在Python中绘制图像子图可以使用多个库,其中最常用的就是Matplotlib库。通过使用Matplotlib的subplot
和subplots
函数、利用GridSpec
来创建复杂的布局、以及自定义子图的大小和形状,你可以实现多种多样的子图绘制需求。下面将详细介绍如何使用这些工具和方法来创建和管理子图。
一、使用subplot
函数
subplot
函数可以快速创建简单的子图布局。其基本用法是plt.subplot(nrows, ncols, index)
,其中nrows
是子图的行数,ncols
是子图的列数,index
是子图的索引,从1开始。
import matplotlib.pyplot as plt
创建一个2行2列的子图
plt.figure(figsize=(10, 10))
plt.subplot(2, 2, 1)
plt.plot([1, 2, 3], [1, 4, 9])
plt.title('Subplot 1')
plt.subplot(2, 2, 2)
plt.plot([1, 2, 3], [1, 2, 3])
plt.title('Subplot 2')
plt.subplot(2, 2, 3)
plt.plot([1, 2, 3], [1, 0, 1])
plt.title('Subplot 3')
plt.subplot(2, 2, 4)
plt.plot([1, 2, 3], [3, 2, 1])
plt.title('Subplot 4')
plt.tight_layout()
plt.show()
二、使用subplots
函数
subplots
函数提供了更灵活和直观的方式来创建子图。其基本用法是fig, axs = plt.subplots(nrows, ncols)
,其中fig
是图形对象,axs
是子图对象的数组。
import matplotlib.pyplot as plt
创建一个2行2列的子图
fig, axs = plt.subplots(2, 2, figsize=(10, 10))
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 0].set_title('Subplot 1')
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[0, 1].set_title('Subplot 2')
axs[1, 0].plot([1, 2, 3], [1, 0, 1])
axs[1, 0].set_title('Subplot 3')
axs[1, 1].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].set_title('Subplot 4')
fig.tight_layout()
plt.show()
三、使用GridSpec
创建复杂布局
当需要创建复杂的子图布局时,GridSpec
是一个强大的工具。它允许创建具有不同大小和形状的子图。
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
fig = plt.figure(figsize=(10, 10))
gs = gridspec.GridSpec(3, 3)
ax1 = fig.add_subplot(gs[0, :])
ax1.plot([1, 2, 3], [1, 4, 9])
ax1.set_title('Subplot 1')
ax2 = fig.add_subplot(gs[1, :-1])
ax2.plot([1, 2, 3], [1, 2, 3])
ax2.set_title('Subplot 2')
ax3 = fig.add_subplot(gs[1:, -1])
ax3.plot([1, 2, 3], [1, 0, 1])
ax3.set_title('Subplot 3')
ax4 = fig.add_subplot(gs[-1, 0])
ax4.plot([1, 2, 3], [3, 2, 1])
ax4.set_title('Subplot 4')
ax5 = fig.add_subplot(gs[-1, -2])
ax5.plot([1, 2, 3], [2, 3, 1])
ax5.set_title('Subplot 5')
fig.tight_layout()
plt.show()
四、自定义子图的大小和形状
有时候你可能需要特定大小和形状的子图,这可以通过调整figsize
参数来实现。此外,还可以使用add_gridspec
来进一步自定义子图的布局。
import matplotlib.pyplot as plt
创建一个自定义大小的子图
fig, axs = plt.subplots(2, 2, figsize=(12, 8))
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 0].set_title('Subplot 1')
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[0, 1].set_title('Subplot 2')
axs[1, 0].plot([1, 2, 3], [1, 0, 1])
axs[1, 0].set_title('Subplot 3')
axs[1, 1].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].set_title('Subplot 4')
fig.tight_layout()
plt.show()
五、结合多个子图方法
在实际应用中,你可以结合多个子图方法来创建更复杂和美观的图形。例如,使用GridSpec
来定义大致的布局,然后在特定区域中使用subplots
来创建更多子图。
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
fig = plt.figure(figsize=(15, 10))
gs = gridspec.GridSpec(2, 2, width_ratios=[3, 1], height_ratios=[1, 3])
ax1 = fig.add_subplot(gs[0, 0])
ax1.plot([1, 2, 3], [1, 4, 9])
ax1.set_title('Large Subplot')
sub_gs = gridspec.GridSpecFromSubplotSpec(3, 1, subplot_spec=gs[1, 0])
for i in range(3):
ax = fig.add_subplot(sub_gs[i])
