要用Python计算企业利润,可以通过编写代码实现。首先需要明确收入、成本和利润的关系,确定收入和成本的数据来源、编写计算逻辑、进行数据验证。本文将从以下几个方面详细介绍如何用Python求企业利润。
一、收入和成本的定义和数据获取
收入通常来自销售商品或服务的总金额,它可以通过销售记录、发票等途径获取。成本则包括制造成本、运营成本、人力成本等,可以通过财务记录、采购单等获取。
1、收入的定义和获取
收入可以从多个来源获取,例如数据库、Excel文件、API等。以下是几种常见的获取方式。
- 从数据库获取:企业的销售记录通常存储在数据库中,可以通过SQL查询获取。
import sqlite3
def get_revenue_from_db(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute("SELECT SUM(amount) FROM sales")
revenue = cursor.fetchone()[0]
conn.close()
return revenue
- 从Excel文件获取:如果销售记录存储在Excel文件中,可以使用
pandas
库读取数据。
import pandas as pd
def get_revenue_from_excel(file_path):
df = pd.read_excel(file_path)
revenue = df['amount'].sum()
return revenue
2、成本的定义和获取
成本包括直接成本和间接成本,可以通过财务记录、采购订单等方式获取。
- 从数据库获取:
def get_cost_from_db(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute("SELECT SUM(amount) FROM costs")
cost = cursor.fetchone()[0]
conn.close()
return cost
- 从Excel文件获取:
def get_cost_from_excel(file_path):
df = pd.read_excel(file_path)
cost = df['amount'].sum()
return cost
二、编写利润计算逻辑
利润计算的基本公式是利润 = 收入 – 成本。在获取收入和成本数据后,可以直接用此公式计算利润。
def calculate_profit(revenue, cost):
profit = revenue - cost
return profit
三、数据验证和结果展示
在实际操作中,需要验证数据的准确性,确保计算结果的可靠性。可以通过对比历史数据、检查异常值等方式进行验证。
1、数据验证
- 历史数据对比:对比当前数据与历史数据,检查是否存在异常波动。
def validate_data(current_revenue, current_cost, historical_revenue, historical_cost):
revenue_change = (current_revenue - historical_revenue) / historical_revenue
cost_change = (current_cost - historical_cost) / historical_cost
if abs(revenue_change) > 0.2 or abs(cost_change) > 0.2:
print("Warning: Significant change in revenue or cost")
else:
print("Data validation passed")
- 异常值检查:检查数据中是否存在异常值,如收入或成本为负值。
def check_for_anomalies(revenue, cost):
if revenue < 0 or cost < 0:
print("Error: Negative value found in revenue or cost")
else:
print("No anomalies found")
2、结果展示
计算结果可以通过打印、生成报告、数据可视化等方式展示。
- 打印结果:
def display_result(profit):
print(f"The calculated profit is: {profit}")
- 生成报告:可以使用
pandas
库生成报告并保存为Excel文件。
def generate_report(revenue, cost, profit, file_path):
data = {'Revenue': [revenue], 'Cost': [cost], 'Profit': [profit]}
df = pd.DataFrame(data)
df.to_excel(file_path, index=False)
print(f"Report saved to {file_path}")
- 数据可视化:使用
matplotlib
库进行数据可视化。
import matplotlib.pyplot as plt
def plot_profit(revenue, cost, profit):
labels = ['Revenue', 'Cost', 'Profit']
values = [revenue, cost, profit]
plt.bar(labels, values)
plt.title('Revenue, Cost, and Profit')
plt.show()
四、综合示例
将以上内容综合起来,编写一个完整的Python程序计算企业利润。
import sqlite3
import pandas as pd
import matplotlib.pyplot as plt
