实现某些方法。我们可以使用 abc
模块中的 ABC
类和 abstractmethod
装饰器来实现这一点。
使用抽象基类的基本语法
from abc import ABC, abstractmethod
class AbstractAccount(ABC):
@abstractmethod
def deposit(self, amount):
pass
@abstractmethod
def withdraw(self, amount):
pass
示例代码
from abc import ABC, abstractmethod
class AbstractAccount(ABC):
@abstractmethod
def deposit(self, amount):
pass
@abstractmethod
def withdraw(self, amount):
pass
class SavingsAccount(AbstractAccount):
def __init__(self, balance=0):
self.balance = balance
def deposit(self, amount):
self.balance += amount
print(f"{amount} deposited. New balance is {self.balance}")
def withdraw(self, amount):
if amount > self.balance:
print("Insufficient funds")
else:
self.balance -= amount
print(f"{amount} withdrawn. New balance is {self.balance}")
savings = SavingsAccount()
savings.deposit(500)
savings.withdraw(100)
七、继承与多态
多态是面向对象编程的一个重要概念,它允许我们使用统一的接口来调用不同子类的实现。在Python中,多态可以通过继承和方法重写来实现。
多态的基本概念
多态允许我们定义一个接口或基类,并在不同的子类中实现这个接口或基类的方法。这样,我们可以使用统一的接口来处理不同类型的对象,而不需要关心它们的具体类型。
示例代码
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
animals = [Dog(), Cat()]
for animal in animals:
print(animal.speak())
在这个例子中,我们定义了一个基类 Animal
,并在两个子类 Dog
和 Cat
中实现了 speak
方法。然后,我们可以使用统一的接口来调用不同子类的实现。
八、组合与继承的对比
在面向对象编程中,组合和继承是两种常见的代码复用方式。虽然继承可以让子类重用父类的方法,但在某些情况下,组合可能是更好的选择。
组合的基本概念
组合是一种将一个类的实例作为另一个类的属性的方式。这种方式可以让我们在不使用继承的情况下,实现代码复用。
示例代码
class Engine:
def start(self):
print("Engine started")
class Car:
def __init__(self):
self.engine = Engine()
def start(self):
self.engine.start()
print("Car started")
car = Car()
car.start()
在这个例子中,我们定义了一个 Engine
类,并将它作为 Car
类的一个属性。这样,Car
类可以使用 Engine
类的方法,而不需要继承 Engine
类。
九、继承与封装
封装是面向对象编程的一个重要概念,它允许我们将对象的状态和行为隐藏起来,只暴露必要的接口。在继承中,我们可以使用封装来控制子类对父类的访问。
封装的基本概念
封装可以通过将属性和方法设为私有来实现。在Python中,可以通过在属性和方法名前加下划线 _
来表示它们是私有的。
示例代码
class Parent:
def __init__(self):
self._private_attribute = "I am private"
def _private_method(self):
print("This is a private method")
class Child(Parent):
def access_private(self):
print(self._private_attribute)
self._private_method()
child = Child()
child.access_private()
在这个例子中,我们在 Parent
类中定义了一个私有属性 _private_attribute
和一个私有方法 _private_method
。子类 Child
可以访问这些私有属性和方法,但它们在类外部是不可见的。
十、继承与接口
接口是面向对象编程中的一个重要概念,它定义了一组方法,而不实现这些方法。在Python中,可以通过抽象基类来实现接口。
接口的基本概念
接口定义了一组方法,这些方法必须在实现接口的类中被实现。在Python中,可以通过 abc
模块中的 ABC
类和 abstractmethod
装饰器来定义接口。
示例代码
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius 2
shapes = [Rectangle(3, 4), Circle(2)]
for shape in shapes:
print(shape.area())
在这个例子中,我们定义了一个接口 Shape
,并在两个子类 Rectangle
和 Circle
中实现了 area
方法。这样,我们可以使用统一的接口来处理不同类型的对象。
十一、继承与装饰器
