在Python中,类的使用主要包括定义类、创建实例、调用方法、继承等几个方面。定义类是使用class关键字,创建实例是通过类名加括号,调用方法是通过实例名加方法名,继承是通过在类名后加上父类名来实现。下面将详细介绍每一个方面。
一、定义类
在Python中,类是使用class
关键字定义的。类的定义包括类名、类属性和类方法。类名通常使用驼峰命名法。
class MyClass:
class_attribute = 'This is a class attribute'
def __init__(self, instance_attribute):
self.instance_attribute = instance_attribute
def instance_method(self):
return f'This is an instance method. The instance attribute is {self.instance_attribute}'
在上面的例子中,MyClass
是类名,class_attribute
是类属性,__init__
是初始化方法(构造函数),instance_attribute
是实例属性,instance_method
是实例方法。
详细描述:__init__
方法是一个特殊的方法,它在类的实例被创建时自动调用,用于初始化实例的属性。self
参数是指向实例本身的引用,通过它可以访问实例的属性和方法。
二、创建实例
创建类的实例非常简单,只需调用类名并传递必要的参数即可。
my_instance = MyClass('This is an instance attribute')
在上面的例子中,我们创建了一个MyClass
类的实例my_instance
,并传递了一个参数'This is an instance attribute
',这个参数将被__init__
方法接收并赋值给实例属性instance_attribute
。
三、调用方法
在Python中,调用方法是通过实例名加上方法名来实现的。
print(my_instance.instance_method())
在上面的例子中,我们调用了实例my_instance
的instance_method
方法,并打印了返回值。
四、继承
继承是面向对象编程中的一个重要概念,通过继承可以实现代码的重用和扩展。在Python中,继承是通过在类名后加上父类名来实现的。
class SubClass(MyClass):
def __init__(self, instance_attribute, additional_attribute):
super().__init__(instance_attribute)
self.additional_attribute = additional_attribute
def additional_method(self):
return f'This is an additional method. The additional attribute is {self.additional_attribute}'
在上面的例子中,SubClass
继承了MyClass
,并在初始化方法中调用了父类的__init__
方法(通过super()
函数),然后添加了一个新的实例属性additional_attribute
和一个新的实例方法additional_method
。
五、类的更多用法
1、类属性和实例属性
类属性是类级别的属性,所有实例共享,而实例属性是实例级别的属性,每个实例都有自己独立的值。
class Car:
wheels = 4 # 类属性
def __init__(self, color):
self.color = color # 实例属性
car1 = Car('red')
car2 = Car('blue')
print(car1.wheels) # 输出4
print(car2.wheels) # 输出4
print(car1.color) # 输出red
print(car2.color) # 输出blue
在上面的例子中,wheels
是类属性,所有实例共享,而color
是实例属性,每个实例都有自己的值。
2、类方法和静态方法
类方法是绑定到类的而不是实例的方法,通常用来操作类属性或调用类方法。静态方法是与类相关联的方法,但不操作类或实例属性。
class MyClass:
class_attribute = 'class attribute'
@classmethod
def class_method(cls):
return f'This is a class method. The class attribute is {cls.class_attribute}'
@staticmethod
def static_method():
return 'This is a static method.'
print(MyClass.class_method()) # 输出: This is a class method. The class attribute is class attribute
print(MyClass.static_method()) # 输出: This is a static method.