def get_revenue_from_db(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute("SELECT SUM(amount) FROM sales")
revenue = cursor.fetchone()[0]
conn.close()
return revenue
def get_cost_from_db(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute("SELECT SUM(amount) FROM costs")
cost = cursor.fetchone()[0]
conn.close()
return cost
def calculate_profit(revenue, cost):
profit = revenue - cost
return profit
def validate_data(current_revenue, current_cost, historical_revenue, historical_cost):
revenue_change = (current_revenue - historical_revenue) / historical_revenue
cost_change = (current_cost - historical_cost) / historical_cost
if abs(revenue_change) > 0.2 or abs(cost_change) > 0.2:
print("Warning: Significant change in revenue or cost")
else:
print("Data validation passed")
def check_for_anomalies(revenue, cost):
if revenue < 0 or cost < 0:
print("Error: Negative value found in revenue or cost")
else:
print("No anomalies found")
def display_result(profit):
print(f"The calculated profit is: {profit}")
def generate_report(revenue, cost, profit, file_path):
data = {'Revenue': [revenue], 'Cost': [cost], 'Profit': [profit]}
df = pd.DataFrame(data)
df.to_excel(file_path, index=False)
print(f"Report saved to {file_path}")
def plot_profit(revenue, cost, profit):
labels = ['Revenue', 'Cost', 'Profit']
values = [revenue, cost, profit]
plt.bar(labels, values)
plt.title('Revenue, Cost, and Profit')
plt.show()
Example usage
db_path = 'path_to_your_database.db'
historical_revenue = 100000 # Example historical data
historical_cost = 50000 # Example historical data
revenue = get_revenue_from_db(db_path)
cost = get_cost_from_db(db_path)
profit = calculate_profit(revenue, cost)
validate_data(revenue, cost, historical_revenue, historical_cost)
check_for_anomalies(revenue, cost)
display_result(profit)
generate_report(revenue, cost, profit, 'profit_report.xlsx')
plot_profit(revenue, cost, profit)
五、总结
通过以上步骤,使用Python计算企业利润的过程已经完整展示。收入和成本的数据获取、利润计算逻辑的编写、数据验证和结果展示,每一步都至关重要。实际应用中,还可以根据具体需求进行扩展和优化,例如增加更多的成本分类、引入更复杂的收入模型等。通过这些方法,可以更准确地计算和分析企业的利润,从而为企业决策提供有力支持。
相关问答FAQs:
如何用Python计算企业的总收入?
要计算企业的总收入,首先需要确定所有的销售数据。这些数据通常包括每个产品或服务的销售量和单价。可以使用Python中的Pandas库来处理数据,读取销售记录,然后通过简单的乘法和求和操作来计算总收入。例如,可以使用df['销售量'] * df['单价']
来计算每个产品的收入,并使用sum()
函数来得到总收入。
在Python中如何处理成本数据以计算利润?
计算企业利润需要减去成本。成本数据可能包括固定成本和变动成本。可以使用Python读取成本数据文件,将其存入数据框中,并使用合适的数学运算来计算总成本。例如,可以通过对固定成本和变动成本进行求和来获得总成本。随后用总收入减去总成本即可得出利润。
有哪些Python库可以帮助计算企业利润?
Python有多个库可以帮助进行利润计算,尤其是Pandas和NumPy。Pandas适合处理表格数据,方便读取和操作销售及成本数据;而NumPy提供了高效的数组计算功能,适合进行大规模的数据分析。此外,Matplotlib和Seaborn等可视化库可以帮助将计算结果以图形形式呈现,便于分析企业的利润表现。
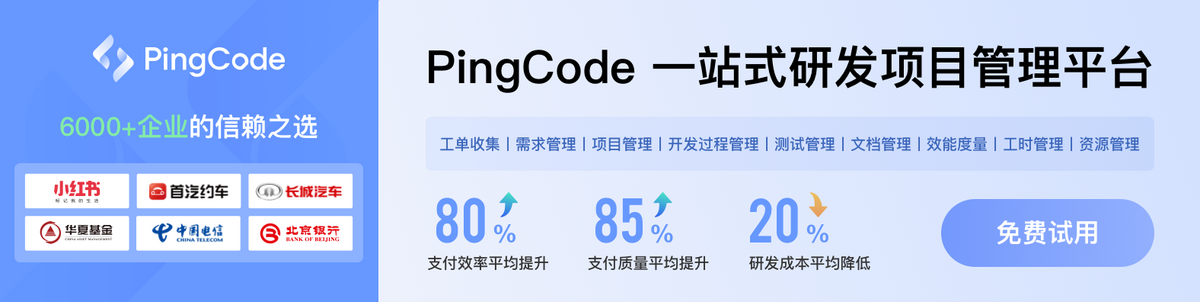