用Python提取信息的方法包括:正则表达式、BeautifulSoup、Scrapy、Pandas、Natural Language Toolkit (NLTK)等。其中,正则表达式用于模式匹配,BeautifulSoup和Scrapy用于网页数据抓取,Pandas用于结构化数据处理,NLTK用于自然语言处理。以下将详细描述如何使用正则表达式提取信息。
一、正则表达式
正则表达式(Regular Expressions)是一种用来匹配字符串中字符组合的模式。它在Python中通过re
模块使用。
1、基本用法
正则表达式可以用来查找、替换和提取文本中的特定模式。以下是一些常见的用法:
import re
查找所有匹配的字符串
pattern = r'\d+' # 匹配所有数字
text = "There are 123 apples and 456 oranges."
matches = re.findall(pattern, text)
print(matches) # ['123', '456']
替换匹配的字符串
replaced_text = re.sub(pattern, 'NUMBER', text)
print(replaced_text) # "There are NUMBER apples and NUMBER oranges."
提取匹配的字符串
match = re.search(pattern, text)
if match:
print(match.group()) # '123'
2、高级用法
通过捕获组(Capturing Groups)和非捕获组(Non-Capturing Groups),可以更精细地提取信息:
# 捕获组
pattern = r'(\d+) apples and (\d+) oranges'
match = re.search(pattern, text)
if match:
apples = match.group(1)
oranges = match.group(2)
print(f'Apples: {apples}, Oranges: {oranges}') # "Apples: 123, Oranges: 456"
二、BeautifulSoup
BeautifulSoup是一个用于解析HTML和XML文档的库,适用于从网页中提取数据。
1、安装和基本用法
首先,安装BeautifulSoup和requests库:
pip install beautifulsoup4 requests
然后,使用以下代码从网页中提取信息:
import requests
from bs4 import BeautifulSoup
获取网页内容
url = 'http://example.com'
response = requests.get(url)
html_content = response.content
解析网页内容
soup = BeautifulSoup(html_content, 'html.parser')
提取特定信息,例如标题和所有链接
title = soup.title.string
links = soup.find_all('a')
print(f'Title: {title}')
for link in links:
print(link.get('href'))
2、高级用法
通过更复杂的查询方法,可以更精细地提取信息:
# 查找特定的类或ID
specific_div = soup.find('div', {'class': 'specific-class'})
print(specific_div.text)
使用CSS选择器
selected_items = soup.select('div.specific-class > ul > li')
for item in selected_items:
print(item.text)
三、Scrapy
Scrapy是一个强大的网页抓取框架,适用于需要大量抓取数据的情况。
1、安装和基本用法
首先,安装Scrapy:
pip install scrapy
创建一个新的Scrapy项目:
scrapy startproject myproject
编写一个爬虫(Spider)来抓取数据:
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com']
def parse(self, response):
title = response.css('title::text').get()
self.log(f'Title: {title}')
运行爬虫:
scrapy crawl myspider
2、高级用法
通过使用Scrapy的Item和Pipeline,可以更系统地处理和存储抓取到的数据:
# items.py
import scrapy
class MyItem(scrapy.Item):
title = scrapy.Field()
link = scrapy.Field()
myspider.py
import scrapy
from myproject.items import MyItem
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com']
def parse(self, response):
item = MyItem()
item['title'] = response.css('title::text').get()
item['link'] = response.css('a::attr(href)').get()
yield item
pipelines.py
class MyPipeline:
def process_item(self, item, spider):
print(f"Title: {item['title']}, Link: {item['link']}")
return item
settings.py
ITEM_PIPELINES = {
'myproject.pipelines.MyPipeline': 300,
}
四、Pandas
Pandas是一个强大的数据分析工具库,适用于处理结构化数据。
1、安装和基本用法
首先,安装Pandas:
pip install pandas
然后,使用以下代码读取和处理CSV文件中的数据:
import pandas as pd
读取CSV文件
df = pd.read_csv('data.csv')
查看数据
print(df.head())
提取特定列
column_data = df['column_name']
print(column_data)
2、高级用法
通过DataFrame的查询和操作方法,可以更复杂地处理数据:
# 过滤数据
filtered_df = df[df['column_name'] > 10]
print(filtered_df)
分组和聚合数据
grouped_df = df.groupby('group_column').sum()
print(grouped_df)
数据清洗
df['column_name'] = df['column_name'].str.strip()
df.dropna(inplace=True)
五、Natural Language Toolkit (NLTK)
NLTK是一个用于自然语言处理的库,适用于从文本中提取信息。
1、安装和基本用法
首先,安装NLTK:
pip install nltk
然后,使用以下代码进行基本的文本处理:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
text = "This is a sample sentence."