在上面的例子中,class_method
是类方法,使用@classmethod
装饰器定义,static_method
是静态方法,使用@staticmethod
装饰器定义。
3、私有属性和方法
在Python中,私有属性和方法是通过在属性和方法名前加下划线来表示的。
class MyClass:
def __init__(self):
self._private_attribute = 'This is a private attribute'
def _private_method(self):
return 'This is a private method'
def public_method(self):
return self._private_method()
my_instance = MyClass()
print(my_instance.public_method()) # 输出: This is a private method
print(my_instance._private_method()) # 这样会报错,不能直接访问私有方法
在上面的例子中,_private_attribute
和_private_method
是私有属性和方法,不能在类外部直接访问。
4、特殊方法
Python类中有许多特殊的方法(也称为魔法方法),它们以双下划线开头和结尾,用于实现某些特定的行为。
class MyClass:
def __init__(self, value):
self.value = value
def __str__(self):
return f'MyClass with value {self.value}'
def __add__(self, other):
return MyClass(self.value + other.value)
my_instance1 = MyClass(5)
my_instance2 = MyClass(10)
print(my_instance1) # 输出: MyClass with value 5
print(my_instance1 + my_instance2) # 输出: MyClass with value 15
在上面的例子中,__str__
方法用于定义类的字符串表示,__add__
方法用于定义加法运算符的行为。
六、类的高级用法
1、属性装饰器
属性装饰器@property
用于将方法转换为只读属性。
class MyClass:
def __init__(self, value):
self._value = value
@property
def value(self):
return self._value
my_instance = MyClass(10)
print(my_instance.value) # 输出: 10
my_instance.value = 20 # 这样会报错,属性是只读的
在上面的例子中,value
方法被@property
装饰器转换为只读属性,不能直接修改。
2、数据类
Python 3.7引入了数据类(dataclass),用于简化类的定义。
from dataclasses import dataclass
@dataclass
class MyClass:
name: str
age: int
my_instance = MyClass('Alice', 30)
print(my_instance) # 输出: MyClass(name='Alice', age=30)
在上面的例子中,@dataclass
装饰器自动生成了初始化方法和其他常用的方法,大大简化了类的定义。
七、类的实际应用
1、面向对象编程
面向对象编程(OOP)是一种编程范式,通过使用类和对象来组织代码。OOP的主要特性包括封装、继承和多态。
class Animal:
def speak(self):
raise NotImplementedError("Subclasses must implement this method")
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
animals = [Dog(), Cat()]
for animal in animals:
print(animal.speak())
在上面的例子中,Animal
是一个抽象类,定义了一个抽象方法speak
,Dog
和Cat
类继承了Animal
并实现了speak
方法。通过多态,animals
列表中的每个实例都可以调用speak
方法,输出不同的结果。
2、设计模式
设计模式是解决特定问题的通用解决方案。在Python中,类常用于实现各种设计模式,如单例模式、工厂模式、观察者模式等。
单例模式
单例模式确保一个类只有一个实例,并提供全局访问点。
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if not cls._instance:
cls._instance = super().__new__(cls, *args, kwargs)
return cls._instance
singleton1 = Singleton()
singleton2 = Singleton()
print(singleton1 is singleton2) # 输出: True
在上面的例子中,Singleton
类通过重写__new__
方法确保只有一个实例。
工厂模式
工厂模式用于创建对象,而不公开实例化逻辑。
class AnimalFactory:
@staticmethod
def create_animal(animal_type):
if animal_type == 'dog':
return Dog()
elif animal_type == 'cat':
return Cat()
else:
raise ValueError("Unknown animal type")
animal_factory = AnimalFactory()
dog = animal_factory.create_animal('dog')
cat = animal_factory.create_animal('cat')
print(dog.speak()) # 输出: Woof!
print(cat.speak()) # 输出: Meow!