ax.plot([1, 2, 3], [3 - i, 2 - i, 1 - i])
ax.set_title(f'Subplot {i+1}')
ax_right = fig.add_subplot(gs[:, 1])
ax_right.plot([1, 2, 3], [1, 4, 9])
ax_right.set_title('Right Subplot')
fig.tight_layout()
plt.show()
六、子图的样式和美化
为了使子图更加美观,可以使用Matplotlib提供的各种样式和美化功能。例如,调整颜色、设置网格、添加标签和标题等。
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, axs = plt.subplots(2, 2, figsize=(12, 8))
axs[0, 0].plot(x, y1, color='r', linestyle='-', linewidth=2)
axs[0, 0].set_title('Sine Wave')
axs[0, 0].grid(True)
axs[0, 1].plot(x, y2, color='b', linestyle='--', linewidth=2)
axs[0, 1].set_title('Cosine Wave')
axs[0, 1].grid(True)
axs[1, 0].scatter(x, y1, color='g')
axs[1, 0].set_title('Sine Scatter')
axs[1, 0].grid(True)
axs[1, 1].bar([1, 2, 3], [3, 2, 1], color='purple')
axs[1, 1].set_title('Bar Chart')
axs[1, 1].grid(True)
fig.suptitle('Various Plots', fontsize=16)
fig.tight_layout(rect=[0, 0, 1, 0.95])
plt.show()
七、子图间的共享轴
在某些情况下,子图之间共享一个轴可以更好地对比数据。subplots
函数提供了sharex
和sharey
参数来实现这一点。
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, axs = plt.subplots(2, 1, figsize=(12, 8), sharex=True)
axs[0].plot(x, y1, color='r', linestyle='-', linewidth=2)
axs[0].set_title('Sine Wave')
axs[0].grid(True)
axs[1].plot(x, y2, color='b', linestyle='--', linewidth=2)
axs[1].set_title('Cosine Wave')
axs[1].grid(True)
fig.suptitle('Shared X-Axis', fontsize=16)
fig.tight_layout(rect=[0, 0, 1, 0.95])
plt.show()
八、子图内嵌
有时候你可能需要在一个子图内嵌入另一个子图,这可以通过axes
对象的inset_axes
方法来实现。
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, ax = plt.subplots(figsize=(8, 6))
ax.plot(x, y, color='r')
ax.set_title('Main Plot')
创建内嵌子图
inset_ax = ax.inset_axes([0.5, 0.5, 0.4, 0.4])
inset_ax.plot(x, np.cos(x), color='b')
inset_ax.set_title('Inset Plot')
plt.show()
九、保存子图
在绘制完子图后,你可能需要将其保存为文件。savefig
函数可以帮助你实现这一点。
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, axs = plt.subplots(2, 1, figsize=(12, 8), sharex=True)
axs[0].plot(x, y1, color='r', linestyle='-', linewidth=2)
axs[0].set_title('Sine Wave')
axs[0].grid(True)
axs[1].plot(x, y2, color='b', linestyle='--', linewidth=2)
axs[1].set_title('Cosine Wave')
axs[1].grid(True)
fig.suptitle('Shared X-Axis', fontsize=16)
fig.tight_layout(rect=[0, 0, 1, 0.95])
保存子图为文件
fig.savefig('subplot_example.png')
plt.show()
十、总结
通过上述方法,你可以灵活地在Python中使用Matplotlib来绘制各种子图。通过使用subplot
和subplots
函数、利用GridSpec
来创建复杂的布局、以及自定义子图的大小和形状,可以满足大多数的绘图需求。此外,结合子图的样式美化、共享轴、内嵌子图以及保存子图的技巧,你可以创建更加专业和美观的图表。希望这些方法和技巧能够帮助你在数据可视化的过程中更加得心应手。
相关问答FAQs:
如何在Python中创建多个子图?
在Python中,使用Matplotlib库可以方便地创建多个子图。你可以使用plt.subplot()
或plt.subplots()
函数来生成子图。plt.subplot()
允许你在一个图形中定义多个子图的布局,而plt.subplots()
则返回一个包含子图的数组,使得对每个子图的操作更加直观和方便。
如何调整子图之间的间距?
在创建多个子图时,调整子图之间的间距可以使用plt.subplots_adjust()
函数。你可以通过参数如left
、right
、top
、bottom
、wspace
和hspace
来精确控制子图的布局,确保图形的可读性和美观性。
如何在子图中添加标题和标签?
为每个子图添加标题和标签可以增强图形的可理解性。使用ax.set_title()
为每个子图设置标题,同时可以使用ax.set_xlabel()
和ax.set_ylabel()
为每个子图添加X轴和Y轴的标签。这样,观众在查看图形时能够更清晰地理解每个子图所展示的信息。
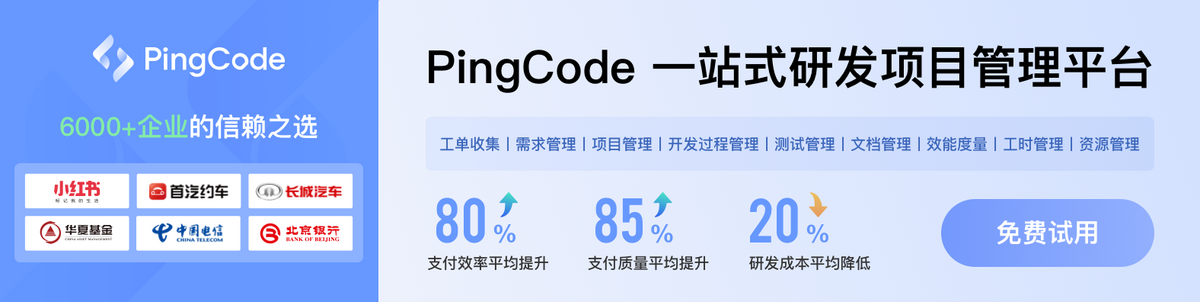