tokens = word_tokenize(text)
print(tokens) # ['This', 'is', 'a', 'sample', 'sentence', '.']
2、高级用法
通过更多的NLTK工具,可以进行更复杂的自然语言处理任务:
# 词性标注
nltk.download('averaged_perceptron_tagger')
tagged_tokens = nltk.pos_tag(tokens)
print(tagged_tokens) # [('This', 'DT'), ('is', 'VBZ'), ('a', 'DT'), ('sample', 'NN'), ('sentence', 'NN'), ('.', '.')]
命名实体识别
nltk.download('maxent_ne_chunker')
nltk.download('words')
entities = nltk.ne_chunk(tagged_tokens)
print(entities)
语法解析
grammar = "NP: {<DT>?<JJ>*<NN>}"
cp = nltk.RegexpParser(grammar)
result = cp.parse(tagged_tokens)
print(result)
通过以上几种方法,Python可以高效地从不同类型的数据源中提取信息。选择合适的方法可以根据具体的需求和数据源的类型来决定。
相关问答FAQs:
如何使用Python提取特定格式的数据?
使用Python提取特定格式的数据通常涉及使用正则表达式、Beautiful Soup库或Pandas库等工具。对于文本文件或网页内容,正则表达式可以帮助你匹配和提取符合特定模式的字符串。Beautiful Soup非常适合从HTML和XML文档中提取数据,而Pandas则可以处理结构化数据,例如CSV或Excel文件。通过结合这些工具,可以高效地提取所需的信息。
在Python中提取信息的常用库有哪些?
常用的库包括Beautiful Soup、Requests、Pandas、Scrapy和re(正则表达式)。Beautiful Soup用于解析HTML和XML文档,Requests用于发送HTTP请求并获取网页内容。Pandas则适合处理表格数据,Scrapy是一个强大的爬虫框架,可以抓取和提取数据。使用这些库,可以根据需要灵活选择合适的工具。
如何处理提取到的信息以进行分析?
提取到的信息通常需要进一步处理才能进行分析。可以使用Pandas进行数据清洗和整理,例如去除重复项、填补缺失值和转换数据类型。还可以使用Matplotlib或Seaborn等可视化库对数据进行可视化,以便更直观地理解和分析信息。通过这些步骤,可以将提取到的数据转化为有用的见解。
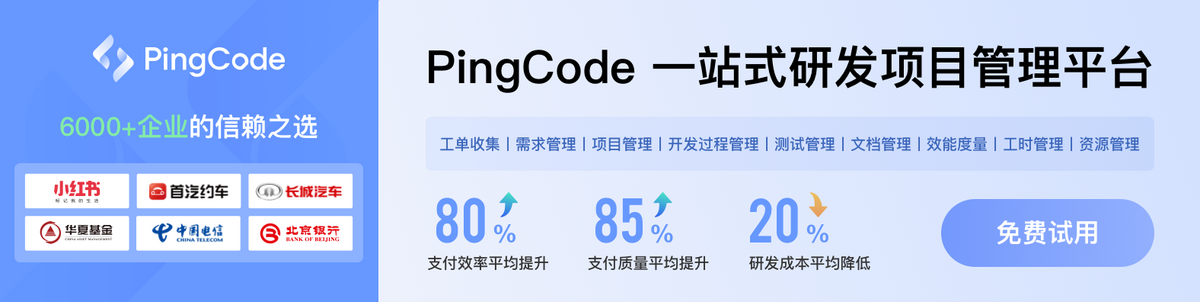