装饰器是一种修改函数或方法行为的设计模式。在Python中,装饰器可以用于修改继承的方法。
装饰器的基本概念
装饰器是一个返回函数的高阶函数。它可以在不修改原函数代码的情况下,扩展或修改函数的行为。
示例代码
def log_decorator(func):
def wrapper(*args, kwargs):
print(f"Calling {func.__name__}")
result = func(*args, kwargs)
print(f"Finished {func.__name__}")
return result
return wrapper
class Parent:
@log_decorator
def some_method(self):
print("Parent method")
class Child(Parent):
@log_decorator
def some_method(self):
print("Child method")
super().some_method()
c = Child()
c.some_method()
在这个例子中,我们定义了一个装饰器 log_decorator
,并将它应用于 Parent
类和 Child
类中的 some_method
方法。这样,我们可以在调用方法时打印日志信息。
十二、继承与mixin
Mixin是一种设计模式,它允许我们将多个类的功能组合在一起。在Python中,可以通过多重继承来实现mixin。
Mixin的基本概念
Mixin是一种只提供方法实现,而不定义任何状态(即不定义 __init__
方法)的类。它通常用于将多个功能组合在一起。
示例代码
class LogMixin:
def log(self, message):
print(f"LOG: {message}")
class Engine:
def start(self):
print("Engine started")
class Car(Engine, LogMixin):
def start(self):
super().start()
self.log("Car started")
car = Car()
car.start()
在这个例子中,我们定义了一个 LogMixin
类,它提供了一个 log
方法。然后,我们将 LogMixin
类和 Engine
类组合在一起,创建了一个 Car
类。这样,Car
类可以使用 LogMixin
类的方法,而不需要继承 LogMixin
类。
十三、继承与组合的实际应用
在实际应用中,我们可以将继承和组合结合起来使用,以实现更灵活的设计。
实际应用示例
假设我们需要创建一个文件处理系统,它包括读取文件、写入文件和压缩文件的功能。我们可以使用继承和组合来实现这个系统。
示例代码
class FileReader:
def read(self, file_path):
with open(file_path, 'r') as file:
return file.read()
class FileWriter:
def write(self, file_path, content):
with open(file_path, 'w') as file:
file.write(content)
class FileCompressor:
def compress(self, file_path):
print(f"Compressing {file_path}")
class FileHandler(FileReader, FileWriter, FileCompressor):
pass
handler = FileHandler()
content = handler.read('example.txt')
handler.write('example_copy.txt', content)
handler.compress('example_copy.txt')
在这个例子中,我们定义了三个类 FileReader
、FileWriter
和 FileCompressor
,它们分别提供读取文件、写入文件和压缩文件的功能。然后,我们将这些类组合在一起,创建了一个 FileHandler
类。这样,FileHandler
类可以使用所有这些功能,而不需要继承它们。
十四、总结
在Python 3中,通过继承函数可以实现代码复用和扩展。我们可以通过定义父类的方法,在子类中重写这些方法,并使用super()
调用父类的方法。此外,我们还可以使用抽象基类、多态、组合、装饰器和mixin等技术来实现更灵活和可维护的设计。在实际项目中,合理使用这些技术可以帮助我们编写更简洁、高效和可维护的代码。
相关问答FAQs:
如何在Python3中实现函数的继承?
在Python3中,函数本身并不直接支持继承。通常,函数是属于模块或类的。但如果你想要在子类中使用父类中的函数,可以通过重写或调用父类的方法来实现。具体来说,可以在子类中定义一个方法,并在该方法内调用父类的方法。
我可以在子类中对父类函数进行修改吗?
当然可以。在Python中,子类可以重写父类的方法。这意味着你可以在子类中定义一个与父类同名的方法,从而覆盖父类的方法实现。这对于扩展或修改父类的行为非常有用。
如何使用super()函数来调用父类的方法?
使用super()
函数可以方便地调用父类的方法。在子类中,你可以通过super().父类方法名()
来调用父类的方法。这种方式不仅清晰易懂,而且在多重继承的情况下能够确保调用到正确的父类方法。
在函数继承中,如何确保参数的传递正确?
当你在子类中重写父类的方法时,确保在子类方法中正确处理参数是非常重要的。通常,你可以使用*args
和**kwargs
来接受任意数量的位置参数和关键字参数,这样能够确保在子类中调用父类方法时,参数能够被正确传递。
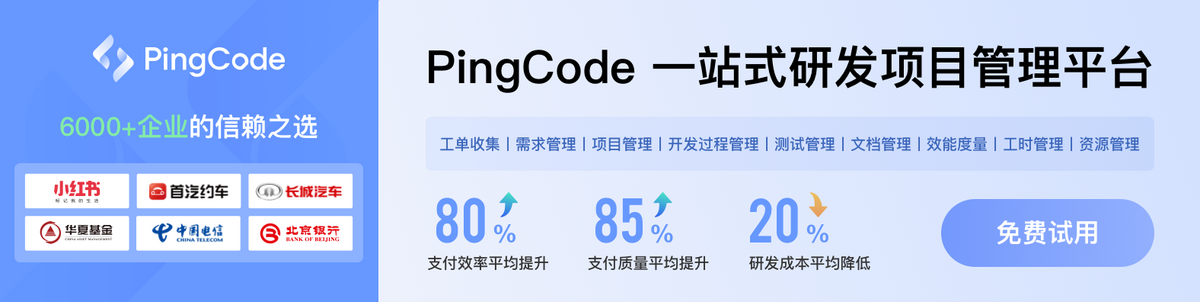