在上面的例子中,AnimalFactory
类使用静态方法create_animal
根据传递的参数创建不同的动物实例。
八、类的最佳实践
1、遵循PEP 8编码规范
PEP 8是Python的编码规范,遵循PEP 8可以提高代码的可读性和可维护性。类名应使用驼峰命名法,方法和属性名应使用小写字母和下划线分隔。
class MyClass:
def my_method(self):
pass
2、适当使用类和实例属性
类属性适用于所有实例共享的数据,实例属性适用于每个实例独立的数据。避免过度使用类属性和实例属性,以保持代码简洁和清晰。
class Car:
wheels = 4 # 类属性
def __init__(self, color):
self.color = color # 实例属性
3、合理使用继承和组合
继承用于表示"is-a"关系,组合用于表示"has-a"关系。避免深层次的继承层次,以保持代码的可维护性和可扩展性。
class Engine:
def start(self):
return "Engine started"
class Car:
def __init__(self, color):
self.color = color
self.engine = Engine() # 组合关系
def start(self):
return self.engine.start()
在上面的例子中,Car
类组合了Engine
类,表示"Car has an Engine"的关系。
4、使用抽象基类
抽象基类用于定义接口和强制子类实现特定方法。Python提供了abc
模块来定义抽象基类。
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
dog = Dog()
print(dog.speak()) # 输出: Woof!
在上面的例子中,Animal
是一个抽象基类,定义了一个抽象方法speak
,Dog
类继承了Animal
并实现了speak
方法。
5、编写单元测试
编写单元测试可以确保类的行为符合预期,提高代码的可靠性和可维护性。Python提供了unittest
模块来编写单元测试。
import unittest
class TestMyClass(unittest.TestCase):
def test_instance_method(self):
my_instance = MyClass('test')
self.assertEqual(my_instance.instance_method(), 'This is an instance method. The instance attribute is test')
if __name__ == '__main__':
unittest.main()
在上面的例子中,我们使用unittest
模块编写了一个单元测试,测试MyClass
类的instance_method
方法。
九、总结
在Python中,类是面向对象编程的基础,通过类可以封装数据和行为,实现代码的重用和扩展。定义类、创建实例、调用方法和继承是类的基本用法。此外,Python还提供了类属性和实例属性、类方法和静态方法、私有属性和方法、特殊方法、属性装饰器、数据类等高级用法。通过合理使用类和遵循最佳实践,可以编写出高质量的Python代码。
希望通过本文的介绍,您对Python中的类有了更深入的理解,并能够在实际项目中灵活应用。
相关问答FAQs:
Python中的类与对象有什么区别?
类是对象的蓝图或模板,定义了对象的属性和方法。对象是类的实例,具有类所定义的属性和行为。通过类,可以创建多个对象,每个对象都可以拥有不同的属性值,但它们共享相同的方法。
在Python中创建类时,__init__方法的作用是什么?__init__
方法是类的构造函数,用于初始化对象的属性。当创建一个类的实例时,__init__
方法会自动被调用,它可以接受参数以初始化对象的属性,使得对象在创建时就具备必要的状态。
如何在Python类中实现继承?
继承允许一个类获取另一个类的属性和方法。在Python中,可以通过在定义子类时将父类名放在括号内来实现。例如:class 子类名(父类名):
。这使得子类能够重用父类的代码,同时也可以重写父类的方法以实现特定的功能。
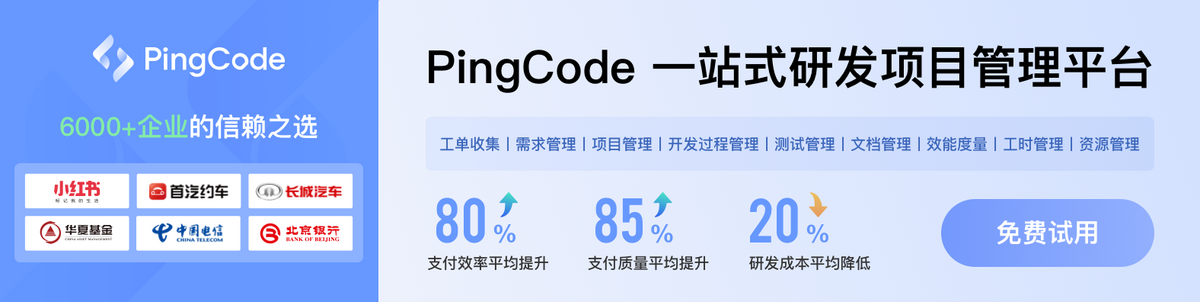